Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / StringBlob.cs / 1 / StringBlob.cs
//---------------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // // Description: // Manages a list of strings stored in an array of chars. This code is // ported from the SAPI source code. Starting from scratch, this code // would not make a lot of sense. However, since grammar generarion must // produce bit per bit equivallent output between the SAPI COM object and // the managed compiler, we must to use it. // // History: // 5/1/2004 jeanfp Created from the Sapi Managed code //--------------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Speech.Internal { ////// Summary description for StringBlob. /// internal class StringBlob { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal StringBlob () { } internal StringBlob (char [] pszStringArray) { int cch = pszStringArray.Length; if (cch > 0) { // First string is always empty. if (pszStringArray [0] != 0) { throw new FormatException (SR.Get (SRID.RecognizerInvalidBinaryGrammar)); } // First pass to copy data and count strings. for (int iPos = 1, iEnd = cch, iStart = 1; iPos < iEnd; iPos++) { if (pszStringArray [iPos] == '\0') { string sWord = new string (pszStringArray, iStart, iPos - iStart); _refStrings.Add (sWord); _offsetStrings.Add (_totalStringSizes); _strings.Add (sWord, ++_cWords); _totalStringSizes += sWord.Length + 1; iStart = iPos + 1; } } } } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region internal Methods // // The ID for a null string is always 0, the ID for subsequent strings is the // index of the string + 1; // internal int Add (string psz, out int idWord) { int offset = 0; idWord = 0; if (!string.IsNullOrEmpty (psz)) { // Check if the string is already in the table if (!_strings.TryGetValue (psz, out idWord)) { System.Diagnostics.Debug.Assert (_strings.Count == _refStrings.Count); // No add it to the string table idWord = ++_cWords; offset = _totalStringSizes; _refStrings.Add (psz); _offsetStrings.Add (offset); _strings.Add (psz, _cWords); _totalStringSizes += psz.Length + 1; } else { offset = OffsetFromId (idWord); } } return offset; } // Returns idWord; use IndexFromId to recover string offset internal int Find (string psz) { // Compatibility the SAPI version if (string.IsNullOrEmpty (psz) || _cWords == 0) { return 0; } // Use the dictionary to find the value int iWord; return _strings.TryGetValue (psz, out iWord) ? iWord : -1; } internal string this [int index] { get { if ((index < 1) || index > _cWords) { throw new InvalidOperationException (); } return _refStrings [index - 1]; } } ////// Only DEBUG code should use this /// /// ///internal string FromOffset (int offset) { int iPos = 1; int iWord = 1; System.Diagnostics.Debug.Assert (offset > 0); if (offset == 1 && _cWords >= 1) { return this [iWord]; } foreach (string s in _refStrings) { iWord++; iPos += s.Length + 1; if (offset == iPos) { return this [iWord]; } } return null; } internal int StringSize () { return _cWords > 0 ? _totalStringSizes : 0; } internal int SerializeSize () { return ((StringSize () * _sizeOfChar + 3) & ~3) / 2; } internal char [] SerializeData () { // force a 0xcccc at the end of the buffer if the length is odd int iEnd = SerializeSize (); char [] aData = new char [iEnd]; // aData [0] is set by the framework to zero int iPos = 1; foreach (string s in _refStrings) { for (int i = 0; i < s.Length; i++) { aData [iPos++] = s [i]; } aData [iPos++] = '\0'; } if (StringSize () % 2 == 1) { aData [iPos++] = (char) 0xCCCC; } System.Diagnostics.Debug.Assert (iEnd == 0 || iPos == SerializeSize ()); return aData; } internal int OffsetFromId (int index) { System.Diagnostics.Debug.Assert (index <= _cWords); if (index > 0) { return _offsetStrings [index - 1]; } return 0; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region internal Properties internal int Count { get { return _cWords; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields // List of words, end-to-end private Dictionary _strings = new Dictionary (); // List of indices in the dictionary of words private List _refStrings = new List (); // List of indices in the dictionary of words private List _offsetStrings = new List (); // Number of words private int _cWords; // Cached value for the total string sizes - The first digit is always zero. private int _totalStringSizes = 1; // .Net is Unicode so 2 bytes per characters private const int _sizeOfChar = 2; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // // // Description: // Manages a list of strings stored in an array of chars. This code is // ported from the SAPI source code. Starting from scratch, this code // would not make a lot of sense. However, since grammar generarion must // produce bit per bit equivallent output between the SAPI COM object and // the managed compiler, we must to use it. // // History: // 5/1/2004 jeanfp Created from the Sapi Managed code //--------------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Speech.Internal { ////// Summary description for StringBlob. /// internal class StringBlob { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal StringBlob () { } internal StringBlob (char [] pszStringArray) { int cch = pszStringArray.Length; if (cch > 0) { // First string is always empty. if (pszStringArray [0] != 0) { throw new FormatException (SR.Get (SRID.RecognizerInvalidBinaryGrammar)); } // First pass to copy data and count strings. for (int iPos = 1, iEnd = cch, iStart = 1; iPos < iEnd; iPos++) { if (pszStringArray [iPos] == '\0') { string sWord = new string (pszStringArray, iStart, iPos - iStart); _refStrings.Add (sWord); _offsetStrings.Add (_totalStringSizes); _strings.Add (sWord, ++_cWords); _totalStringSizes += sWord.Length + 1; iStart = iPos + 1; } } } } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region internal Methods // // The ID for a null string is always 0, the ID for subsequent strings is the // index of the string + 1; // internal int Add (string psz, out int idWord) { int offset = 0; idWord = 0; if (!string.IsNullOrEmpty (psz)) { // Check if the string is already in the table if (!_strings.TryGetValue (psz, out idWord)) { System.Diagnostics.Debug.Assert (_strings.Count == _refStrings.Count); // No add it to the string table idWord = ++_cWords; offset = _totalStringSizes; _refStrings.Add (psz); _offsetStrings.Add (offset); _strings.Add (psz, _cWords); _totalStringSizes += psz.Length + 1; } else { offset = OffsetFromId (idWord); } } return offset; } // Returns idWord; use IndexFromId to recover string offset internal int Find (string psz) { // Compatibility the SAPI version if (string.IsNullOrEmpty (psz) || _cWords == 0) { return 0; } // Use the dictionary to find the value int iWord; return _strings.TryGetValue (psz, out iWord) ? iWord : -1; } internal string this [int index] { get { if ((index < 1) || index > _cWords) { throw new InvalidOperationException (); } return _refStrings [index - 1]; } } ////// Only DEBUG code should use this /// /// ///internal string FromOffset (int offset) { int iPos = 1; int iWord = 1; System.Diagnostics.Debug.Assert (offset > 0); if (offset == 1 && _cWords >= 1) { return this [iWord]; } foreach (string s in _refStrings) { iWord++; iPos += s.Length + 1; if (offset == iPos) { return this [iWord]; } } return null; } internal int StringSize () { return _cWords > 0 ? _totalStringSizes : 0; } internal int SerializeSize () { return ((StringSize () * _sizeOfChar + 3) & ~3) / 2; } internal char [] SerializeData () { // force a 0xcccc at the end of the buffer if the length is odd int iEnd = SerializeSize (); char [] aData = new char [iEnd]; // aData [0] is set by the framework to zero int iPos = 1; foreach (string s in _refStrings) { for (int i = 0; i < s.Length; i++) { aData [iPos++] = s [i]; } aData [iPos++] = '\0'; } if (StringSize () % 2 == 1) { aData [iPos++] = (char) 0xCCCC; } System.Diagnostics.Debug.Assert (iEnd == 0 || iPos == SerializeSize ()); return aData; } internal int OffsetFromId (int index) { System.Diagnostics.Debug.Assert (index <= _cWords); if (index > 0) { return _offsetStrings [index - 1]; } return 0; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region internal Properties internal int Count { get { return _cWords; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields // List of words, end-to-end private Dictionary _strings = new Dictionary (); // List of indices in the dictionary of words private List _refStrings = new List (); // List of indices in the dictionary of words private List _offsetStrings = new List (); // Number of words private int _cWords; // Cached value for the total string sizes - The first digit is always zero. private int _totalStringSizes = 1; // .Net is Unicode so 2 bytes per characters private const int _sizeOfChar = 2; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
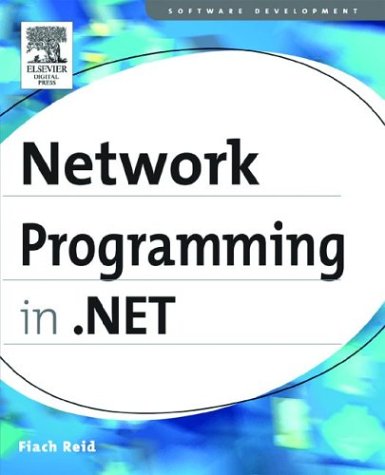
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CellRelation.cs
- GridViewRowPresenter.cs
- BasicAsyncResult.cs
- SubpageParaClient.cs
- EnumerableCollectionView.cs
- ListControl.cs
- DataGridCellsPanel.cs
- ImageIndexEditor.cs
- TextMarkerSource.cs
- ObservableDictionary.cs
- StrongNamePublicKeyBlob.cs
- Monitor.cs
- AnimationClock.cs
- LogicalExpressionTypeConverter.cs
- httpserverutility.cs
- OracleCommand.cs
- ComplexLine.cs
- PathData.cs
- FormatConvertedBitmap.cs
- CursorConverter.cs
- ListControlDataBindingHandler.cs
- WebUtility.cs
- RowVisual.cs
- BamlLocalizabilityResolver.cs
- CanonicalXml.cs
- CompositeActivityCodeGenerator.cs
- CreateParams.cs
- SafeLibraryHandle.cs
- AutomationPropertyInfo.cs
- RegisteredDisposeScript.cs
- RequestContext.cs
- InvokeProviderWrapper.cs
- ItemsPresenter.cs
- PostBackOptions.cs
- XmlSchemaProviderAttribute.cs
- hebrewshape.cs
- AssemblyBuilder.cs
- HttpAsyncResult.cs
- ArrayItemValue.cs
- ReadOnlyNameValueCollection.cs
- AnimationTimeline.cs
- SchemaEntity.cs
- HtmlButton.cs
- ObjectQuery.cs
- SpeakProgressEventArgs.cs
- SQLInt32.cs
- X509ChainPolicy.cs
- SqlDataSourceParameterParser.cs
- Gdiplus.cs
- ImageConverter.cs
- EntityClassGenerator.cs
- NTAccount.cs
- XmlDataImplementation.cs
- EncoderExceptionFallback.cs
- WebPartZone.cs
- Privilege.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- OutOfMemoryException.cs
- OwnerDrawPropertyBag.cs
- ManagementQuery.cs
- SoapReflectionImporter.cs
- FilterQuery.cs
- ScriptManager.cs
- ContentPropertyAttribute.cs
- ParameterBuilder.cs
- Int16Converter.cs
- ListControl.cs
- Point4D.cs
- XPathSelectionIterator.cs
- ToolStripPanelRow.cs
- EntityDataSourceSelectedEventArgs.cs
- WebPartConnectionsEventArgs.cs
- CaseInsensitiveHashCodeProvider.cs
- Int16.cs
- basemetadatamappingvisitor.cs
- Hashtable.cs
- ElementHostAutomationPeer.cs
- ModelFactory.cs
- SqlDataSourceStatusEventArgs.cs
- COAUTHIDENTITY.cs
- CmsInterop.cs
- InitialServerConnectionReader.cs
- StrokeNodeOperations2.cs
- LinqDataSourceView.cs
- DisplayToken.cs
- ColorContext.cs
- SrgsRuleRef.cs
- BitSet.cs
- TreeNodeStyle.cs
- WorkflowCreationContext.cs
- XmlnsDefinitionAttribute.cs
- Focus.cs
- RectangleGeometry.cs
- KeyConverter.cs
- BitmapCacheBrush.cs
- FileUpload.cs
- DataTablePropertyDescriptor.cs
- Converter.cs
- nulltextnavigator.cs
- ScopedKnownTypes.cs