Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Reflection / Emit / ParameterBuilder.cs / 1 / ParameterBuilder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ParameterBuilder ** ** ** ParameterBuilder is used to create/associate parameter information ** ** ===========================================================*/ namespace System.Reflection.Emit { using System.Runtime.InteropServices; using System; using System.Reflection; using System.Security.Permissions; [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_ParameterBuilder))] [System.Runtime.InteropServices.ComVisible(true)] public class ParameterBuilder : _ParameterBuilder { // set ParamMarshal [Obsolete("An alternate API is available: Emit the MarshalAs custom attribute instead. http://go.microsoft.com/fwlink/?linkid=14202")] public virtual void SetMarshal(UnmanagedMarshal unmanagedMarshal) { if (unmanagedMarshal == null) { throw new ArgumentNullException("unmanagedMarshal"); } byte [] ubMarshal = unmanagedMarshal.InternalGetBytes(); TypeBuilder.InternalSetMarshalInfo( m_methodBuilder.GetModule(), m_pdToken.Token, ubMarshal, ubMarshal.Length); } // Set the default value of the parameter public virtual void SetConstant(Object defaultValue) { TypeBuilder.SetConstantValue( m_methodBuilder.GetModule(), m_pdToken.Token, m_iPosition == 0 ? m_methodBuilder.m_returnType : m_methodBuilder.m_parameterTypes[m_iPosition-1], defaultValue); } // Use this function if client decides to form the custom attribute blob themselves [System.Runtime.InteropServices.ComVisible(true)] public void SetCustomAttribute(ConstructorInfo con, byte[] binaryAttribute) { if (con == null) throw new ArgumentNullException("con"); if (binaryAttribute == null) throw new ArgumentNullException("binaryAttribute"); TypeBuilder.InternalCreateCustomAttribute( m_pdToken.Token, ((ModuleBuilder )m_methodBuilder.GetModule()).GetConstructorToken(con).Token, binaryAttribute, m_methodBuilder.GetModule(), false); } // Use this function if client wishes to build CustomAttribute using CustomAttributeBuilder public void SetCustomAttribute(CustomAttributeBuilder customBuilder) { if (customBuilder == null) { throw new ArgumentNullException("customBuilder"); } customBuilder.CreateCustomAttribute((ModuleBuilder) (m_methodBuilder .GetModule()), m_pdToken.Token); } //******************************* // Make a private constructor so these cannot be constructed externally. //******************************* private ParameterBuilder() {} internal ParameterBuilder( MethodBuilder methodBuilder, int sequence, ParameterAttributes attributes, String strParamName) // can be NULL string { m_iPosition = sequence; m_strParamName = strParamName; m_methodBuilder = methodBuilder; m_strParamName = strParamName; m_attributes = attributes; m_pdToken = new ParameterToken( TypeBuilder.InternalSetParamInfo( m_methodBuilder.GetModule(), m_methodBuilder.GetToken().Token, sequence, attributes, strParamName)); } public virtual ParameterToken GetToken() { return m_pdToken; } void _ParameterBuilder.GetTypeInfoCount(out uint pcTInfo) { throw new NotImplementedException(); } void _ParameterBuilder.GetTypeInfo(uint iTInfo, uint lcid, IntPtr ppTInfo) { throw new NotImplementedException(); } void _ParameterBuilder.GetIDsOfNames([In] ref Guid riid, IntPtr rgszNames, uint cNames, uint lcid, IntPtr rgDispId) { throw new NotImplementedException(); } void _ParameterBuilder.Invoke(uint dispIdMember, [In] ref Guid riid, uint lcid, short wFlags, IntPtr pDispParams, IntPtr pVarResult, IntPtr pExcepInfo, IntPtr puArgErr) { throw new NotImplementedException(); } internal virtual int MetadataTokenInternal { get { return m_pdToken.Token; } } public virtual String Name { get {return m_strParamName;} } public virtual int Position { get {return m_iPosition;} } // < public virtual int Attributes { get {return (int) m_attributes;} } public bool IsIn { get {return ((m_attributes & ParameterAttributes.In) != 0);} } public bool IsOut { get {return ((m_attributes & ParameterAttributes.Out) != 0);} } public bool IsOptional { get {return ((m_attributes & ParameterAttributes.Optional) != 0);} } private String m_strParamName; private int m_iPosition; private ParameterAttributes m_attributes; private MethodBuilder m_methodBuilder; private ParameterToken m_pdToken; } }
Link Menu
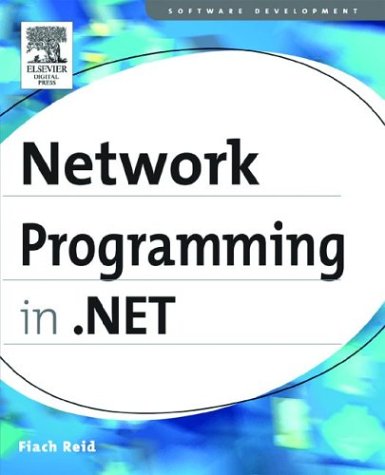
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlInternalConnectionTds.cs
- AttachedPropertyMethodSelector.cs
- Constants.cs
- EntityWrapperFactory.cs
- SyndicationDeserializer.cs
- ProjectionPath.cs
- VirtualizedItemPattern.cs
- HttpHeaderCollection.cs
- Utils.cs
- ApplicationCommands.cs
- DataGridColumnHeaderCollection.cs
- RuleSettingsCollection.cs
- ISCIIEncoding.cs
- GatewayDefinition.cs
- Vector3DCollectionValueSerializer.cs
- _Rfc2616CacheValidators.cs
- ProxyHelper.cs
- TiffBitmapEncoder.cs
- CommandDesigner.cs
- WaitHandle.cs
- PatternMatcher.cs
- SqlHelper.cs
- ProjectionPlan.cs
- XmlCharacterData.cs
- ReverseInheritProperty.cs
- DataGridItemCollection.cs
- StringBuilder.cs
- RegionInfo.cs
- ToolTipService.cs
- MembershipSection.cs
- DbParameterHelper.cs
- BrowserCapabilitiesFactory.cs
- Thickness.cs
- SelectorItemAutomationPeer.cs
- ResizeGrip.cs
- UshortList2.cs
- ClientUrlResolverWrapper.cs
- SevenBitStream.cs
- DisableDpiAwarenessAttribute.cs
- StrokeDescriptor.cs
- BamlRecordWriter.cs
- XamlWrappingReader.cs
- ZipIOLocalFileDataDescriptor.cs
- ButtonBaseAutomationPeer.cs
- LabelLiteral.cs
- SymmetricKeyWrap.cs
- ColumnMapVisitor.cs
- EndGetFileNameFromUserRequest.cs
- ResumeStoryboard.cs
- DeviceOverridableAttribute.cs
- BindingBase.cs
- EnterpriseServicesHelper.cs
- ExternalCalls.cs
- QueryPageSettingsEventArgs.cs
- UnmanagedMarshal.cs
- NativeWrapper.cs
- Path.cs
- Attributes.cs
- ContentIterators.cs
- StreamHelper.cs
- ToolCreatedEventArgs.cs
- MetadataCache.cs
- EFColumnProvider.cs
- TextTreeObjectNode.cs
- AssertSection.cs
- JsonCollectionDataContract.cs
- XamlSerializationHelper.cs
- log.cs
- ListViewUpdatedEventArgs.cs
- SubclassTypeValidatorAttribute.cs
- SystemEvents.cs
- XmlMapping.cs
- FramingDecoders.cs
- DataGridCaption.cs
- LoginName.cs
- CallSiteOps.cs
- DrawingGroupDrawingContext.cs
- BrowserCapabilitiesFactoryBase.cs
- HyperLinkField.cs
- _LoggingObject.cs
- ToolStripItemImageRenderEventArgs.cs
- CurrencyWrapper.cs
- FileRecordSequenceHelper.cs
- Lookup.cs
- DataColumn.cs
- XmlStreamedByteStreamReader.cs
- EntityDescriptor.cs
- RegexCompiler.cs
- WebPartManager.cs
- DBCommandBuilder.cs
- ExpressionBindingsDialog.cs
- IndentedTextWriter.cs
- XmlSchemaInferenceException.cs
- ObjectSet.cs
- SchemaRegistration.cs
- BulletChrome.cs
- arabicshape.cs
- PermissionToken.cs
- TemplateEditingVerb.cs
- TeredoHelper.cs