Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / ToolTipService.cs / 1305600 / ToolTipService.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Input; using System.Windows.Media; using MS.Internal.KnownBoxes; using System.ComponentModel; namespace System.Windows.Controls { ////// Service class that provides the system implementation for displaying ToolTips. /// public static class ToolTipService { #region Attached Properties ////// The DependencyProperty for the ToolTip property. /// public static readonly DependencyProperty ToolTipProperty = DependencyProperty.RegisterAttached( "ToolTip", // Name typeof(object), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata((object) null)); ////// Gets the value of the ToolTip property on the specified object. /// /// The object on which to query the ToolTip property. ///The value of the ToolTip property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static object GetToolTip(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return element.GetValue(ToolTipProperty); } ////// Sets the ToolTip property on the specified object. /// /// The object on which to set the ToolTip property. /// /// The value of the ToolTip property. If the value is of type ToolTip, then /// that is the ToolTip that will be used (without any modification). If the value /// is of any other type, then that value will be used as the content for a ToolTip /// provided by this service, and the other attached properties of this service /// will be used to configure the ToolTip. /// public static void SetToolTip(DependencyObject element, object value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ToolTipProperty, value); } ////// The DependencyProperty for the HorizontalOffset property. /// public static readonly DependencyProperty HorizontalOffsetProperty = DependencyProperty.RegisterAttached("HorizontalOffset", // Name typeof(double), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(0d)); // Default Value ////// Gets the value of the HorizontalOffset property. /// /// The object on which to query the property. ///The value of the property. [TypeConverter(typeof(LengthConverter))] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static double GetHorizontalOffset(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(HorizontalOffsetProperty); } ////// Sets the value of the HorizontalOffset property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetHorizontalOffset(DependencyObject element, double value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HorizontalOffsetProperty, value); } ////// The DependencyProperty for the VerticalOffset property. /// public static readonly DependencyProperty VerticalOffsetProperty = DependencyProperty.RegisterAttached("VerticalOffset", // Name typeof(double), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(0d)); // Default Value ////// Gets the value of the VerticalOffset property. /// /// The object on which to query the property. ///The value of the property. [TypeConverter(typeof(LengthConverter))] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static double GetVerticalOffset(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(VerticalOffsetProperty); } ////// Sets the value of the VerticalOffset property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetVerticalOffset(DependencyObject element, double value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(VerticalOffsetProperty, value); } ////// The DependencyProperty for HasDropShadow /// public static readonly DependencyProperty HasDropShadowProperty = DependencyProperty.RegisterAttached("HasDropShadow", // Name typeof(bool), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); // Default Value ////// Gets the value of the HasDropShadow property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetHasDropShadow(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(HasDropShadowProperty); } ////// Sets the value of the HasDropShadow property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetHasDropShadow(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HasDropShadowProperty, BooleanBoxes.Box(value)); } ////// The DependencyProperty for the PlacementTarget property. /// public static readonly DependencyProperty PlacementTargetProperty = DependencyProperty.RegisterAttached("PlacementTarget", // Name typeof(UIElement), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata((UIElement)null)); // Default Value ////// Gets the value of the PlacementTarget property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static UIElement GetPlacementTarget(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (UIElement)element.GetValue(PlacementTargetProperty); } ////// Sets the value of the PlacementTarget property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacementTarget(DependencyObject element, UIElement value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementTargetProperty, value); } ////// The DependencyProperty for the PlacementRectangle property. /// public static readonly DependencyProperty PlacementRectangleProperty = DependencyProperty.RegisterAttached("PlacementRectangle", // Name typeof(Rect), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(Rect.Empty)); // Default Value ////// Gets the value of the PlacementRectangle property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static Rect GetPlacementRectangle(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (Rect)element.GetValue(PlacementRectangleProperty); } ////// Sets the value of the PlacementRectangle property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacementRectangle(DependencyObject element, Rect value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementRectangleProperty, value); } ////// The DependencyProperty for the Placement property. /// public static readonly DependencyProperty PlacementProperty = DependencyProperty.RegisterAttached("Placement", // Name typeof(PlacementMode), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(PlacementMode.Mouse)); // Default Value ////// Gets the value of the Placement property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static PlacementMode GetPlacement(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (PlacementMode)element.GetValue(PlacementProperty); } ////// Sets the value of the Placement property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacement(DependencyObject element, PlacementMode value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementProperty, value); } ////// The DependencyProperty for the ShowOnDisabled property. /// public static readonly DependencyProperty ShowOnDisabledProperty = DependencyProperty.RegisterAttached("ShowOnDisabled", // Name typeof(bool), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); // Default Value ////// Gets the value of the ShowOnDisabled property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetShowOnDisabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(ShowOnDisabledProperty); } ////// Sets the value of the ShowOnDisabled property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetShowOnDisabled(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ShowOnDisabledProperty, BooleanBoxes.Box(value)); } ////// Read-only Key Token for the IsOpen property. /// private static readonly DependencyPropertyKey IsOpenPropertyKey = DependencyProperty.RegisterAttachedReadOnly("IsOpen", // Name typeof(bool), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); // Default Value ////// The DependencyProperty for the IsOpen property. /// public static readonly DependencyProperty IsOpenProperty = IsOpenPropertyKey.DependencyProperty; ////// Gets the value of the IsOpen property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetIsOpen(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(IsOpenProperty); } ////// Sets the value of the IsOpen property. /// /// The object on which to set the value. /// The desired value of the property. private static void SetIsOpen(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsOpenPropertyKey, BooleanBoxes.Box(value)); } ////// The DependencyProperty for the IsEnabled property. /// public static readonly DependencyProperty IsEnabledProperty = DependencyProperty.RegisterAttached("IsEnabled", // Name typeof(bool), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.TrueBox)); // Default Value ////// Gets the value of the IsEnabled property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetIsEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(IsEnabledProperty); } ////// Sets the value of the IsEnabled property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetIsEnabled(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsEnabledProperty, BooleanBoxes.Box(value)); } private static bool PositiveValueValidation(object o) { return ((int)o) >= 0; } ////// The DependencyProperty for the ShowDuration property. /// public static readonly DependencyProperty ShowDurationProperty = DependencyProperty.RegisterAttached("ShowDuration", // Name typeof(int), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(5000), // Default Value new ValidateValueCallback(PositiveValueValidation)); // Value validation ////// Gets the value of the ShowDuration property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetShowDuration(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(ShowDurationProperty); } ////// Sets the value of the ShowDuration property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetShowDuration(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ShowDurationProperty, value); } ////// The DependencyProperty for the InitialShowDelay property. /// public static readonly DependencyProperty InitialShowDelayProperty = DependencyProperty.RegisterAttached("InitialShowDelay", // Name typeof(int), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(SystemParameters.MouseHoverTimeMilliseconds), // Default Value new ValidateValueCallback(PositiveValueValidation)); // Value validation ////// Gets the value of the InitialShowDelay property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetInitialShowDelay(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(InitialShowDelayProperty); } ////// Sets the value of the InitialShowDelay property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetInitialShowDelay(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(InitialShowDelayProperty, value); } ////// The DependencyProperty for the BetweenShowDelay property. /// public static readonly DependencyProperty BetweenShowDelayProperty = DependencyProperty.RegisterAttached("BetweenShowDelay", // Name typeof(int), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(100), // Default Value new ValidateValueCallback(PositiveValueValidation)); // Value validation ////// Gets the value of the BetweenShowDelay property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetBetweenShowDelay(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(BetweenShowDelayProperty); } ////// Sets the value of the BetweenShowDelay property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetBetweenShowDelay(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(BetweenShowDelayProperty, value); } #endregion #region Events ////// The event raised when a ToolTip is going to be shown on an element. /// /// Mark the event as handled if manually showing a ToolTip. /// /// Replacing the value of the ToolTip property is allowed /// (example: for delay-loading). Do not mark the event as handled /// in this case if the system is to show the ToolTip. /// public static readonly RoutedEvent ToolTipOpeningEvent = EventManager.RegisterRoutedEvent("ToolTipOpening", RoutingStrategy.Direct, typeof(ToolTipEventHandler), typeof(ToolTipService)); ////// The event raised when a ToolTip on an element that was shown /// should now be hidden. /// public static readonly RoutedEvent ToolTipClosingEvent = EventManager.RegisterRoutedEvent("ToolTipClosing", RoutingStrategy.Direct, typeof(ToolTipEventHandler), typeof(ToolTipService)); #endregion #region Implementation internal static readonly RoutedEvent FindToolTipEvent = EventManager.RegisterRoutedEvent("FindToolTip", RoutingStrategy.Bubble, typeof(FindToolTipEventHandler), typeof(ToolTipService)); static ToolTipService() { EventManager.RegisterClassHandler(typeof(UIElement), FindToolTipEvent, new FindToolTipEventHandler(OnFindToolTip)); EventManager.RegisterClassHandler(typeof(ContentElement), FindToolTipEvent, new FindToolTipEventHandler(OnFindToolTip)); EventManager.RegisterClassHandler(typeof(UIElement3D), FindToolTipEvent, new FindToolTipEventHandler(OnFindToolTip)); } private static void OnFindToolTip(object sender, FindToolTipEventArgs e) { if (e.TargetElement == null) { DependencyObject o = sender as DependencyObject; if (o != null) { if (PopupControlService.Current.StopLookingForToolTip(o)) { // Stop looking e.Handled = true; e.KeepCurrentActive = true; } else { if (ToolTipIsEnabled(o)) { // Store for later e.TargetElement = o; e.Handled = true; } } } } } private static bool ToolTipIsEnabled(DependencyObject o) { if ((GetToolTip(o) != null) && GetIsEnabled(o)) { if (PopupControlService.IsElementEnabled(o) || GetShowOnDisabled(o)) { return true; } } return false; } #endregion } ////// The callback type for the events when a ToolTip should open or close. /// public delegate void ToolTipEventHandler(object sender, ToolTipEventArgs e); ////// Event arguments for the events when a ToolTip should open or close. /// public sealed class ToolTipEventArgs : RoutedEventArgs { ////// Called internally to create opening or closing event arguments. /// /// Whether this is the opening or closing event. internal ToolTipEventArgs(bool opening) { if (opening) { RoutedEvent = ToolTipService.ToolTipOpeningEvent; } else { RoutedEvent = ToolTipService.ToolTipClosingEvent; } } ////// Invokes the event handler. /// /// The delegate to call. /// The target of the event. protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { ToolTipEventHandler handler = (ToolTipEventHandler)genericHandler; handler(genericTarget, this); } } internal delegate void FindToolTipEventHandler(object sender, FindToolTipEventArgs e); internal sealed class FindToolTipEventArgs : RoutedEventArgs { internal FindToolTipEventArgs() { RoutedEvent = ToolTipService.FindToolTipEvent; } internal DependencyObject TargetElement { get { return _targetElement; } set { _targetElement = value; } } internal bool KeepCurrentActive { get { return _keepCurrentActive; } set { _keepCurrentActive = value; } } ////// Invokes the event handler. /// /// The delegate to call. /// The target of the event. protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { FindToolTipEventHandler handler = (FindToolTipEventHandler)genericHandler; handler(genericTarget, this); } private DependencyObject _targetElement; private bool _keepCurrentActive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Input; using System.Windows.Media; using MS.Internal.KnownBoxes; using System.ComponentModel; namespace System.Windows.Controls { ////// Service class that provides the system implementation for displaying ToolTips. /// public static class ToolTipService { #region Attached Properties ////// The DependencyProperty for the ToolTip property. /// public static readonly DependencyProperty ToolTipProperty = DependencyProperty.RegisterAttached( "ToolTip", // Name typeof(object), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata((object) null)); ////// Gets the value of the ToolTip property on the specified object. /// /// The object on which to query the ToolTip property. ///The value of the ToolTip property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static object GetToolTip(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return element.GetValue(ToolTipProperty); } ////// Sets the ToolTip property on the specified object. /// /// The object on which to set the ToolTip property. /// /// The value of the ToolTip property. If the value is of type ToolTip, then /// that is the ToolTip that will be used (without any modification). If the value /// is of any other type, then that value will be used as the content for a ToolTip /// provided by this service, and the other attached properties of this service /// will be used to configure the ToolTip. /// public static void SetToolTip(DependencyObject element, object value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ToolTipProperty, value); } ////// The DependencyProperty for the HorizontalOffset property. /// public static readonly DependencyProperty HorizontalOffsetProperty = DependencyProperty.RegisterAttached("HorizontalOffset", // Name typeof(double), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(0d)); // Default Value ////// Gets the value of the HorizontalOffset property. /// /// The object on which to query the property. ///The value of the property. [TypeConverter(typeof(LengthConverter))] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static double GetHorizontalOffset(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(HorizontalOffsetProperty); } ////// Sets the value of the HorizontalOffset property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetHorizontalOffset(DependencyObject element, double value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HorizontalOffsetProperty, value); } ////// The DependencyProperty for the VerticalOffset property. /// public static readonly DependencyProperty VerticalOffsetProperty = DependencyProperty.RegisterAttached("VerticalOffset", // Name typeof(double), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(0d)); // Default Value ////// Gets the value of the VerticalOffset property. /// /// The object on which to query the property. ///The value of the property. [TypeConverter(typeof(LengthConverter))] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static double GetVerticalOffset(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(VerticalOffsetProperty); } ////// Sets the value of the VerticalOffset property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetVerticalOffset(DependencyObject element, double value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(VerticalOffsetProperty, value); } ////// The DependencyProperty for HasDropShadow /// public static readonly DependencyProperty HasDropShadowProperty = DependencyProperty.RegisterAttached("HasDropShadow", // Name typeof(bool), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); // Default Value ////// Gets the value of the HasDropShadow property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetHasDropShadow(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(HasDropShadowProperty); } ////// Sets the value of the HasDropShadow property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetHasDropShadow(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HasDropShadowProperty, BooleanBoxes.Box(value)); } ////// The DependencyProperty for the PlacementTarget property. /// public static readonly DependencyProperty PlacementTargetProperty = DependencyProperty.RegisterAttached("PlacementTarget", // Name typeof(UIElement), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata((UIElement)null)); // Default Value ////// Gets the value of the PlacementTarget property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static UIElement GetPlacementTarget(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (UIElement)element.GetValue(PlacementTargetProperty); } ////// Sets the value of the PlacementTarget property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacementTarget(DependencyObject element, UIElement value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementTargetProperty, value); } ////// The DependencyProperty for the PlacementRectangle property. /// public static readonly DependencyProperty PlacementRectangleProperty = DependencyProperty.RegisterAttached("PlacementRectangle", // Name typeof(Rect), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(Rect.Empty)); // Default Value ////// Gets the value of the PlacementRectangle property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static Rect GetPlacementRectangle(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (Rect)element.GetValue(PlacementRectangleProperty); } ////// Sets the value of the PlacementRectangle property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacementRectangle(DependencyObject element, Rect value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementRectangleProperty, value); } ////// The DependencyProperty for the Placement property. /// public static readonly DependencyProperty PlacementProperty = DependencyProperty.RegisterAttached("Placement", // Name typeof(PlacementMode), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(PlacementMode.Mouse)); // Default Value ////// Gets the value of the Placement property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static PlacementMode GetPlacement(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (PlacementMode)element.GetValue(PlacementProperty); } ////// Sets the value of the Placement property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacement(DependencyObject element, PlacementMode value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementProperty, value); } ////// The DependencyProperty for the ShowOnDisabled property. /// public static readonly DependencyProperty ShowOnDisabledProperty = DependencyProperty.RegisterAttached("ShowOnDisabled", // Name typeof(bool), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); // Default Value ////// Gets the value of the ShowOnDisabled property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetShowOnDisabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(ShowOnDisabledProperty); } ////// Sets the value of the ShowOnDisabled property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetShowOnDisabled(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ShowOnDisabledProperty, BooleanBoxes.Box(value)); } ////// Read-only Key Token for the IsOpen property. /// private static readonly DependencyPropertyKey IsOpenPropertyKey = DependencyProperty.RegisterAttachedReadOnly("IsOpen", // Name typeof(bool), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); // Default Value ////// The DependencyProperty for the IsOpen property. /// public static readonly DependencyProperty IsOpenProperty = IsOpenPropertyKey.DependencyProperty; ////// Gets the value of the IsOpen property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetIsOpen(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(IsOpenProperty); } ////// Sets the value of the IsOpen property. /// /// The object on which to set the value. /// The desired value of the property. private static void SetIsOpen(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsOpenPropertyKey, BooleanBoxes.Box(value)); } ////// The DependencyProperty for the IsEnabled property. /// public static readonly DependencyProperty IsEnabledProperty = DependencyProperty.RegisterAttached("IsEnabled", // Name typeof(bool), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.TrueBox)); // Default Value ////// Gets the value of the IsEnabled property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetIsEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(IsEnabledProperty); } ////// Sets the value of the IsEnabled property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetIsEnabled(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsEnabledProperty, BooleanBoxes.Box(value)); } private static bool PositiveValueValidation(object o) { return ((int)o) >= 0; } ////// The DependencyProperty for the ShowDuration property. /// public static readonly DependencyProperty ShowDurationProperty = DependencyProperty.RegisterAttached("ShowDuration", // Name typeof(int), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(5000), // Default Value new ValidateValueCallback(PositiveValueValidation)); // Value validation ////// Gets the value of the ShowDuration property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetShowDuration(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(ShowDurationProperty); } ////// Sets the value of the ShowDuration property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetShowDuration(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ShowDurationProperty, value); } ////// The DependencyProperty for the InitialShowDelay property. /// public static readonly DependencyProperty InitialShowDelayProperty = DependencyProperty.RegisterAttached("InitialShowDelay", // Name typeof(int), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(SystemParameters.MouseHoverTimeMilliseconds), // Default Value new ValidateValueCallback(PositiveValueValidation)); // Value validation ////// Gets the value of the InitialShowDelay property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetInitialShowDelay(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(InitialShowDelayProperty); } ////// Sets the value of the InitialShowDelay property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetInitialShowDelay(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(InitialShowDelayProperty, value); } ////// The DependencyProperty for the BetweenShowDelay property. /// public static readonly DependencyProperty BetweenShowDelayProperty = DependencyProperty.RegisterAttached("BetweenShowDelay", // Name typeof(int), // Type typeof(ToolTipService), // Owner new FrameworkPropertyMetadata(100), // Default Value new ValidateValueCallback(PositiveValueValidation)); // Value validation ////// Gets the value of the BetweenShowDelay property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static int GetBetweenShowDelay(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (int)element.GetValue(BetweenShowDelayProperty); } ////// Sets the value of the BetweenShowDelay property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetBetweenShowDelay(DependencyObject element, int value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(BetweenShowDelayProperty, value); } #endregion #region Events ////// The event raised when a ToolTip is going to be shown on an element. /// /// Mark the event as handled if manually showing a ToolTip. /// /// Replacing the value of the ToolTip property is allowed /// (example: for delay-loading). Do not mark the event as handled /// in this case if the system is to show the ToolTip. /// public static readonly RoutedEvent ToolTipOpeningEvent = EventManager.RegisterRoutedEvent("ToolTipOpening", RoutingStrategy.Direct, typeof(ToolTipEventHandler), typeof(ToolTipService)); ////// The event raised when a ToolTip on an element that was shown /// should now be hidden. /// public static readonly RoutedEvent ToolTipClosingEvent = EventManager.RegisterRoutedEvent("ToolTipClosing", RoutingStrategy.Direct, typeof(ToolTipEventHandler), typeof(ToolTipService)); #endregion #region Implementation internal static readonly RoutedEvent FindToolTipEvent = EventManager.RegisterRoutedEvent("FindToolTip", RoutingStrategy.Bubble, typeof(FindToolTipEventHandler), typeof(ToolTipService)); static ToolTipService() { EventManager.RegisterClassHandler(typeof(UIElement), FindToolTipEvent, new FindToolTipEventHandler(OnFindToolTip)); EventManager.RegisterClassHandler(typeof(ContentElement), FindToolTipEvent, new FindToolTipEventHandler(OnFindToolTip)); EventManager.RegisterClassHandler(typeof(UIElement3D), FindToolTipEvent, new FindToolTipEventHandler(OnFindToolTip)); } private static void OnFindToolTip(object sender, FindToolTipEventArgs e) { if (e.TargetElement == null) { DependencyObject o = sender as DependencyObject; if (o != null) { if (PopupControlService.Current.StopLookingForToolTip(o)) { // Stop looking e.Handled = true; e.KeepCurrentActive = true; } else { if (ToolTipIsEnabled(o)) { // Store for later e.TargetElement = o; e.Handled = true; } } } } } private static bool ToolTipIsEnabled(DependencyObject o) { if ((GetToolTip(o) != null) && GetIsEnabled(o)) { if (PopupControlService.IsElementEnabled(o) || GetShowOnDisabled(o)) { return true; } } return false; } #endregion } ////// The callback type for the events when a ToolTip should open or close. /// public delegate void ToolTipEventHandler(object sender, ToolTipEventArgs e); ////// Event arguments for the events when a ToolTip should open or close. /// public sealed class ToolTipEventArgs : RoutedEventArgs { ////// Called internally to create opening or closing event arguments. /// /// Whether this is the opening or closing event. internal ToolTipEventArgs(bool opening) { if (opening) { RoutedEvent = ToolTipService.ToolTipOpeningEvent; } else { RoutedEvent = ToolTipService.ToolTipClosingEvent; } } ////// Invokes the event handler. /// /// The delegate to call. /// The target of the event. protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { ToolTipEventHandler handler = (ToolTipEventHandler)genericHandler; handler(genericTarget, this); } } internal delegate void FindToolTipEventHandler(object sender, FindToolTipEventArgs e); internal sealed class FindToolTipEventArgs : RoutedEventArgs { internal FindToolTipEventArgs() { RoutedEvent = ToolTipService.FindToolTipEvent; } internal DependencyObject TargetElement { get { return _targetElement; } set { _targetElement = value; } } internal bool KeepCurrentActive { get { return _keepCurrentActive; } set { _keepCurrentActive = value; } } ////// Invokes the event handler. /// /// The delegate to call. /// The target of the event. protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { FindToolTipEventHandler handler = (FindToolTipEventHandler)genericHandler; handler(genericTarget, this); } private DependencyObject _targetElement; private bool _keepCurrentActive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
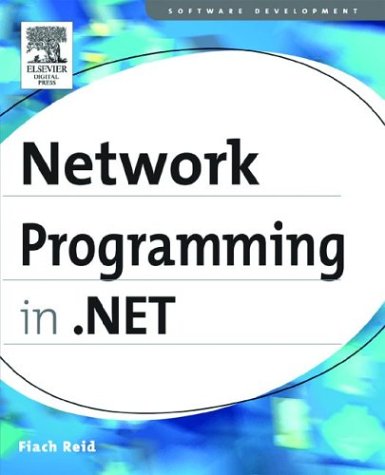
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceContainer.cs
- Column.cs
- CompensationTokenData.cs
- OptimisticConcurrencyException.cs
- CmsInterop.cs
- TripleDES.cs
- TableLayoutRowStyleCollection.cs
- ZipIOLocalFileDataDescriptor.cs
- CompatibleComparer.cs
- MaskedTextBoxDesignerActionList.cs
- SyntaxCheck.cs
- ComplexPropertyEntry.cs
- DSASignatureDeformatter.cs
- ErrorTableItemStyle.cs
- Assembly.cs
- PerformanceCounterCategory.cs
- ZipIOLocalFileBlock.cs
- TransportConfigurationTypeElement.cs
- RegisteredScript.cs
- SoapAttributeAttribute.cs
- InstanceHandleReference.cs
- RTLAwareMessageBox.cs
- HTTPNotFoundHandler.cs
- MimeObjectFactory.cs
- OutArgumentConverter.cs
- WindowsFormsHostAutomationPeer.cs
- QilLoop.cs
- IntPtr.cs
- GroupItemAutomationPeer.cs
- _ServiceNameStore.cs
- mediaeventshelper.cs
- DataGridTablesFactory.cs
- SubqueryRules.cs
- ProviderConnectionPoint.cs
- ScrollChrome.cs
- WebPartCatalogAddVerb.cs
- Asn1IntegerConverter.cs
- FactoryGenerator.cs
- SectionXmlInfo.cs
- ReadingWritingEntityEventArgs.cs
- FolderLevelBuildProviderCollection.cs
- SafeMILHandle.cs
- ComponentCollection.cs
- XsltLibrary.cs
- ReadOnlyHierarchicalDataSource.cs
- brushes.cs
- SelectionChangedEventArgs.cs
- TimeZone.cs
- DataTableClearEvent.cs
- GeometryModel3D.cs
- X509RecipientCertificateClientElement.cs
- ToolBarOverflowPanel.cs
- VariableQuery.cs
- CompositeDataBoundControl.cs
- EntityExpressionVisitor.cs
- VectorKeyFrameCollection.cs
- ReceiveActivityValidator.cs
- Number.cs
- ChangeTracker.cs
- filewebrequest.cs
- ProfilePropertyNameValidator.cs
- GridView.cs
- SqlUtils.cs
- COM2PropertyBuilderUITypeEditor.cs
- ViewValidator.cs
- Propagator.Evaluator.cs
- XmlWriterSettings.cs
- FileSecurity.cs
- ConnectionPoint.cs
- AmbientEnvironment.cs
- ResourceDictionaryCollection.cs
- DeadCharTextComposition.cs
- SchemaImporterExtensionsSection.cs
- XmlUtil.cs
- _NegoState.cs
- SrgsRuleRef.cs
- TileBrush.cs
- InfoCardPolicy.cs
- DynamicResourceExtension.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- DataGridViewUtilities.cs
- GridView.cs
- GridItem.cs
- ReadOnlyMetadataCollection.cs
- DataObject.cs
- HttpContext.cs
- _AutoWebProxyScriptEngine.cs
- XAMLParseException.cs
- IndexedGlyphRun.cs
- BookmarkTable.cs
- SafeEventLogReadHandle.cs
- TextElementCollection.cs
- HttpModuleActionCollection.cs
- SessionPageStatePersister.cs
- Debug.cs
- ProviderBase.cs
- Debugger.cs
- CacheAxisQuery.cs
- XmlWriterSettings.cs
- DrawItemEvent.cs