Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Design / system / Data / EntityModel / Emitters / Utils.cs / 1 / Utils.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Reflection; namespace System.Data.EntityModel.Emitters { ////// Summary description for Utils /// internal static class Utils { #region Static Fields public const string WebFrameworkNamespace = "System.Data.Services.Client"; public const string WebFrameworkCommonNamespace = "System.Data.Services.Common"; public const string AdoFrameworkNamespace = "System.Data.Objects"; public const string AdoFrameworkDataClassesNamespace = "System.Data.Objects.DataClasses"; public const string AdoFrameworkMetadataEdmNamespace = "System.Data.Metadata.Edm"; public const string AdoEntityClientNamespace = "System.Data.EntityClient"; public const string SetValidValueMethodName = "SetValidValue"; public const string ReportPropertyChangingMethodName = "ReportPropertyChanging"; public const string ReportPropertyChangedMethodName = "ReportPropertyChanged"; public const string GetValidValueMethodName = "GetValidValue"; public const string VerifyComplexObjectIsNotNullName = "VerifyComplexObjectIsNotNull"; // this is the list of keywords we check against for names. // If a name matches this keyword we prefix it, this is only used when creating parameter names from property, etc. names(see FixKeyword) private static string[] _keywords = new string[] { "class", "event", }; // to guarantee uniqueness these must all be unique, begin with and end with an underscore and not contain internal underscores private static string[] _privateMemberPrefixes = new string[(int)PrivateMemberPrefixId.Count] { "_", "_Initialize_", "PropertyInfo", "_pi", }; // suffix that is added to field names to create a boolean field used to indicate whether or // not a complex property has been explicitly initialized private static string _complexPropertyInitializedSuffix = "Initialized"; private static List> _typeReservedNames = InitializeTypeReservedNames(); /// /// Initialize some statics that cannot be initialized in member declaration... /// static List> InitializeTypeReservedNames() { Dictionary typeReservedNames = new Dictionary (StringComparer.Ordinal); const BindingFlags bindingFlags = BindingFlags.FlattenHierarchy | BindingFlags.NonPublic | BindingFlags.Public | BindingFlags.Instance | BindingFlags.Static; foreach (MemberInfo member in typeof(object).GetMembers(bindingFlags)) { if (ShouldReserveName(member)) { if (!typeReservedNames.ContainsKey(member.Name)) { typeReservedNames.Add(member.Name, null); } } } List > pairs = new List >(); foreach (KeyValuePair pair in typeReservedNames) { pairs.Add(pair); } return pairs; } private static bool ShouldReserveName(MemberInfo member) { if (member is EventInfo) { return ShouldReserveName((EventInfo)member); } else if (member is FieldInfo) { return ShouldReserveName((FieldInfo)member); } else if (member is MethodBase) { return ShouldReserveName((MethodBase)member); } else if (member is PropertyInfo) { return ShouldReserveName((PropertyInfo)member); } else { Debug.Assert(member is Type, "Did you add a new type of member?"); return ShouldReserveName((Type)member); } } private static bool ShouldReserveName(EventInfo member) { bool hasNonPrivate = false; MethodInfo miAdd = member.GetAddMethod(); if (miAdd != null) { hasNonPrivate |= ShouldReserveName(miAdd, false); } MethodInfo miRemove = member.GetRemoveMethod(); if (miRemove != null) { hasNonPrivate |= ShouldReserveName(miRemove, false); } return hasNonPrivate; } private static bool ShouldReserveName(PropertyInfo member) { bool hasNonPrivate = false; MethodInfo miSet = member.GetSetMethod(); if (miSet != null) { hasNonPrivate |= ShouldReserveName(miSet, false); } MethodInfo miGet = member.GetGetMethod(); if (miGet != null) { hasNonPrivate |= ShouldReserveName(miGet, false); } return hasNonPrivate; } private static bool ShouldReserveName(FieldInfo member) { return !member.IsPrivate && !member.IsAssembly && !member.IsSpecialName; } private static bool ShouldReserveName(MethodBase member, bool checkForSpecial) { return !member.IsPrivate && !member.IsAssembly && (!checkForSpecial || !member.IsSpecialName); } private static bool ShouldReserveName(MethodBase member) { return ShouldReserveName(member, true); } private static bool ShouldReserveName(Type member) { // we don't want to keep types return false; } #endregion #region Public Methods /// /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Globalization", "CA1308", Justification = "required for this feature")] public static string CamelCase(string text) { if (string.IsNullOrEmpty(text)) return text; if (text.Length == 1) return text[0].ToString(System.Globalization.CultureInfo.InvariantCulture).ToLowerInvariant(); return text[0].ToString(System.Globalization.CultureInfo.InvariantCulture).ToLowerInvariant() + text.Substring(1); } /// /// /// /// ///public static string PascalCase(string text) { if (string.IsNullOrEmpty(text)) return text; if (text.Length == 1) return text[0].ToString(System.Globalization.CultureInfo.InvariantCulture).ToUpperInvariant(); return text[0].ToString(System.Globalization.CultureInfo.InvariantCulture).ToUpperInvariant() + text.Substring(1); } /// /// /// /// /// ///private static string FixKeyword(string name, string prefix) { foreach (string keyword in _keywords) { if (name == keyword) { return prefix + PascalCase(name); } } return name; } /// /// /// /// /// ///public static string FixParameterName(string name, string prefix) { return CamelCase(FixKeyword(name, prefix)); } /// /// /// /// ///public static string FieldNameFromPropName(string propName) { return PrivateMemberPrefix(PrivateMemberPrefixId.Field) + propName; } /// /// Generate the name of a field that is used to indicate whether a complex property has been explicitly initialized /// /// Name of the property associated that with this field ///Generated field name public static string ComplexPropertyInitializedNameFromPropName(string propName) { return FieldNameFromPropName(propName) + _complexPropertyInitializedSuffix; } ////// get the prefix ussed for a private member /// /// ///public static string PrivateMemberPrefix(PrivateMemberPrefixId id) { return _privateMemberPrefixes[(int)id]; } /// /// /// /// /// ///public static bool TryGetPrimitiveTypeKind(EdmType type, out PrimitiveTypeKind modelType) { if (!Helper.IsPrimitiveType(type)) { // set it to something bogus because I have to modelType = PrimitiveTypeKind.Binary; return false; } modelType = ((PrimitiveType)type).PrimitiveTypeKind; return true; } /// /// /// /// ///public static string[] SplitName(string name) { Debug.Assert(!string.IsNullOrEmpty(name), "name parameter is null or empty"); if (name.Length > 0 && name[0] == '.') return name.Substring(1).Split('.'); return name.Split('.'); } public static string GetFullyQualifiedCodeGenerationAttributeName(string attribute) { return XmlConstants.CodeGenerationSchemaNamespace + ":" + attribute; } /// /// check if a name is reserved for a type /// /// the object representing the schema type being defined /// the member name ///true if the name is reserved by the type public static bool DoesTypeReserveMemberName(StructuralType type, string name, StringComparison comparison) { Type reservingType = null; if (!TryGetReservedName(name, comparison, out reservingType)) { return false; } // if reserving types is null it means the name is reserved for all types. if (reservingType == null) { return true; } return (reservingType == type.GetType()); } public static bool TryGetReservedName(string name, StringComparison comparison, out Type applyToSpecificType) { applyToSpecificType = null; foreach (KeyValuePairpair in _typeReservedNames) { if (pair.Key.Equals(name, comparison)) { applyToSpecificType = pair.Value; return true; } } return false; } public static string SetSpecialCaseForFxCopOnPropertyName(string propertyName) { // FxCop consider 'iD' as violation, we will change any property that is 'id'(case insensitive) to 'ID' if (StringComparer.OrdinalIgnoreCase.Equals(propertyName, "id")) { return "ID"; } return propertyName; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Reflection; namespace System.Data.EntityModel.Emitters { ////// Summary description for Utils /// internal static class Utils { #region Static Fields public const string WebFrameworkNamespace = "System.Data.Services.Client"; public const string WebFrameworkCommonNamespace = "System.Data.Services.Common"; public const string AdoFrameworkNamespace = "System.Data.Objects"; public const string AdoFrameworkDataClassesNamespace = "System.Data.Objects.DataClasses"; public const string AdoFrameworkMetadataEdmNamespace = "System.Data.Metadata.Edm"; public const string AdoEntityClientNamespace = "System.Data.EntityClient"; public const string SetValidValueMethodName = "SetValidValue"; public const string ReportPropertyChangingMethodName = "ReportPropertyChanging"; public const string ReportPropertyChangedMethodName = "ReportPropertyChanged"; public const string GetValidValueMethodName = "GetValidValue"; public const string VerifyComplexObjectIsNotNullName = "VerifyComplexObjectIsNotNull"; // this is the list of keywords we check against for names. // If a name matches this keyword we prefix it, this is only used when creating parameter names from property, etc. names(see FixKeyword) private static string[] _keywords = new string[] { "class", "event", }; // to guarantee uniqueness these must all be unique, begin with and end with an underscore and not contain internal underscores private static string[] _privateMemberPrefixes = new string[(int)PrivateMemberPrefixId.Count] { "_", "_Initialize_", "PropertyInfo", "_pi", }; // suffix that is added to field names to create a boolean field used to indicate whether or // not a complex property has been explicitly initialized private static string _complexPropertyInitializedSuffix = "Initialized"; private static List> _typeReservedNames = InitializeTypeReservedNames(); /// /// Initialize some statics that cannot be initialized in member declaration... /// static List> InitializeTypeReservedNames() { Dictionary typeReservedNames = new Dictionary (StringComparer.Ordinal); const BindingFlags bindingFlags = BindingFlags.FlattenHierarchy | BindingFlags.NonPublic | BindingFlags.Public | BindingFlags.Instance | BindingFlags.Static; foreach (MemberInfo member in typeof(object).GetMembers(bindingFlags)) { if (ShouldReserveName(member)) { if (!typeReservedNames.ContainsKey(member.Name)) { typeReservedNames.Add(member.Name, null); } } } List > pairs = new List >(); foreach (KeyValuePair pair in typeReservedNames) { pairs.Add(pair); } return pairs; } private static bool ShouldReserveName(MemberInfo member) { if (member is EventInfo) { return ShouldReserveName((EventInfo)member); } else if (member is FieldInfo) { return ShouldReserveName((FieldInfo)member); } else if (member is MethodBase) { return ShouldReserveName((MethodBase)member); } else if (member is PropertyInfo) { return ShouldReserveName((PropertyInfo)member); } else { Debug.Assert(member is Type, "Did you add a new type of member?"); return ShouldReserveName((Type)member); } } private static bool ShouldReserveName(EventInfo member) { bool hasNonPrivate = false; MethodInfo miAdd = member.GetAddMethod(); if (miAdd != null) { hasNonPrivate |= ShouldReserveName(miAdd, false); } MethodInfo miRemove = member.GetRemoveMethod(); if (miRemove != null) { hasNonPrivate |= ShouldReserveName(miRemove, false); } return hasNonPrivate; } private static bool ShouldReserveName(PropertyInfo member) { bool hasNonPrivate = false; MethodInfo miSet = member.GetSetMethod(); if (miSet != null) { hasNonPrivate |= ShouldReserveName(miSet, false); } MethodInfo miGet = member.GetGetMethod(); if (miGet != null) { hasNonPrivate |= ShouldReserveName(miGet, false); } return hasNonPrivate; } private static bool ShouldReserveName(FieldInfo member) { return !member.IsPrivate && !member.IsAssembly && !member.IsSpecialName; } private static bool ShouldReserveName(MethodBase member, bool checkForSpecial) { return !member.IsPrivate && !member.IsAssembly && (!checkForSpecial || !member.IsSpecialName); } private static bool ShouldReserveName(MethodBase member) { return ShouldReserveName(member, true); } private static bool ShouldReserveName(Type member) { // we don't want to keep types return false; } #endregion #region Public Methods /// /// /// /// ///[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Globalization", "CA1308", Justification = "required for this feature")] public static string CamelCase(string text) { if (string.IsNullOrEmpty(text)) return text; if (text.Length == 1) return text[0].ToString(System.Globalization.CultureInfo.InvariantCulture).ToLowerInvariant(); return text[0].ToString(System.Globalization.CultureInfo.InvariantCulture).ToLowerInvariant() + text.Substring(1); } /// /// /// /// ///public static string PascalCase(string text) { if (string.IsNullOrEmpty(text)) return text; if (text.Length == 1) return text[0].ToString(System.Globalization.CultureInfo.InvariantCulture).ToUpperInvariant(); return text[0].ToString(System.Globalization.CultureInfo.InvariantCulture).ToUpperInvariant() + text.Substring(1); } /// /// /// /// /// ///private static string FixKeyword(string name, string prefix) { foreach (string keyword in _keywords) { if (name == keyword) { return prefix + PascalCase(name); } } return name; } /// /// /// /// /// ///public static string FixParameterName(string name, string prefix) { return CamelCase(FixKeyword(name, prefix)); } /// /// /// /// ///public static string FieldNameFromPropName(string propName) { return PrivateMemberPrefix(PrivateMemberPrefixId.Field) + propName; } /// /// Generate the name of a field that is used to indicate whether a complex property has been explicitly initialized /// /// Name of the property associated that with this field ///Generated field name public static string ComplexPropertyInitializedNameFromPropName(string propName) { return FieldNameFromPropName(propName) + _complexPropertyInitializedSuffix; } ////// get the prefix ussed for a private member /// /// ///public static string PrivateMemberPrefix(PrivateMemberPrefixId id) { return _privateMemberPrefixes[(int)id]; } /// /// /// /// /// ///public static bool TryGetPrimitiveTypeKind(EdmType type, out PrimitiveTypeKind modelType) { if (!Helper.IsPrimitiveType(type)) { // set it to something bogus because I have to modelType = PrimitiveTypeKind.Binary; return false; } modelType = ((PrimitiveType)type).PrimitiveTypeKind; return true; } /// /// /// /// ///public static string[] SplitName(string name) { Debug.Assert(!string.IsNullOrEmpty(name), "name parameter is null or empty"); if (name.Length > 0 && name[0] == '.') return name.Substring(1).Split('.'); return name.Split('.'); } public static string GetFullyQualifiedCodeGenerationAttributeName(string attribute) { return XmlConstants.CodeGenerationSchemaNamespace + ":" + attribute; } /// /// check if a name is reserved for a type /// /// the object representing the schema type being defined /// the member name ///true if the name is reserved by the type public static bool DoesTypeReserveMemberName(StructuralType type, string name, StringComparison comparison) { Type reservingType = null; if (!TryGetReservedName(name, comparison, out reservingType)) { return false; } // if reserving types is null it means the name is reserved for all types. if (reservingType == null) { return true; } return (reservingType == type.GetType()); } public static bool TryGetReservedName(string name, StringComparison comparison, out Type applyToSpecificType) { applyToSpecificType = null; foreach (KeyValuePairpair in _typeReservedNames) { if (pair.Key.Equals(name, comparison)) { applyToSpecificType = pair.Value; return true; } } return false; } public static string SetSpecialCaseForFxCopOnPropertyName(string propertyName) { // FxCop consider 'iD' as violation, we will change any property that is 'id'(case insensitive) to 'ID' if (StringComparer.OrdinalIgnoreCase.Equals(propertyName, "id")) { return "ID"; } return propertyName; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
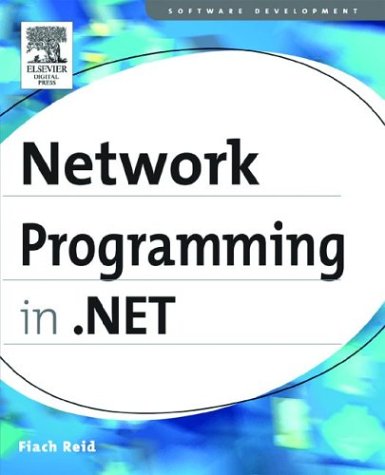
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Filter.cs
- DesignBindingConverter.cs
- AssemblyUtil.cs
- SystemIcmpV4Statistics.cs
- MaskInputRejectedEventArgs.cs
- PersonalizationProviderCollection.cs
- LabelLiteral.cs
- KeyPullup.cs
- DataObjectMethodAttribute.cs
- ContentDesigner.cs
- ClientScriptManager.cs
- ChannelServices.cs
- Inline.cs
- DataGridCommandEventArgs.cs
- SafeNativeMethods.cs
- MexBindingBindingCollectionElement.cs
- DiagnosticSection.cs
- Margins.cs
- PermissionAttributes.cs
- StringConcat.cs
- LocalBuilder.cs
- BevelBitmapEffect.cs
- IdentifierService.cs
- SpecialNameAttribute.cs
- ActivitiesCollection.cs
- LoadItemsEventArgs.cs
- ResizeGrip.cs
- OdbcConnectionPoolProviderInfo.cs
- SqlBuilder.cs
- CompositeFontFamily.cs
- ArgumentValidation.cs
- DeferredTextReference.cs
- EditModeSwitchButton.cs
- HtmlEmptyTagControlBuilder.cs
- EnvelopedPkcs7.cs
- DbDataSourceEnumerator.cs
- ActivationProxy.cs
- MimeWriter.cs
- ItemTypeToolStripMenuItem.cs
- Event.cs
- UncommonField.cs
- PageBuildProvider.cs
- AsyncOperationManager.cs
- Variable.cs
- HwndProxyElementProvider.cs
- GridProviderWrapper.cs
- ObjectIDGenerator.cs
- OdbcError.cs
- AsynchronousChannelMergeEnumerator.cs
- TextProperties.cs
- PkcsUtils.cs
- XmlWriterSettings.cs
- AutoGeneratedFieldProperties.cs
- SafeFileMapViewHandle.cs
- ValueProviderWrapper.cs
- SessionStateModule.cs
- ClipboardData.cs
- CheckedPointers.cs
- XPathSelfQuery.cs
- httpstaticobjectscollection.cs
- DataGridViewLayoutData.cs
- SafeCertificateStore.cs
- XmlDomTextWriter.cs
- PixelShader.cs
- AssemblyCollection.cs
- PathFigure.cs
- ObjectAnimationUsingKeyFrames.cs
- EntityAdapter.cs
- SecurityContext.cs
- DecoderBestFitFallback.cs
- UserControlBuildProvider.cs
- BindingMAnagerBase.cs
- XmlHierarchyData.cs
- BuildProviderCollection.cs
- RegexBoyerMoore.cs
- PackageStore.cs
- DateTimeOffsetStorage.cs
- FileIOPermission.cs
- X509AudioLogo.cs
- HtmlGenericControl.cs
- Activity.cs
- CellIdBoolean.cs
- FormsAuthenticationUser.cs
- MSAAEventDispatcher.cs
- KeyboardDevice.cs
- MetaChildrenColumn.cs
- RsaSecurityTokenAuthenticator.cs
- UnsettableComboBox.cs
- CallbackValidatorAttribute.cs
- DataTemplateSelector.cs
- MediaCommands.cs
- WindowsHyperlink.cs
- TargetException.cs
- InheritablePropertyChangeInfo.cs
- EventMappingSettingsCollection.cs
- TextModifierScope.cs
- CallbackValidator.cs
- PtsContext.cs
- TimeoutConverter.cs
- ClientRuntime.cs