Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Brush.cs / 2 / Brush.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.ComponentModel; using Microsoft.Win32; using System.Drawing; using System.Drawing.Internal; using System.Globalization; /** * Represent a Brush object */ ////// /// public abstract class Brush : MarshalByRefObject, ICloneable, IDisposable { #if FINALIZATION_WATCH private string allocationSite = Graphics.GetAllocationStack(); #endif // handle to native GDI+ brush object to be used on demand. ////// Classes derrived from this abstract base class define objects used to fill the /// interiors of graphical shapes such as rectangles, ellipses, pies, polygons, and paths. /// ///private IntPtr nativeBrush; /// /// /// When overriden in a derived class, creates /// an exact copy of this public abstract object Clone(); ///. /// /// Sets the native GDI+ brush reference. /// Note: This method is intended to be used by derived classes only! (internal protected doesn't work as in C++). /// protected internal void SetNativeBrush(IntPtr brush) { Debug.Assert( brush != IntPtr.Zero, "WARNING: Assigning null to the GDI+ native brush object."); Debug.Assert( this.nativeBrush == IntPtr.Zero, "WARNING: Initialized GDI+ native brush object being assigned a new value."); this.nativeBrush = brush; } ////// Gets the GDI+ native object reference. Triggers GDI+ obect initialization. /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] internal IntPtr NativeBrush { get { //Need to comment this line out to allow for checking this.NativePen == IntPtr.Zero. //Debug.Assert(this.nativeBrush != IntPtr.Zero, "this.nativeBrush == null." ); return this.nativeBrush; } } ////// /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Deletes this ///. /// protected virtual void Dispose(bool disposing) { #if FINALIZATION_WATCH if (!disposing && nativeBrush != IntPtr.Zero ) Debug.WriteLine("**********************\nDisposed through finalization:\n" + allocationSite); #endif if (this.nativeBrush != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteBrush(new HandleRef(this, this.nativeBrush)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ this.nativeBrush = IntPtr.Zero; } } } /** * Object cleanup */ /// /// /// ~Brush() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Releases memory allocated for this ///. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.ComponentModel; using Microsoft.Win32; using System.Drawing; using System.Drawing.Internal; using System.Globalization; /** * Represent a Brush object */ ////// /// public abstract class Brush : MarshalByRefObject, ICloneable, IDisposable { #if FINALIZATION_WATCH private string allocationSite = Graphics.GetAllocationStack(); #endif // handle to native GDI+ brush object to be used on demand. ////// Classes derrived from this abstract base class define objects used to fill the /// interiors of graphical shapes such as rectangles, ellipses, pies, polygons, and paths. /// ///private IntPtr nativeBrush; /// /// /// When overriden in a derived class, creates /// an exact copy of this public abstract object Clone(); ///. /// /// Sets the native GDI+ brush reference. /// Note: This method is intended to be used by derived classes only! (internal protected doesn't work as in C++). /// protected internal void SetNativeBrush(IntPtr brush) { Debug.Assert( brush != IntPtr.Zero, "WARNING: Assigning null to the GDI+ native brush object."); Debug.Assert( this.nativeBrush == IntPtr.Zero, "WARNING: Initialized GDI+ native brush object being assigned a new value."); this.nativeBrush = brush; } ////// Gets the GDI+ native object reference. Triggers GDI+ obect initialization. /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] internal IntPtr NativeBrush { get { //Need to comment this line out to allow for checking this.NativePen == IntPtr.Zero. //Debug.Assert(this.nativeBrush != IntPtr.Zero, "this.nativeBrush == null." ); return this.nativeBrush; } } ////// /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Deletes this ///. /// protected virtual void Dispose(bool disposing) { #if FINALIZATION_WATCH if (!disposing && nativeBrush != IntPtr.Zero ) Debug.WriteLine("**********************\nDisposed through finalization:\n" + allocationSite); #endif if (this.nativeBrush != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteBrush(new HandleRef(this, this.nativeBrush)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ this.nativeBrush = IntPtr.Zero; } } } /** * Object cleanup */ /// /// /// ~Brush() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Releases memory allocated for this ///. ///
Link Menu
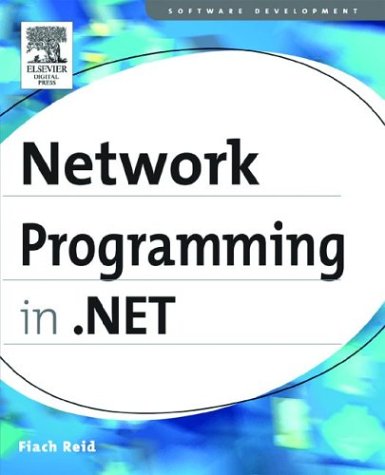
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlAttributeOverrides.cs
- XmlNotation.cs
- TabItem.cs
- SqlCommandBuilder.cs
- XmlILOptimizerVisitor.cs
- SignedInfo.cs
- Item.cs
- EtwTrace.cs
- MetadataItem.cs
- SqlCommandSet.cs
- WebPartsPersonalization.cs
- Thread.cs
- RuntimeHelpers.cs
- WeakReferenceList.cs
- RelationshipEntry.cs
- EventProperty.cs
- ListViewItem.cs
- CodeMemberMethod.cs
- ReadOnlyDictionary.cs
- DetailsViewDeleteEventArgs.cs
- GeneralTransform3D.cs
- TextTreeTextElementNode.cs
- DataSourceHelper.cs
- GridViewColumn.cs
- DbConnectionPoolIdentity.cs
- BinaryObjectWriter.cs
- VerificationAttribute.cs
- EntityParameter.cs
- FocusManager.cs
- ContractDescription.cs
- UpDownBase.cs
- DataTableNewRowEvent.cs
- Bits.cs
- UpdateTracker.cs
- SymbolMethod.cs
- PropertyGroupDescription.cs
- FragmentQuery.cs
- ComponentSerializationService.cs
- TagMapInfo.cs
- DataGridViewMethods.cs
- XmlCDATASection.cs
- DataGridAddNewRow.cs
- XPathNodePointer.cs
- TextRangeAdaptor.cs
- MembershipUser.cs
- XamlClipboardData.cs
- SortExpressionBuilder.cs
- DetailsViewUpdateEventArgs.cs
- ExpanderAutomationPeer.cs
- DataGridViewCellLinkedList.cs
- TypeFieldSchema.cs
- TableAdapterManagerNameHandler.cs
- StringPropertyBuilder.cs
- WindowsFormsDesignerOptionService.cs
- HealthMonitoringSection.cs
- TypeToken.cs
- TrackingCondition.cs
- LicenseManager.cs
- WebPartMinimizeVerb.cs
- HighContrastHelper.cs
- BamlLocalizationDictionary.cs
- AlternateView.cs
- ButtonAutomationPeer.cs
- NullableConverter.cs
- MemberProjectionIndex.cs
- ApplicationDirectory.cs
- ToolStripMenuItemDesigner.cs
- QueryContinueDragEvent.cs
- InkCollectionBehavior.cs
- SqlBulkCopyColumnMappingCollection.cs
- WindowsListViewGroupSubsetLink.cs
- CacheAxisQuery.cs
- TransportSecurityProtocol.cs
- TreeSet.cs
- XamlToRtfWriter.cs
- FileLevelControlBuilderAttribute.cs
- VariableElement.cs
- StyleBamlRecordReader.cs
- xml.cs
- EmbeddedObject.cs
- PersonalizationStateQuery.cs
- HttpClientCertificate.cs
- OraclePermission.cs
- datacache.cs
- AttributeQuery.cs
- FontFamilyIdentifier.cs
- _AutoWebProxyScriptWrapper.cs
- DocumentViewerAutomationPeer.cs
- BitArray.cs
- KeyTime.cs
- PrimaryKeyTypeConverter.cs
- DrawingBrush.cs
- DbProviderSpecificTypePropertyAttribute.cs
- LinkedList.cs
- EntityContainerRelationshipSet.cs
- DataServices.cs
- MetadataCache.cs
- FastPropertyAccessor.cs
- ContainerParagraph.cs
- ListViewItemSelectionChangedEvent.cs