Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / TransportSecurityProtocol.cs / 1 / TransportSecurityProtocol.cs
//---------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Security { using System.Collections.Generic; using System.ServiceModel; using System.ServiceModel.Description; using System.Collections.ObjectModel; using System.IO; using System.Runtime.InteropServices; using System.IdentityModel.Tokens; using System.IdentityModel.Selectors; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.ServiceModel.Security.Tokens; using System.Security.Cryptography; using System.ServiceModel.Channels; using System.Text; using System.Xml; using System.Xml.Serialization; using System.ServiceModel.Diagnostics; class TransportSecurityProtocol : SecurityProtocol { public TransportSecurityProtocol(TransportSecurityProtocolFactory factory, EndpointAddress target, Uri via) : base(factory, target, via) { } public override void SecureOutgoingMessage(ref Message message, TimeSpan timeout) { if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("message"); } this.CommunicationObject.ThrowIfClosedOrNotOpen(); string actor = string.Empty; // message.Version.Envelope.UltimateDestinationActor; try { if (this.SecurityProtocolFactory.ActAsInitiator) { SecureOutgoingMessageAtInitiator(ref message, actor, timeout); } else { SecureOutgoingMessageAtResponder(ref message, actor); } base.OnOutgoingMessageSecured(message); } catch { base.OnSecureOutgoingMessageFailure(message); throw; } } protected virtual void SecureOutgoingMessageAtInitiator(ref Message message, string actor, TimeSpan timeout) { IListsupportingTokens; TryGetSupportingTokens(this.SecurityProtocolFactory, this.Target, this.Via, message, timeout, true, out supportingTokens); SetUpDelayedSecurityExecution(ref message, actor, supportingTokens); } protected void SecureOutgoingMessageAtResponder(ref Message message, string actor) { if (this.SecurityProtocolFactory.AddTimestamp) { SendSecurityHeader securityHeader = CreateSendSecurityHeaderForTransportProtocol(message, actor, this.SecurityProtocolFactory); message = securityHeader.SetupExecution(); } } internal void SetUpDelayedSecurityExecution(ref Message message, string actor, IList supportingTokens) { SendSecurityHeader securityHeader = CreateSendSecurityHeaderForTransportProtocol(message, actor, this.SecurityProtocolFactory); AddSupportingTokens(securityHeader, supportingTokens); message = securityHeader.SetupExecution(); } public override IAsyncResult BeginSecureOutgoingMessage(Message message, TimeSpan timeout, SecurityProtocolCorrelationState correlationState, AsyncCallback callback, object state) { if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("message"); } this.CommunicationObject.ThrowIfClosedOrNotOpen(); string actor = string.Empty; // message.Version.Envelope.UltimateDestinationActor; try { if (this.SecurityProtocolFactory.ActAsInitiator) { return this.BeginSecureOutgoingMessageAtInitiatorCore(message, actor, timeout, callback, state); } else { SecureOutgoingMessageAtResponder(ref message, actor); return new TypedCompletedAsyncResult (message, callback, state); } } catch (Exception exception) { // Always immediately rethrow fatal exceptions. if (DiagnosticUtility.IsFatal(exception)) throw; base.OnSecureOutgoingMessageFailure(message); throw; } } public override IAsyncResult BeginSecureOutgoingMessage(Message message, TimeSpan timeout, AsyncCallback callback, object state) { return BeginSecureOutgoingMessage(message, timeout, null, callback, state); } protected virtual IAsyncResult BeginSecureOutgoingMessageAtInitiatorCore(Message message, string actor, TimeSpan timeout, AsyncCallback callback, object state) { IList supportingTokens; TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); if (TryGetSupportingTokens(this.SecurityProtocolFactory, this.Target, this.Via, message, timeoutHelper.RemainingTime(), false, out supportingTokens)) { SetUpDelayedSecurityExecution(ref message, actor, supportingTokens); return new TypedCompletedAsyncResult (message, callback, state); } else { return new SecureOutgoingMessageAsyncResult(actor, message, this, timeout, callback, state); } } protected virtual Message EndSecureOutgoingMessageAtInitiatorCore(IAsyncResult result) { if (result is TypedCompletedAsyncResult ) { return TypedCompletedAsyncResult .End(result); } else { return SecureOutgoingMessageAsyncResult.End(result); } } public override void EndSecureOutgoingMessage(IAsyncResult result, out Message message) { SecurityProtocolCorrelationState dummyState; this.EndSecureOutgoingMessage(result, out message, out dummyState); } public override void EndSecureOutgoingMessage(IAsyncResult result, out Message message, out SecurityProtocolCorrelationState newCorrelationState) { if (result == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("result"); } newCorrelationState = null; try { if (result is TypedCompletedAsyncResult ) { message = TypedCompletedAsyncResult .End(result); } else { message = this.EndSecureOutgoingMessageAtInitiatorCore(result); } base.OnOutgoingMessageSecured(message); } catch (Exception exception) { // Always immediately rethrow fatal exceptions. if (DiagnosticUtility.IsFatal(exception)) throw; base.OnSecureOutgoingMessageFailure(null); throw; } } public sealed override void VerifyIncomingMessage(ref Message message, TimeSpan timeout) { if (message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("message"); } this.CommunicationObject.ThrowIfClosedOrNotOpen(); try { VerifyIncomingMessageCore(ref message, timeout); } catch (MessageSecurityException e) { base.OnVerifyIncomingMessageFailure(message, e); throw; } catch (Exception e) { // Always immediately rethrow fatal exceptions. if (DiagnosticUtility.IsFatal(e)) throw; base.OnVerifyIncomingMessageFailure(message, e); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessageSecurityException(SR.GetString(SR.MessageSecurityVerificationFailed), e)); } } protected void AttachRecipientSecurityProperty(Message message, IList basicTokens, IList endorsingTokens, IList signedEndorsingTokens, IList signedTokens, Dictionary > tokenPoliciesMapping) { SecurityMessageProperty security = SecurityMessageProperty.GetOrCreate(message); AddSupportingTokenSpecification(security, basicTokens, endorsingTokens, signedEndorsingTokens, signedTokens, tokenPoliciesMapping); security.ServiceSecurityContext = new ServiceSecurityContext(security.GetInitiatorTokenAuthorizationPolicies()); } protected virtual void VerifyIncomingMessageCore(ref Message message, TimeSpan timeout) { TransportSecurityProtocolFactory factory = (TransportSecurityProtocolFactory)this.SecurityProtocolFactory; string actor = string.Empty; // message.Version.Envelope.UltimateDestinationActor; ReceiveSecurityHeader securityHeader = factory.StandardsManager.TryCreateReceiveSecurityHeader(message, actor, factory.IncomingAlgorithmSuite, (factory.ActAsInitiator) ? MessageDirection.Output : MessageDirection.Input); bool expectBasicTokens; bool expectEndorsingTokens; bool expectSignedTokens; IList supportingAuthenticators = factory.GetSupportingTokenAuthenticators(message.Headers.Action, out expectSignedTokens, out expectBasicTokens, out expectEndorsingTokens); if (securityHeader == null) { bool expectSupportingTokens = expectEndorsingTokens || expectSignedTokens || expectBasicTokens; if ((factory.ActAsInitiator && !factory.AddTimestamp) || (!factory.ActAsInitiator && !factory.AddTimestamp && !expectSupportingTokens)) { return; } else { if (String.IsNullOrEmpty(actor)) throw System.ServiceModel.Diagnostics.TraceUtility.ThrowHelperError(new MessageSecurityException( SR.GetString(SR.UnableToFindSecurityHeaderInMessageNoActor)), message); else throw System.ServiceModel.Diagnostics.TraceUtility.ThrowHelperError(new MessageSecurityException( SR.GetString(SR.UnableToFindSecurityHeaderInMessage, actor)), message); } } securityHeader.RequireMessageProtection = false; securityHeader.ExpectBasicTokens = expectBasicTokens; securityHeader.ExpectSignedTokens = expectSignedTokens; securityHeader.ExpectEndorsingTokens = expectEndorsingTokens; securityHeader.MaxReceivedMessageSize = factory.SecurityBindingElement.MaxReceivedMessageSize; securityHeader.ReaderQuotas = factory.SecurityBindingElement.ReaderQuotas; TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); if (!factory.ActAsInitiator) { securityHeader.ConfigureTransportBindingServerReceiveHeader(supportingAuthenticators); securityHeader.ConfigureOutOfBandTokenResolver(MergeOutOfBandResolvers(supportingAuthenticators, EmptyReadOnlyCollection .Instance)); if (factory.ExpectKeyDerivation) { securityHeader.DerivedTokenAuthenticator = factory.DerivedKeyTokenAuthenticator; } } securityHeader.SetTimeParameters(factory.NonceCache, factory.ReplayWindow, factory.MaxClockSkew); securityHeader.Process(timeoutHelper.RemainingTime()); message = securityHeader.ProcessedMessage; if (!factory.ActAsInitiator) { AttachRecipientSecurityProperty(message, securityHeader.BasicSupportingTokens, securityHeader.EndorsingSupportingTokens, securityHeader.SignedEndorsingSupportingTokens, securityHeader.SignedSupportingTokens, securityHeader.SecurityTokenAuthorizationPoliciesMapping); } base.OnIncomingMessageVerified(message); } sealed class SecureOutgoingMessageAsyncResult : GetSupportingTokensAsyncResult { Message message; string actor; TransportSecurityProtocol binding; public SecureOutgoingMessageAsyncResult(string actor, Message message, TransportSecurityProtocol binding, TimeSpan timeout, AsyncCallback callback, object state) : base(message, binding, timeout, callback, state) { this.actor = actor; this.message = message; this.binding = binding; this.Start(); } protected override bool OnGetSupportingTokensDone(TimeSpan timeout) { this.binding.SetUpDelayedSecurityExecution(ref this.message, this.actor, this.SupportingTokens); return true; } internal static Message End(IAsyncResult result) { SecureOutgoingMessageAsyncResult self = AsyncResult.End (result); return self.message; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
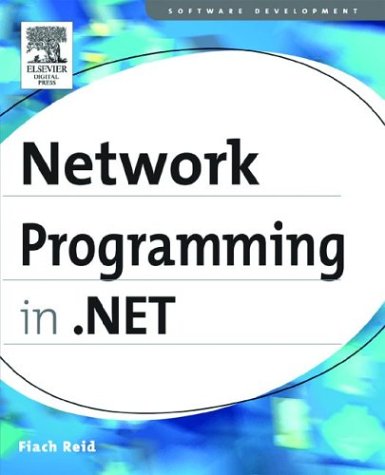
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CapiHashAlgorithm.cs
- DecoderFallbackWithFailureFlag.cs
- ItemsControlAutomationPeer.cs
- InheritanceService.cs
- Policy.cs
- AuthenticationService.cs
- ValidationError.cs
- ControlCachePolicy.cs
- DataControlField.cs
- ComboBoxAutomationPeer.cs
- EntityDataSourceReferenceGroup.cs
- QilVisitor.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- PageClientProxyGenerator.cs
- ValidationError.cs
- EnumBuilder.cs
- SevenBitStream.cs
- ButtonPopupAdapter.cs
- DynamicActionMessageFilter.cs
- PartialTrustVisibleAssemblyCollection.cs
- VisualTarget.cs
- Visual3D.cs
- InkCanvasSelectionAdorner.cs
- HttpModuleActionCollection.cs
- RewritingValidator.cs
- AssemblyAssociatedContentFileAttribute.cs
- Positioning.cs
- BuildProvider.cs
- PostBackOptions.cs
- Control.cs
- GradientBrush.cs
- RecordConverter.cs
- IisTraceWebEventProvider.cs
- FileVersion.cs
- VerificationAttribute.cs
- ViewCellRelation.cs
- ArglessEventHandlerProxy.cs
- PolicyLevel.cs
- ProfilePropertyNameValidator.cs
- Metadata.cs
- FocusManager.cs
- KeyConstraint.cs
- SqlGatherConsumedAliases.cs
- ResourcesChangeInfo.cs
- WindowsRebar.cs
- MatrixIndependentAnimationStorage.cs
- SplayTreeNode.cs
- SqlDataSourceEnumerator.cs
- TextBox.cs
- EventDescriptorCollection.cs
- ScriptComponentDescriptor.cs
- PointAnimationUsingPath.cs
- objectquery_tresulttype.cs
- EncodingDataItem.cs
- EntityParameterCollection.cs
- HtmlToClrEventProxy.cs
- Attribute.cs
- Hyperlink.cs
- SchemaManager.cs
- Menu.cs
- HtmlTableRowCollection.cs
- CursorConverter.cs
- CounterSampleCalculator.cs
- ObjectToIdCache.cs
- ResourcePermissionBase.cs
- XmlSigningNodeWriter.cs
- GuidelineCollection.cs
- ConnectionProviderAttribute.cs
- ScriptingSectionGroup.cs
- XmlILStorageConverter.cs
- PropertyEmitterBase.cs
- ActivityInterfaces.cs
- KnownBoxes.cs
- FontStretchConverter.cs
- WebPartMinimizeVerb.cs
- FieldToken.cs
- QueryTreeBuilder.cs
- NamedPipeProcessProtocolHandler.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- DataServiceStreamProviderWrapper.cs
- ToolStripSeparator.cs
- IsolatedStoragePermission.cs
- GlyphShapingProperties.cs
- _AutoWebProxyScriptWrapper.cs
- PositiveTimeSpanValidatorAttribute.cs
- SecurityDescriptor.cs
- SerializationException.cs
- DecimalConstantAttribute.cs
- DesigntimeLicenseContextSerializer.cs
- RelationshipSet.cs
- SmiConnection.cs
- ObjectTokenCategory.cs
- GridPatternIdentifiers.cs
- ConstructorNeedsTagAttribute.cs
- AsyncPostBackErrorEventArgs.cs
- SafeLibraryHandle.cs
- InnerItemCollectionView.cs
- BaseWebProxyFinder.cs
- MarginCollapsingState.cs
- SectionRecord.cs