Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWebControls / System / Data / WebControls / EntityDataSourceReferenceGroup.cs / 1599186 / EntityDataSourceReferenceGroup.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.EntityClient; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.ComponentModel; using System.Data.Common; using System.Data.Objects.DataClasses; using System.Data.Objects; using System.Data; using System.Runtime.CompilerServices; namespace System.Web.UI.WebControls { ////// Groups together reference columns pointing at the same association end. /// internal abstract class EntityDataSourceReferenceGroup { private readonly AssociationSetEnd end; protected EntityDataSourceReferenceGroup(AssociationSetEnd end) { EntityDataSourceUtil.CheckArgumentNull(end, "end"); this.end = end; } internal AssociationSetEnd End { get { return this.end; } } [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] internal static EntityDataSourceReferenceGroup Create(Type entityType, AssociationSetEnd end) { EntityDataSourceUtil.CheckArgumentNull(entityType, "entityType"); Type groupType = typeof(EntityDataSourceReferenceGroup<>).MakeGenericType(entityType); return (EntityDataSourceReferenceGroup)Activator.CreateInstance(groupType, new object[] { end }); } internal abstract void SetKeyValues(EntityDataSourceWrapper wrapper, DictionarynewKeyValues); internal abstract EntityKey GetEntityKey(EntityDataSourceWrapper entity); } internal class EntityDataSourceReferenceGroup : EntityDataSourceReferenceGroup where T : class { public EntityDataSourceReferenceGroup(AssociationSetEnd end) : base(end) { } internal override void SetKeyValues(EntityDataSourceWrapper wrapper, Dictionary newKeyValues) { EntityDataSourceUtil.CheckArgumentNull(wrapper, "wrapper"); EntityReference reference = GetRelatedReference(wrapper); EntityKey originalEntityKeys = reference.EntityKey; if (null != newKeyValues) { if(null != originalEntityKeys) { // mix the missing keys from the original values foreach (var originalEntityKey in originalEntityKeys.EntityKeyValues) { object newKeyValue; if (newKeyValues.TryGetValue(originalEntityKey.Key, out newKeyValue)) { // if any part of the key is null, the EntityKey is null if (null == newKeyValue) { newKeyValues = null; break; } } else { // add the original value for this partial key since it is not saved in the viewstate newKeyValues.Add(originalEntityKey.Key, originalEntityKey.Value); } } } else { // what we have in the newKeyValues should be sufficient to set the key // but if any value is null, the whole key is null foreach (var newKey in newKeyValues) { if (null == newKey.Value) { newKeyValues = null; break; } } } } if (null == newKeyValues) { // if the entity key is a compound key, and if any partial key is null, then the entitykey is null reference.EntityKey = null; } else { reference.EntityKey = new EntityKey(EntityDataSourceUtil.GetQualifiedEntitySetName(End.EntitySet), (IEnumerable >)newKeyValues); } } internal override EntityKey GetEntityKey(EntityDataSourceWrapper entity) { EntityKey key = GetRelatedReference(entity).EntityKey; return key; } private EntityReference GetRelatedReference(EntityDataSourceWrapper entity) { RelationshipManager relationshipManager = entity.RelationshipManager; Debug.Assert(relationshipManager != null, "couldn't get a relationship manager"); EntityReference reference = relationshipManager.GetRelatedReference ( this.End.ParentAssociationSet.ElementType.FullName, this.End.CorrespondingAssociationEndMember.Name); return reference; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.EntityClient; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.ComponentModel; using System.Data.Common; using System.Data.Objects.DataClasses; using System.Data.Objects; using System.Data; using System.Runtime.CompilerServices; namespace System.Web.UI.WebControls { ////// Groups together reference columns pointing at the same association end. /// internal abstract class EntityDataSourceReferenceGroup { private readonly AssociationSetEnd end; protected EntityDataSourceReferenceGroup(AssociationSetEnd end) { EntityDataSourceUtil.CheckArgumentNull(end, "end"); this.end = end; } internal AssociationSetEnd End { get { return this.end; } } [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] internal static EntityDataSourceReferenceGroup Create(Type entityType, AssociationSetEnd end) { EntityDataSourceUtil.CheckArgumentNull(entityType, "entityType"); Type groupType = typeof(EntityDataSourceReferenceGroup<>).MakeGenericType(entityType); return (EntityDataSourceReferenceGroup)Activator.CreateInstance(groupType, new object[] { end }); } internal abstract void SetKeyValues(EntityDataSourceWrapper wrapper, DictionarynewKeyValues); internal abstract EntityKey GetEntityKey(EntityDataSourceWrapper entity); } internal class EntityDataSourceReferenceGroup : EntityDataSourceReferenceGroup where T : class { public EntityDataSourceReferenceGroup(AssociationSetEnd end) : base(end) { } internal override void SetKeyValues(EntityDataSourceWrapper wrapper, Dictionary newKeyValues) { EntityDataSourceUtil.CheckArgumentNull(wrapper, "wrapper"); EntityReference reference = GetRelatedReference(wrapper); EntityKey originalEntityKeys = reference.EntityKey; if (null != newKeyValues) { if(null != originalEntityKeys) { // mix the missing keys from the original values foreach (var originalEntityKey in originalEntityKeys.EntityKeyValues) { object newKeyValue; if (newKeyValues.TryGetValue(originalEntityKey.Key, out newKeyValue)) { // if any part of the key is null, the EntityKey is null if (null == newKeyValue) { newKeyValues = null; break; } } else { // add the original value for this partial key since it is not saved in the viewstate newKeyValues.Add(originalEntityKey.Key, originalEntityKey.Value); } } } else { // what we have in the newKeyValues should be sufficient to set the key // but if any value is null, the whole key is null foreach (var newKey in newKeyValues) { if (null == newKey.Value) { newKeyValues = null; break; } } } } if (null == newKeyValues) { // if the entity key is a compound key, and if any partial key is null, then the entitykey is null reference.EntityKey = null; } else { reference.EntityKey = new EntityKey(EntityDataSourceUtil.GetQualifiedEntitySetName(End.EntitySet), (IEnumerable >)newKeyValues); } } internal override EntityKey GetEntityKey(EntityDataSourceWrapper entity) { EntityKey key = GetRelatedReference(entity).EntityKey; return key; } private EntityReference GetRelatedReference(EntityDataSourceWrapper entity) { RelationshipManager relationshipManager = entity.RelationshipManager; Debug.Assert(relationshipManager != null, "couldn't get a relationship manager"); EntityReference reference = relationshipManager.GetRelatedReference ( this.End.ParentAssociationSet.ElementType.FullName, this.End.CorrespondingAssociationEndMember.Name); return reference; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
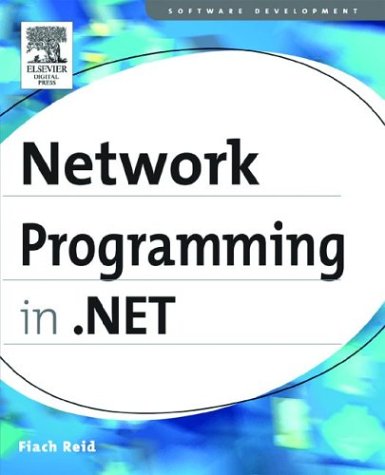
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberAccessException.cs
- WindowsFormsHostPropertyMap.cs
- HwndTarget.cs
- SerializableReadOnlyDictionary.cs
- DataPagerFieldCommandEventArgs.cs
- PersistencePipeline.cs
- Emitter.cs
- GridViewUpdatedEventArgs.cs
- ResourceSet.cs
- XmlSerializerOperationFormatter.cs
- Dictionary.cs
- PathStreamGeometryContext.cs
- EditorReuseAttribute.cs
- WebResourceUtil.cs
- DataGridLength.cs
- ExtendedPropertyDescriptor.cs
- BitmapSizeOptions.cs
- SafeNativeMethodsCLR.cs
- FormViewAutoFormat.cs
- EnumDataContract.cs
- WindowsFormsLinkLabel.cs
- SQLUtility.cs
- NumericExpr.cs
- QueryServiceConfigHandle.cs
- Timer.cs
- CacheSection.cs
- SqlWebEventProvider.cs
- unsafeIndexingFilterStream.cs
- Point.cs
- RegexRunnerFactory.cs
- AuditLogLocation.cs
- Vector3D.cs
- MasterPageParser.cs
- WebZone.cs
- EncryptedPackageFilter.cs
- DateTimeFormatInfoScanner.cs
- WbmpConverter.cs
- _ScatterGatherBuffers.cs
- filewebresponse.cs
- ObjectStateFormatter.cs
- EventLogEntry.cs
- RMPermissions.cs
- IntegerValidator.cs
- URIFormatException.cs
- DoubleAnimationClockResource.cs
- EventLogPermissionEntry.cs
- FilterQueryOptionExpression.cs
- TcpTransportSecurityElement.cs
- XPathNavigatorKeyComparer.cs
- ExclusiveTcpListener.cs
- BamlLocalizabilityResolver.cs
- DynamicDataManager.cs
- ClaimComparer.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- ReceiveSecurityHeaderEntry.cs
- MsmqAppDomainProtocolHandler.cs
- AppSettingsExpressionBuilder.cs
- ReachFixedDocumentSerializerAsync.cs
- UpdateRecord.cs
- CodeAttributeArgumentCollection.cs
- GlyphRunDrawing.cs
- UrlMappingsSection.cs
- EventItfInfo.cs
- DmlSqlGenerator.cs
- IOThreadTimer.cs
- HotSpotCollection.cs
- HttpClientCertificate.cs
- CompressEmulationStream.cs
- ZoneLinkButton.cs
- TextBox.cs
- ResourcePart.cs
- __ComObject.cs
- Application.cs
- GatewayIPAddressInformationCollection.cs
- LinqDataSource.cs
- MetadataProperty.cs
- Ops.cs
- DocumentXPathNavigator.cs
- ChainOfDependencies.cs
- FtpRequestCacheValidator.cs
- BitmapEffectInput.cs
- Calendar.cs
- SpellerInterop.cs
- AttachedPropertiesService.cs
- Array.cs
- ProxyWebPart.cs
- followingquery.cs
- oledbmetadatacollectionnames.cs
- ManifestResourceInfo.cs
- XmlImplementation.cs
- ObjectListDesigner.cs
- PingReply.cs
- WorkflowMarkupSerializationProvider.cs
- SecurityBindingElementImporter.cs
- ObjectStateEntryDbDataRecord.cs
- Reference.cs
- WsdlBuildProvider.cs
- UnknownWrapper.cs
- Utils.cs
- CatchBlock.cs