Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Markup / Localizer / BamlLocalizationDictionary.cs / 1 / BamlLocalizationDictionary.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: BamlLocalizationDictionary.cs // // Contents: BamlLocalizationDictionary and BamlLocalizationDictionaryEnumerator // // History: 8/3/2004 Move to System.Windows namespace // 11/30/2004 [....] Move to System.Windows.Markup.Localization namespace // Renamed class to BamlLocalizationDictionary // 03/24/2005 [....] Move to System.Windows.Markup.Localizer namespace //----------------------------------------------------------------------- using System; using System.IO; using System.Globalization; using System.Runtime.InteropServices; using System.Collections; using System.Collections.Generic; using System.Windows.Markup; using System.Diagnostics; using System.Text; using System.Windows; namespace System.Windows.Markup.Localizer { ////// BamlLocalizationDictionaryEnumerator /// public sealed class BamlLocalizationDictionaryEnumerator : IDictionaryEnumerator { internal BamlLocalizationDictionaryEnumerator(IEnumerator enumerator) { _enumerator = enumerator; } ////// move to the next entry /// public bool MoveNext() { return _enumerator.MoveNext(); } ////// reset /// public void Reset() { _enumerator.Reset(); } ////// gets the DictionaryEntry /// public DictionaryEntry Entry { get{ return (DictionaryEntry) _enumerator.Current; } } ////// gets the key /// public BamlLocalizableResourceKey Key { get{ return (BamlLocalizableResourceKey) Entry.Key; } } ////// gets the value /// public BamlLocalizableResource Value { get{ return (BamlLocalizableResource) Entry.Value; } } ////// return the current entry /// public DictionaryEntry Current { get { return this.Entry; } } //------------------------------------ // Interfaces //------------------------------------ ////// Return the current object /// ///object object IEnumerator.Current { get { return this.Current; } } ////// return the key /// ///key object IDictionaryEnumerator.Key { get { return this.Key; } } ////// Value /// ///value object IDictionaryEnumerator.Value { get { return this.Value; } } //--------------------------------------- // Private //--------------------------------------- private IEnumerator _enumerator; } ////// Enumerator that enumerates all the localizable resources in /// a baml stream /// public sealed class BamlLocalizationDictionary : IDictionary { ////// Constructor that creates an empty baml resource dictionary /// public BamlLocalizationDictionary() { _dictionary = new Dictionary(); } /// /// gets value indicating whether the dictionary has fixed size /// ///true for fixed size, false otherwise. public bool IsFixedSize { get { return false; } } ////// get value indicating whether it is readonly /// ///true for readonly, false otherwise. public bool IsReadOnly { get { return false;} } ////// Return the key to the root element if the root element is localizable, return null otherwise /// ////// Modifications can be added to the proeprties of the root element which will have a global effect /// on the UI. For example, changing CulutreInfo or FlowDirection on the root element (if applicable) /// will have impact to the whole UI. /// public BamlLocalizableResourceKey RootElementKey { get { return _rootElementKey; } } ////// gets the collection of keys /// ///a collection of keys public ICollection Keys { get { return ((IDictionary)_dictionary).Keys; } } ////// gets the collection of values /// ///a collection of values public ICollection Values { get { return ((IDictionary)_dictionary).Values; } } ////// Gets or sets a localizable resource by the key /// /// BamlLocalizableResourceKey key ///BamlLocalizableResource object identified by the key public BamlLocalizableResource this[BamlLocalizableResourceKey key] { get { CheckNonNullParam(key, "key"); return _dictionary[key]; } set { CheckNonNullParam(key, "key"); _dictionary[key] = value; } } ////// Adds a localizable resource with the provided key /// /// the BamlLocalizableResourceKey key /// the BamlLocalizableResource public void Add(BamlLocalizableResourceKey key, BamlLocalizableResource value) { CheckNonNullParam(key, "key"); _dictionary.Add(key, value); } ////// removes all the resources in the dictionary. /// public void Clear() { _dictionary.Clear(); } ////// removes the localizable resource with the specified key /// /// the key public void Remove(BamlLocalizableResourceKey key) { _dictionary.Remove(key); } ////// determines whether the dictionary contains the localizable resource /// with the specified key /// /// ///public bool Contains(BamlLocalizableResourceKey key) { CheckNonNullParam(key, "key"); return _dictionary.ContainsKey(key); } /// /// returns an IDictionaryEnumerator for the dictionary. /// ///the enumerator for the dictionary public BamlLocalizationDictionaryEnumerator GetEnumerator() { return new BamlLocalizationDictionaryEnumerator( ((IDictionary)_dictionary).GetEnumerator() ); } ////// gets the number of localizable resources in the dictionary /// ///number of localizable resources public int Count { get { return _dictionary.Count; } } ////// Copies the dictionary's elements to a one-dimensional /// Array instance at the specified index. /// public void CopyTo(DictionaryEntry[] array, int arrayIndex) { CheckNonNullParam(array, "array"); if (arrayIndex < 0) { throw new ArgumentOutOfRangeException( "arrayIndex", SR.Get(SRID.ParameterCannotBeNegative) ); } if (arrayIndex >= array.Length) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "arrayIndex", "array" ), "arrayIndex" ); } if (Count > (array.Length - arrayIndex)) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array" ) ); } foreach(KeyValuePairpair in _dictionary) { DictionaryEntry entry = new DictionaryEntry(pair.Key, pair.Value); array[arrayIndex++] = entry; } } #region interface ICollection, IEnumerable, IDictionary //------------------------------ // interface functions //------------------------------ bool IDictionary.Contains(object key) { CheckNonNullParam(key, "key"); return ((IDictionary)_dictionary).Contains(key); } void IDictionary.Add(object key, object value) { CheckNonNullParam(key, "key"); ((IDictionary) _dictionary).Add(key, value); } void IDictionary.Remove(object key) { CheckNonNullParam(key, "key"); ((IDictionary) _dictionary).Remove(key); } object IDictionary.this[object key] { get { CheckNonNullParam(key, "key"); return ((IDictionary)_dictionary)[key]; } set { CheckNonNullParam(key, "key"); ((IDictionary)_dictionary)[key] = value; } } IDictionaryEnumerator IDictionary.GetEnumerator() { return this.GetEnumerator(); } void ICollection.CopyTo(Array array, int index) { if (array != null && array.Rank != 1) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_ArrayCannotBeMultidimensional ), "array" ); } CopyTo(array as DictionaryEntry[], index); } int ICollection.Count { get { return Count; } } object ICollection.SyncRoot { get { return ((IDictionary)_dictionary).SyncRoot; } } bool ICollection.IsSynchronized { get { return ((IDictionary)_dictionary).IsSynchronized; } } IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } #endregion //------------------------------ // internal functions //------------------------------ internal BamlLocalizationDictionary Copy() { BamlLocalizationDictionary newDictionary = new BamlLocalizationDictionary(); foreach (KeyValuePair pair in _dictionary) { BamlLocalizableResource resourceCopy = pair.Value == null ? null : new BamlLocalizableResource(pair.Value); newDictionary.Add(pair.Key, resourceCopy); } newDictionary._rootElementKey = _rootElementKey; // return the new dictionary return newDictionary; } internal void SetRootElementKey(BamlLocalizableResourceKey key) { _rootElementKey = key; } //------------------------------ // private methods //------------------------------ private void CheckNonNullParam(object param, string paramName) { if (param == null) throw new ArgumentNullException(paramName); } //------------------------------ // private member //------------------------------ private IDictionary _dictionary; private BamlLocalizableResourceKey _rootElementKey; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
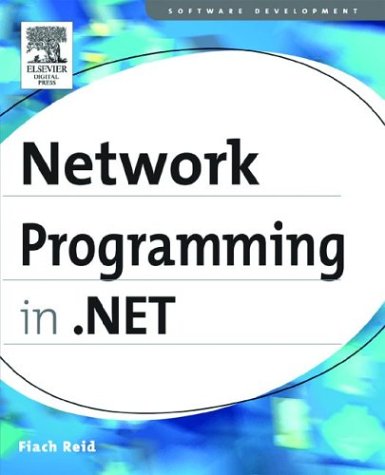
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentSchemaValidator.cs
- DtrList.cs
- SafeEventHandle.cs
- ItemAutomationPeer.cs
- SoapEnumAttribute.cs
- SimpleWorkerRequest.cs
- XAMLParseException.cs
- _OverlappedAsyncResult.cs
- Page.cs
- ComponentResourceManager.cs
- MutexSecurity.cs
- RegistryPermission.cs
- PiiTraceSource.cs
- DataFieldConverter.cs
- ColumnMapProcessor.cs
- BidOverLoads.cs
- SmtpReplyReader.cs
- TextTrailingWordEllipsis.cs
- MulticastIPAddressInformationCollection.cs
- TextStore.cs
- ObjectDataSourceFilteringEventArgs.cs
- EventSetter.cs
- StorageFunctionMapping.cs
- CompositionAdorner.cs
- Int16AnimationUsingKeyFrames.cs
- FilterQueryOptionExpression.cs
- ElementHostPropertyMap.cs
- HttpApplicationStateWrapper.cs
- Currency.cs
- WindowVisualStateTracker.cs
- GlyphManager.cs
- InstanceDataCollectionCollection.cs
- StreamInfo.cs
- ApplicationId.cs
- Point3DCollection.cs
- HyperLink.cs
- BinaryNode.cs
- CodeBlockBuilder.cs
- Model3D.cs
- ValidationErrorEventArgs.cs
- ValidationSummary.cs
- ActiveXHelper.cs
- PrinterUnitConvert.cs
- MimeBasePart.cs
- ToolStripCodeDomSerializer.cs
- CompoundFileStorageReference.cs
- BitmapImage.cs
- ChtmlCommandAdapter.cs
- XamlUtilities.cs
- SignatureToken.cs
- HttpValueCollection.cs
- ReadOnlyMetadataCollection.cs
- ModuleElement.cs
- XmlSignificantWhitespace.cs
- DataGridViewLayoutData.cs
- OleDbInfoMessageEvent.cs
- CacheAxisQuery.cs
- XsdBuildProvider.cs
- GuidConverter.cs
- StringTraceRecord.cs
- Component.cs
- BitmapImage.cs
- OrthographicCamera.cs
- EndpointNameMessageFilter.cs
- HwndTarget.cs
- ReadOnlyMetadataCollection.cs
- DbModificationCommandTree.cs
- SubtreeProcessor.cs
- HostSecurityManager.cs
- Atom10FormatterFactory.cs
- Double.cs
- FileInfo.cs
- UnsafeNativeMethods.cs
- ExpressionCopier.cs
- RegionData.cs
- BindingNavigatorDesigner.cs
- SecurityResources.cs
- IItemProperties.cs
- ConfigurationSectionGroup.cs
- UICuesEvent.cs
- DataControlField.cs
- EntityCommand.cs
- XamlParser.cs
- FixedSOMGroup.cs
- Addressing.cs
- SafeNativeMethods.cs
- PtsCache.cs
- XmlLinkedNode.cs
- ToolStripSeparatorRenderEventArgs.cs
- WmiEventSink.cs
- OrderingQueryOperator.cs
- QueueProcessor.cs
- TextElement.cs
- OracleDataReader.cs
- InProcStateClientManager.cs
- ObjectDataSourceSelectingEventArgs.cs
- ToolBarButtonClickEvent.cs
- SoapSchemaImporter.cs
- SHA512.cs
- MultiTargetingUtil.cs