Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / PerspectiveCamera.cs / 1 / PerspectiveCamera.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class PerspectiveCamera : ProjectionCamera { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new PerspectiveCamera Clone() { return (PerspectiveCamera)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new PerspectiveCamera CloneCurrentValue() { return (PerspectiveCamera)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void FieldOfViewPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { PerspectiveCamera target = ((PerspectiveCamera) d); target.PropertyChanged(FieldOfViewProperty); } #region Public Properties ////// FieldOfView - double. Default value is (double)45.0. /// public double FieldOfView { get { return (double) GetValue(FieldOfViewProperty); } set { SetValueInternal(FieldOfViewProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PerspectiveCamera(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform3D vTransform = Transform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform3D.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } // Obtain handles for animated properties DUCE.ResourceHandle hNearPlaneDistanceAnimations = GetAnimationResourceHandle(NearPlaneDistanceProperty, channel); DUCE.ResourceHandle hFarPlaneDistanceAnimations = GetAnimationResourceHandle(FarPlaneDistanceProperty, channel); DUCE.ResourceHandle hPositionAnimations = GetAnimationResourceHandle(PositionProperty, channel); DUCE.ResourceHandle hLookDirectionAnimations = GetAnimationResourceHandle(LookDirectionProperty, channel); DUCE.ResourceHandle hUpDirectionAnimations = GetAnimationResourceHandle(UpDirectionProperty, channel); DUCE.ResourceHandle hFieldOfViewAnimations = GetAnimationResourceHandle(FieldOfViewProperty, channel); // Pack & send command packet DUCE.MILCMD_PERSPECTIVECAMERA data; unsafe { data.Type = MILCMD.MilCmdPerspectiveCamera; data.Handle = _duceResource.GetHandle(channel); data.htransform = hTransform; if (hNearPlaneDistanceAnimations.IsNull) { data.nearPlaneDistance = NearPlaneDistance; } data.hNearPlaneDistanceAnimations = hNearPlaneDistanceAnimations; if (hFarPlaneDistanceAnimations.IsNull) { data.farPlaneDistance = FarPlaneDistance; } data.hFarPlaneDistanceAnimations = hFarPlaneDistanceAnimations; if (hPositionAnimations.IsNull) { data.position = CompositionResourceManager.Point3DToMilPoint3F(Position); } data.hPositionAnimations = hPositionAnimations; if (hLookDirectionAnimations.IsNull) { data.lookDirection = CompositionResourceManager.Vector3DToMilPoint3F(LookDirection); } data.hLookDirectionAnimations = hLookDirectionAnimations; if (hUpDirectionAnimations.IsNull) { data.upDirection = CompositionResourceManager.Vector3DToMilPoint3F(UpDirection); } data.hUpDirectionAnimations = hUpDirectionAnimations; if (hFieldOfViewAnimations.IsNull) { data.fieldOfView = FieldOfView; } data.hFieldOfViewAnimations = hFieldOfViewAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_PERSPECTIVECAMERA)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_PERSPECTIVECAMERA)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the PerspectiveCamera.FieldOfView property. /// public static readonly DependencyProperty FieldOfViewProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_FieldOfView = (double)45.0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static PerspectiveCamera() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(PerspectiveCamera); FieldOfViewProperty = RegisterProperty("FieldOfView", typeof(double), typeofThis, (double)45.0, new PropertyChangedCallback(FieldOfViewPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class PerspectiveCamera : ProjectionCamera { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new PerspectiveCamera Clone() { return (PerspectiveCamera)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new PerspectiveCamera CloneCurrentValue() { return (PerspectiveCamera)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void FieldOfViewPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { PerspectiveCamera target = ((PerspectiveCamera) d); target.PropertyChanged(FieldOfViewProperty); } #region Public Properties ////// FieldOfView - double. Default value is (double)45.0. /// public double FieldOfView { get { return (double) GetValue(FieldOfViewProperty); } set { SetValueInternal(FieldOfViewProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PerspectiveCamera(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Transform3D vTransform = Transform; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform3D.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } // Obtain handles for animated properties DUCE.ResourceHandle hNearPlaneDistanceAnimations = GetAnimationResourceHandle(NearPlaneDistanceProperty, channel); DUCE.ResourceHandle hFarPlaneDistanceAnimations = GetAnimationResourceHandle(FarPlaneDistanceProperty, channel); DUCE.ResourceHandle hPositionAnimations = GetAnimationResourceHandle(PositionProperty, channel); DUCE.ResourceHandle hLookDirectionAnimations = GetAnimationResourceHandle(LookDirectionProperty, channel); DUCE.ResourceHandle hUpDirectionAnimations = GetAnimationResourceHandle(UpDirectionProperty, channel); DUCE.ResourceHandle hFieldOfViewAnimations = GetAnimationResourceHandle(FieldOfViewProperty, channel); // Pack & send command packet DUCE.MILCMD_PERSPECTIVECAMERA data; unsafe { data.Type = MILCMD.MilCmdPerspectiveCamera; data.Handle = _duceResource.GetHandle(channel); data.htransform = hTransform; if (hNearPlaneDistanceAnimations.IsNull) { data.nearPlaneDistance = NearPlaneDistance; } data.hNearPlaneDistanceAnimations = hNearPlaneDistanceAnimations; if (hFarPlaneDistanceAnimations.IsNull) { data.farPlaneDistance = FarPlaneDistance; } data.hFarPlaneDistanceAnimations = hFarPlaneDistanceAnimations; if (hPositionAnimations.IsNull) { data.position = CompositionResourceManager.Point3DToMilPoint3F(Position); } data.hPositionAnimations = hPositionAnimations; if (hLookDirectionAnimations.IsNull) { data.lookDirection = CompositionResourceManager.Vector3DToMilPoint3F(LookDirection); } data.hLookDirectionAnimations = hLookDirectionAnimations; if (hUpDirectionAnimations.IsNull) { data.upDirection = CompositionResourceManager.Vector3DToMilPoint3F(UpDirection); } data.hUpDirectionAnimations = hUpDirectionAnimations; if (hFieldOfViewAnimations.IsNull) { data.fieldOfView = FieldOfView; } data.hFieldOfViewAnimations = hFieldOfViewAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_PERSPECTIVECAMERA)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_PERSPECTIVECAMERA)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Transform3D vTransform = Transform; if (vTransform != null) ((DUCE.IResource)vTransform).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the PerspectiveCamera.FieldOfView property. /// public static readonly DependencyProperty FieldOfViewProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_FieldOfView = (double)45.0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static PerspectiveCamera() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(PerspectiveCamera); FieldOfViewProperty = RegisterProperty("FieldOfView", typeof(double), typeofThis, (double)45.0, new PropertyChangedCallback(FieldOfViewPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
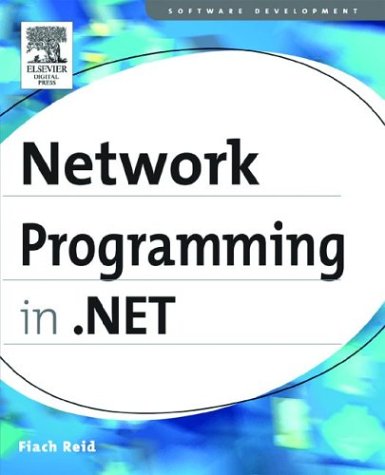
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MsmqIntegrationBindingCollectionElement.cs
- OdbcReferenceCollection.cs
- StorageAssociationTypeMapping.cs
- RenderDataDrawingContext.cs
- ScrollableControl.cs
- dsa.cs
- IRCollection.cs
- SqlMethodAttribute.cs
- WasAdminWrapper.cs
- CheckBox.cs
- BitmapEffectGroup.cs
- PackageRelationship.cs
- EventHandlerList.cs
- TimeManager.cs
- QueryOperatorEnumerator.cs
- MarkupProperty.cs
- _HeaderInfoTable.cs
- _NetworkingPerfCounters.cs
- LexicalChunk.cs
- ButtonField.cs
- SecurityContext.cs
- ConfigXmlCDataSection.cs
- EntityClassGenerator.cs
- DataGridViewCellFormattingEventArgs.cs
- PatternMatcher.cs
- sapiproxy.cs
- DataGridViewCellConverter.cs
- ConditionalAttribute.cs
- AttachedPropertyMethodSelector.cs
- NamespaceQuery.cs
- WinFormsUtils.cs
- ContentType.cs
- WindowHelperService.cs
- TypeGeneratedEventArgs.cs
- HandlerWithFactory.cs
- TextLine.cs
- NotFiniteNumberException.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- PersistenceMetadataNamespace.cs
- DesignerLabelAdapter.cs
- SessionSwitchEventArgs.cs
- DynamicMethod.cs
- DataGridViewButtonCell.cs
- ComNativeDescriptor.cs
- ClientOperation.cs
- InstanceDescriptor.cs
- HtmlSelect.cs
- PerformanceCounterCategory.cs
- TransportBindingElementImporter.cs
- ConfigXmlCDataSection.cs
- WebPartTracker.cs
- FileStream.cs
- DesignTableCollection.cs
- LocatorManager.cs
- NavigationProperty.cs
- SafeLibraryHandle.cs
- BaseCodeDomTreeGenerator.cs
- TextBreakpoint.cs
- BaseValidator.cs
- SrgsElementList.cs
- FacetDescription.cs
- safelink.cs
- CheckBox.cs
- WindowAutomationPeer.cs
- Graphics.cs
- EvidenceBase.cs
- ProcessManager.cs
- CancellationTokenRegistration.cs
- XsdCachingReader.cs
- XmlNodeList.cs
- EventNotify.cs
- AxisAngleRotation3D.cs
- TimerElapsedEvenArgs.cs
- DesignBindingPropertyDescriptor.cs
- DBCommandBuilder.cs
- DrawTreeNodeEventArgs.cs
- LocalizableAttribute.cs
- DateTimeFormatInfoScanner.cs
- CodeBinaryOperatorExpression.cs
- VisualBasicSettingsHandler.cs
- Substitution.cs
- _LocalDataStoreMgr.cs
- WebControlsSection.cs
- EntityDesignerBuildProvider.cs
- ScriptingSectionGroup.cs
- ConnectionPointCookie.cs
- Color.cs
- DocumentXPathNavigator.cs
- _NestedSingleAsyncResult.cs
- DataServiceKeyAttribute.cs
- ParserHooks.cs
- IsolatedStorageFile.cs
- LocatorGroup.cs
- FileSystemWatcher.cs
- ActivityTrace.cs
- QueryStringParameter.cs
- RenderTargetBitmap.cs
- TextEffect.cs
- ErrorRuntimeConfig.cs
- BaseTreeIterator.cs