Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Imaging / TiffBitmapEncoder.cs / 1 / TiffBitmapEncoder.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved // // File: TiffBitmapEncoder.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Security; using System.Security.Permissions; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Imaging; namespace System.Windows.Media.Imaging { #region TiffCompressOption ////// Compress options for saving TIFF bitmap /// public enum TiffCompressOption { ////// Don't care the compression schema /// In this case, the encoder will try to save the bitmap using the best /// compression schema /// Default = 0, ////// No compression /// None = 1, ////// Use CCITT3 compression schema. This works only for Black/white bitmap /// Ccitt3 = 2, ////// Use CCITT4 compression schema. This works only for Black/white bitmap /// Ccitt4 = 3, ////// Use LZW compression schema. /// Lzw = 4, ////// Use RLE compression schema. This works only for Black/white bitmap /// Rle = 5, ////// Use ZIP-deflate compression. /// Zip = 6 }; #endregion #region TiffBitmapEncoder ////// Built-in Encoder for Tiff files. /// public sealed class TiffBitmapEncoder : BitmapEncoder { #region Constructors ////// Constructor for TiffBitmapEncoder /// ////// Critical - will eventually create unmanaged resources /// PublicOK - all inputs are verified /// [SecurityCritical ] public TiffBitmapEncoder() : base(true) { _supportsPreview = false; _supportsGlobalThumbnail = false; _supportsGlobalMetadata = false; _supportsFrameThumbnails = true; _supportsMultipleFrames = true; _supportsFrameMetadata = true; } #endregion #region Public Properties ////// Set the compression type. There are 3 compression types that require the /// format to be BlackWhite (TIFFCompressCCITT3, TIFFCompressCCITT4, and TIFFCompressRLE). /// Setting any of those 3 compression types automatically sets the encode format as well. /// Setting the format to something else will clear any of those compression types. /// public TiffCompressOption Compression { get { return _compressionMethod; } set { _compressionMethod = value; } } #endregion #region Internal Properties / Methods ////// Returns the container format for this encoder /// ////// Critical - uses guid to create unmanaged resources /// internal override Guid ContainerFormat { [SecurityCritical] get { return _containerFormat; } } ////// Returns whether metadata is fixed size or not. /// internal override bool IsMetadataFixedSize { get { return true; } } ////// Setups the encoder and other properties before encoding each frame /// ////// Critical - calls Critical Initialize() /// [SecurityCritical] internal override void SetupFrame(SafeMILHandle frameEncodeHandle, SafeMILHandle encoderOptions) { PROPBAG2 propBag = new PROPBAG2(); PROPVARIANT propValue = new PROPVARIANT(); // There is only one encoder option supported here: if (_compressionMethod != c_defaultCompressionMethod) { try { propBag.Init("TiffCompressionMethod"); propValue.Init((byte) _compressionMethod); HRESULT.Check(UnsafeNativeMethods.IPropertyBag2.Write( encoderOptions, 1, ref propBag, ref propValue)); } finally { propBag.Clear(); propValue.Clear(); } } HRESULT.Check(UnsafeNativeMethods.WICBitmapFrameEncode.Initialize( frameEncodeHandle, encoderOptions )); } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members ////// Critical - CLSID used for creation of critical resources /// [SecurityCritical] private Guid _containerFormat = MILGuidData.GUID_ContainerFormatTiff; private const TiffCompressOption c_defaultCompressionMethod = TiffCompressOption.Default; private TiffCompressOption _compressionMethod = c_defaultCompressionMethod; #endregion } #endregion // TiffBitmapEncoder } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved // // File: TiffBitmapEncoder.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Security; using System.Security.Permissions; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Media.Imaging; namespace System.Windows.Media.Imaging { #region TiffCompressOption ////// Compress options for saving TIFF bitmap /// public enum TiffCompressOption { ////// Don't care the compression schema /// In this case, the encoder will try to save the bitmap using the best /// compression schema /// Default = 0, ////// No compression /// None = 1, ////// Use CCITT3 compression schema. This works only for Black/white bitmap /// Ccitt3 = 2, ////// Use CCITT4 compression schema. This works only for Black/white bitmap /// Ccitt4 = 3, ////// Use LZW compression schema. /// Lzw = 4, ////// Use RLE compression schema. This works only for Black/white bitmap /// Rle = 5, ////// Use ZIP-deflate compression. /// Zip = 6 }; #endregion #region TiffBitmapEncoder ////// Built-in Encoder for Tiff files. /// public sealed class TiffBitmapEncoder : BitmapEncoder { #region Constructors ////// Constructor for TiffBitmapEncoder /// ////// Critical - will eventually create unmanaged resources /// PublicOK - all inputs are verified /// [SecurityCritical ] public TiffBitmapEncoder() : base(true) { _supportsPreview = false; _supportsGlobalThumbnail = false; _supportsGlobalMetadata = false; _supportsFrameThumbnails = true; _supportsMultipleFrames = true; _supportsFrameMetadata = true; } #endregion #region Public Properties ////// Set the compression type. There are 3 compression types that require the /// format to be BlackWhite (TIFFCompressCCITT3, TIFFCompressCCITT4, and TIFFCompressRLE). /// Setting any of those 3 compression types automatically sets the encode format as well. /// Setting the format to something else will clear any of those compression types. /// public TiffCompressOption Compression { get { return _compressionMethod; } set { _compressionMethod = value; } } #endregion #region Internal Properties / Methods ////// Returns the container format for this encoder /// ////// Critical - uses guid to create unmanaged resources /// internal override Guid ContainerFormat { [SecurityCritical] get { return _containerFormat; } } ////// Returns whether metadata is fixed size or not. /// internal override bool IsMetadataFixedSize { get { return true; } } ////// Setups the encoder and other properties before encoding each frame /// ////// Critical - calls Critical Initialize() /// [SecurityCritical] internal override void SetupFrame(SafeMILHandle frameEncodeHandle, SafeMILHandle encoderOptions) { PROPBAG2 propBag = new PROPBAG2(); PROPVARIANT propValue = new PROPVARIANT(); // There is only one encoder option supported here: if (_compressionMethod != c_defaultCompressionMethod) { try { propBag.Init("TiffCompressionMethod"); propValue.Init((byte) _compressionMethod); HRESULT.Check(UnsafeNativeMethods.IPropertyBag2.Write( encoderOptions, 1, ref propBag, ref propValue)); } finally { propBag.Clear(); propValue.Clear(); } } HRESULT.Check(UnsafeNativeMethods.WICBitmapFrameEncode.Initialize( frameEncodeHandle, encoderOptions )); } #endregion #region Internal Abstract /// Need to implement this to derive from the "sealed" object internal override void SealObject() { throw new NotImplementedException(); } #endregion #region Data Members ////// Critical - CLSID used for creation of critical resources /// [SecurityCritical] private Guid _containerFormat = MILGuidData.GUID_ContainerFormatTiff; private const TiffCompressOption c_defaultCompressionMethod = TiffCompressOption.Default; private TiffCompressOption _compressionMethod = c_defaultCompressionMethod; #endregion } #endregion // TiffBitmapEncoder } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
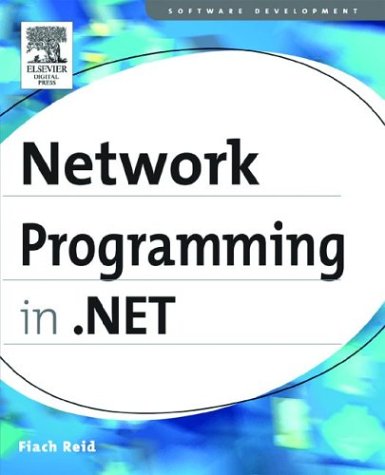
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StandardCommands.cs
- TCEAdapterGenerator.cs
- Glyph.cs
- UrlParameterReader.cs
- GroupedContextMenuStrip.cs
- XPathBuilder.cs
- SettingsAttributes.cs
- ThaiBuddhistCalendar.cs
- LinkLabelLinkClickedEvent.cs
- Pts.cs
- Underline.cs
- BoolExpressionVisitors.cs
- HatchBrush.cs
- XPathNodeHelper.cs
- DrawingVisualDrawingContext.cs
- HttpAsyncResult.cs
- GraphicsContainer.cs
- StaticSiteMapProvider.cs
- Debugger.cs
- PersonalizationDictionary.cs
- MexBindingElement.cs
- SqlDependency.cs
- TextTreeTextNode.cs
- AdvancedBindingEditor.cs
- DodSequenceMerge.cs
- ContentPathSegment.cs
- InternalMappingException.cs
- SafeNativeMethods.cs
- Application.cs
- MetadataArtifactLoaderResource.cs
- SBCSCodePageEncoding.cs
- SocketException.cs
- SQLUtility.cs
- EventLog.cs
- arabicshape.cs
- HandlerBase.cs
- SettingsAttributes.cs
- DecoderReplacementFallback.cs
- SignatureConfirmations.cs
- WriteLine.cs
- CellParaClient.cs
- HttpCacheVaryByContentEncodings.cs
- ExplicitDiscriminatorMap.cs
- WriteTimeStream.cs
- AnnotationComponentChooser.cs
- Group.cs
- VisualTreeHelper.cs
- ModifyActivitiesPropertyDescriptor.cs
- XamlFigureLengthSerializer.cs
- SqlNodeAnnotation.cs
- RedBlackList.cs
- Misc.cs
- HashHelper.cs
- __TransparentProxy.cs
- ResourceManager.cs
- XmlMapping.cs
- PageOutputColor.cs
- BroadcastEventHelper.cs
- TrackingServices.cs
- DoubleLinkList.cs
- followingsibling.cs
- ConfigurationSchemaErrors.cs
- SemaphoreSecurity.cs
- MembershipUser.cs
- PeerNameRecordCollection.cs
- AuthenticationManager.cs
- DeviceContext.cs
- XmlReader.cs
- PropertyChangedEventArgs.cs
- SqlBuilder.cs
- PageParser.cs
- Label.cs
- GridViewUpdatedEventArgs.cs
- SolidColorBrush.cs
- StylusDownEventArgs.cs
- CustomDictionarySources.cs
- XmlSchemaProviderAttribute.cs
- DefaultParameterValueAttribute.cs
- ActivationArguments.cs
- SpeechRecognizer.cs
- XmlDataCollection.cs
- BufferAllocator.cs
- ZipIOBlockManager.cs
- CapabilitiesPattern.cs
- FontCacheLogic.cs
- DesignTimeTemplateParser.cs
- DBCSCodePageEncoding.cs
- String.cs
- HtmlAnchor.cs
- FormViewModeEventArgs.cs
- ScrollItemPatternIdentifiers.cs
- ApplicationSettingsBase.cs
- PreviewPrintController.cs
- CryptoApi.cs
- SystemBrushes.cs
- Size3DConverter.cs
- MailSettingsSection.cs
- IntSecurity.cs
- CorrelationManager.cs
- ImmutableObjectAttribute.cs