Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Ink / Quad.cs / 1 / Quad.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { ////// A helper structure used in StrokeNode and StrokeNodeOperation implementations /// to store endpoints of the quad connecting two nodes of a stroke. /// The vertices of a quad are supposed to be clockwise with points A and D located /// on the begin node and B and C on the end one. /// internal struct Quad { #region Statics private static Quad s_empty = new Quad(new Point(0, 0), new Point(0, 0), new Point(0, 0), new Point(0, 0)); #endregion #region API ///Returns the static object representing an empty (unitialized) quad internal static Quad Empty { get { return s_empty; } } ///Constructor internal Quad(Point a, Point b, Point c, Point d) { _A = a; _B = b; _C = c; _D = d; } ///The A vertex of the quad internal Point A { get { return _A; } set { _A = value; } } ///The B vertex of the quad internal Point B { get { return _B; } set { _B = value; } } ///The C vertex of the quad internal Point C { get { return _C; } set { _C = value; } } ///The D vertex of the quad internal Point D { get { return _D; } set { _D = value; } } // Returns quad's vertex by index where A is of the index 0, B - is 1, etc internal Point this[int index] { get { switch (index) { case 0: return _A; case 1: return _B; case 2: return _C; case 3: return _D; default: throw new IndexOutOfRangeException("index"); } } } ///Tells whether the quad is invalid (empty) internal bool IsEmpty { get { return (_A == _B) && (_C == _D); } } internal void GetPoints(ListpointBuffer) { pointBuffer.Add(_A); pointBuffer.Add(_B); pointBuffer.Add(_C); pointBuffer.Add(_D); } /// Returns the bounds of the quad internal Rect Bounds { get { return IsEmpty ? Rect.Empty : Rect.Union(new Rect(_A, _B), new Rect(_C, _D)); } } #endregion #region Fields private Point _A; private Point _B; private Point _C; private Point _D; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { ////// A helper structure used in StrokeNode and StrokeNodeOperation implementations /// to store endpoints of the quad connecting two nodes of a stroke. /// The vertices of a quad are supposed to be clockwise with points A and D located /// on the begin node and B and C on the end one. /// internal struct Quad { #region Statics private static Quad s_empty = new Quad(new Point(0, 0), new Point(0, 0), new Point(0, 0), new Point(0, 0)); #endregion #region API ///Returns the static object representing an empty (unitialized) quad internal static Quad Empty { get { return s_empty; } } ///Constructor internal Quad(Point a, Point b, Point c, Point d) { _A = a; _B = b; _C = c; _D = d; } ///The A vertex of the quad internal Point A { get { return _A; } set { _A = value; } } ///The B vertex of the quad internal Point B { get { return _B; } set { _B = value; } } ///The C vertex of the quad internal Point C { get { return _C; } set { _C = value; } } ///The D vertex of the quad internal Point D { get { return _D; } set { _D = value; } } // Returns quad's vertex by index where A is of the index 0, B - is 1, etc internal Point this[int index] { get { switch (index) { case 0: return _A; case 1: return _B; case 2: return _C; case 3: return _D; default: throw new IndexOutOfRangeException("index"); } } } ///Tells whether the quad is invalid (empty) internal bool IsEmpty { get { return (_A == _B) && (_C == _D); } } internal void GetPoints(ListpointBuffer) { pointBuffer.Add(_A); pointBuffer.Add(_B); pointBuffer.Add(_C); pointBuffer.Add(_D); } /// Returns the bounds of the quad internal Rect Bounds { get { return IsEmpty ? Rect.Empty : Rect.Union(new Rect(_A, _B), new Rect(_C, _D)); } } #endregion #region Fields private Point _A; private Point _B; private Point _C; private Point _D; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
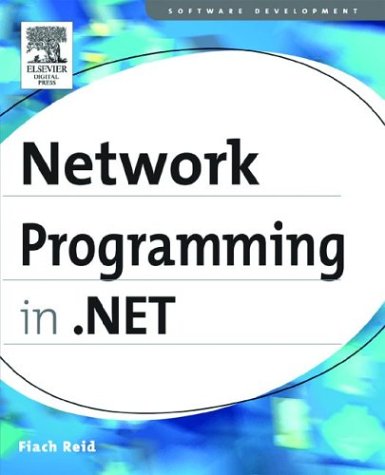
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeRegistryHandle.cs
- SqlAliasesReferenced.cs
- QueryMath.cs
- KeyedCollection.cs
- UIElement3D.cs
- ADMembershipUser.cs
- MimePart.cs
- FileLevelControlBuilderAttribute.cs
- RelationshipConverter.cs
- PermissionToken.cs
- CompositeDataBoundControl.cs
- ReferencedCollectionType.cs
- IEnumerable.cs
- ModuleBuilderData.cs
- MetadataHelper.cs
- FileDialog_Vista_Interop.cs
- WebPartZone.cs
- ContextQuery.cs
- BasicDesignerLoader.cs
- DependencyProperty.cs
- PackUriHelper.cs
- WorkflowWebService.cs
- TypeConverterHelper.cs
- SafeWaitHandle.cs
- Matrix.cs
- ImageDrawing.cs
- Converter.cs
- MessageBox.cs
- MessageDecoder.cs
- BamlLocalizer.cs
- Attachment.cs
- Page.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- XmlDocumentSerializer.cs
- EffectiveValueEntry.cs
- SQLBinaryStorage.cs
- GuidelineSet.cs
- SoapMessage.cs
- EventLogPermissionEntryCollection.cs
- dbdatarecord.cs
- NullPackagingPolicy.cs
- DelayedRegex.cs
- OdbcPermission.cs
- OracleColumn.cs
- TransformValueSerializer.cs
- VisualStyleTypesAndProperties.cs
- UpdateRecord.cs
- serverconfig.cs
- CopyOfAction.cs
- FieldAccessException.cs
- ListViewTableRow.cs
- AmbiguousMatchException.cs
- StorageScalarPropertyMapping.cs
- ColumnClickEvent.cs
- UnitySerializationHolder.cs
- WebPartMinimizeVerb.cs
- XmlDocumentType.cs
- SqlConnectionPoolProviderInfo.cs
- FocusChangedEventArgs.cs
- XmlDeclaration.cs
- ConnectionManagementElement.cs
- IPGlobalProperties.cs
- ToolStripRenderEventArgs.cs
- XmlObjectSerializerReadContextComplexJson.cs
- ComponentResourceManager.cs
- SyndicationFeed.cs
- BaseValidatorDesigner.cs
- DataGridColumn.cs
- DnsPermission.cs
- ProcessManager.cs
- ElapsedEventArgs.cs
- VisualStyleRenderer.cs
- MappedMetaModel.cs
- Pair.cs
- MessageSecurityOverMsmq.cs
- BmpBitmapEncoder.cs
- ScriptBehaviorDescriptor.cs
- Exception.cs
- EntitySqlQueryBuilder.cs
- ScrollViewer.cs
- RoleManagerModule.cs
- DataView.cs
- Mutex.cs
- TextElementEnumerator.cs
- XamlSerializationHelper.cs
- TextServicesContext.cs
- DBCommand.cs
- Attributes.cs
- ResourceAttributes.cs
- WebColorConverter.cs
- LinearGradientBrush.cs
- DrawingCollection.cs
- TriggerAction.cs
- AtomContentProperty.cs
- DecimalAnimationBase.cs
- ScriptComponentDescriptor.cs
- MoveSizeWinEventHandler.cs
- RowSpanVector.cs
- SerializableAttribute.cs
- ObjectKeyFrameCollection.cs