Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / QueryMath.cs / 1 / QueryMath.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System.Collections; using System.Diagnostics; internal enum MathOperator { None, Plus, Minus, Div, Multiply, Mod, Negate } internal class MathOpcode : Opcode { MathOperator mathOp; internal MathOpcode(OpcodeID id, MathOperator op) : base(id) { this.mathOp = op; } internal override bool Equals(Opcode op) { if (base.Equals(op)) { return (this.mathOp == ((MathOpcode) op).mathOp); } return false; } #if DEBUG_FILTER public override string ToString() { return string.Format("{0} {1}", base.ToString(), this.mathOp.ToString()); } #endif } internal class PlusOpcode : MathOpcode { internal PlusOpcode() : base(OpcodeID.Plus, MathOperator.Plus) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); Value[] values = context.Values; for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Add(values[x].Double); } context.PopFrame(); return this.next; } } internal class MinusOpcode : MathOpcode { internal MinusOpcode() : base(OpcodeID.Minus, MathOperator.Minus) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); Value[] values = context.Values; for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Double = values[x].Double - values[y].Double; } context.PopFrame(); return this.next; } } internal class MultiplyOpcode : MathOpcode { internal MultiplyOpcode() : base(OpcodeID.Multiply, MathOperator.Multiply) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); Value[] values = context.Values; for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Multiply(values[x].Double); } context.PopFrame(); return this.next; } } internal class DivideOpcode : MathOpcode { internal DivideOpcode() : base(OpcodeID.Divide, MathOperator.Div) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); Value[] values = context.Values; for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Double = values[x].Double / values[y].Double; } context.PopFrame(); return this.next; } } internal class ModulusOpcode : MathOpcode { internal ModulusOpcode() : base(OpcodeID.Mod, MathOperator.Mod) { } internal override Opcode Eval(ProcessingContext context) { StackFrame argX = context.TopArg; StackFrame argY = context.SecondArg; Value[] values = context.Values; DiagnosticUtility.DebugAssert(argX.Count == argY.Count, ""); for (int x = argX.basePtr, y = argY.basePtr; x <= argX.endPtr; ++x, ++y) { DiagnosticUtility.DebugAssert(values[x].IsType(ValueDataType.Double), ""); DiagnosticUtility.DebugAssert(values[y].IsType(ValueDataType.Double), ""); values[y].Double = values[x].Double % values[y].Double; } context.PopFrame(); return this.next; } } internal class NegateOpcode : MathOpcode { internal NegateOpcode() : base(OpcodeID.Negate, MathOperator.Negate) { } internal override Opcode Eval(ProcessingContext context) { StackFrame frame = context.TopArg; Value[] values = context.Values; for (int i = frame.basePtr; i <= frame.endPtr; ++i) { values[i].Negate(); } return this.next; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
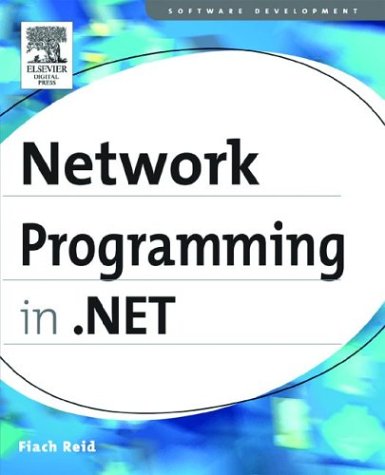
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebServiceReceiveDesigner.cs
- EventLogEntryCollection.cs
- ParseNumbers.cs
- Descriptor.cs
- TypeCollectionDesigner.xaml.cs
- ComboBox.cs
- XamlTreeBuilderBamlRecordWriter.cs
- TextViewSelectionProcessor.cs
- DataError.cs
- CodeLabeledStatement.cs
- UdpDiscoveryMessageFilter.cs
- XmlSchemaChoice.cs
- ColorAnimationBase.cs
- TextEditorContextMenu.cs
- Stacktrace.cs
- OperandQuery.cs
- EnterpriseServicesHelper.cs
- DataSourceGroupCollection.cs
- Function.cs
- SiteMap.cs
- GroupByExpressionRewriter.cs
- FileLevelControlBuilderAttribute.cs
- ContentPlaceHolder.cs
- OrCondition.cs
- DataServiceBehavior.cs
- contentDescriptor.cs
- templategroup.cs
- XmlCharType.cs
- SrgsText.cs
- RoutedEventHandlerInfo.cs
- MetadataElement.cs
- StateManagedCollection.cs
- DocumentGridPage.cs
- StreamGeometry.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs
- DPAPIProtectedConfigurationProvider.cs
- SystemNetHelpers.cs
- SerializationBinder.cs
- Win32NamedPipes.cs
- UpdatePanel.cs
- WebConfigurationFileMap.cs
- DuplicateWaitObjectException.cs
- XsdDuration.cs
- ToolStripOverflowButton.cs
- Registry.cs
- TextEditorSpelling.cs
- BatchParser.cs
- StatusBarItem.cs
- AutomationProperty.cs
- ValueSerializerAttribute.cs
- DataGridItemCollection.cs
- ProfileProvider.cs
- FunctionUpdateCommand.cs
- WindowsListViewScroll.cs
- StructuredTypeInfo.cs
- LocationUpdates.cs
- XomlCompilerError.cs
- ArgumentNullException.cs
- ZoneButton.cs
- XmlSchemaProviderAttribute.cs
- ReliableOutputSessionChannel.cs
- EntityStoreSchemaGenerator.cs
- ResourceReferenceExpressionConverter.cs
- FormViewActionList.cs
- SelectionItemPattern.cs
- TriggerAction.cs
- ILGenerator.cs
- URIFormatException.cs
- Bidi.cs
- Wildcard.cs
- TreeNodeBindingCollection.cs
- UInt16.cs
- DeferredReference.cs
- CheckBoxRenderer.cs
- RootBuilder.cs
- CompilerResults.cs
- IssuedTokenServiceElement.cs
- DesignerLoader.cs
- MemberMemberBinding.cs
- NamespaceDecl.cs
- Html32TextWriter.cs
- IList.cs
- MessageBox.cs
- ThreadAttributes.cs
- ComponentResourceKey.cs
- Pkcs7Recipient.cs
- SuppressMessageAttribute.cs
- ErasingStroke.cs
- ScaleTransform3D.cs
- LicFileLicenseProvider.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs
- PropertyDescriptor.cs
- HMACSHA384.cs
- SqlBooleanMismatchVisitor.cs
- ConversionContext.cs
- ITextView.cs
- RoamingStoreFileUtility.cs
- _NetworkingPerfCounters.cs
- EventLogEntryCollection.cs
- CompleteWizardStep.cs