Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Security / Cryptography / HashAlgorithm.cs / 1 / HashAlgorithm.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // HashAlgorithm.cs // namespace System.Security.Cryptography { using System.IO; [System.Runtime.InteropServices.ComVisible(true)] public abstract class HashAlgorithm : ICryptoTransform { protected int HashSizeValue; protected internal byte[] HashValue; protected int State = 0; private bool m_bDisposed = false; protected HashAlgorithm() {} // // public properties // public virtual int HashSize { get { return HashSizeValue; } } public virtual byte[] Hash { get { if (m_bDisposed) throw new ObjectDisposedException(null, Environment.GetResourceString("ObjectDisposed_Generic")); if (State != 0) throw new CryptographicUnexpectedOperationException(Environment.GetResourceString("Cryptography_HashNotYetFinalized")); return (byte[]) HashValue.Clone(); } } // // public methods // static public HashAlgorithm Create() { return Create("System.Security.Cryptography.HashAlgorithm"); } static public HashAlgorithm Create(String hashName) { return (HashAlgorithm) CryptoConfig.CreateFromName(hashName); } public byte[] ComputeHash(Stream inputStream) { if (m_bDisposed) throw new ObjectDisposedException(null, Environment.GetResourceString("ObjectDisposed_Generic")); // Default the buffer size to 4K. byte[] buffer = new byte[4096]; int bytesRead; do { bytesRead = inputStream.Read(buffer, 0, 4096); if (bytesRead > 0) { HashCore(buffer, 0, bytesRead); } } while (bytesRead > 0); HashValue = HashFinal(); byte[] Tmp = (byte[]) HashValue.Clone(); Initialize(); return(Tmp); } public byte[] ComputeHash(byte[] buffer) { if (m_bDisposed) throw new ObjectDisposedException(null, Environment.GetResourceString("ObjectDisposed_Generic")); // Do some validation if (buffer == null) throw new ArgumentNullException("buffer"); HashCore(buffer, 0, buffer.Length); HashValue = HashFinal(); byte[] Tmp = (byte[]) HashValue.Clone(); Initialize(); return(Tmp); } public byte[] ComputeHash(byte[] buffer, int offset, int count) { if (m_bDisposed) throw new ObjectDisposedException(null, Environment.GetResourceString("ObjectDisposed_Generic")); // Do some validation if (buffer == null) throw new ArgumentNullException("buffer"); if (offset < 0) throw new ArgumentOutOfRangeException("offset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0 || (count > buffer.Length)) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidValue")); if ((buffer.Length - count) < offset) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); HashCore(buffer, offset, count); HashValue = HashFinal(); byte[] Tmp = (byte[]) HashValue.Clone(); Initialize(); return(Tmp); } // ICryptoTransform methods // we assume any HashAlgorithm can take input a byte at a time public virtual int InputBlockSize { get { return(1); } } public virtual int OutputBlockSize { get { return(1); } } public virtual bool CanTransformMultipleBlocks { get { return(true); } } public virtual bool CanReuseTransform { get { return(true); } } // We implement TransformBlock and TransformFinalBlock here public int TransformBlock(byte[] inputBuffer, int inputOffset, int inputCount, byte[] outputBuffer, int outputOffset) { if (m_bDisposed) throw new ObjectDisposedException(null, Environment.GetResourceString("ObjectDisposed_Generic")); // Do some validation, we let BlockCopy do the destination array validation if (inputBuffer == null) throw new ArgumentNullException("inputBuffer"); if (inputOffset < 0) throw new ArgumentOutOfRangeException("inputOffset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (inputCount < 0 || (inputCount > inputBuffer.Length)) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidValue")); if ((inputBuffer.Length - inputCount) < inputOffset) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); // Change the State value State = 1; HashCore(inputBuffer, inputOffset, inputCount); if ((outputBuffer != null) && ((inputBuffer != outputBuffer) || (inputOffset != outputOffset))) Buffer.BlockCopy(inputBuffer, inputOffset, outputBuffer, outputOffset, inputCount); return inputCount; } public byte[] TransformFinalBlock(byte[] inputBuffer, int inputOffset, int inputCount) { if (m_bDisposed) throw new ObjectDisposedException(null, Environment.GetResourceString("ObjectDisposed_Generic")); // Do some validation if (inputBuffer == null) throw new ArgumentNullException("inputBuffer"); if (inputOffset < 0) throw new ArgumentOutOfRangeException("inputOffset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (inputCount < 0 || (inputCount > inputBuffer.Length)) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidValue")); if ((inputBuffer.Length - inputCount) < inputOffset) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); HashCore(inputBuffer, inputOffset, inputCount); HashValue = HashFinal(); byte[] outputBytes = new byte[inputCount]; if (inputCount != 0) Buffer.InternalBlockCopy(inputBuffer, inputOffset, outputBytes, 0, inputCount); // reset the State value State = 0; return outputBytes; } // IDisposable methods ///void IDisposable.Dispose() { Dispose(true); GC.SuppressFinalize(this); } public void Clear() { ((IDisposable) this).Dispose(); } protected virtual void Dispose(bool disposing) { if (disposing) { if (HashValue != null) Array.Clear(HashValue, 0, HashValue.Length); HashValue = null; m_bDisposed = true; } } // // abstract public methods // public abstract void Initialize(); protected abstract void HashCore(byte[] array, int ibStart, int cbSize); protected abstract byte[] HashFinal(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
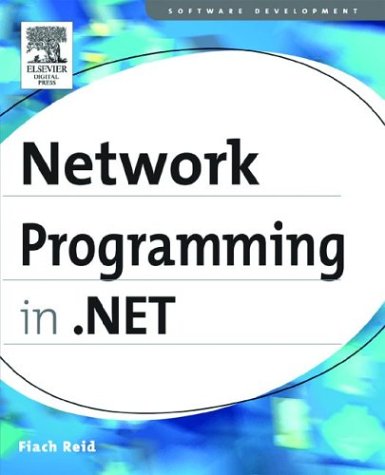
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlValueConverter.cs
- Fonts.cs
- LayoutTableCell.cs
- cache.cs
- BaseAddressPrefixFilterElement.cs
- Visitors.cs
- StringArrayEditor.cs
- WebSysDefaultValueAttribute.cs
- ReceiveMessageContent.cs
- TypeConverterAttribute.cs
- Int32Collection.cs
- SHA256.cs
- SiteMapNode.cs
- TypeConverterAttribute.cs
- XmlProcessingInstruction.cs
- ExtensionSimplifierMarkupObject.cs
- CodeEntryPointMethod.cs
- ThreadWorkerController.cs
- ClientTargetCollection.cs
- SocketAddress.cs
- HwndAppCommandInputProvider.cs
- ListControlDesigner.cs
- HMACSHA512.cs
- StyleSheet.cs
- CacheHelper.cs
- WindowsContainer.cs
- UserInitiatedNavigationPermission.cs
- SoapSchemaImporter.cs
- ProjectionCamera.cs
- SharedUtils.cs
- ObjectDataSourceStatusEventArgs.cs
- EntityUtil.cs
- TranslateTransform3D.cs
- JoinSymbol.cs
- SpAudioStreamWrapper.cs
- WebUtil.cs
- UTF7Encoding.cs
- ToolBarOverflowPanel.cs
- HashHelpers.cs
- Geometry3D.cs
- RequestCachingSection.cs
- EntityDataSourceViewSchema.cs
- HostingMessageProperty.cs
- BaseResourcesBuildProvider.cs
- BrushConverter.cs
- SystemTcpStatistics.cs
- InputProcessorProfilesLoader.cs
- DbCommandTree.cs
- SecurityUtils.cs
- CodeSnippetStatement.cs
- SerializationInfo.cs
- Crypto.cs
- OrthographicCamera.cs
- ExpandableObjectConverter.cs
- CreateParams.cs
- Context.cs
- HtmlWindow.cs
- SessionState.cs
- RuleRefElement.cs
- TextRange.cs
- XmlSerializationGeneratedCode.cs
- Assembly.cs
- GPStream.cs
- TextTreeExtractElementUndoUnit.cs
- OleDbReferenceCollection.cs
- ToolStripOverflow.cs
- SymbolPair.cs
- XmlElementAttribute.cs
- UnaryOperationBinder.cs
- FlowSwitchLink.cs
- MSHTMLHost.cs
- ToReply.cs
- ColorConverter.cs
- GridViewAutoFormat.cs
- TextSelectionHighlightLayer.cs
- Menu.cs
- Thumb.cs
- DebugView.cs
- RegionInfo.cs
- printdlgexmarshaler.cs
- WindowsGrip.cs
- HostingEnvironmentException.cs
- OutputCacheProfileCollection.cs
- DesignerActionVerbList.cs
- MILUtilities.cs
- AgileSafeNativeMemoryHandle.cs
- InternalControlCollection.cs
- ZipIOCentralDirectoryFileHeader.cs
- DesignTimeTemplateParser.cs
- VisualStyleElement.cs
- TableParagraph.cs
- DPAPIProtectedConfigurationProvider.cs
- WebConfigurationFileMap.cs
- LicenseProviderAttribute.cs
- VideoDrawing.cs
- UnsafeNativeMethodsTablet.cs
- EncoderExceptionFallback.cs
- XmlILModule.cs
- ConnectorDragDropGlyph.cs
- ListBase.cs