Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridBoolColumn.cs / 1305376 / DataGridBoolColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Windows.Forms; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; ////// /// public class DataGridBoolColumn : DataGridColumnStyle { private static readonly int idealCheckSize = 14; private bool isEditing = false; private bool isSelected = false; private bool allowNull = true; private int editingRow = -1; private object currentValue = Convert.DBNull; private object trueValue = true; private object falseValue = false; private object nullValue = Convert.DBNull; private static readonly object EventTrueValue = new object(); private static readonly object EventFalseValue = new object(); private static readonly object EventAllowNull = new object(); ///Specifies a column in /// which each cell contains a check box for representing /// a boolean value. ////// /// public DataGridBoolColumn() : base() {} ///Initializes a new instance of the ///class. /// /// public DataGridBoolColumn(PropertyDescriptor prop) : base(prop) {} ///Initializes a new instance of a ///with the specified . /// /// public DataGridBoolColumn(PropertyDescriptor prop, bool isDefault) : base(prop, isDefault){} ///[To be supplied.] ////// /// [TypeConverterAttribute(typeof(StringConverter)), DefaultValue(true)] public object TrueValue { get { return trueValue; } set { if (!trueValue.Equals(value)) { this.trueValue = value; OnTrueValueChanged(EventArgs.Empty); Invalidate(); } } } ///Gets or sets the actual value used when setting the /// value of the column to ///. public event EventHandler TrueValueChanged { add { Events.AddHandler(EventTrueValue, value); } remove { Events.RemoveHandler(EventTrueValue, value); } } /// /// /// [TypeConverterAttribute(typeof(StringConverter)), DefaultValue(false)] public object FalseValue { get { return falseValue; } set { if (!falseValue.Equals(value)) { this.falseValue = value; OnFalseValueChanged(EventArgs.Empty); Invalidate(); } } } ///Gets or sets the actual value used when setting the value of the column to /// ///. public event EventHandler FalseValueChanged { add { Events.AddHandler(EventFalseValue, value); } remove { Events.RemoveHandler(EventFalseValue, value); } } /// /// /// [TypeConverterAttribute(typeof(StringConverter))] public object NullValue { get { return nullValue; } set { if (!nullValue.Equals(value)) { this.nullValue = value; OnFalseValueChanged(EventArgs.Empty); Invalidate(); } } } // =----------------------------------------------------------------- // = Methods // =----------------------------------------------------------------- // when the grid is in addNewRow it means that the user did not start typing // so there is no data to be pushed back into the backEnd. // make isEditing false so that in the Commit call we do not do any work. // ///Gets or sets the actual value used when setting the value of the column to /// ///. protected internal override void ConcedeFocus() { base.ConcedeFocus(); this.isSelected = false; this.isEditing = false; } private Rectangle GetCheckBoxBounds(Rectangle bounds, bool alignToRight) { if (alignToRight) return new Rectangle(bounds.X +((bounds.Width - idealCheckSize) /2), bounds.Y +((bounds.Height - idealCheckSize) / 2), bounds.Width < idealCheckSize ? bounds.Width : idealCheckSize, idealCheckSize); else return new Rectangle(Math.Max(0,bounds.X +((bounds.Width - idealCheckSize) /2)), Math.Max(0,bounds.Y +((bounds.Height - idealCheckSize) / 2)), bounds.Width < idealCheckSize ? bounds.Width : idealCheckSize, idealCheckSize); } /// /// /// protected internal override object GetColumnValueAtRow(CurrencyManager lm, int row) { object baseValue = base.GetColumnValueAtRow(lm, row); object value = Convert.DBNull; if (baseValue.Equals(trueValue)) { value = true; } else if (baseValue.Equals(falseValue)) { value = false; } return value; } private bool IsReadOnly() { bool ret = this.ReadOnly; if (this.DataGridTableStyle != null) { ret = ret || this.DataGridTableStyle.ReadOnly; if (this.DataGridTableStyle.DataGrid != null) ret = ret || this.DataGridTableStyle.DataGrid.ReadOnly; } return ret; } ///Gets the value at the specified row. ////// /// protected internal override void SetColumnValueAtRow(CurrencyManager lm, int row, object value) { object baseValue = null; if (true.Equals(value)) { baseValue = TrueValue; } else if (false.Equals(value)) { baseValue = FalseValue; } else if (Convert.IsDBNull(value)) { baseValue = NullValue; } currentValue = baseValue; base.SetColumnValueAtRow(lm, row, baseValue); } ///Sets the value a a specified row. ////// /// protected internal override Size GetPreferredSize(Graphics g, object value) { return new Size(idealCheckSize+2, idealCheckSize+2); } ///Gets the optimum width and height of a cell given /// a specific value to contain. ////// /// protected internal override int GetMinimumHeight() { return idealCheckSize+2; } ///Gets /// the height of a cell in a column. ////// /// protected internal override int GetPreferredHeight(Graphics g, object value) { return idealCheckSize + 2; } ////// Gets the height used when resizing columns. /// ////// /// protected internal override void Abort(int rowNum) { isSelected = false; isEditing = false; Invalidate(); return; } ////// Initiates a request to interrupt an edit procedure. /// ////// /// protected internal override bool Commit(CurrencyManager dataSource, int rowNum) { isSelected = false; // always invalidate Invalidate(); if (!isEditing) return true; SetColumnValueAtRow(dataSource, rowNum, currentValue); isEditing = false; return true; } ////// Initiates a request to complete an editing procedure. /// ////// /// protected internal override void Edit(CurrencyManager source, int rowNum, Rectangle bounds, bool readOnly, string displayText, bool cellIsVisible) { // toggle state right now... isSelected = true; // move the focus away from the previous column and give it to the grid // DataGrid grid = this.DataGridTableStyle.DataGrid; if (!grid.Focused) grid.FocusInternal(); if (!readOnly && !IsReadOnly()) { editingRow = rowNum; currentValue = GetColumnValueAtRow(source, rowNum); } base.Invalidate(); } ////// Prepares the cell for editing a value. /// ////// internal override bool KeyPress(int rowNum, Keys keyData) { if (isSelected && editingRow == rowNum && !IsReadOnly()) { if ((keyData & Keys.KeyCode) == Keys.Space) { ToggleValue(); Invalidate(); return true; } } return base.KeyPress(rowNum, keyData); } ////// Provides a handler for determining which key was pressed, and whether to /// process it. /// ////// /// internal override bool MouseDown(int rowNum, int x, int y) { base.MouseDown(rowNum, x, y); if (isSelected && editingRow == rowNum && !IsReadOnly()) { ToggleValue(); Invalidate(); return true; } return false; } private void OnTrueValueChanged(EventArgs e) { EventHandler eh = this.Events[EventTrueValue] as EventHandler; if (eh != null) eh(this, e); } private void OnFalseValueChanged(EventArgs e) { EventHandler eh = this.Events[EventFalseValue] as EventHandler; if (eh != null) eh(this, e); } private void OnAllowNullChanged(EventArgs e) { EventHandler eh = this.Events[EventAllowNull] as EventHandler; if (eh != null) eh(this, e); } ////// Indicates whether the a mouse down event occurred at the specified row, at /// the specified x and y coordinates. /// ////// /// /// protected internal override void Paint(Graphics g, Rectangle bounds, CurrencyManager source, int rowNum) { Paint(g,bounds,source, rowNum, false); } ///Draws the ////// with the given , /// and row number. /// /// /// protected internal override void Paint(Graphics g, Rectangle bounds, CurrencyManager source, int rowNum, bool alignToRight) { Paint(g,bounds,source, rowNum, this.DataGridTableStyle.BackBrush, this.DataGridTableStyle.ForeBrush, alignToRight); } ///Draws the ////// with the given , , /// row number, and alignment settings. /// /// protected internal override void Paint(Graphics g, Rectangle bounds, CurrencyManager source, int rowNum, Brush backBrush, Brush foreBrush, bool alignToRight) { object value = (isEditing && editingRow == rowNum) ? currentValue : GetColumnValueAtRow(source, rowNum); ButtonState checkedState = ButtonState.Inactive; if (!Convert.IsDBNull(value)) { checkedState = ((bool)value ? ButtonState.Checked : ButtonState.Normal); } Rectangle box = GetCheckBoxBounds(bounds, alignToRight); Region r = g.Clip; g.ExcludeClip(box); System.Drawing.Brush selectionBrush = this.DataGridTableStyle.IsDefault ? this.DataGridTableStyle.DataGrid.SelectionBackBrush : this.DataGridTableStyle.SelectionBackBrush; if (isSelected && editingRow == rowNum && !IsReadOnly()) { g.FillRectangle(selectionBrush, bounds); } else g.FillRectangle(backBrush, bounds); g.Clip = r; if (checkedState == ButtonState.Inactive) { ControlPaint.DrawMixedCheckBox(g, box, ButtonState.Checked); } else { ControlPaint.DrawCheckBox(g, box, checkedState); } // if the column is read only we should still show selection if (IsReadOnly() && isSelected && source.Position == rowNum) { bounds.Inflate(-1,-1); System.Drawing.Pen pen = new System.Drawing.Pen(selectionBrush); pen.DashStyle = System.Drawing.Drawing2D.DashStyle.Dash; g.DrawRectangle(pen, bounds); pen.Dispose(); // restore the bounds rectangle bounds.Inflate(1,1); } } ///Draws the ///with the given , , /// row number, , and . /// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.DataGridBoolColumnAllowNullValue) ] public bool AllowNull { get { return allowNull; } set { if (allowNull != value) { allowNull = value; // if we do not allow null, and the gridColumn had // a null in it, discard it if (!value && Convert.IsDBNull(currentValue)) { currentValue = false; Invalidate(); } OnAllowNullChanged(EventArgs.Empty); } } } ///Gets or sets a value indicating whether null values are allowed. ///public event EventHandler AllowNullChanged { add { Events.AddHandler(EventAllowNull, value); } remove { Events.RemoveHandler(EventAllowNull, value); } } /// /// /// protected internal override void EnterNullValue() { // do not throw an exception when the column is marked as readOnly or // does not allowNull if (!this.AllowNull || IsReadOnly()) return; if (currentValue != Convert.DBNull) { currentValue = Convert.DBNull; Invalidate(); } } private void ResetNullValue() { NullValue = Convert.DBNull; } private bool ShouldSerializeNullValue() { return nullValue != Convert.DBNull; } private void ToggleValue() { if (currentValue is bool && ((bool)currentValue) == false) { currentValue = true; } else { if (AllowNull) { if (Convert.IsDBNull(currentValue)) { currentValue = false; } else { currentValue = Convert.DBNull; } } else { currentValue = false; } } // we started editing isEditing = true; // tell the dataGrid that things are changing // we put Rectangle.Empty cause toggle will invalidate the row anyhow this.DataGridTableStyle.DataGrid.ColumnStartedEditing(Rectangle.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Enters a ///into the column.
Link Menu
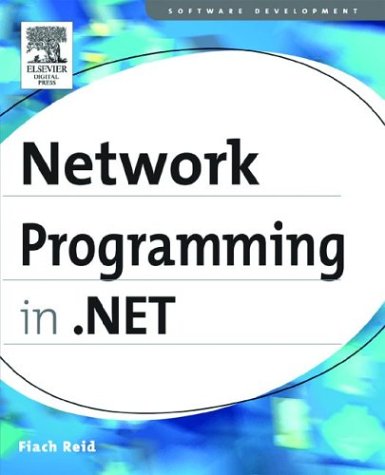
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectSecurity.cs
- CurrencyManager.cs
- loginstatus.cs
- MachineSettingsSection.cs
- HtmlInputReset.cs
- HotSpotCollection.cs
- XPathAxisIterator.cs
- ToolStripGrip.cs
- SoapFault.cs
- _SpnDictionary.cs
- HintTextConverter.cs
- ImageClickEventArgs.cs
- RawUIStateInputReport.cs
- IsolatedStorageFileStream.cs
- CachedBitmap.cs
- Triplet.cs
- RootNamespaceAttribute.cs
- RenderCapability.cs
- XmlCharType.cs
- LogExtent.cs
- HttpHostedTransportConfiguration.cs
- Light.cs
- CssStyleCollection.cs
- OracleDataReader.cs
- DbConnectionInternal.cs
- MenuEventArgs.cs
- DelegatingHeader.cs
- OdbcEnvironmentHandle.cs
- DataGridLinkButton.cs
- DirectoryObjectSecurity.cs
- ListDictionary.cs
- NativeMethods.cs
- AsymmetricSecurityProtocolFactory.cs
- MiniAssembly.cs
- RemotingConfiguration.cs
- BoundColumn.cs
- TrackingServices.cs
- FixedPageStructure.cs
- WebPartConnectionsCancelVerb.cs
- HandleCollector.cs
- Filter.cs
- MetadataFile.cs
- MediaContext.cs
- EntityCollectionChangedParams.cs
- BitmapVisualManager.cs
- StorageFunctionMapping.cs
- DocumentReferenceCollection.cs
- DbDataAdapter.cs
- VoiceInfo.cs
- DataGridViewEditingControlShowingEventArgs.cs
- XmlNodeWriter.cs
- MaterialCollection.cs
- SingleObjectCollection.cs
- StateDesignerConnector.cs
- GeneratedView.cs
- Select.cs
- TimeoutException.cs
- DesignBindingPropertyDescriptor.cs
- DataBindingHandlerAttribute.cs
- XmlUTF8TextReader.cs
- OperationCanceledException.cs
- LinkLabelLinkClickedEvent.cs
- NotificationContext.cs
- ValueUnavailableException.cs
- CodeStatementCollection.cs
- RequestChannel.cs
- XmlSchemaException.cs
- ExpandedWrapper.cs
- TraceContext.cs
- WebSysDescriptionAttribute.cs
- CodeAssignStatement.cs
- TableLayoutRowStyleCollection.cs
- SafeHandles.cs
- DelegatingMessage.cs
- AsymmetricSignatureDeformatter.cs
- odbcmetadatafactory.cs
- TransactionTable.cs
- ExtendedPropertyInfo.cs
- IncrementalReadDecoders.cs
- CodeExporter.cs
- DurationConverter.cs
- ViewCellRelation.cs
- ObjectSet.cs
- TripleDESCryptoServiceProvider.cs
- ClassicBorderDecorator.cs
- WindowsSolidBrush.cs
- TableRowGroupCollection.cs
- XmlSerializationReader.cs
- MultiSelector.cs
- CodePageEncoding.cs
- DataColumnMapping.cs
- TdsParserSessionPool.cs
- SqlProvider.cs
- RegexCode.cs
- EventManager.cs
- CorrelationManager.cs
- ErasingStroke.cs
- COSERVERINFO.cs
- ContentPathSegment.cs
- CompoundFileIOPermission.cs