Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / CapabilitiesPattern.cs / 1 / CapabilitiesPattern.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Collections; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; using System.Text; using System.Text.RegularExpressions; using System.Threading; using System.Web.Caching; using System.Web.Compilation; using System.Web.Hosting; using System.Security.Permissions; // // Represents a single pattern to be expanded // internal class CapabilitiesPattern { internal String[] _strings; internal int[] _rules; internal const int Literal = 0; // literal string internal const int Reference = 1; // regex reference ${name} or $number internal const int Variable = 2; // regex reference %{name} internal static readonly Regex refPat = new Regex("\\G\\$(?:(?\\d+)|\\{(? \\w+)\\})"); internal static readonly Regex varPat = new Regex("\\G\\%\\{(? \\w+)\\}"); internal static readonly Regex textPat = new Regex("\\G[^$%\\\\]*(?:\\.[^$%\\\\]*)*"); internal static readonly Regex errorPat = new Regex(".{0,8}"); internal static readonly CapabilitiesPattern Default = new CapabilitiesPattern(); internal CapabilitiesPattern() { _strings = new String[1]; _strings[0] = String.Empty; _rules = new int[1]; _rules[0] = Variable; } internal CapabilitiesPattern(String text) { ArrayList strings = new ArrayList(); ArrayList rules = new ArrayList(); int textpos = 0; for (;;) { Match match = null; // 1: scan text if ((match = textPat.Match(text, textpos)).Success && match.Length > 0) { rules.Add(Literal); strings.Add(Regex.Unescape(match.ToString())); textpos = match.Index + match.Length; } if (textpos == text.Length) break; // 2: look for regex references if ((match = refPat.Match(text, textpos)).Success) { rules.Add(Reference); strings.Add(match.Groups["name"].Value); } // 3: look for variables else if ((match = varPat.Match(text, textpos)).Success) { rules.Add(Variable); strings.Add(match.Groups["name"].Value); } // 4: encountered a syntax error ( else { match = errorPat.Match(text, textpos); throw new ArgumentException( SR.GetString(SR.Unrecognized_construct_in_pattern, match.ToString(), text)); } textpos = match.Index + match.Length; } _strings = (String[])strings.ToArray(typeof(String)); _rules = new int[rules.Count]; for (int i = 0; i < rules.Count; i++) _rules[i] = (int)rules[i]; } internal virtual String Expand(CapabilitiesState matchstate) { StringBuilder sb = null; String result = null; for (int i = 0; i < _rules.Length; i++) { if (sb == null && result != null) sb = new StringBuilder(result); switch (_rules[i]) { case Literal: result = _strings[i]; break; case Reference: result = matchstate.ResolveReference(_strings[i]); break; case Variable: result = matchstate.ResolveVariable(_strings[i]); break; } if (sb != null && result != null) sb.Append(result); } if (sb != null) return sb.ToString(); if (result != null) return result; return String.Empty; } #if DBG internal virtual String Dump() { StringBuilder sb = new StringBuilder(); for (int i = 0; i < _rules.Length; i++) { switch (_rules[i]) { case Literal: sb.Append("\"" + _strings[i] + "\""); break; case Reference: sb.Append("${" + _strings[i] + "}"); break; default: sb.Append("??"); break; } if (i < _rules.Length - 1) sb.Append(" "); } return sb.ToString(); } internal virtual String Dump(String indent) { return indent + Dump() + "\n"; } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Collections; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; using System.Text; using System.Text.RegularExpressions; using System.Threading; using System.Web.Caching; using System.Web.Compilation; using System.Web.Hosting; using System.Security.Permissions; // // Represents a single pattern to be expanded // internal class CapabilitiesPattern { internal String[] _strings; internal int[] _rules; internal const int Literal = 0; // literal string internal const int Reference = 1; // regex reference ${name} or $number internal const int Variable = 2; // regex reference %{name} internal static readonly Regex refPat = new Regex("\\G\\$(?:(?\\d+)|\\{(? \\w+)\\})"); internal static readonly Regex varPat = new Regex("\\G\\%\\{(? \\w+)\\}"); internal static readonly Regex textPat = new Regex("\\G[^$%\\\\]*(?:\\.[^$%\\\\]*)*"); internal static readonly Regex errorPat = new Regex(".{0,8}"); internal static readonly CapabilitiesPattern Default = new CapabilitiesPattern(); internal CapabilitiesPattern() { _strings = new String[1]; _strings[0] = String.Empty; _rules = new int[1]; _rules[0] = Variable; } internal CapabilitiesPattern(String text) { ArrayList strings = new ArrayList(); ArrayList rules = new ArrayList(); int textpos = 0; for (;;) { Match match = null; // 1: scan text if ((match = textPat.Match(text, textpos)).Success && match.Length > 0) { rules.Add(Literal); strings.Add(Regex.Unescape(match.ToString())); textpos = match.Index + match.Length; } if (textpos == text.Length) break; // 2: look for regex references if ((match = refPat.Match(text, textpos)).Success) { rules.Add(Reference); strings.Add(match.Groups["name"].Value); } // 3: look for variables else if ((match = varPat.Match(text, textpos)).Success) { rules.Add(Variable); strings.Add(match.Groups["name"].Value); } // 4: encountered a syntax error ( else { match = errorPat.Match(text, textpos); throw new ArgumentException( SR.GetString(SR.Unrecognized_construct_in_pattern, match.ToString(), text)); } textpos = match.Index + match.Length; } _strings = (String[])strings.ToArray(typeof(String)); _rules = new int[rules.Count]; for (int i = 0; i < rules.Count; i++) _rules[i] = (int)rules[i]; } internal virtual String Expand(CapabilitiesState matchstate) { StringBuilder sb = null; String result = null; for (int i = 0; i < _rules.Length; i++) { if (sb == null && result != null) sb = new StringBuilder(result); switch (_rules[i]) { case Literal: result = _strings[i]; break; case Reference: result = matchstate.ResolveReference(_strings[i]); break; case Variable: result = matchstate.ResolveVariable(_strings[i]); break; } if (sb != null && result != null) sb.Append(result); } if (sb != null) return sb.ToString(); if (result != null) return result; return String.Empty; } #if DBG internal virtual String Dump() { StringBuilder sb = new StringBuilder(); for (int i = 0; i < _rules.Length; i++) { switch (_rules[i]) { case Literal: sb.Append("\"" + _strings[i] + "\""); break; case Reference: sb.Append("${" + _strings[i] + "}"); break; default: sb.Append("??"); break; } if (i < _rules.Length - 1) sb.Append(" "); } return sb.ToString(); } internal virtual String Dump(String indent) { return indent + Dump() + "\n"; } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
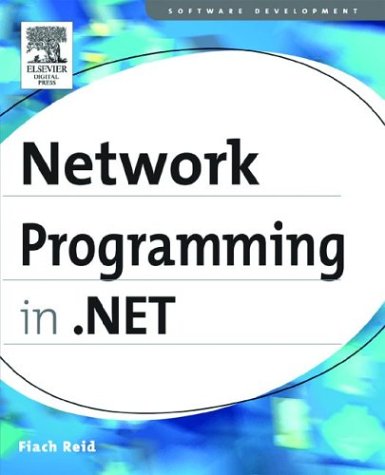
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InstanceDescriptor.cs
- CreateUserWizardStep.cs
- ConditionalBranch.cs
- TextAnchor.cs
- StylusTip.cs
- SizeLimitedCache.cs
- SystemIPInterfaceProperties.cs
- WebHostScriptMappingsInstallComponent.cs
- ScrollData.cs
- PropertyInformationCollection.cs
- XmlAtomicValue.cs
- MetaTableHelper.cs
- SafeRightsManagementHandle.cs
- ConstraintManager.cs
- DesignerCategoryAttribute.cs
- PropertyDescriptorGridEntry.cs
- FormClosedEvent.cs
- WorkflowLayouts.cs
- FontInfo.cs
- WindowsFormsSectionHandler.cs
- HuffCodec.cs
- SignedXmlDebugLog.cs
- DataGridRow.cs
- Error.cs
- ByteArrayHelperWithString.cs
- SimpleWebHandlerParser.cs
- Screen.cs
- QuaternionAnimationUsingKeyFrames.cs
- VectorCollection.cs
- RadioButtonRenderer.cs
- XPathSelectionIterator.cs
- PageCache.cs
- TableAutomationPeer.cs
- EdgeProfileValidation.cs
- MarkupExtensionParser.cs
- Stroke.cs
- OleDbTransaction.cs
- EntityContainerRelationshipSetEnd.cs
- FileDialog.cs
- DictionaryCustomTypeDescriptor.cs
- ElementAtQueryOperator.cs
- CustomErrorsSection.cs
- AnimatedTypeHelpers.cs
- TextDecoration.cs
- SizeConverter.cs
- CustomSignedXml.cs
- EdmError.cs
- RenameRuleObjectDialog.cs
- ProxyWebPartManager.cs
- WindowsGraphics2.cs
- WebPartDescriptionCollection.cs
- CompositeControl.cs
- ApplicationContext.cs
- Positioning.cs
- XmlSchemaInclude.cs
- DataListItemCollection.cs
- StreamFormatter.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- ConfigurationValues.cs
- TextEffectCollection.cs
- DefaultObjectMappingItemCollection.cs
- AxHost.cs
- VirtualizedCellInfoCollection.cs
- CodePageEncoding.cs
- SolidBrush.cs
- ReflectPropertyDescriptor.cs
- FontStretch.cs
- FixedTextSelectionProcessor.cs
- DataSourceListEditor.cs
- RadioButtonAutomationPeer.cs
- CryptoStream.cs
- TextEditorMouse.cs
- XhtmlBasicValidationSummaryAdapter.cs
- _IPv6Address.cs
- DrawingGroupDrawingContext.cs
- DataServiceClientException.cs
- DbProviderFactoriesConfigurationHandler.cs
- TemplatedControlDesigner.cs
- ConfigurationProperty.cs
- GACMembershipCondition.cs
- TrackingStringDictionary.cs
- CodeCommentStatement.cs
- BitmapEffectCollection.cs
- EventToken.cs
- ToolStripSplitButton.cs
- NameGenerator.cs
- WebResourceUtil.cs
- ServiceEndpointElement.cs
- GuidConverter.cs
- CompilerWrapper.cs
- recordstatefactory.cs
- EntitySetDataBindingList.cs
- CustomValidator.cs
- TriState.cs
- XPathSelfQuery.cs
- OracleBinary.cs
- RowToFieldTransformer.cs
- X509SubjectKeyIdentifierClause.cs
- SchemaName.cs
- AssemblyBuilder.cs