Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / TextSegment.cs / 1 / TextSegment.cs
//---------------------------------------------------------------------------- // // File: TextSegment.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A pair of TextPositions used to denote a run of TextContainer content. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Collections; ////// A pair of TextPositions used to denote a run of TextContainer content. /// // internal struct TextSegment { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor. /// /// /// Position preceeding the TextSegment's content. /// /// /// Position following the TextSegment's content. /// ////// If startPosition or endPosition are TextNavigators (derived from /// TextPointer), the TextSegment constructor will store new TextPointer /// instances internally. The values returned by the Start and End /// properties are always immutable TextPositions. /// internal TextSegment(ITextPointer startPosition, ITextPointer endPosition) : this(startPosition, endPosition, false) { } ////// Constructor. /// /// /// Position preceeding the TextSegment's content. /// /// /// Position following the TextSegment's content. /// /// /// Whether preserves LogicalDirection of start and end positions. /// internal TextSegment(ITextPointer startPosition, ITextPointer endPosition, bool preserveLogicalDirection) { ValidationHelper.VerifyPositionPair(startPosition, endPosition); if (startPosition.CompareTo(endPosition) == 0) { // To preserve segment emptiness // we use the same instance of a pointer // for both segment ends. _start = startPosition.GetFrozenPointer(startPosition.LogicalDirection); _end = _start; } else { Invariant.Assert(startPosition.CompareTo(endPosition) < 0); _start = startPosition.GetFrozenPointer(preserveLogicalDirection ? startPosition.LogicalDirection : LogicalDirection.Backward); _end = endPosition.GetFrozenPointer(preserveLogicalDirection ? endPosition.LogicalDirection : LogicalDirection.Forward); } } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// returns true if the segment contains a given position /// // internal bool Contains(ITextPointer position) { return (!this.IsNull && this._start.CompareTo(position) <= 0 && position.CompareTo(this._end) <= 0); } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Position preceeding the TextSegment's content. /// internal ITextPointer Start { get { return _start; } } ////// Position following the TextSegment's content. /// internal ITextPointer End { get { return _end; } } internal bool IsNull { get { return _start == null || _end == null; } } #endregion Internal Properties ////// The "TextSegment.Null" value. /// ////// TextSegtemt.Null is used in contexts where text segment is missing. /// internal static readonly TextSegment Null = new TextSegment(); //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // Position preceeding the TextSegment's content. private readonly ITextPointer _start; // Position following the TextSegment's content. private readonly ITextPointer _end; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextSegment.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A pair of TextPositions used to denote a run of TextContainer content. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Collections; ////// A pair of TextPositions used to denote a run of TextContainer content. /// // internal struct TextSegment { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor. /// /// /// Position preceeding the TextSegment's content. /// /// /// Position following the TextSegment's content. /// ////// If startPosition or endPosition are TextNavigators (derived from /// TextPointer), the TextSegment constructor will store new TextPointer /// instances internally. The values returned by the Start and End /// properties are always immutable TextPositions. /// internal TextSegment(ITextPointer startPosition, ITextPointer endPosition) : this(startPosition, endPosition, false) { } ////// Constructor. /// /// /// Position preceeding the TextSegment's content. /// /// /// Position following the TextSegment's content. /// /// /// Whether preserves LogicalDirection of start and end positions. /// internal TextSegment(ITextPointer startPosition, ITextPointer endPosition, bool preserveLogicalDirection) { ValidationHelper.VerifyPositionPair(startPosition, endPosition); if (startPosition.CompareTo(endPosition) == 0) { // To preserve segment emptiness // we use the same instance of a pointer // for both segment ends. _start = startPosition.GetFrozenPointer(startPosition.LogicalDirection); _end = _start; } else { Invariant.Assert(startPosition.CompareTo(endPosition) < 0); _start = startPosition.GetFrozenPointer(preserveLogicalDirection ? startPosition.LogicalDirection : LogicalDirection.Backward); _end = endPosition.GetFrozenPointer(preserveLogicalDirection ? endPosition.LogicalDirection : LogicalDirection.Forward); } } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// returns true if the segment contains a given position /// // internal bool Contains(ITextPointer position) { return (!this.IsNull && this._start.CompareTo(position) <= 0 && position.CompareTo(this._end) <= 0); } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Position preceeding the TextSegment's content. /// internal ITextPointer Start { get { return _start; } } ////// Position following the TextSegment's content. /// internal ITextPointer End { get { return _end; } } internal bool IsNull { get { return _start == null || _end == null; } } #endregion Internal Properties ////// The "TextSegment.Null" value. /// ////// TextSegtemt.Null is used in contexts where text segment is missing. /// internal static readonly TextSegment Null = new TextSegment(); //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // Position preceeding the TextSegment's content. private readonly ITextPointer _start; // Position following the TextSegment's content. private readonly ITextPointer _end; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
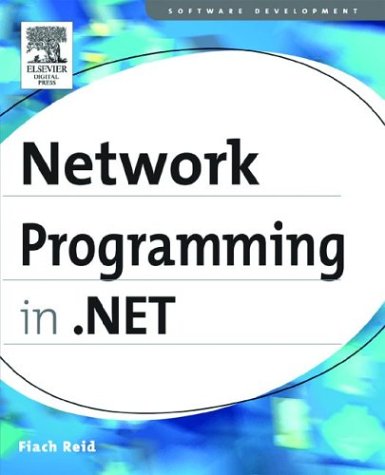
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AdapterDictionary.cs
- BooleanProjectedSlot.cs
- MimeMapping.cs
- XmlCharCheckingReader.cs
- GetPageCompletedEventArgs.cs
- ProviderConnectionPointCollection.cs
- FixedDocumentSequencePaginator.cs
- DuplicateWaitObjectException.cs
- Point4D.cs
- TableDetailsRow.cs
- DrawItemEvent.cs
- FrameSecurityDescriptor.cs
- RegexCaptureCollection.cs
- ScaleTransform.cs
- EmptyQuery.cs
- DataTableReaderListener.cs
- ServerProtocol.cs
- CharacterBufferReference.cs
- BrowserTree.cs
- CursorConverter.cs
- PeerResolver.cs
- Trigger.cs
- DecoderFallbackWithFailureFlag.cs
- TableRow.cs
- AutomationProperties.cs
- RowUpdatedEventArgs.cs
- SelectiveScrollingGrid.cs
- ReachPageContentCollectionSerializer.cs
- DomainUpDown.cs
- Model3D.cs
- XmlSchemaImporter.cs
- EndpointNameMessageFilter.cs
- SubMenuStyle.cs
- SingleAnimationBase.cs
- DrawingCollection.cs
- BindValidationContext.cs
- TypeUtil.cs
- ThreadStaticAttribute.cs
- WeakEventTable.cs
- ComponentResourceKeyConverter.cs
- PageContentAsyncResult.cs
- CheckedListBox.cs
- HostDesigntimeLicenseContext.cs
- BulletDecorator.cs
- ScrollViewer.cs
- Canvas.cs
- CompositeControl.cs
- _ChunkParse.cs
- ItemCollectionEditor.cs
- WizardStepBase.cs
- FixedPageStructure.cs
- CmsUtils.cs
- VariableQuery.cs
- BaseCodePageEncoding.cs
- DoubleLinkList.cs
- ContentOperations.cs
- UsernameTokenFactoryCredential.cs
- MarkerProperties.cs
- WindowsListViewScroll.cs
- WhitespaceRule.cs
- CategoryGridEntry.cs
- StylusButtonEventArgs.cs
- CapiSymmetricAlgorithm.cs
- HostingEnvironmentException.cs
- FontNameEditor.cs
- SqlInfoMessageEvent.cs
- WrappedIUnknown.cs
- PictureBox.cs
- CardSpacePolicyElement.cs
- Grammar.cs
- InvalidCommandTreeException.cs
- WorkflowQueue.cs
- QueryOperatorEnumerator.cs
- DataGridCellClipboardEventArgs.cs
- SQLBinaryStorage.cs
- RectIndependentAnimationStorage.cs
- SqlExpander.cs
- XmlWriterTraceListener.cs
- DesignerVerb.cs
- Part.cs
- IriParsingElement.cs
- BaseCodePageEncoding.cs
- MulticastDelegate.cs
- FileCodeGroup.cs
- ProfileProvider.cs
- NamedPipeChannelFactory.cs
- BrushConverter.cs
- SafeHandle.cs
- TimeManager.cs
- CodeDelegateInvokeExpression.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- DbParameterCollectionHelper.cs
- ServiceMemoryGates.cs
- EtwTrace.cs
- KoreanCalendar.cs
- ErrorBehavior.cs
- AudienceUriMode.cs
- HtmlElementErrorEventArgs.cs
- DictionaryCustomTypeDescriptor.cs
- Calendar.cs