Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Automation / AutomationProperties.cs / 1 / AutomationProperties.cs
using System; namespace System.Windows.Automation { /// public static class AutomationProperties { #region AutomationId ////// AutomationId Property /// public static readonly DependencyProperty AutomationIdProperty = DependencyProperty.RegisterAttached( "AutomationId", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AutomationId property on a DependencyObject. /// public static void SetAutomationId(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AutomationIdProperty, value); } ////// Helper for reading AutomationId property from a DependencyObject. /// public static string GetAutomationId(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AutomationIdProperty)); } #endregion AutomationId #region Name ////// Name Property /// public static readonly DependencyProperty NameProperty = DependencyProperty.RegisterAttached( "Name", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting Name property on a DependencyObject. /// public static void SetName(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(NameProperty, value); } ////// Helper for reading Name property from a DependencyObject. /// public static string GetName(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(NameProperty)); } #endregion Name #region HelpText ////// HelpText Property /// public static readonly DependencyProperty HelpTextProperty = DependencyProperty.RegisterAttached( "HelpText", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting HelpText property on a DependencyObject. /// public static void SetHelpText(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HelpTextProperty, value); } ////// Helper for reading HelpText property from a DependencyObject. /// public static string GetHelpText(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(HelpTextProperty)); } #endregion HelpText #region AcceleratorKey ////// AcceleratorKey Property /// public static readonly DependencyProperty AcceleratorKeyProperty = DependencyProperty.RegisterAttached( "AcceleratorKey", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AcceleratorKey property on a DependencyObject. /// public static void SetAcceleratorKey(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AcceleratorKeyProperty, value); } ////// Helper for reading AcceleratorKey property from a DependencyObject. /// public static string GetAcceleratorKey(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AcceleratorKeyProperty)); } #endregion AcceleratorKey #region AccessKey ////// AccessKey Property /// public static readonly DependencyProperty AccessKeyProperty = DependencyProperty.RegisterAttached( "AccessKey", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AccessKey property on a DependencyObject. /// public static void SetAccessKey(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AccessKeyProperty, value); } ////// Helper for reading AccessKey property from a DependencyObject. /// public static string GetAccessKey(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AccessKeyProperty)); } #endregion AccessKey #region ItemStatus ////// ItemStatus Property /// public static readonly DependencyProperty ItemStatusProperty = DependencyProperty.RegisterAttached( "ItemStatus", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting ItemStatus property on a DependencyObject. /// public static void SetItemStatus(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ItemStatusProperty, value); } ////// Helper for reading ItemStatus property from a DependencyObject. /// public static string GetItemStatus(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(ItemStatusProperty)); } #endregion ItemStatus #region ItemType ////// ItemType Property /// public static readonly DependencyProperty ItemTypeProperty = DependencyProperty.RegisterAttached( "ItemType", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting ItemType property on a DependencyObject. /// public static void SetItemType(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ItemTypeProperty, value); } ////// Helper for reading ItemType property from a DependencyObject. /// public static string GetItemType(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(ItemTypeProperty)); } #endregion ItemType #region IsColumnHeader ////// IsColumnHeader Property /// public static readonly DependencyProperty IsColumnHeaderProperty = DependencyProperty.RegisterAttached( "IsColumnHeader", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsColumnHeader property on a DependencyObject. /// public static void SetIsColumnHeader(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsColumnHeaderProperty, value); } ////// Helper for reading IsColumnHeader property from a DependencyObject. /// public static bool GetIsColumnHeader(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsColumnHeaderProperty)); } #endregion IsColumnHeader #region IsRowHeader ////// IsRowHeader Property /// public static readonly DependencyProperty IsRowHeaderProperty = DependencyProperty.RegisterAttached( "IsRowHeader", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsRowHeader property on a DependencyObject. /// public static void SetIsRowHeader(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsRowHeaderProperty, value); } ////// Helper for reading IsRowHeader property from a DependencyObject. /// public static bool GetIsRowHeader(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsRowHeaderProperty)); } #endregion IsRowHeader #region IsRequiredForForm ////// IsRequiredForForm Property /// public static readonly DependencyProperty IsRequiredForFormProperty = DependencyProperty.RegisterAttached( "IsRequiredForForm", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsRequiredForForm property on a DependencyObject. /// public static void SetIsRequiredForForm(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsRequiredForFormProperty, value); } ////// Helper for reading IsRequiredForForm property from a DependencyObject. /// public static bool GetIsRequiredForForm(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsRequiredForFormProperty)); } #endregion IsRequiredForForm #region LabeledBy ////// LabeledBy Property /// public static readonly DependencyProperty LabeledByProperty = DependencyProperty.RegisterAttached( "LabeledBy", typeof(UIElement), typeof(AutomationProperties), new UIPropertyMetadata((UIElement)null)); ////// Helper for setting LabeledBy property on a DependencyObject. /// public static void SetLabeledBy(DependencyObject element, UIElement value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LabeledByProperty, value); } ////// Helper for reading LabeledBy property from a DependencyObject. /// public static UIElement GetLabeledBy(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((UIElement)element.GetValue(LabeledByProperty)); } #endregion LabeledBy #region private implementation // Validation callback for string properties private static bool IsNotNull(object value) { return (value != null); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Windows.Automation { /// public static class AutomationProperties { #region AutomationId ////// AutomationId Property /// public static readonly DependencyProperty AutomationIdProperty = DependencyProperty.RegisterAttached( "AutomationId", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AutomationId property on a DependencyObject. /// public static void SetAutomationId(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AutomationIdProperty, value); } ////// Helper for reading AutomationId property from a DependencyObject. /// public static string GetAutomationId(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AutomationIdProperty)); } #endregion AutomationId #region Name ////// Name Property /// public static readonly DependencyProperty NameProperty = DependencyProperty.RegisterAttached( "Name", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting Name property on a DependencyObject. /// public static void SetName(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(NameProperty, value); } ////// Helper for reading Name property from a DependencyObject. /// public static string GetName(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(NameProperty)); } #endregion Name #region HelpText ////// HelpText Property /// public static readonly DependencyProperty HelpTextProperty = DependencyProperty.RegisterAttached( "HelpText", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting HelpText property on a DependencyObject. /// public static void SetHelpText(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HelpTextProperty, value); } ////// Helper for reading HelpText property from a DependencyObject. /// public static string GetHelpText(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(HelpTextProperty)); } #endregion HelpText #region AcceleratorKey ////// AcceleratorKey Property /// public static readonly DependencyProperty AcceleratorKeyProperty = DependencyProperty.RegisterAttached( "AcceleratorKey", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AcceleratorKey property on a DependencyObject. /// public static void SetAcceleratorKey(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AcceleratorKeyProperty, value); } ////// Helper for reading AcceleratorKey property from a DependencyObject. /// public static string GetAcceleratorKey(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AcceleratorKeyProperty)); } #endregion AcceleratorKey #region AccessKey ////// AccessKey Property /// public static readonly DependencyProperty AccessKeyProperty = DependencyProperty.RegisterAttached( "AccessKey", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting AccessKey property on a DependencyObject. /// public static void SetAccessKey(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(AccessKeyProperty, value); } ////// Helper for reading AccessKey property from a DependencyObject. /// public static string GetAccessKey(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(AccessKeyProperty)); } #endregion AccessKey #region ItemStatus ////// ItemStatus Property /// public static readonly DependencyProperty ItemStatusProperty = DependencyProperty.RegisterAttached( "ItemStatus", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting ItemStatus property on a DependencyObject. /// public static void SetItemStatus(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ItemStatusProperty, value); } ////// Helper for reading ItemStatus property from a DependencyObject. /// public static string GetItemStatus(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(ItemStatusProperty)); } #endregion ItemStatus #region ItemType ////// ItemType Property /// public static readonly DependencyProperty ItemTypeProperty = DependencyProperty.RegisterAttached( "ItemType", typeof(string), typeof(AutomationProperties), new UIPropertyMetadata(string.Empty), new ValidateValueCallback(IsNotNull)); ////// Helper for setting ItemType property on a DependencyObject. /// public static void SetItemType(DependencyObject element, string value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ItemTypeProperty, value); } ////// Helper for reading ItemType property from a DependencyObject. /// public static string GetItemType(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((string)element.GetValue(ItemTypeProperty)); } #endregion ItemType #region IsColumnHeader ////// IsColumnHeader Property /// public static readonly DependencyProperty IsColumnHeaderProperty = DependencyProperty.RegisterAttached( "IsColumnHeader", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsColumnHeader property on a DependencyObject. /// public static void SetIsColumnHeader(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsColumnHeaderProperty, value); } ////// Helper for reading IsColumnHeader property from a DependencyObject. /// public static bool GetIsColumnHeader(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsColumnHeaderProperty)); } #endregion IsColumnHeader #region IsRowHeader ////// IsRowHeader Property /// public static readonly DependencyProperty IsRowHeaderProperty = DependencyProperty.RegisterAttached( "IsRowHeader", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsRowHeader property on a DependencyObject. /// public static void SetIsRowHeader(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsRowHeaderProperty, value); } ////// Helper for reading IsRowHeader property from a DependencyObject. /// public static bool GetIsRowHeader(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsRowHeaderProperty)); } #endregion IsRowHeader #region IsRequiredForForm ////// IsRequiredForForm Property /// public static readonly DependencyProperty IsRequiredForFormProperty = DependencyProperty.RegisterAttached( "IsRequiredForForm", typeof(bool), typeof(AutomationProperties), new UIPropertyMetadata(MS.Internal.KnownBoxes.BooleanBoxes.FalseBox)); ////// Helper for setting IsRequiredForForm property on a DependencyObject. /// public static void SetIsRequiredForForm(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsRequiredForFormProperty, value); } ////// Helper for reading IsRequiredForForm property from a DependencyObject. /// public static bool GetIsRequiredForForm(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((bool)element.GetValue(IsRequiredForFormProperty)); } #endregion IsRequiredForForm #region LabeledBy ////// LabeledBy Property /// public static readonly DependencyProperty LabeledByProperty = DependencyProperty.RegisterAttached( "LabeledBy", typeof(UIElement), typeof(AutomationProperties), new UIPropertyMetadata((UIElement)null)); ////// Helper for setting LabeledBy property on a DependencyObject. /// public static void SetLabeledBy(DependencyObject element, UIElement value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LabeledByProperty, value); } ////// Helper for reading LabeledBy property from a DependencyObject. /// public static UIElement GetLabeledBy(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((UIElement)element.GetValue(LabeledByProperty)); } #endregion LabeledBy #region private implementation // Validation callback for string properties private static bool IsNotNull(object value) { return (value != null); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
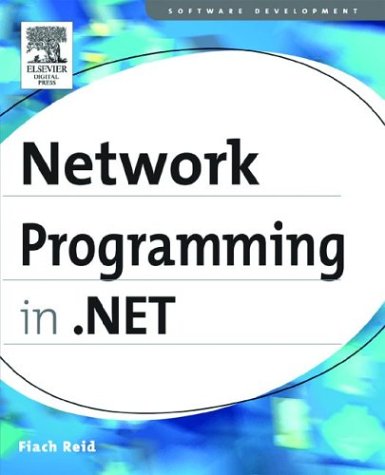
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttributeCollection.cs
- columnmapfactory.cs
- PrintDialogException.cs
- GeneralTransformCollection.cs
- ToolStripItemBehavior.cs
- shaperfactoryquerycacheentry.cs
- SqlException.cs
- BehaviorService.cs
- Win32Exception.cs
- PersonalizationProviderHelper.cs
- CheckableControlBaseAdapter.cs
- TextRangeAdaptor.cs
- QilPatternFactory.cs
- ExeConfigurationFileMap.cs
- DataSourceConverter.cs
- TemplateBamlTreeBuilder.cs
- MenuItemBinding.cs
- XmlUrlResolver.cs
- TargetControlTypeCache.cs
- XPathException.cs
- Ipv6Element.cs
- WinFormsSecurity.cs
- DiagnosticsConfigurationHandler.cs
- filewebresponse.cs
- Regex.cs
- MouseEventArgs.cs
- SplashScreenNativeMethods.cs
- TemplateXamlParser.cs
- OdbcDataReader.cs
- Codec.cs
- DbResourceAllocator.cs
- PKCS1MaskGenerationMethod.cs
- CheckBoxFlatAdapter.cs
- ProcessProtocolHandler.cs
- DataRow.cs
- Margins.cs
- PropertyDescriptorComparer.cs
- HebrewCalendar.cs
- Accessible.cs
- BitmapPalette.cs
- RegexRunner.cs
- CheckBoxBaseAdapter.cs
- TextBoxAutomationPeer.cs
- XmlReader.cs
- ExpressionBuilderCollection.cs
- EventsTab.cs
- SQLBoolean.cs
- AnnotationHelper.cs
- recordstatefactory.cs
- Emitter.cs
- DataSourceView.cs
- OleDbPermission.cs
- AdapterDictionary.cs
- SqlNodeTypeOperators.cs
- SHA256Managed.cs
- DBCommand.cs
- WebPartMenuStyle.cs
- HierarchicalDataSourceConverter.cs
- ValueTable.cs
- CapiSafeHandles.cs
- DependencyObjectProvider.cs
- DataReaderContainer.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- _TLSstream.cs
- HtmlTextViewAdapter.cs
- AutoGeneratedField.cs
- ZipIOExtraFieldPaddingElement.cs
- BitmapSource.cs
- SiteMapPath.cs
- CharUnicodeInfo.cs
- LayoutTableCell.cs
- StoreAnnotationsMap.cs
- SortedList.cs
- SuppressMergeCheckAttribute.cs
- HttpEncoderUtility.cs
- DbConvert.cs
- Gdiplus.cs
- TextContainerHelper.cs
- MasterPageBuildProvider.cs
- CookieProtection.cs
- XmlReturnWriter.cs
- RegexTree.cs
- MenuItem.cs
- BindingGroup.cs
- HighlightVisual.cs
- ServiceProviders.cs
- ActivationServices.cs
- FormViewUpdatedEventArgs.cs
- FunctionUpdateCommand.cs
- COAUTHINFO.cs
- WindowsEditBoxRange.cs
- IntegrationExceptionEventArgs.cs
- FaultException.cs
- ColorDialog.cs
- XmlTextAttribute.cs
- SQLCharsStorage.cs
- ParseHttpDate.cs
- EncoderBestFitFallback.cs
- SourceLocationProvider.cs
- SimpleHandlerFactory.cs