Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusSystemGestureEventArgs.cs / 1 / StylusSystemGestureEventArgs.cs
using System; using System.Collections; using System.ComponentModel; using System.Windows.Media; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// The StylusSystemGestureEventArgs class provides access to the logical /// Stylus device for all derived event args. /// public class StylusSystemGestureEventArgs : StylusEventArgs { ///////////////////////////////////////////////////////////////////// ////// Initializes a new instance of the StylusSystemGestureEventArgs class. /// /// /// The logical Stylus device associated with this event. /// /// /// The time when the input occured. /// /// /// The type of system gesture. /// public StylusSystemGestureEventArgs( StylusDevice stylusDevice, int timestamp, SystemGesture systemGesture) : base(stylusDevice, timestamp) { if (systemGesture != SystemGesture.Tap && systemGesture != SystemGesture.RightTap && systemGesture != SystemGesture.Drag && systemGesture != SystemGesture.RightDrag && systemGesture != SystemGesture.HoldEnter && systemGesture != SystemGesture.HoldLeave && systemGesture != SystemGesture.HoverEnter && systemGesture != SystemGesture.HoverLeave && systemGesture != SystemGesture.None) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "systemGesture")); } _id = systemGesture; } ///////////////////////////////////////////////////////////////////// ////// Initializes a new instance of the StylusSystemGestureEventArgs class. /// /// /// The logical Stylus device associated with this event. /// /// /// The time when the input occured. /// /// /// The type of system gesture. /// /// /// The X location reported with this system gesture. In tablet /// device coordinates. /// /// /// The Y location reported with this system gesture. In tablet /// device coordinates. /// /// /// The button state at the time of the system gesture. /// Note: A flick gesture will pass the flick data in the parameter. /// internal StylusSystemGestureEventArgs( StylusDevice stylusDevice, int timestamp, SystemGesture systemGesture, int gestureX, int gestureY, int buttonState) : base(stylusDevice, timestamp) { if (systemGesture != SystemGesture.Tap && systemGesture != SystemGesture.RightTap && systemGesture != SystemGesture.Drag && systemGesture != SystemGesture.RightDrag && systemGesture != SystemGesture.HoldEnter && systemGesture != SystemGesture.HoldLeave && systemGesture != SystemGesture.HoverEnter && systemGesture != SystemGesture.HoverLeave && systemGesture != SystemGesture.Flick && systemGesture != SystemGesture.None) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "systemGesture")); } _id = systemGesture; _buttonState = buttonState; _gestureX = gestureX; _gestureY = gestureY; } ///////////////////////////////////////////////////////////////////// ////// Field to retrieve which gesture occurred. /// public SystemGesture SystemGesture { get { return _id; } } ///////////////////////////////////////////////////////////////////// ////// Field to retrieve the button state reported with this /// system gesture. /// /// NOTE: For a Flick gesture this param contains the flick /// and not the button state. /// internal int ButtonState { get { return _buttonState; } } ///////////////////////////////////////////////////////////////////// ////// Field to retrieve the X location of the system gesture. /// This is in tablet device coordinates. /// internal int GestureX { get { return _gestureX; } } ///////////////////////////////////////////////////////////////////// ////// Field to retrieve the Y location of the system gesture. /// This is in tablet device coordinates. /// internal int GestureY { get { return _gestureY; } } ///////////////////////////////////////////////////////////////////// ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { StylusSystemGestureEventHandler handler = (StylusSystemGestureEventHandler) genericHandler; handler(genericTarget, this); } ///////////////////////////////////////////////////////////////////// SystemGesture _id; int _buttonState; int _gestureX; int _gestureY; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.ComponentModel; using System.Windows.Media; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// The StylusSystemGestureEventArgs class provides access to the logical /// Stylus device for all derived event args. /// public class StylusSystemGestureEventArgs : StylusEventArgs { ///////////////////////////////////////////////////////////////////// ////// Initializes a new instance of the StylusSystemGestureEventArgs class. /// /// /// The logical Stylus device associated with this event. /// /// /// The time when the input occured. /// /// /// The type of system gesture. /// public StylusSystemGestureEventArgs( StylusDevice stylusDevice, int timestamp, SystemGesture systemGesture) : base(stylusDevice, timestamp) { if (systemGesture != SystemGesture.Tap && systemGesture != SystemGesture.RightTap && systemGesture != SystemGesture.Drag && systemGesture != SystemGesture.RightDrag && systemGesture != SystemGesture.HoldEnter && systemGesture != SystemGesture.HoldLeave && systemGesture != SystemGesture.HoverEnter && systemGesture != SystemGesture.HoverLeave && systemGesture != SystemGesture.None) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "systemGesture")); } _id = systemGesture; } ///////////////////////////////////////////////////////////////////// ////// Initializes a new instance of the StylusSystemGestureEventArgs class. /// /// /// The logical Stylus device associated with this event. /// /// /// The time when the input occured. /// /// /// The type of system gesture. /// /// /// The X location reported with this system gesture. In tablet /// device coordinates. /// /// /// The Y location reported with this system gesture. In tablet /// device coordinates. /// /// /// The button state at the time of the system gesture. /// Note: A flick gesture will pass the flick data in the parameter. /// internal StylusSystemGestureEventArgs( StylusDevice stylusDevice, int timestamp, SystemGesture systemGesture, int gestureX, int gestureY, int buttonState) : base(stylusDevice, timestamp) { if (systemGesture != SystemGesture.Tap && systemGesture != SystemGesture.RightTap && systemGesture != SystemGesture.Drag && systemGesture != SystemGesture.RightDrag && systemGesture != SystemGesture.HoldEnter && systemGesture != SystemGesture.HoldLeave && systemGesture != SystemGesture.HoverEnter && systemGesture != SystemGesture.HoverLeave && systemGesture != SystemGesture.Flick && systemGesture != SystemGesture.None) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "systemGesture")); } _id = systemGesture; _buttonState = buttonState; _gestureX = gestureX; _gestureY = gestureY; } ///////////////////////////////////////////////////////////////////// ////// Field to retrieve which gesture occurred. /// public SystemGesture SystemGesture { get { return _id; } } ///////////////////////////////////////////////////////////////////// ////// Field to retrieve the button state reported with this /// system gesture. /// /// NOTE: For a Flick gesture this param contains the flick /// and not the button state. /// internal int ButtonState { get { return _buttonState; } } ///////////////////////////////////////////////////////////////////// ////// Field to retrieve the X location of the system gesture. /// This is in tablet device coordinates. /// internal int GestureX { get { return _gestureX; } } ///////////////////////////////////////////////////////////////////// ////// Field to retrieve the Y location of the system gesture. /// This is in tablet device coordinates. /// internal int GestureY { get { return _gestureY; } } ///////////////////////////////////////////////////////////////////// ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { StylusSystemGestureEventHandler handler = (StylusSystemGestureEventHandler) genericHandler; handler(genericTarget, this); } ///////////////////////////////////////////////////////////////////// SystemGesture _id; int _buttonState; int _gestureX; int _gestureY; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
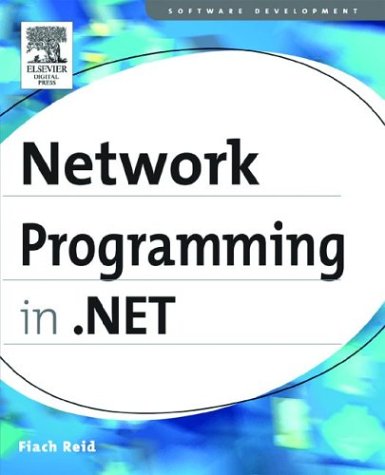
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParallelLoopState.cs
- NativeMethods.cs
- StorageEntitySetMapping.cs
- ModuleElement.cs
- XmlILStorageConverter.cs
- SafeArrayRankMismatchException.cs
- SessionPageStateSection.cs
- IxmlLineInfo.cs
- TransformerInfoCollection.cs
- SequentialOutput.cs
- BamlStream.cs
- SubordinateTransaction.cs
- TypeSystemHelpers.cs
- Figure.cs
- RuleSettingsCollection.cs
- AutoResetEvent.cs
- Empty.cs
- XhtmlConformanceSection.cs
- TcpClientSocketManager.cs
- SqlTypesSchemaImporter.cs
- DataStreamFromComStream.cs
- BooleanSwitch.cs
- GestureRecognitionResult.cs
- ImageAttributes.cs
- DataStreams.cs
- PropertyItemInternal.cs
- BitmapEffectDrawingContextWalker.cs
- LoginCancelEventArgs.cs
- HttpServerVarsCollection.cs
- BaseDataBoundControl.cs
- AnnotationHighlightLayer.cs
- LiteralControl.cs
- TreeViewCancelEvent.cs
- WsdlWriter.cs
- SystemIPv4InterfaceProperties.cs
- Aggregates.cs
- XmlUtil.cs
- FileDialog_Vista_Interop.cs
- QuinticEase.cs
- ComplexBindingPropertiesAttribute.cs
- wmiprovider.cs
- DataBoundLiteralControl.cs
- ScriptingAuthenticationServiceSection.cs
- HtmlInputHidden.cs
- RegisteredArrayDeclaration.cs
- OrderByBuilder.cs
- OracleCommandBuilder.cs
- RegexRunner.cs
- Message.cs
- FilteredAttributeCollection.cs
- EmptyWorkItem.cs
- DefaultEvaluationContext.cs
- WebServiceResponseDesigner.cs
- SlipBehavior.cs
- FormsAuthenticationUserCollection.cs
- InkCollectionBehavior.cs
- ConsoleKeyInfo.cs
- SBCSCodePageEncoding.cs
- FormCollection.cs
- FunctionDescription.cs
- CorrelationValidator.cs
- OdbcPermission.cs
- _AutoWebProxyScriptHelper.cs
- AnnotationAdorner.cs
- InputScope.cs
- DatagridviewDisplayedBandsData.cs
- CodeGenerator.cs
- IgnoreFileBuildProvider.cs
- FontWeightConverter.cs
- GeneralTransformGroup.cs
- ManagementQuery.cs
- PingReply.cs
- EntitySetBaseCollection.cs
- AQNBuilder.cs
- PeerNameRegistration.cs
- ActionFrame.cs
- WebPartsPersonalizationAuthorization.cs
- ComPlusDiagnosticTraceRecords.cs
- StorageEndPropertyMapping.cs
- HMACSHA384.cs
- ping.cs
- rsa.cs
- GenericArgumentsUpdater.cs
- DataGridViewRowsAddedEventArgs.cs
- MD5.cs
- Matrix3DValueSerializer.cs
- DoWorkEventArgs.cs
- UIntPtr.cs
- IgnorePropertiesAttribute.cs
- RuleConditionDialog.cs
- WriteLineDesigner.xaml.cs
- MulticastNotSupportedException.cs
- FixedTextView.cs
- CodeParameterDeclarationExpressionCollection.cs
- FamilyMap.cs
- EditingCommands.cs
- ConfigXmlCDataSection.cs
- UnsafeNativeMethodsPenimc.cs
- MsmqInputSessionChannelListener.cs
- IncrementalHitTester.cs