Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / Vertex.cs / 1 / Vertex.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Data.Common.Utils.Boolean { using System.Diagnostics; using System.Globalization; ////// A node in a Reduced Ordered Boolean Decision Diagram. Reads as: /// /// if 'Variable' then 'Then' else 'Else' /// /// Invariant: the Then and Else children must refer to 'deeper' variables, /// or variables with a higher value. Otherwise, the graph is not 'Ordered'. /// All creation of vertices is mediated by the Solver class which ensures /// each vertex is unique. Otherwise, the graph is not 'Reduced'. /// sealed class Vertex : IEquatable{ /// /// Initializes a sink BDD node (zero or one) /// private Vertex() { this.Variable = int.MaxValue; this.Children = new Vertex[] { }; } internal Vertex(int variable, Vertex[] children) { EntityUtil.BoolExprAssert(variable < int.MaxValue, "exceeded number of supported variables"); AssertConstructorArgumentsValid(variable, children); this.Variable = variable; this.Children = children; } [Conditional("DEBUG")] private static void AssertConstructorArgumentsValid(int variable, Vertex[] children) { Debug.Assert(null != children, "internal vertices must define children"); Debug.Assert(2 <= children.Length, "internal vertices must have at least two children"); Debug.Assert(0 < variable, "internal vertices must have 0 < variable"); foreach (Vertex child in children) { Debug.Assert(variable < child.Variable, "children must have greater variable"); } } ////// Sink node representing the Boolean function '1' (true) /// internal static readonly Vertex One = new Vertex(); ////// Sink node representing the Boolean function '0' (false) /// internal static readonly Vertex Zero = new Vertex(); ////// Gets the variable tested by this vertex. If this is a sink node, returns /// int.MaxValue since there is no variable to test (and since this is a leaf, /// this non-existent variable is 'deeper' than any existing variable; the /// variable value is larger than any real variable) /// internal readonly int Variable; ////// Note: do not modify elements. /// Gets the result when Variable evaluates to true. If this is a sink node, /// returns null. /// internal readonly Vertex[] Children; ////// Returns true if this is '1'. /// internal bool IsOne() { return object.ReferenceEquals(Vertex.One, this); } ////// Returns true if this is '0'. /// internal bool IsZero() { return object.ReferenceEquals(Vertex.Zero, this); } ////// Returns true if this is '0' or '1'. /// internal bool IsSink() { return Variable == int.MaxValue; } public bool Equals(Vertex other) { return object.ReferenceEquals(this, other); } public override bool Equals(object obj) { Debug.Fail("used typed Equals"); return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } public override string ToString() { if (IsOne()) { return "_1_"; } if (IsZero()) { return "_0_"; } return String.Format(CultureInfo.InvariantCulture, "<{0}, {1}>", Variable, StringUtil.ToCommaSeparatedString(Children)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Data.Common.Utils.Boolean { using System.Diagnostics; using System.Globalization; ////// A node in a Reduced Ordered Boolean Decision Diagram. Reads as: /// /// if 'Variable' then 'Then' else 'Else' /// /// Invariant: the Then and Else children must refer to 'deeper' variables, /// or variables with a higher value. Otherwise, the graph is not 'Ordered'. /// All creation of vertices is mediated by the Solver class which ensures /// each vertex is unique. Otherwise, the graph is not 'Reduced'. /// sealed class Vertex : IEquatable{ /// /// Initializes a sink BDD node (zero or one) /// private Vertex() { this.Variable = int.MaxValue; this.Children = new Vertex[] { }; } internal Vertex(int variable, Vertex[] children) { EntityUtil.BoolExprAssert(variable < int.MaxValue, "exceeded number of supported variables"); AssertConstructorArgumentsValid(variable, children); this.Variable = variable; this.Children = children; } [Conditional("DEBUG")] private static void AssertConstructorArgumentsValid(int variable, Vertex[] children) { Debug.Assert(null != children, "internal vertices must define children"); Debug.Assert(2 <= children.Length, "internal vertices must have at least two children"); Debug.Assert(0 < variable, "internal vertices must have 0 < variable"); foreach (Vertex child in children) { Debug.Assert(variable < child.Variable, "children must have greater variable"); } } ////// Sink node representing the Boolean function '1' (true) /// internal static readonly Vertex One = new Vertex(); ////// Sink node representing the Boolean function '0' (false) /// internal static readonly Vertex Zero = new Vertex(); ////// Gets the variable tested by this vertex. If this is a sink node, returns /// int.MaxValue since there is no variable to test (and since this is a leaf, /// this non-existent variable is 'deeper' than any existing variable; the /// variable value is larger than any real variable) /// internal readonly int Variable; ////// Note: do not modify elements. /// Gets the result when Variable evaluates to true. If this is a sink node, /// returns null. /// internal readonly Vertex[] Children; ////// Returns true if this is '1'. /// internal bool IsOne() { return object.ReferenceEquals(Vertex.One, this); } ////// Returns true if this is '0'. /// internal bool IsZero() { return object.ReferenceEquals(Vertex.Zero, this); } ////// Returns true if this is '0' or '1'. /// internal bool IsSink() { return Variable == int.MaxValue; } public bool Equals(Vertex other) { return object.ReferenceEquals(this, other); } public override bool Equals(object obj) { Debug.Fail("used typed Equals"); return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } public override string ToString() { if (IsOne()) { return "_1_"; } if (IsZero()) { return "_0_"; } return String.Format(CultureInfo.InvariantCulture, "<{0}, {1}>", Variable, StringUtil.ToCommaSeparatedString(Children)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
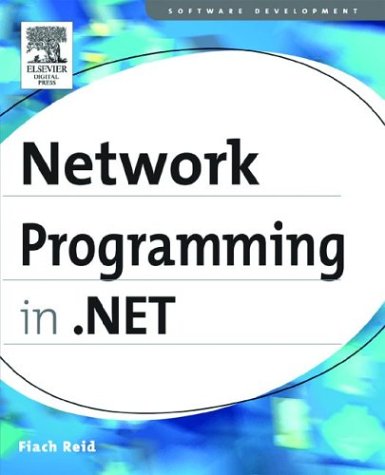
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartConnectionsEventArgs.cs
- SendMailErrorEventArgs.cs
- ObjectDataProvider.cs
- LambdaCompiler.Unary.cs
- FormViewDeletedEventArgs.cs
- SBCSCodePageEncoding.cs
- PasswordDeriveBytes.cs
- COM2TypeInfoProcessor.cs
- PerfService.cs
- SecurityHeaderTokenResolver.cs
- PartManifestEntry.cs
- XPathSelfQuery.cs
- OletxEnlistment.cs
- FillRuleValidation.cs
- TextEditorTables.cs
- ObjectManager.cs
- VectorAnimation.cs
- _Events.cs
- QueryCoreOp.cs
- ToolStripItemRenderEventArgs.cs
- BinaryWriter.cs
- SimpleTextLine.cs
- MatrixAnimationBase.cs
- DesignerHost.cs
- CodeGeneratorAttribute.cs
- ClassHandlersStore.cs
- TextElementCollectionHelper.cs
- DataService.cs
- RedistVersionInfo.cs
- BroadcastEventHelper.cs
- Vector.cs
- TypeConverterHelper.cs
- ShapeTypeface.cs
- InvokeMemberBinder.cs
- WmlLabelAdapter.cs
- MetadataImporterQuotas.cs
- cryptoapiTransform.cs
- KnowledgeBase.cs
- ListBindingHelper.cs
- ComponentChangedEvent.cs
- ContentElement.cs
- IOThreadTimer.cs
- BuildProviderAppliesToAttribute.cs
- DBCommandBuilder.cs
- SystemSounds.cs
- AutomationProperty.cs
- CustomErrorsSectionWrapper.cs
- TemplatePagerField.cs
- InkCanvasSelection.cs
- CompareInfo.cs
- ControlBindingsConverter.cs
- TimeEnumHelper.cs
- ByteBufferPool.cs
- Lasso.cs
- CellLabel.cs
- ClientSideQueueItem.cs
- SubpageParaClient.cs
- PackageDigitalSignature.cs
- InternalReceiveMessage.cs
- Page.cs
- NonDualMessageSecurityOverHttpElement.cs
- QuotedPrintableStream.cs
- MatrixUtil.cs
- DebugView.cs
- TemplateBindingExtensionConverter.cs
- MembershipValidatePasswordEventArgs.cs
- TreeNodeStyle.cs
- JsonFormatWriterGenerator.cs
- FunctionUpdateCommand.cs
- HwndSourceParameters.cs
- TreeNodeBinding.cs
- SecurityContextTokenCache.cs
- ChainOfResponsibility.cs
- CodeGenerator.cs
- ComponentSerializationService.cs
- ChannelEndpointElementCollection.cs
- ParentUndoUnit.cs
- ReferencedCollectionType.cs
- PolyBezierSegment.cs
- HttpMethodAttribute.cs
- RefreshInfo.cs
- BlurEffect.cs
- FrameworkElementAutomationPeer.cs
- PlatformCulture.cs
- DesignerAttribute.cs
- LineSegment.cs
- PlanCompiler.cs
- Vector3DValueSerializer.cs
- TableLayoutStyleCollection.cs
- BitmapImage.cs
- DynamicRenderer.cs
- PhonemeConverter.cs
- QuotedPrintableStream.cs
- BoundColumn.cs
- CompatibleComparer.cs
- DataGridColumnsPage.cs
- MessageDirection.cs
- ProtocolViolationException.cs
- NameObjectCollectionBase.cs
- control.ime.cs