Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SapiInterop / sapiproxy.cs / 1 / sapiproxy.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // All the calls to SAPI interfaces are wraped into the class 'SapiRecognizer', // 'SapiContext' and 'SapiGrammar'. // // The SAPI call are executed in the context of a proxy that is either a // pass-through or forward the request to an MTA thread for SAPI 5.1 // // History: // 4/1/2006 jeanfp //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Threading; using System.Runtime.InteropServices; namespace System.Speech.Internal.SapiInterop { internal abstract class SapiProxy : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors public virtual void Dispose () { GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods internal abstract object Invoke (ObjectDelegate pfn); internal abstract void Invoke2 (VoidDelegate pfn); #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal ISpRecognizer Recognizer { get { return _recognizer; } } internal ISpRecognizer2 Recognizer2 { get { if (_recognizer2 == null) { _recognizer2 = (ISpRecognizer2) _recognizer; } return _recognizer2; } } internal ISpeechRecognizer SapiSpeechRecognizer { get { if (_speechRecognizer == null) { _speechRecognizer = (ISpeechRecognizer) _recognizer; } return _speechRecognizer; } } #endregion //******************************************************************* // // Protected Fields // //******************************************************************** #region Protected Fields protected ISpeechRecognizer _speechRecognizer; protected ISpRecognizer2 _recognizer2; protected ISpRecognizer _recognizer; #endregion //******************************************************************* // // Internal Types // //******************************************************************* #region Protected Fields internal class PassThrough : SapiProxy, IDisposable { //******************************************************************* // // Constructors // //******************************************************************** #region Constructors internal PassThrough (ISpRecognizer recognizer) { _recognizer = recognizer; } ~PassThrough () { Dispose (false); } public override void Dispose () { try { Dispose (true); } finally { base.Dispose (); } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods override internal object Invoke (ObjectDelegate pfn) { return pfn.Invoke (); } override internal void Invoke2 (VoidDelegate pfn) { pfn.Invoke (); } #endregion //******************************************************************** // // Private Methods // //******************************************************************* #region Private Methods private void Dispose (bool disposing) { _recognizer2 = null; _speechRecognizer = null; Marshal.ReleaseComObject (_recognizer); } #endregion } #if !SPEECHSERVER #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56500 // Remove all the catch all statements warnings used by the interop layer internal class MTAThread : SapiProxy, IDisposable { //******************************************************************** // // Constructors // //******************************************************************* #region Constructors internal MTAThread (SapiRecognizer.RecognizerType type) { _mta = new Thread (new ThreadStart (SapiMTAThread)); if (!_mta.TrySetApartmentState (ApartmentState.MTA)) { throw new InvalidOperationException (); } _mta.IsBackground = true; _mta.Start (); if (type == SapiRecognizer.RecognizerType.InProc) { Invoke2 (delegate { _recognizer = (ISpRecognizer) new SpInprocRecognizer (); }); } else { Invoke2 (delegate { _recognizer = (ISpRecognizer) new SpSharedRecognizer (); }); } } ~MTAThread () { Dispose (false); } public override void Dispose () { try { Dispose (true); } finally { base.Dispose (); } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods override internal object Invoke (ObjectDelegate pfn) { lock (this) { _doit = pfn; _process.Set (); _done.WaitOne (); if (_exception == null) { return _result; } else { throw _exception; } } } override internal void Invoke2 (VoidDelegate pfn) { lock (this) { _doit2 = pfn; _process.Set (); _done.WaitOne (); if (_exception != null) { throw _exception; } } } #endregion //******************************************************************** // // Private Methods // //******************************************************************* #region Private Methods private void Dispose (bool disposing) { lock (this) { _recognizer2 = null; _speechRecognizer = null; Invoke2 (delegate { Marshal.ReleaseComObject (_recognizer); }); ((IDisposable) _process).Dispose (); ((IDisposable) _done).Dispose (); _mta.Abort (); } base.Dispose (); } private void SapiMTAThread () { while (true) { try { _process.WaitOne (); _exception = null; if (_doit != null) { _result = _doit.Invoke (); _doit = null; } else { _doit2.Invoke (); _doit2 = null; } } catch (Exception e) { _exception = e; } _done.Set (); } } #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private Thread _mta; private AutoResetEvent _process = new AutoResetEvent (false); private AutoResetEvent _done = new AutoResetEvent (false); private ObjectDelegate _doit; private VoidDelegate _doit2; private object _result; private Exception _exception; #endregion } #endif internal delegate object ObjectDelegate (); internal delegate void VoidDelegate (); } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // All the calls to SAPI interfaces are wraped into the class 'SapiRecognizer', // 'SapiContext' and 'SapiGrammar'. // // The SAPI call are executed in the context of a proxy that is either a // pass-through or forward the request to an MTA thread for SAPI 5.1 // // History: // 4/1/2006 jeanfp //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Threading; using System.Runtime.InteropServices; namespace System.Speech.Internal.SapiInterop { internal abstract class SapiProxy : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors public virtual void Dispose () { GC.SuppressFinalize (this); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods internal abstract object Invoke (ObjectDelegate pfn); internal abstract void Invoke2 (VoidDelegate pfn); #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal ISpRecognizer Recognizer { get { return _recognizer; } } internal ISpRecognizer2 Recognizer2 { get { if (_recognizer2 == null) { _recognizer2 = (ISpRecognizer2) _recognizer; } return _recognizer2; } } internal ISpeechRecognizer SapiSpeechRecognizer { get { if (_speechRecognizer == null) { _speechRecognizer = (ISpeechRecognizer) _recognizer; } return _speechRecognizer; } } #endregion //******************************************************************* // // Protected Fields // //******************************************************************** #region Protected Fields protected ISpeechRecognizer _speechRecognizer; protected ISpRecognizer2 _recognizer2; protected ISpRecognizer _recognizer; #endregion //******************************************************************* // // Internal Types // //******************************************************************* #region Protected Fields internal class PassThrough : SapiProxy, IDisposable { //******************************************************************* // // Constructors // //******************************************************************** #region Constructors internal PassThrough (ISpRecognizer recognizer) { _recognizer = recognizer; } ~PassThrough () { Dispose (false); } public override void Dispose () { try { Dispose (true); } finally { base.Dispose (); } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods override internal object Invoke (ObjectDelegate pfn) { return pfn.Invoke (); } override internal void Invoke2 (VoidDelegate pfn) { pfn.Invoke (); } #endregion //******************************************************************** // // Private Methods // //******************************************************************* #region Private Methods private void Dispose (bool disposing) { _recognizer2 = null; _speechRecognizer = null; Marshal.ReleaseComObject (_recognizer); } #endregion } #if !SPEECHSERVER #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56500 // Remove all the catch all statements warnings used by the interop layer internal class MTAThread : SapiProxy, IDisposable { //******************************************************************** // // Constructors // //******************************************************************* #region Constructors internal MTAThread (SapiRecognizer.RecognizerType type) { _mta = new Thread (new ThreadStart (SapiMTAThread)); if (!_mta.TrySetApartmentState (ApartmentState.MTA)) { throw new InvalidOperationException (); } _mta.IsBackground = true; _mta.Start (); if (type == SapiRecognizer.RecognizerType.InProc) { Invoke2 (delegate { _recognizer = (ISpRecognizer) new SpInprocRecognizer (); }); } else { Invoke2 (delegate { _recognizer = (ISpRecognizer) new SpSharedRecognizer (); }); } } ~MTAThread () { Dispose (false); } public override void Dispose () { try { Dispose (true); } finally { base.Dispose (); } } #endregion //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods override internal object Invoke (ObjectDelegate pfn) { lock (this) { _doit = pfn; _process.Set (); _done.WaitOne (); if (_exception == null) { return _result; } else { throw _exception; } } } override internal void Invoke2 (VoidDelegate pfn) { lock (this) { _doit2 = pfn; _process.Set (); _done.WaitOne (); if (_exception != null) { throw _exception; } } } #endregion //******************************************************************** // // Private Methods // //******************************************************************* #region Private Methods private void Dispose (bool disposing) { lock (this) { _recognizer2 = null; _speechRecognizer = null; Invoke2 (delegate { Marshal.ReleaseComObject (_recognizer); }); ((IDisposable) _process).Dispose (); ((IDisposable) _done).Dispose (); _mta.Abort (); } base.Dispose (); } private void SapiMTAThread () { while (true) { try { _process.WaitOne (); _exception = null; if (_doit != null) { _result = _doit.Invoke (); _doit = null; } else { _doit2.Invoke (); _doit2 = null; } } catch (Exception e) { _exception = e; } _done.Set (); } } #endregion //******************************************************************** // // Private Fields // //******************************************************************** #region Private Fields private Thread _mta; private AutoResetEvent _process = new AutoResetEvent (false); private AutoResetEvent _done = new AutoResetEvent (false); private ObjectDelegate _doit; private VoidDelegate _doit2; private object _result; private Exception _exception; #endregion } #endif internal delegate object ObjectDelegate (); internal delegate void VoidDelegate (); } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
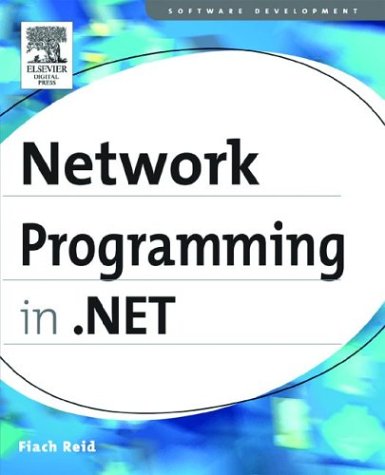
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DisplayMemberTemplateSelector.cs
- ConditionBrowserDialog.cs
- Int32Converter.cs
- Attributes.cs
- CatalogPartChrome.cs
- WorkflowMarkupSerializer.cs
- SspiHelper.cs
- WebPermission.cs
- CultureTable.cs
- loginstatus.cs
- PatternMatcher.cs
- _SecureChannel.cs
- TimeoutValidationAttribute.cs
- Model3D.cs
- IdentityNotMappedException.cs
- QueryContinueDragEvent.cs
- TdsParser.cs
- DecimalAnimationBase.cs
- GroupAggregateExpr.cs
- ToolStripTextBox.cs
- EntitySet.cs
- LambdaCompiler.cs
- precedingsibling.cs
- FormattedText.cs
- COM2ColorConverter.cs
- ItemChangedEventArgs.cs
- HostingEnvironmentException.cs
- AddInStore.cs
- TdsParserHelperClasses.cs
- CheckBoxList.cs
- DataKeyCollection.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- _FtpControlStream.cs
- DynamicMetaObjectBinder.cs
- DataSourceHelper.cs
- DynamicResourceExtension.cs
- nulltextcontainer.cs
- QilCloneVisitor.cs
- MimeTypeMapper.cs
- GeometryGroup.cs
- XmlArrayItemAttributes.cs
- TextPatternIdentifiers.cs
- CapabilitiesUse.cs
- SoapSchemaExporter.cs
- XPathAncestorQuery.cs
- LineVisual.cs
- WebPartConnectionsConnectVerb.cs
- CapabilitiesPattern.cs
- MatrixAnimationUsingPath.cs
- AtomParser.cs
- ManifestResourceInfo.cs
- SynchronizingStream.cs
- BaseInfoTable.cs
- GridViewSortEventArgs.cs
- ToolStripPanelSelectionBehavior.cs
- RoutedUICommand.cs
- DirectoryNotFoundException.cs
- TypeDescriptorFilterService.cs
- GroupBoxRenderer.cs
- ObjectCacheHost.cs
- AxWrapperGen.cs
- MessageDecoder.cs
- MsmqIntegrationChannelFactory.cs
- FileDialogPermission.cs
- HtmlInputControl.cs
- TextBoxBase.cs
- CalculatedColumn.cs
- PointAnimationUsingPath.cs
- ValueUnavailableException.cs
- CategoryAttribute.cs
- Touch.cs
- StylusPoint.cs
- BindToObject.cs
- XmlTypeAttribute.cs
- WebPartManager.cs
- GuidConverter.cs
- Rect.cs
- RuntimeConfigurationRecord.cs
- CatalogZone.cs
- ToolStripControlHost.cs
- AutomationAttributeInfo.cs
- OutputCacheProfileCollection.cs
- CryptoProvider.cs
- ADConnectionHelper.cs
- COM2Properties.cs
- RuntimeResourceSet.cs
- LogArchiveSnapshot.cs
- SafeWaitHandle.cs
- PointAnimationClockResource.cs
- RelationalExpressions.cs
- DivideByZeroException.cs
- SettingsPropertyCollection.cs
- SerialErrors.cs
- ZeroOpNode.cs
- BinaryParser.cs
- RemoteX509AsymmetricSecurityKey.cs
- BitmapEffectDrawingContextWalker.cs
- GroupItemAutomationPeer.cs
- MatchSingleFxEngineOpcode.cs
- DBCSCodePageEncoding.cs