Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / SystemIcons.cs / 1 / SystemIcons.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Diagnostics; using System; ////// /// Icon objects for Windows system-wide icons. /// public sealed class SystemIcons { private static Icon _application ; private static Icon _asterisk ; private static Icon _error ; private static Icon _exclamation ; private static Icon _hand ; private static Icon _information ; private static Icon _question ; private static Icon _warning ; private static Icon _winlogo ; private static Icon _shield ; // not creatable... // private SystemIcons() { } ////// /// Icon is the default Application icon. (WIN32: IDI_APPLICATION) /// public static Icon Application { get { if (_application == null) _application = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_APPLICATION )); return _application; } } ////// /// Icon is the system Asterisk icon. (WIN32: IDI_ASTERISK) /// public static Icon Asterisk { get { if (_asterisk== null) _asterisk = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_ASTERISK )); return _asterisk; } } ////// /// Icon is the system Error icon. (WIN32: IDI_ERROR) /// public static Icon Error { get { if (_error == null) _error = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_ERROR )); return _error; } } ////// /// Icon is the system Exclamation icon. (WIN32: IDI_EXCLAMATION) /// public static Icon Exclamation { get { if (_exclamation == null) _exclamation = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_EXCLAMATION )); return _exclamation; } } ////// /// Icon is the system Hand icon. (WIN32: IDI_HAND) /// public static Icon Hand { get { if (_hand == null) _hand = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_HAND )); return _hand; } } ////// /// Icon is the system Information icon. (WIN32: IDI_INFORMATION) /// public static Icon Information { get { if (_information == null) _information = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_INFORMATION )); return _information; } } ////// /// Icon is the system Question icon. (WIN32: IDI_QUESTION) /// public static Icon Question { get { if (_question== null) _question = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_QUESTION )); return _question; } } ////// /// Icon is the system Warning icon. (WIN32: IDI_WARNING) /// public static Icon Warning { get { if (_warning == null) _warning = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_WARNING )); return _warning; } } ////// /// Icon is the Windows Logo icon. (WIN32: IDI_WINLOGO) /// public static Icon WinLogo { get { if (_winlogo == null) _winlogo = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_WINLOGO )); return _winlogo; } } ////// /// Icon is the Windows Shield Icon. /// public static Icon Shield { get { if (_shield == null) { _shield = new Icon(typeof(SystemIcons), "ShieldIcon.ico"); } return _shield; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Diagnostics; using System; ////// /// Icon objects for Windows system-wide icons. /// public sealed class SystemIcons { private static Icon _application ; private static Icon _asterisk ; private static Icon _error ; private static Icon _exclamation ; private static Icon _hand ; private static Icon _information ; private static Icon _question ; private static Icon _warning ; private static Icon _winlogo ; private static Icon _shield ; // not creatable... // private SystemIcons() { } ////// /// Icon is the default Application icon. (WIN32: IDI_APPLICATION) /// public static Icon Application { get { if (_application == null) _application = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_APPLICATION )); return _application; } } ////// /// Icon is the system Asterisk icon. (WIN32: IDI_ASTERISK) /// public static Icon Asterisk { get { if (_asterisk== null) _asterisk = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_ASTERISK )); return _asterisk; } } ////// /// Icon is the system Error icon. (WIN32: IDI_ERROR) /// public static Icon Error { get { if (_error == null) _error = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_ERROR )); return _error; } } ////// /// Icon is the system Exclamation icon. (WIN32: IDI_EXCLAMATION) /// public static Icon Exclamation { get { if (_exclamation == null) _exclamation = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_EXCLAMATION )); return _exclamation; } } ////// /// Icon is the system Hand icon. (WIN32: IDI_HAND) /// public static Icon Hand { get { if (_hand == null) _hand = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_HAND )); return _hand; } } ////// /// Icon is the system Information icon. (WIN32: IDI_INFORMATION) /// public static Icon Information { get { if (_information == null) _information = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_INFORMATION )); return _information; } } ////// /// Icon is the system Question icon. (WIN32: IDI_QUESTION) /// public static Icon Question { get { if (_question== null) _question = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_QUESTION )); return _question; } } ////// /// Icon is the system Warning icon. (WIN32: IDI_WARNING) /// public static Icon Warning { get { if (_warning == null) _warning = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_WARNING )); return _warning; } } ////// /// Icon is the Windows Logo icon. (WIN32: IDI_WINLOGO) /// public static Icon WinLogo { get { if (_winlogo == null) _winlogo = new Icon( SafeNativeMethods.LoadIcon( NativeMethods.NullHandleRef, SafeNativeMethods.IDI_WINLOGO )); return _winlogo; } } ////// /// Icon is the Windows Shield Icon. /// public static Icon Shield { get { if (_shield == null) { _shield = new Icon(typeof(SystemIcons), "ShieldIcon.ico"); } return _shield; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
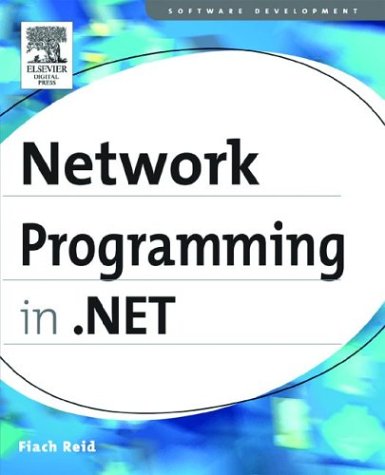
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewSelectedColumnCollection.cs
- HeaderedItemsControl.cs
- RelationshipDetailsCollection.cs
- DragDrop.cs
- FileDialogPermission.cs
- ProcessModuleCollection.cs
- InternalConfigHost.cs
- SecurityHelper.cs
- WindowsGraphicsWrapper.cs
- Monitor.cs
- SspiSafeHandles.cs
- CodeDirectiveCollection.cs
- IIS7WorkerRequest.cs
- isolationinterop.cs
- TagNameToTypeMapper.cs
- StringComparer.cs
- CapabilitiesSection.cs
- SynchronousReceiveElement.cs
- hwndwrapper.cs
- OracleDateTime.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- SizeIndependentAnimationStorage.cs
- LambdaCompiler.Generated.cs
- HttpWriter.cs
- SHA512Cng.cs
- SafeMarshalContext.cs
- DoubleCollectionConverter.cs
- MulticastIPAddressInformationCollection.cs
- PageBuildProvider.cs
- MasterPageCodeDomTreeGenerator.cs
- WebPartCollection.cs
- Select.cs
- ColorIndependentAnimationStorage.cs
- Constraint.cs
- TextBox.cs
- MonitorWrapper.cs
- DotAtomReader.cs
- TableRow.cs
- Label.cs
- WorkflowInstanceExtensionManager.cs
- InvalidDocumentContentsException.cs
- WebPartAuthorizationEventArgs.cs
- TimeoutException.cs
- UnaryNode.cs
- SqlDataSourceQuery.cs
- UpdateCommand.cs
- ReadOnlyCollectionBuilder.cs
- GetChildSubtree.cs
- StringAnimationBase.cs
- CheckBoxFlatAdapter.cs
- Internal.cs
- COM2EnumConverter.cs
- StorageFunctionMapping.cs
- RenamedEventArgs.cs
- UInt32.cs
- IndicCharClassifier.cs
- SingleTagSectionHandler.cs
- HitTestWithGeometryDrawingContextWalker.cs
- PersistenceProviderFactory.cs
- WSHttpSecurityElement.cs
- HttpHandlerActionCollection.cs
- TextSerializer.cs
- CryptographicAttribute.cs
- StringComparer.cs
- HostSecurityManager.cs
- Sql8ConformanceChecker.cs
- XmlDictionaryReaderQuotas.cs
- MimeMapping.cs
- CaseInsensitiveComparer.cs
- DbDataReader.cs
- ApplicationInterop.cs
- mediapermission.cs
- FormsIdentity.cs
- CharacterMetricsDictionary.cs
- COM2Properties.cs
- IdentifierService.cs
- StylusPointDescription.cs
- ChildTable.cs
- NumericPagerField.cs
- NotSupportedException.cs
- UnsafeNativeMethods.cs
- Pkcs7Recipient.cs
- SqlMultiplexer.cs
- ContentControl.cs
- FixedSOMPage.cs
- ModuleBuilderData.cs
- EmptyImpersonationContext.cs
- TreeViewEvent.cs
- counter.cs
- EasingFunctionBase.cs
- SmiConnection.cs
- Int64.cs
- TraceContext.cs
- base64Transforms.cs
- rsa.cs
- HTMLTagNameToTypeMapper.cs
- SqlTransaction.cs
- CqlLexer.cs
- CompilationRelaxations.cs
- LoadedEvent.cs