Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Runtime / Serialization / Formatters / SerTrace.cs / 1 / SerTrace.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SerTrace ** ** ** Purpose: Routine used for Debugging ** ** ===========================================================*/ namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Security.Permissions; using System.Reflection; using System.Diagnostics; #if FEATURE_PAL // To turn on tracing, add the following to the per-machine // rotor.ini file, inside the [Rotor] section: // ManagedLogFacility=0x32 // where: #else // To turn on tracing the set registry // HKEY_CURRENT_USER -> Software -> Microsoft -> .NETFramework // new DWORD value ManagedLogFacility 0x32 where #endif // 0x2 is System.Runtime.Serialization // 0x10 is Binary Formatter // 0x20 is Soap Formatter // // Turn on Logging in the jitmgr // remoting Wsdl logging ///[StrongNameIdentityPermissionAttribute(SecurityAction.LinkDemand, PublicKey = "0x" + AssemblyRef.EcmaPublicKeyFull, Name="System.Runtime.Remoting" )] [System.Runtime.InteropServices.ComVisible(true)] public sealed class InternalRM { /// [System.Diagnostics.Conditional("_LOGGING")] public static void InfoSoap(params Object[]messages) { BCLDebug.Trace("SOAP", messages); } //[System.Diagnostics.Conditional("_LOGGING")] /// public static bool SoapCheckEnabled() { return BCLDebug.CheckEnabled("SOAP"); } } /// [StrongNameIdentityPermissionAttribute(SecurityAction.LinkDemand, PublicKey = "0x" + AssemblyRef.MicrosoftPublicKeyFull, Name="System.Runtime.Serialization.Formatters.Soap" )] [System.Runtime.InteropServices.ComVisible(true)] public sealed class InternalST { private InternalST() { } /// [System.Diagnostics.Conditional("_LOGGING")] public static void InfoSoap(params Object[]messages) { BCLDebug.Trace("SOAP", messages); } //[System.Diagnostics.Conditional("_LOGGING")] /// public static bool SoapCheckEnabled() { return BCLDebug.CheckEnabled("Soap"); } /// [System.Diagnostics.Conditional("SER_LOGGING")] public static void Soap(params Object[]messages) { if (!(messages[0] is String)) messages[0] = (messages[0].GetType()).Name+" "; else messages[0] = messages[0]+" "; BCLDebug.Trace("SOAP",messages); } /// [System.Diagnostics.Conditional("_DEBUG")] public static void SoapAssert(bool condition, String message) { BCLDebug.Assert(condition, message); } /// public static void SerializationSetValue(FieldInfo fi, Object target, Object value) { if ( fi == null) throw new ArgumentNullException("fi"); if (target == null) throw new ArgumentNullException("target"); if (value == null) throw new ArgumentNullException("value"); FormatterServices.SerializationSetValue(fi, target, value); } /// public static Assembly LoadAssemblyFromString(String assemblyString) { return FormatterServices.LoadAssemblyFromString(assemblyString); } } internal static class SerTrace { [Conditional("_LOGGING")] internal static void InfoLog(params Object[]messages) { BCLDebug.Trace("BINARY", messages); } [Conditional("SER_LOGGING")] internal static void Log(params Object[]messages) { if (!(messages[0] is String)) messages[0] = (messages[0].GetType()).Name+" "; else messages[0] = messages[0]+" "; BCLDebug.Trace("BINARY",messages); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SerTrace ** ** ** Purpose: Routine used for Debugging ** ** ===========================================================*/ namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Security.Permissions; using System.Reflection; using System.Diagnostics; #if FEATURE_PAL // To turn on tracing, add the following to the per-machine // rotor.ini file, inside the [Rotor] section: // ManagedLogFacility=0x32 // where: #else // To turn on tracing the set registry // HKEY_CURRENT_USER -> Software -> Microsoft -> .NETFramework // new DWORD value ManagedLogFacility 0x32 where #endif // 0x2 is System.Runtime.Serialization // 0x10 is Binary Formatter // 0x20 is Soap Formatter // // Turn on Logging in the jitmgr // remoting Wsdl logging /// [StrongNameIdentityPermissionAttribute(SecurityAction.LinkDemand, PublicKey = "0x" + AssemblyRef.EcmaPublicKeyFull, Name="System.Runtime.Remoting" )] [System.Runtime.InteropServices.ComVisible(true)] public sealed class InternalRM { /// [System.Diagnostics.Conditional("_LOGGING")] public static void InfoSoap(params Object[]messages) { BCLDebug.Trace("SOAP", messages); } //[System.Diagnostics.Conditional("_LOGGING")] /// public static bool SoapCheckEnabled() { return BCLDebug.CheckEnabled("SOAP"); } } /// [StrongNameIdentityPermissionAttribute(SecurityAction.LinkDemand, PublicKey = "0x" + AssemblyRef.MicrosoftPublicKeyFull, Name="System.Runtime.Serialization.Formatters.Soap" )] [System.Runtime.InteropServices.ComVisible(true)] public sealed class InternalST { private InternalST() { } /// [System.Diagnostics.Conditional("_LOGGING")] public static void InfoSoap(params Object[]messages) { BCLDebug.Trace("SOAP", messages); } //[System.Diagnostics.Conditional("_LOGGING")] /// public static bool SoapCheckEnabled() { return BCLDebug.CheckEnabled("Soap"); } /// [System.Diagnostics.Conditional("SER_LOGGING")] public static void Soap(params Object[]messages) { if (!(messages[0] is String)) messages[0] = (messages[0].GetType()).Name+" "; else messages[0] = messages[0]+" "; BCLDebug.Trace("SOAP",messages); } /// [System.Diagnostics.Conditional("_DEBUG")] public static void SoapAssert(bool condition, String message) { BCLDebug.Assert(condition, message); } /// public static void SerializationSetValue(FieldInfo fi, Object target, Object value) { if ( fi == null) throw new ArgumentNullException("fi"); if (target == null) throw new ArgumentNullException("target"); if (value == null) throw new ArgumentNullException("value"); FormatterServices.SerializationSetValue(fi, target, value); } /// public static Assembly LoadAssemblyFromString(String assemblyString) { return FormatterServices.LoadAssemblyFromString(assemblyString); } } internal static class SerTrace { [Conditional("_LOGGING")] internal static void InfoLog(params Object[]messages) { BCLDebug.Trace("BINARY", messages); } [Conditional("SER_LOGGING")] internal static void Log(params Object[]messages) { if (!(messages[0] is String)) messages[0] = (messages[0].GetType()).Name+" "; else messages[0] = messages[0]+" "; BCLDebug.Trace("BINARY",messages); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
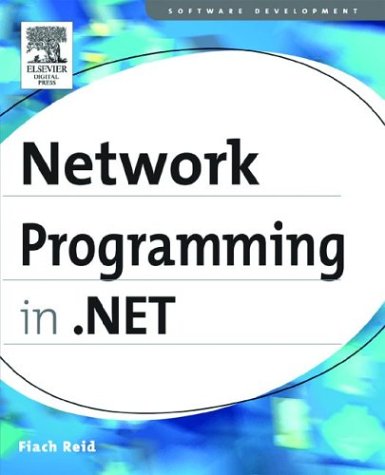
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyTabChangedEvent.cs
- SQLBinaryStorage.cs
- StringSorter.cs
- FlowLayoutPanel.cs
- AuthStoreRoleProvider.cs
- formatstringdialog.cs
- BooleanConverter.cs
- PerformanceCounterManager.cs
- COAUTHINFO.cs
- SkewTransform.cs
- FormatSettings.cs
- TreeNodeStyleCollection.cs
- Literal.cs
- KeyGesture.cs
- DrawingAttributesDefaultValueFactory.cs
- Vector.cs
- NameValueConfigurationCollection.cs
- ListDictionaryInternal.cs
- Aggregates.cs
- recordstatescratchpad.cs
- WebPartTransformer.cs
- HostedTcpTransportManager.cs
- SubMenuStyleCollection.cs
- SafeRightsManagementQueryHandle.cs
- Solver.cs
- StoryFragments.cs
- DBAsyncResult.cs
- MexHttpsBindingCollectionElement.cs
- FileLogRecord.cs
- CodeObject.cs
- ADMembershipProvider.cs
- IpcChannelHelper.cs
- LicFileLicenseProvider.cs
- WebPartZone.cs
- ContextMenuStrip.cs
- _NegoStream.cs
- PerformanceCountersElement.cs
- Transform.cs
- SectionXmlInfo.cs
- WeakReference.cs
- RNGCryptoServiceProvider.cs
- IsolationInterop.cs
- TextTreeNode.cs
- EventLog.cs
- Parser.cs
- PropertyMapper.cs
- CutCopyPasteHelper.cs
- DbParameterCollectionHelper.cs
- GridItemPattern.cs
- BmpBitmapDecoder.cs
- Int32EqualityComparer.cs
- HttpDigestClientElement.cs
- ActivityDesignerResources.cs
- XslCompiledTransform.cs
- HWStack.cs
- FixedSOMContainer.cs
- HostSecurityManager.cs
- counter.cs
- SerialStream.cs
- SHA384.cs
- ValueProviderWrapper.cs
- FileUtil.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- BamlResourceSerializer.cs
- UnsafeNetInfoNativeMethods.cs
- WindowsUpDown.cs
- XmlDocumentSurrogate.cs
- Grant.cs
- AnimationClock.cs
- ThreadExceptionEvent.cs
- DesignerCatalogPartChrome.cs
- ContentOperations.cs
- LassoSelectionBehavior.cs
- CustomErrorsSectionWrapper.cs
- BufferAllocator.cs
- TaskScheduler.cs
- XsltSettings.cs
- AutoGeneratedFieldProperties.cs
- XPathItem.cs
- RegexRunner.cs
- IgnoreSection.cs
- TableRow.cs
- HtmlInputSubmit.cs
- HebrewCalendar.cs
- GridPattern.cs
- HtmlHistory.cs
- UnrecognizedPolicyAssertionElement.cs
- BypassElementCollection.cs
- IResourceProvider.cs
- DirectoryInfo.cs
- SettingsPropertyCollection.cs
- CodeTypeParameter.cs
- FromRequest.cs
- WindowsTreeView.cs
- SettingsProperty.cs
- PolicyLevel.cs
- BuildDependencySet.cs
- NestedContainer.cs
- FileDialogCustomPlace.cs
- WebResourceUtil.cs