Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / TableItemPattern.cs / 1 / TableItemPattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for TableItem Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Used to expose grid items with header information. /// #if (INTERNAL_COMPILE) internal class TableItemPattern: GridItemPattern #else public class TableItemPattern: GridItemPattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableItemPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern, cached) { _hPattern = hPattern; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///TableItem pattern public static readonly new AutomationPattern Pattern = TableItemPatternIdentifiers.Pattern; ///Property ID: RowHeaderItems - Collection of all row headers for this cell public static readonly AutomationProperty RowHeaderItemsProperty = TableItemPatternIdentifiers.RowHeaderItemsProperty; ///Property ID: ColumnHeaderItems - Collection of all column headers for this cell public static readonly AutomationProperty ColumnHeaderItemsProperty = TableItemPatternIdentifiers.ColumnHeaderItemsProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// new public TableItemPatternInformation Cached { get { Misc.ValidateCached(_cached); return new TableItemPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// new public TableItemPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new TableItemPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static new object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new TableItemPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct TableItemPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal TableItemPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// the row number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Row { get { return (int)_el.GetPatternPropertyValue(RowProperty, _useCache); } } ////// /// the column number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Column { get { return (int)_el.GetPatternPropertyValue(ColumnProperty, _useCache); } } ////// /// count of how many rows the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int RowSpan { get { return (int)_el.GetPatternPropertyValue(RowSpanProperty, _useCache); } } ////// /// count of how many columns the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int ColumnSpan { get { return (int)_el.GetPatternPropertyValue(ColumnSpanProperty, _useCache); } } ////// /// The logical element that supports the GripPattern for this Item /// /// ////// This API does not work inside the secure execution environment. /// public AutomationElement ContainingGrid { get { return (AutomationElement)_el.GetPatternPropertyValue(ContainingGridProperty, _useCache); } } ////// Collection of all row headers for this cell /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetRowHeaderItems() { return (AutomationElement[])_el.GetPatternPropertyValue(RowHeaderItemsProperty, _useCache); } ////// Collection of all column headers for this cell /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetColumnHeaderItems() { return (AutomationElement[])_el.GetPatternPropertyValue(ColumnHeaderItemsProperty, _useCache); } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for TableItem Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Used to expose grid items with header information. /// #if (INTERNAL_COMPILE) internal class TableItemPattern: GridItemPattern #else public class TableItemPattern: GridItemPattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableItemPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern, cached) { _hPattern = hPattern; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///TableItem pattern public static readonly new AutomationPattern Pattern = TableItemPatternIdentifiers.Pattern; ///Property ID: RowHeaderItems - Collection of all row headers for this cell public static readonly AutomationProperty RowHeaderItemsProperty = TableItemPatternIdentifiers.RowHeaderItemsProperty; ///Property ID: ColumnHeaderItems - Collection of all column headers for this cell public static readonly AutomationProperty ColumnHeaderItemsProperty = TableItemPatternIdentifiers.ColumnHeaderItemsProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// new public TableItemPatternInformation Cached { get { Misc.ValidateCached(_cached); return new TableItemPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// new public TableItemPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new TableItemPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static new object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new TableItemPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct TableItemPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal TableItemPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// the row number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Row { get { return (int)_el.GetPatternPropertyValue(RowProperty, _useCache); } } ////// /// the column number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Column { get { return (int)_el.GetPatternPropertyValue(ColumnProperty, _useCache); } } ////// /// count of how many rows the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int RowSpan { get { return (int)_el.GetPatternPropertyValue(RowSpanProperty, _useCache); } } ////// /// count of how many columns the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int ColumnSpan { get { return (int)_el.GetPatternPropertyValue(ColumnSpanProperty, _useCache); } } ////// /// The logical element that supports the GripPattern for this Item /// /// ////// This API does not work inside the secure execution environment. /// public AutomationElement ContainingGrid { get { return (AutomationElement)_el.GetPatternPropertyValue(ContainingGridProperty, _useCache); } } ////// Collection of all row headers for this cell /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetRowHeaderItems() { return (AutomationElement[])_el.GetPatternPropertyValue(RowHeaderItemsProperty, _useCache); } ////// Collection of all column headers for this cell /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetColumnHeaderItems() { return (AutomationElement[])_el.GetPatternPropertyValue(ColumnHeaderItemsProperty, _useCache); } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
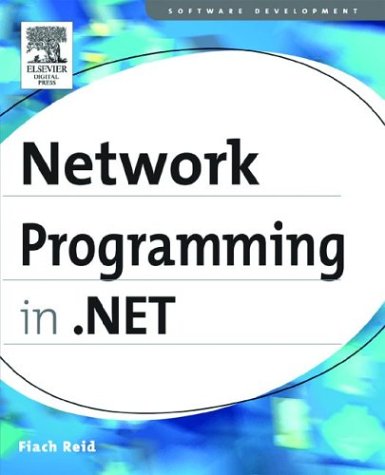
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExecutedRoutedEventArgs.cs
- InputMethod.cs
- XD.cs
- NamedPipeConnectionPool.cs
- DateTimeValueSerializerContext.cs
- MethodAccessException.cs
- TextElementCollectionHelper.cs
- MailFileEditor.cs
- WebPartZoneCollection.cs
- ChannelServices.cs
- IntSumAggregationOperator.cs
- CryptoStream.cs
- InvalidOleVariantTypeException.cs
- ProcessThread.cs
- TypefaceCollection.cs
- StrokeFIndices.cs
- LayoutInformation.cs
- __ConsoleStream.cs
- sortedlist.cs
- HttpRuntime.cs
- SoapHeader.cs
- XmlObjectSerializerWriteContext.cs
- Binding.cs
- DuplicateWaitObjectException.cs
- FutureFactory.cs
- arc.cs
- NamedPipeHostedTransportConfiguration.cs
- PageRouteHandler.cs
- RSAPKCS1KeyExchangeFormatter.cs
- TypeNameHelper.cs
- XhtmlBasicPanelAdapter.cs
- WebScriptMetadataFormatter.cs
- MediaElementAutomationPeer.cs
- SettingsSection.cs
- Model3DGroup.cs
- CallbackValidator.cs
- SynchronizingStream.cs
- DataGridViewSelectedColumnCollection.cs
- LocalizedNameDescriptionPair.cs
- ListBindingConverter.cs
- DiagnosticTrace.cs
- FilteredSchemaElementLookUpTable.cs
- _Rfc2616CacheValidators.cs
- Rfc2898DeriveBytes.cs
- DataGridViewCellValueEventArgs.cs
- LineProperties.cs
- SecurityUniqueId.cs
- ErrorHandler.cs
- ToolStripGrip.cs
- GenericUriParser.cs
- FacetDescription.cs
- RuleConditionDialog.cs
- ConstructorBuilder.cs
- mda.cs
- ListViewUpdatedEventArgs.cs
- WithParamAction.cs
- LowerCaseStringConverter.cs
- TextRunCacheImp.cs
- PreviewPageInfo.cs
- MulticastOption.cs
- SizeConverter.cs
- ParserExtension.cs
- TcpTransportSecurityElement.cs
- InvalidFilterCriteriaException.cs
- DbConnectionPoolOptions.cs
- UpdateExpressionVisitor.cs
- EncoderParameters.cs
- ValidatorUtils.cs
- SystemInformation.cs
- IndentedTextWriter.cs
- AnimatedTypeHelpers.cs
- ReturnEventArgs.cs
- UseLicense.cs
- ProtocolsConfigurationEntry.cs
- ComponentEvent.cs
- BinaryObjectInfo.cs
- MonthChangedEventArgs.cs
- AutoResetEvent.cs
- RowToFieldTransformer.cs
- Formatter.cs
- NativeMethods.cs
- ToolStripSeparator.cs
- XdrBuilder.cs
- GeneralTransform.cs
- OledbConnectionStringbuilder.cs
- GeneratedView.cs
- GradientStop.cs
- ControlDesigner.cs
- ToolZoneDesigner.cs
- WebPartCatalogCloseVerb.cs
- KnowledgeBase.cs
- EntityDataSourceColumn.cs
- SqlUDTStorage.cs
- WeakReferenceList.cs
- WebPartDescriptionCollection.cs
- GraphicsContext.cs
- XmlElementAttributes.cs
- ValueChangedEventManager.cs
- BitmapEffectInputConnector.cs
- EventSourceCreationData.cs