Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripSeparator.cs / 1305376 / ToolStripSeparator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.ComponentModel; using System.Windows.Forms.Design; using System.Diagnostics.CodeAnalysis; using System.Runtime.Versioning; ////// /// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.ToolStrip | ToolStripItemDesignerAvailability.ContextMenuStrip)] public class ToolStripSeparator : ToolStripItem { private const int WINBAR_SEPARATORTHICKNESS = 6; private const int WINBAR_SEPARATORHEIGHT = 23; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] public ToolStripSeparator() { this.ForeColor = SystemColors.ControlDark; } ////// Called when the background of the winbar is being rendered /// ///[ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new bool AutoToolTip { get { return base.AutoToolTip; } set { base.AutoToolTip = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override Image BackgroundImage { get { return base.BackgroundImage; } set { base.BackgroundImage = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override ImageLayout BackgroundImageLayout { get { return base.BackgroundImageLayout; } set { base.BackgroundImageLayout = value; } } /// public override bool CanSelect { get { return DesignMode; } } /// /// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected override Size DefaultSize { get { return new Size(WINBAR_SEPARATORTHICKNESS, WINBAR_SEPARATORTHICKNESS); } } ///protected internal override Padding DefaultMargin { get { return Padding.Empty; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new bool DoubleClickEnabled { get { return base.DoubleClickEnabled; } set { base.DoubleClickEnabled = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override bool Enabled { get { return base.Enabled; } set { base.Enabled = value; } } /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler EnabledChanged { add { base.EnabledChanged += value; } remove { base.EnabledChanged -= value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new ToolStripItemDisplayStyle DisplayStyle { get { return base.DisplayStyle; } set { base.DisplayStyle = value; } } /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler DisplayStyleChanged { add { base.DisplayStyleChanged += value; } remove { base.DisplayStyleChanged -= value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override Font Font { get { return base.Font; } set { base.Font = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new ContentAlignment ImageAlign { get { return base.ImageAlign; } set { base.ImageAlign = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override Image Image { [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] get { return base.Image; } set { base.Image = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), RefreshProperties(RefreshProperties.Repaint), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new int ImageIndex { get { return base.ImageIndex; } set { base.ImageIndex = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new string ImageKey { get { return base.ImageKey; } set { base.ImageKey = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Color ImageTransparentColor { get { return base.ImageTransparentColor; } set { base.ImageTransparentColor = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new ToolStripItemImageScaling ImageScaling { get { return base.ImageScaling; } set { base.ImageScaling = value; } } private bool IsVertical { get { ToolStrip parent = this.ParentInternal; if (parent == null) { parent = Owner; } ToolStripDropDownMenu dropDownMenu = parent as ToolStripDropDownMenu; if (dropDownMenu != null) { return false; } switch (parent.LayoutStyle) { case ToolStripLayoutStyle.VerticalStackWithOverflow: return false; case ToolStripLayoutStyle.HorizontalStackWithOverflow: case ToolStripLayoutStyle.Flow: case ToolStripLayoutStyle.Table: default: return true; } } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override string Text { get { return base.Text; } set { base.Text = value; } } /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler TextChanged { add { base.TextChanged += value; } remove { base.TextChanged -= value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new ContentAlignment TextAlign { get { return base.TextAlign; } set { base.TextAlign = value; } } [Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DefaultValue(ToolStripTextDirection.Horizontal)] public override ToolStripTextDirection TextDirection { get { return base.TextDirection; } set { base.TextDirection = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new TextImageRelation TextImageRelation { get { return base.TextImageRelation; } set { base.TextImageRelation = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new string ToolTipText { get { return base.ToolTipText; } set { base.ToolTipText = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new bool RightToLeftAutoMirrorImage { get { return base.RightToLeftAutoMirrorImage; } set { base.RightToLeftAutoMirrorImage = value; } } [EditorBrowsable(EditorBrowsableState.Advanced)] protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripSeparatorAccessibleObject(this); } /// public override Size GetPreferredSize(Size constrainingSize) { ToolStrip parent = this.ParentInternal; if (parent == null) { parent = Owner; } if (parent == null) { return new Size(WINBAR_SEPARATORTHICKNESS, WINBAR_SEPARATORTHICKNESS); } ToolStripDropDownMenu dropDownMenu = parent as ToolStripDropDownMenu; if (dropDownMenu != null) { return new Size(parent.Width - (parent.Padding.Horizontal - dropDownMenu.ImageMargin.Width), WINBAR_SEPARATORTHICKNESS); } else { if (parent.LayoutStyle != ToolStripLayoutStyle.HorizontalStackWithOverflow || parent.LayoutStyle != ToolStripLayoutStyle.VerticalStackWithOverflow) { // we dont actually know what size to make it, so just keep it a stock size. constrainingSize.Width = WINBAR_SEPARATORHEIGHT; constrainingSize.Height = WINBAR_SEPARATORHEIGHT; } if (IsVertical) { return new Size(WINBAR_SEPARATORTHICKNESS, constrainingSize.Height); } else { return new Size(constrainingSize.Width, WINBAR_SEPARATORTHICKNESS); } } } /// protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { if (this.Owner != null && this.ParentInternal != null) { this.Renderer.DrawSeparator(new ToolStripSeparatorRenderEventArgs(e.Graphics, this, IsVertical)); } } [EditorBrowsable(EditorBrowsableState.Never)] protected override void OnFontChanged(EventArgs e) { // PERF: dont call base, we dont care if the font changes RaiseEvent(EventFontChanged, e); } [SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] [EditorBrowsable(EditorBrowsableState.Never)] internal override bool ShouldSerializeForeColor() { return (ForeColor != SystemColors.ControlDark); } internal protected override void SetBounds(Rectangle rect) { ToolStripDropDownMenu dropDownMenu = this.Owner as ToolStripDropDownMenu; if (dropDownMenu != null) { // Scooch over by the padding amount. The padding is added to // the ToolStripDropDownMenu to keep the non-menu item riffraff // aligned to the text rectangle. When flow layout comes through to set our position // via IArrangedElement DEFY IT! if (dropDownMenu != null) { rect.X = 2; rect.Width = dropDownMenu.Width -4; } } base.SetBounds(rect); } /// /// An implementation of AccessibleChild for use with ToolStripItems /// [System.Runtime.InteropServices.ComVisible(true)] internal class ToolStripSeparatorAccessibleObject : ToolStripItemAccessibleObject { private ToolStripSeparator ownerItem = null; public ToolStripSeparatorAccessibleObject(ToolStripSeparator ownerItem): base(ownerItem) { this.ownerItem = ownerItem; } public override AccessibleRole Role { get { AccessibleRole role = ownerItem.AccessibleRole; if (role != AccessibleRole.Default) { return role; } else { return AccessibleRole.Separator; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.ComponentModel; using System.Windows.Forms.Design; using System.Diagnostics.CodeAnalysis; using System.Runtime.Versioning; ////// /// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.ToolStrip | ToolStripItemDesignerAvailability.ContextMenuStrip)] public class ToolStripSeparator : ToolStripItem { private const int WINBAR_SEPARATORTHICKNESS = 6; private const int WINBAR_SEPARATORHEIGHT = 23; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] public ToolStripSeparator() { this.ForeColor = SystemColors.ControlDark; } ////// Called when the background of the winbar is being rendered /// ///[ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new bool AutoToolTip { get { return base.AutoToolTip; } set { base.AutoToolTip = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override Image BackgroundImage { get { return base.BackgroundImage; } set { base.BackgroundImage = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override ImageLayout BackgroundImageLayout { get { return base.BackgroundImageLayout; } set { base.BackgroundImageLayout = value; } } /// public override bool CanSelect { get { return DesignMode; } } /// /// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected override Size DefaultSize { get { return new Size(WINBAR_SEPARATORTHICKNESS, WINBAR_SEPARATORTHICKNESS); } } ///protected internal override Padding DefaultMargin { get { return Padding.Empty; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new bool DoubleClickEnabled { get { return base.DoubleClickEnabled; } set { base.DoubleClickEnabled = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override bool Enabled { get { return base.Enabled; } set { base.Enabled = value; } } /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler EnabledChanged { add { base.EnabledChanged += value; } remove { base.EnabledChanged -= value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new ToolStripItemDisplayStyle DisplayStyle { get { return base.DisplayStyle; } set { base.DisplayStyle = value; } } /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler DisplayStyleChanged { add { base.DisplayStyleChanged += value; } remove { base.DisplayStyleChanged -= value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override Font Font { get { return base.Font; } set { base.Font = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new ContentAlignment ImageAlign { get { return base.ImageAlign; } set { base.ImageAlign = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override Image Image { [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] get { return base.Image; } set { base.Image = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), RefreshProperties(RefreshProperties.Repaint), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new int ImageIndex { get { return base.ImageIndex; } set { base.ImageIndex = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new string ImageKey { get { return base.ImageKey; } set { base.ImageKey = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new Color ImageTransparentColor { get { return base.ImageTransparentColor; } set { base.ImageTransparentColor = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new ToolStripItemImageScaling ImageScaling { get { return base.ImageScaling; } set { base.ImageScaling = value; } } private bool IsVertical { get { ToolStrip parent = this.ParentInternal; if (parent == null) { parent = Owner; } ToolStripDropDownMenu dropDownMenu = parent as ToolStripDropDownMenu; if (dropDownMenu != null) { return false; } switch (parent.LayoutStyle) { case ToolStripLayoutStyle.VerticalStackWithOverflow: return false; case ToolStripLayoutStyle.HorizontalStackWithOverflow: case ToolStripLayoutStyle.Flow: case ToolStripLayoutStyle.Table: default: return true; } } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public override string Text { get { return base.Text; } set { base.Text = value; } } /// /// [Browsable (false), EditorBrowsable (EditorBrowsableState.Never)] new public event EventHandler TextChanged { add { base.TextChanged += value; } remove { base.TextChanged -= value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new ContentAlignment TextAlign { get { return base.TextAlign; } set { base.TextAlign = value; } } [Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DefaultValue(ToolStripTextDirection.Horizontal)] public override ToolStripTextDirection TextDirection { get { return base.TextDirection; } set { base.TextDirection = value; } } /// [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new TextImageRelation TextImageRelation { get { return base.TextImageRelation; } set { base.TextImageRelation = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new string ToolTipText { get { return base.ToolTipText; } set { base.ToolTipText = value; } } [ Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public new bool RightToLeftAutoMirrorImage { get { return base.RightToLeftAutoMirrorImage; } set { base.RightToLeftAutoMirrorImage = value; } } [EditorBrowsable(EditorBrowsableState.Advanced)] protected override AccessibleObject CreateAccessibilityInstance() { return new ToolStripSeparatorAccessibleObject(this); } /// public override Size GetPreferredSize(Size constrainingSize) { ToolStrip parent = this.ParentInternal; if (parent == null) { parent = Owner; } if (parent == null) { return new Size(WINBAR_SEPARATORTHICKNESS, WINBAR_SEPARATORTHICKNESS); } ToolStripDropDownMenu dropDownMenu = parent as ToolStripDropDownMenu; if (dropDownMenu != null) { return new Size(parent.Width - (parent.Padding.Horizontal - dropDownMenu.ImageMargin.Width), WINBAR_SEPARATORTHICKNESS); } else { if (parent.LayoutStyle != ToolStripLayoutStyle.HorizontalStackWithOverflow || parent.LayoutStyle != ToolStripLayoutStyle.VerticalStackWithOverflow) { // we dont actually know what size to make it, so just keep it a stock size. constrainingSize.Width = WINBAR_SEPARATORHEIGHT; constrainingSize.Height = WINBAR_SEPARATORHEIGHT; } if (IsVertical) { return new Size(WINBAR_SEPARATORTHICKNESS, constrainingSize.Height); } else { return new Size(constrainingSize.Width, WINBAR_SEPARATORTHICKNESS); } } } /// protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { if (this.Owner != null && this.ParentInternal != null) { this.Renderer.DrawSeparator(new ToolStripSeparatorRenderEventArgs(e.Graphics, this, IsVertical)); } } [EditorBrowsable(EditorBrowsableState.Never)] protected override void OnFontChanged(EventArgs e) { // PERF: dont call base, we dont care if the font changes RaiseEvent(EventFontChanged, e); } [SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] [EditorBrowsable(EditorBrowsableState.Never)] internal override bool ShouldSerializeForeColor() { return (ForeColor != SystemColors.ControlDark); } internal protected override void SetBounds(Rectangle rect) { ToolStripDropDownMenu dropDownMenu = this.Owner as ToolStripDropDownMenu; if (dropDownMenu != null) { // Scooch over by the padding amount. The padding is added to // the ToolStripDropDownMenu to keep the non-menu item riffraff // aligned to the text rectangle. When flow layout comes through to set our position // via IArrangedElement DEFY IT! if (dropDownMenu != null) { rect.X = 2; rect.Width = dropDownMenu.Width -4; } } base.SetBounds(rect); } /// /// An implementation of AccessibleChild for use with ToolStripItems /// [System.Runtime.InteropServices.ComVisible(true)] internal class ToolStripSeparatorAccessibleObject : ToolStripItemAccessibleObject { private ToolStripSeparator ownerItem = null; public ToolStripSeparatorAccessibleObject(ToolStripSeparator ownerItem): base(ownerItem) { this.ownerItem = ownerItem; } public override AccessibleRole Role { get { AccessibleRole role = ownerItem.AccessibleRole; if (role != AccessibleRole.Default) { return role; } else { return AccessibleRole.Separator; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
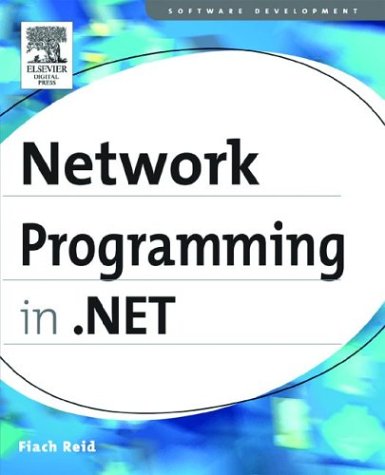
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimerExtension.cs
- OdbcConnectionOpen.cs
- X509Certificate2.cs
- sqlpipe.cs
- ConsumerConnectionPoint.cs
- RangeValuePatternIdentifiers.cs
- WebBrowserHelper.cs
- JsonServiceDocumentSerializer.cs
- DynamicDataExtensions.cs
- TimeSpanValidatorAttribute.cs
- DataServiceKeyAttribute.cs
- IPAddressCollection.cs
- RNGCryptoServiceProvider.cs
- DocumentViewerBase.cs
- TabControlEvent.cs
- TimeEnumHelper.cs
- HttpContextBase.cs
- LinqToSqlWrapper.cs
- SocketException.cs
- FontStretchConverter.cs
- ObjectViewQueryResultData.cs
- Effect.cs
- HtmlLiteralTextAdapter.cs
- SymbolType.cs
- RectKeyFrameCollection.cs
- ListViewContainer.cs
- PageHandlerFactory.cs
- Image.cs
- Timer.cs
- wmiprovider.cs
- FilterFactory.cs
- InfiniteTimeSpanConverter.cs
- ProviderUtil.cs
- ConvertBinder.cs
- ReferencedAssembly.cs
- ApplicationFileCodeDomTreeGenerator.cs
- QilStrConcatenator.cs
- OutputScopeManager.cs
- Pool.cs
- AesManaged.cs
- InvalidFilterCriteriaException.cs
- ContainerControl.cs
- ImageIndexConverter.cs
- DesignerTransactionCloseEvent.cs
- DesignerSerializerAttribute.cs
- AvTraceDetails.cs
- ObjectManager.cs
- CodeTryCatchFinallyStatement.cs
- EventListener.cs
- MasterPageParser.cs
- XmlBinaryWriter.cs
- ConstantExpression.cs
- WebHttpBindingElement.cs
- InteropAutomationProvider.cs
- NetDataContractSerializer.cs
- ColumnHeader.cs
- XmlAnyElementAttributes.cs
- NonSerializedAttribute.cs
- XamlToRtfWriter.cs
- WindowsTitleBar.cs
- Context.cs
- DbSource.cs
- RegisteredExpandoAttribute.cs
- UpdatePanelTriggerCollection.cs
- StorageMappingItemCollection.cs
- UrlMappingCollection.cs
- CellQuery.cs
- TypeDescriptionProvider.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- DBConnection.cs
- TdsParser.cs
- StrongNameHelpers.cs
- EntityDataSourceViewSchema.cs
- FilteredReadOnlyMetadataCollection.cs
- SystemIPGlobalStatistics.cs
- WindowShowOrOpenTracker.cs
- PowerStatus.cs
- SettingsBindableAttribute.cs
- RenderingEventArgs.cs
- DatePickerTextBox.cs
- ConnectionStringSettingsCollection.cs
- cookiecollection.cs
- XmlSchemaObject.cs
- MetadataResolver.cs
- SystemTcpConnection.cs
- BuiltInPermissionSets.cs
- ConfigurationManagerHelperFactory.cs
- Win32.cs
- FontFamily.cs
- UniqueConstraint.cs
- MethodImplAttribute.cs
- JoinQueryOperator.cs
- DataGridViewTextBoxEditingControl.cs
- ChoiceConverter.cs
- TypeBuilderInstantiation.cs
- TextProviderWrapper.cs
- MultiplexingDispatchMessageFormatter.cs
- XslVisitor.cs
- CodeTypeParameter.cs
- ThemeDictionaryExtension.cs