Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / Ink / TextClipboardData.cs / 1 / TextClipboardData.cs
//---------------------------------------------------------------------------- // // File: TextClipboardData.cs // // Description: // A base class which can copy a unicode text to the IDataObject. // It also can get the unicode text from the IDataObject and create a corresponding textbox. // // Features: // // History: // 11/17/2004 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Controls; using System.Windows.Markup; using System.Text; namespace MS.Internal.Ink { internal class TextClipboardData : ElementsClipboardData { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors // The default constructor internal TextClipboardData() : this(null) {} // The constructor with a string as argument internal TextClipboardData(string text) { _text = text; } #endregion Constructors // Checks if the data can be pasted. internal override bool CanPaste(IDataObject dataObject) { return ( dataObject.GetDataPresent(DataFormats.UnicodeText, false) || dataObject.GetDataPresent(DataFormats.Text, false) || dataObject.GetDataPresent(DataFormats.OemText, false) ); } //-------------------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------------------- #region Protected Methods // Checks if the data can be copied. protected override bool CanCopy() { return !string.IsNullOrEmpty(_text); } // Copy the text to the IDataObject protected override void DoCopy(IDataObject dataObject) { // Put the text to the clipboard dataObject.SetData(DataFormats.UnicodeText, _text, true); } // Retrieves the text from the IDataObject instance. // Then create a textbox with the text data. protected override void DoPaste(IDataObject dataObject) { ElementList = new List(); // Get the string from the data object. string text = dataObject.GetData(DataFormats.UnicodeText, true) as string; if ( String.IsNullOrEmpty(text) ) { // OemText can be retrieved as CF_TEXT. text = dataObject.GetData(DataFormats.Text, true) as string; } if ( !String.IsNullOrEmpty(text) ) { // Now, create a text box and set the text to it. TextBox textBox = new TextBox(); textBox.Text = text; textBox.TextWrapping = TextWrapping.Wrap; // Add the textbox to the internal array list. ElementList.Add(textBox); } } #endregion Protected Methods //-------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private string _text; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextClipboardData.cs // // Description: // A base class which can copy a unicode text to the IDataObject. // It also can get the unicode text from the IDataObject and create a corresponding textbox. // // Features: // // History: // 11/17/2004 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Controls; using System.Windows.Markup; using System.Text; namespace MS.Internal.Ink { internal class TextClipboardData : ElementsClipboardData { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors // The default constructor internal TextClipboardData() : this(null) {} // The constructor with a string as argument internal TextClipboardData(string text) { _text = text; } #endregion Constructors // Checks if the data can be pasted. internal override bool CanPaste(IDataObject dataObject) { return ( dataObject.GetDataPresent(DataFormats.UnicodeText, false) || dataObject.GetDataPresent(DataFormats.Text, false) || dataObject.GetDataPresent(DataFormats.OemText, false) ); } //-------------------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------------------- #region Protected Methods // Checks if the data can be copied. protected override bool CanCopy() { return !string.IsNullOrEmpty(_text); } // Copy the text to the IDataObject protected override void DoCopy(IDataObject dataObject) { // Put the text to the clipboard dataObject.SetData(DataFormats.UnicodeText, _text, true); } // Retrieves the text from the IDataObject instance. // Then create a textbox with the text data. protected override void DoPaste(IDataObject dataObject) { ElementList = new List (); // Get the string from the data object. string text = dataObject.GetData(DataFormats.UnicodeText, true) as string; if ( String.IsNullOrEmpty(text) ) { // OemText can be retrieved as CF_TEXT. text = dataObject.GetData(DataFormats.Text, true) as string; } if ( !String.IsNullOrEmpty(text) ) { // Now, create a text box and set the text to it. TextBox textBox = new TextBox(); textBox.Text = text; textBox.TextWrapping = TextWrapping.Wrap; // Add the textbox to the internal array list. ElementList.Add(textBox); } } #endregion Protected Methods //-------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private string _text; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
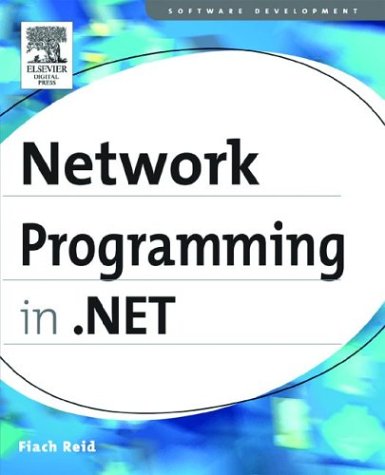
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LineGeometry.cs
- VirtualPath.cs
- LoadGrammarCompletedEventArgs.cs
- Baml6ConstructorInfo.cs
- EntityClientCacheEntry.cs
- UpdateTracker.cs
- ColorConverter.cs
- XmlIterators.cs
- ErrorEventArgs.cs
- SQLResource.cs
- IEnumerable.cs
- SerializationObjectManager.cs
- NavigatingCancelEventArgs.cs
- TextElementEnumerator.cs
- BasicHttpMessageSecurity.cs
- SafeNativeMethods.cs
- EnvironmentPermission.cs
- WebPartConnectionsCancelEventArgs.cs
- DeclaredTypeElementCollection.cs
- BaseDataBoundControlDesigner.cs
- XsdDataContractImporter.cs
- SelectManyQueryOperator.cs
- EdgeModeValidation.cs
- InputLanguageManager.cs
- RolePrincipal.cs
- QuaternionRotation3D.cs
- ExpressionStringBuilder.cs
- ErrorWrapper.cs
- ComponentResourceManager.cs
- NetworkInterface.cs
- UnicodeEncoding.cs
- TreePrinter.cs
- SqlServer2KCompatibilityCheck.cs
- AnyAllSearchOperator.cs
- TextTreeNode.cs
- DispatchChannelSink.cs
- Convert.cs
- ActionItem.cs
- RenamedEventArgs.cs
- RuleConditionDialog.cs
- ConnectionPoint.cs
- IdentifierCollection.cs
- Underline.cs
- BitmapVisualManager.cs
- Encoder.cs
- SqlDataSourceSelectingEventArgs.cs
- BitmapMetadataBlob.cs
- ClusterSafeNativeMethods.cs
- MessageQueuePermission.cs
- LayoutTable.cs
- BidOverLoads.cs
- FixedLineResult.cs
- EraserBehavior.cs
- TrueReadOnlyCollection.cs
- TableSectionStyle.cs
- PageWrapper.cs
- EncoderParameter.cs
- StateWorkerRequest.cs
- PointUtil.cs
- UseAttributeSetsAction.cs
- LicenseException.cs
- UriScheme.cs
- WsatStrings.cs
- HtmlForm.cs
- CapabilitiesSection.cs
- FixedSOMPage.cs
- ListComponentEditor.cs
- Model3D.cs
- HwndSourceKeyboardInputSite.cs
- UserControlParser.cs
- ParameterCollection.cs
- PageHandlerFactory.cs
- PersonalizationStateInfoCollection.cs
- ConnectionManagementElement.cs
- VirtualPathUtility.cs
- ConnectionStringsSection.cs
- TextBox.cs
- LockCookie.cs
- SQLMoney.cs
- FileUtil.cs
- EncoderParameters.cs
- ByeOperation11AsyncResult.cs
- UInt64.cs
- HttpRuntimeSection.cs
- WindowsToolbar.cs
- ConfigLoader.cs
- SimpleFieldTemplateFactory.cs
- As.cs
- TextTreeTextBlock.cs
- remotingproxy.cs
- securestring.cs
- StreamingContext.cs
- EntityDataSourceDataSelectionPanel.designer.cs
- XmlAttributeCache.cs
- SystemIPv4InterfaceProperties.cs
- SurrogateDataContract.cs
- EventLogPermissionEntryCollection.cs
- _FtpDataStream.cs
- NetTcpSectionData.cs
- HttpListenerRequestTraceRecord.cs