Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Animation / AnimationTimeline.cs / 1 / AnimationTimeline.cs
// AnimationTimeline.cs using System; using System.Diagnostics; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// /// public abstract class AnimationTimeline : Timeline { ////// /// protected AnimationTimeline() : base() { } #region Dependency Properties private static void AnimationTimeline_PropertyChangedFunction(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((AnimationTimeline)d).PropertyChanged(e.Property); } ////// /// public static readonly DependencyProperty IsAdditiveProperty = DependencyProperty.Register( "IsAdditive", // Property Name typeof(bool), // Property Type typeof(AnimationTimeline), // Owner Class new PropertyMetadata(false, new PropertyChangedCallback(AnimationTimeline_PropertyChangedFunction))); ////// /// public static readonly DependencyProperty IsCumulativeProperty = DependencyProperty.Register( "IsCumulative", // Property Name typeof(bool), // Property Type typeof(AnimationTimeline), // Owner Class new PropertyMetadata(false, new PropertyChangedCallback(AnimationTimeline_PropertyChangedFunction))); #endregion #region Freezable ////// Creates a copy of this AnimationTimeline. /// ///The copy. public new AnimationTimeline Clone() { return (AnimationTimeline)base.Clone(); } #endregion #region Timeline ////// /// ///protected internal override Clock AllocateClock() { return new AnimationClock(this); } /// /// Creates a new AnimationClock using this AnimationTimeline. /// ///A new AnimationClock. new public AnimationClock CreateClock() { return (AnimationClock)base.CreateClock(); } #endregion ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is not the first in a composition /// chain this value will be the value returned by the previous /// animation in the chain with an animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. If this animation is not the first in a composition /// chain this value will be the value returned by the previous /// animation in the chain with an animationClock that is not Stopped. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public virtual object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); return defaultDestinationValue; } ////// Provide a custom natural Duration when the Duration property is set to Automatic. /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. Default is 1 second for animations. /// protected override Duration GetNaturalDurationCore(Clock clock) { return new TimeSpan(0, 0, 1); } ////// Returns the type of the animation. /// ///public abstract Type TargetPropertyType { get; } /// /// This property is implemented by the animation to return true if the /// animation uses the defaultDestinationValue parameter to the /// GetCurrentValue method as its destination value. Specifically, if /// Progress is equal to 1.0, will this animation return the /// default destination value as its current value. /// public virtual bool IsDestinationDefault { get { ReadPreamble(); return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // AnimationTimeline.cs using System; using System.Diagnostics; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// /// public abstract class AnimationTimeline : Timeline { ////// /// protected AnimationTimeline() : base() { } #region Dependency Properties private static void AnimationTimeline_PropertyChangedFunction(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((AnimationTimeline)d).PropertyChanged(e.Property); } ////// /// public static readonly DependencyProperty IsAdditiveProperty = DependencyProperty.Register( "IsAdditive", // Property Name typeof(bool), // Property Type typeof(AnimationTimeline), // Owner Class new PropertyMetadata(false, new PropertyChangedCallback(AnimationTimeline_PropertyChangedFunction))); ////// /// public static readonly DependencyProperty IsCumulativeProperty = DependencyProperty.Register( "IsCumulative", // Property Name typeof(bool), // Property Type typeof(AnimationTimeline), // Owner Class new PropertyMetadata(false, new PropertyChangedCallback(AnimationTimeline_PropertyChangedFunction))); #endregion #region Freezable ////// Creates a copy of this AnimationTimeline. /// ///The copy. public new AnimationTimeline Clone() { return (AnimationTimeline)base.Clone(); } #endregion #region Timeline ////// /// ///protected internal override Clock AllocateClock() { return new AnimationClock(this); } /// /// Creates a new AnimationClock using this AnimationTimeline. /// ///A new AnimationClock. new public AnimationClock CreateClock() { return (AnimationClock)base.CreateClock(); } #endregion ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is not the first in a composition /// chain this value will be the value returned by the previous /// animation in the chain with an animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. If this animation is not the first in a composition /// chain this value will be the value returned by the previous /// animation in the chain with an animationClock that is not Stopped. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public virtual object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); return defaultDestinationValue; } ////// Provide a custom natural Duration when the Duration property is set to Automatic. /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. Default is 1 second for animations. /// protected override Duration GetNaturalDurationCore(Clock clock) { return new TimeSpan(0, 0, 1); } ////// Returns the type of the animation. /// ///public abstract Type TargetPropertyType { get; } /// /// This property is implemented by the animation to return true if the /// animation uses the defaultDestinationValue parameter to the /// GetCurrentValue method as its destination value. Specifically, if /// Progress is equal to 1.0, will this animation return the /// default destination value as its current value. /// public virtual bool IsDestinationDefault { get { ReadPreamble(); return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
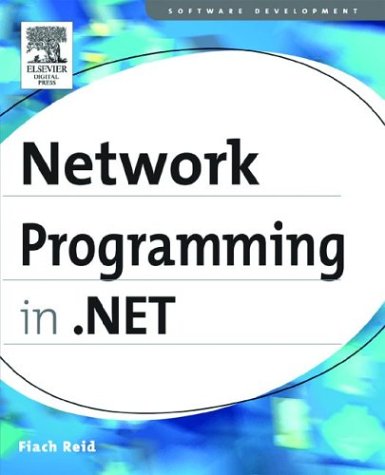
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PackageStore.cs
- TriggerAction.cs
- DataServiceBehavior.cs
- OperationAbortedException.cs
- BufferAllocator.cs
- EmptyStringExpandableObjectConverter.cs
- AsymmetricKeyExchangeFormatter.cs
- TCEAdapterGenerator.cs
- BitmapScalingModeValidation.cs
- PointValueSerializer.cs
- SplayTreeNode.cs
- ProxyHelper.cs
- DefaultMemberAttribute.cs
- PerformanceCounter.cs
- SignalGate.cs
- SvcMapFileLoader.cs
- ComPlusTraceRecord.cs
- ManagementObject.cs
- DuplicateWaitObjectException.cs
- SourceFileInfo.cs
- BitmapMetadataEnumerator.cs
- SamlAuthenticationClaimResource.cs
- FileClassifier.cs
- DeferredBinaryDeserializerExtension.cs
- SerialErrors.cs
- XmlObjectSerializerReadContextComplex.cs
- NativeMethods.cs
- AttachedPropertiesService.cs
- _ListenerAsyncResult.cs
- ColumnReorderedEventArgs.cs
- ContextProperty.cs
- _IPv4Address.cs
- TabItemAutomationPeer.cs
- XmlTypeAttribute.cs
- AsyncWaitHandle.cs
- AppDomainUnloadedException.cs
- ConfigurationStrings.cs
- SiteMap.cs
- TaskFileService.cs
- ScrollChangedEventArgs.cs
- HMAC.cs
- FileDialogPermission.cs
- LayoutUtils.cs
- SettingsContext.cs
- Group.cs
- Image.cs
- ToolStripPanelRenderEventArgs.cs
- ContextItem.cs
- Bidi.cs
- LogConverter.cs
- ContentType.cs
- BitmapCache.cs
- ProcessInfo.cs
- CompilerResults.cs
- RtfControls.cs
- FontConverter.cs
- ObjectResult.cs
- FamilyMap.cs
- DesignerTextWriter.cs
- TextureBrush.cs
- CategoryAttribute.cs
- PeerCustomResolverSettings.cs
- OptimisticConcurrencyException.cs
- CodeTryCatchFinallyStatement.cs
- SecurityKeyIdentifierClause.cs
- Tuple.cs
- ThicknessAnimationBase.cs
- OleDbCommand.cs
- AnalyzedTree.cs
- ShapeTypeface.cs
- DiscriminatorMap.cs
- AsymmetricSecurityProtocolFactory.cs
- ColorComboBox.cs
- ItemsChangedEventArgs.cs
- OutgoingWebResponseContext.cs
- ServicePointManagerElement.cs
- SplitterPanelDesigner.cs
- HwndStylusInputProvider.cs
- CurrentChangedEventManager.cs
- PromptStyle.cs
- QilTargetType.cs
- PrintingPermission.cs
- ResizingMessageFilter.cs
- PropertyPushdownHelper.cs
- BypassElement.cs
- EntityDataSourceWrapperCollection.cs
- EventManager.cs
- WebControlToolBoxItem.cs
- CroppedBitmap.cs
- Propagator.ExtentPlaceholderCreator.cs
- GenericUI.cs
- RegisteredDisposeScript.cs
- BridgeDataReader.cs
- ReachUIElementCollectionSerializer.cs
- InvalidDocumentContentsException.cs
- EnumerableCollectionView.cs
- MachineKeyValidationConverter.cs
- RegistryConfigurationProvider.cs
- ExtendedProperty.cs
- ViewManager.cs