Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / OneOfTypeConst.cs / 2 / OneOfTypeConst.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration.Structures { using DomainBoolExpr = BoolExpr>; using CellConstantSet = Set ; // A class that denotes the boolean expression: "varType in values" // See the comments in OneOfConst for partially and fully-done objects internal class OneOfTypeConst : OneOfConst { #region Constructors // effects: Creates a partial OneOfTypeConst of the form "node in values" internal OneOfTypeConst(JoinTreeNode node, IEnumerable values) : base(new JoinTreeSlot(node), CreateTypeConstants(values)) { } // effects: Creates a partial OneOfTypeConst of the form "node = values" internal OneOfTypeConst(JoinTreeNode node, CellConstant value) : base(new JoinTreeSlot(node), value) { Debug.Assert(value is TypeConstant || value.IsNull(), "Type or NULL expected"); } // effects: Creates an expression of the form "type of node in type constants // corresponding to values", i.e., a boolean expression of the form "TypeOf(slot) in values" // possibleValues are all the values that node's type can take internal OneOfTypeConst(JoinTreeNode node, IEnumerable values, IEnumerable possibleValues) : base(new JoinTreeSlot(node), CreateTypeConstants(values), CreateTypeConstants(possibleValues)) { } // effects: Creates a "fully-done" OneOfTypeConst that represents // "node in values". possibleValues are all the values that node's type can take internal OneOfTypeConst(JoinTreeNode node, IEnumerable values, IEnumerable possibleValues) : base(new JoinTreeSlot(node), values, possibleValues) { } // effects: Creates an expression of the form "slot = value" // possibleValues are all the values that slot can take internal OneOfTypeConst(JoinTreeNode node, CellConstant value, IEnumerable possibleValues) : base(new JoinTreeSlot(node), value, possibleValues) { Debug.Assert(value is TypeConstant || value.IsNull(), "Type or NULL expected"); } // effects: Creates an expression of the form "slot in domain" internal OneOfTypeConst(JoinTreeSlot slot, CellConstantDomain domain) : base(slot, domain) { } #endregion #region Helper static methods // effects: Given a list of types (which can contain nulls), // returns a corresponding list of Type Constants for those types private static IEnumerable CreateTypeConstants(IEnumerable types) { foreach (EdmType type in types) { if (type == null) { yield return CellConstant.Null; } else { yield return new TypeConstant(type); } } } #endregion #region Methods // requires: IsFullyDone is true // effects: Fixes the range of this in accordance with range internal override DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap) { Debug.Assert(IsFullyDone, "Ranges are fixed only for fully done OneOfConsts"); IEnumerable possibleValues = memberDomainMap.GetDomain(Slot.MemberPath); BoolLiteral newLiteral = new OneOfTypeConst(Slot, new CellConstantDomain(range, possibleValues)); return newLiteral.GetDomainBoolExpression(memberDomainMap); } internal override StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { // type comparison: make a big OR // Important to enclose all the OR statements in () ! if (Values.Count > 1) { builder.Append('('); } bool isFirst = true; // Add Cql of the form "(T.A IS OF (ONLY Person) OR .....)" foreach (CellConstant constant in Values.Values) { TypeConstant typeConstant = constant as TypeConstant; Debug.Assert(typeConstant != null || constant.IsNull(), "Constants for type checks must be type constants or NULLs"); if (isFirst == false) { builder.Append(" OR "); } isFirst = false; if (Slot.MemberPath.EdmType is RefType) { builder.Append("Deref("); Slot.MemberPath.AsCql(builder, blockAlias); builder.Append(')'); } else { // non-reference type Slot.MemberPath.AsCql(builder, blockAlias); } if (constant.IsNull()) { builder.Append(" IS NULL"); } else { // type constant builder.Append(" IS OF (ONLY "); CqlWriter.AppendEscapedTypeName(builder, typeConstant.CdmType); builder.Append(')'); } } if (Values.Count > 1) { builder.Append(')'); } return builder; } internal override StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { // Add user readable string of the form "(T.A IS OF (ONLY Person) OR .....)" if (Slot.MemberPath.EdmType is RefType) { builder.Append("Deref("); Slot.MemberPath.AsCql(builder, blockAlias); builder.Append(')'); } else { // non-reference type Slot.MemberPath.AsCql(builder, blockAlias); } if (Values.Count > 1) { builder.Append(" is a ("); } else { builder.Append(" is type "); } bool isFirst = true; foreach (CellConstant constant in Values.Values) { TypeConstant typeConstant = constant as TypeConstant; Debug.Assert(typeConstant != null || constant.IsNull(), "Constants for type checks must be type constants or NULLs"); if (isFirst == false) { builder.Append(" OR "); } if (constant.IsNull()) { builder.Append(" NULL"); } else { CqlWriter.AppendEscapedTypeName(builder, typeConstant.CdmType); } isFirst = false; } if (Values.Count > 1) { builder.Append(')'); } return builder; } #endregion #region String methods internal override void ToCompactString(StringBuilder builder) { builder.Append("type("); Slot.ToCompactString(builder); builder.Append(") IN ("); StringUtil.ToCommaSeparatedStringSorted(builder, Values.Values); builder.Append(")"); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Common.Utils.Boolean; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration.Structures { using DomainBoolExpr = BoolExpr>; using CellConstantSet = Set ; // A class that denotes the boolean expression: "varType in values" // See the comments in OneOfConst for partially and fully-done objects internal class OneOfTypeConst : OneOfConst { #region Constructors // effects: Creates a partial OneOfTypeConst of the form "node in values" internal OneOfTypeConst(JoinTreeNode node, IEnumerable values) : base(new JoinTreeSlot(node), CreateTypeConstants(values)) { } // effects: Creates a partial OneOfTypeConst of the form "node = values" internal OneOfTypeConst(JoinTreeNode node, CellConstant value) : base(new JoinTreeSlot(node), value) { Debug.Assert(value is TypeConstant || value.IsNull(), "Type or NULL expected"); } // effects: Creates an expression of the form "type of node in type constants // corresponding to values", i.e., a boolean expression of the form "TypeOf(slot) in values" // possibleValues are all the values that node's type can take internal OneOfTypeConst(JoinTreeNode node, IEnumerable values, IEnumerable possibleValues) : base(new JoinTreeSlot(node), CreateTypeConstants(values), CreateTypeConstants(possibleValues)) { } // effects: Creates a "fully-done" OneOfTypeConst that represents // "node in values". possibleValues are all the values that node's type can take internal OneOfTypeConst(JoinTreeNode node, IEnumerable values, IEnumerable possibleValues) : base(new JoinTreeSlot(node), values, possibleValues) { } // effects: Creates an expression of the form "slot = value" // possibleValues are all the values that slot can take internal OneOfTypeConst(JoinTreeNode node, CellConstant value, IEnumerable possibleValues) : base(new JoinTreeSlot(node), value, possibleValues) { Debug.Assert(value is TypeConstant || value.IsNull(), "Type or NULL expected"); } // effects: Creates an expression of the form "slot in domain" internal OneOfTypeConst(JoinTreeSlot slot, CellConstantDomain domain) : base(slot, domain) { } #endregion #region Helper static methods // effects: Given a list of types (which can contain nulls), // returns a corresponding list of Type Constants for those types private static IEnumerable CreateTypeConstants(IEnumerable types) { foreach (EdmType type in types) { if (type == null) { yield return CellConstant.Null; } else { yield return new TypeConstant(type); } } } #endregion #region Methods // requires: IsFullyDone is true // effects: Fixes the range of this in accordance with range internal override DomainBoolExpr FixRange(Set range, MemberDomainMap memberDomainMap) { Debug.Assert(IsFullyDone, "Ranges are fixed only for fully done OneOfConsts"); IEnumerable possibleValues = memberDomainMap.GetDomain(Slot.MemberPath); BoolLiteral newLiteral = new OneOfTypeConst(Slot, new CellConstantDomain(range, possibleValues)); return newLiteral.GetDomainBoolExpression(memberDomainMap); } internal override StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { // type comparison: make a big OR // Important to enclose all the OR statements in () ! if (Values.Count > 1) { builder.Append('('); } bool isFirst = true; // Add Cql of the form "(T.A IS OF (ONLY Person) OR .....)" foreach (CellConstant constant in Values.Values) { TypeConstant typeConstant = constant as TypeConstant; Debug.Assert(typeConstant != null || constant.IsNull(), "Constants for type checks must be type constants or NULLs"); if (isFirst == false) { builder.Append(" OR "); } isFirst = false; if (Slot.MemberPath.EdmType is RefType) { builder.Append("Deref("); Slot.MemberPath.AsCql(builder, blockAlias); builder.Append(')'); } else { // non-reference type Slot.MemberPath.AsCql(builder, blockAlias); } if (constant.IsNull()) { builder.Append(" IS NULL"); } else { // type constant builder.Append(" IS OF (ONLY "); CqlWriter.AppendEscapedTypeName(builder, typeConstant.CdmType); builder.Append(')'); } } if (Values.Count > 1) { builder.Append(')'); } return builder; } internal override StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { // Add user readable string of the form "(T.A IS OF (ONLY Person) OR .....)" if (Slot.MemberPath.EdmType is RefType) { builder.Append("Deref("); Slot.MemberPath.AsCql(builder, blockAlias); builder.Append(')'); } else { // non-reference type Slot.MemberPath.AsCql(builder, blockAlias); } if (Values.Count > 1) { builder.Append(" is a ("); } else { builder.Append(" is type "); } bool isFirst = true; foreach (CellConstant constant in Values.Values) { TypeConstant typeConstant = constant as TypeConstant; Debug.Assert(typeConstant != null || constant.IsNull(), "Constants for type checks must be type constants or NULLs"); if (isFirst == false) { builder.Append(" OR "); } if (constant.IsNull()) { builder.Append(" NULL"); } else { CqlWriter.AppendEscapedTypeName(builder, typeConstant.CdmType); } isFirst = false; } if (Values.Count > 1) { builder.Append(')'); } return builder; } #endregion #region String methods internal override void ToCompactString(StringBuilder builder) { builder.Append("type("); Slot.ToCompactString(builder); builder.Append(") IN ("); StringUtil.ToCommaSeparatedStringSorted(builder, Values.Values); builder.Append(")"); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
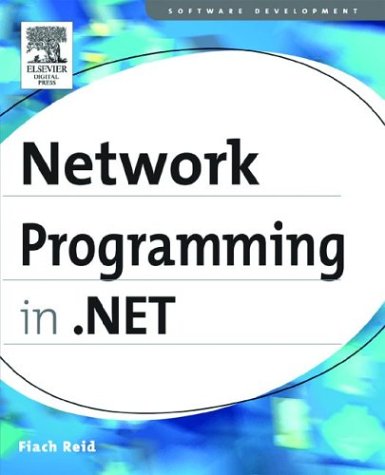
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ButtonBaseAdapter.cs
- JsonReaderWriterFactory.cs
- DataBoundControl.cs
- SpeechRecognitionEngine.cs
- StylusShape.cs
- InputBindingCollection.cs
- TailCallAnalyzer.cs
- XmlSchemaComplexContentExtension.cs
- TemplateLookupAction.cs
- Drawing.cs
- XmlJsonReader.cs
- DataGridViewComponentPropertyGridSite.cs
- BindingGroup.cs
- SmiEventSink_Default.cs
- InputReport.cs
- Formatter.cs
- StorageTypeMapping.cs
- List.cs
- FilteredReadOnlyMetadataCollection.cs
- PersistenceTypeAttribute.cs
- XmlSerializableReader.cs
- OleDbRowUpdatedEvent.cs
- SectionXmlInfo.cs
- IPHostEntry.cs
- WebPartDisplayModeEventArgs.cs
- DataServiceExpressionVisitor.cs
- ComplexTypeEmitter.cs
- Function.cs
- SystemIPInterfaceStatistics.cs
- PageMediaType.cs
- Attribute.cs
- PieceDirectory.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- LeftCellWrapper.cs
- OdbcConnectionOpen.cs
- XPathMultyIterator.cs
- ServiceObjectContainer.cs
- TextSelection.cs
- DoWhile.cs
- XsdBuildProvider.cs
- CharEntityEncoderFallback.cs
- ContourSegment.cs
- ContextStaticAttribute.cs
- ValidatorCompatibilityHelper.cs
- DataExpression.cs
- PersianCalendar.cs
- XPathMultyIterator.cs
- _CookieModule.cs
- FloaterBaseParaClient.cs
- RegionInfo.cs
- OdbcCommandBuilder.cs
- IIS7WorkerRequest.cs
- CalculatedColumn.cs
- PersonalizablePropertyEntry.cs
- SplitContainerDesigner.cs
- HostedHttpContext.cs
- SecureEnvironment.cs
- Parameter.cs
- Clock.cs
- OneWayChannelListener.cs
- StrokeFIndices.cs
- XmlRootAttribute.cs
- Documentation.cs
- SqlMethods.cs
- EnvelopedSignatureTransform.cs
- OptimisticConcurrencyException.cs
- DataGridItem.cs
- DataFormat.cs
- VerticalAlignConverter.cs
- FontFamilyConverter.cs
- DeviceSpecificDesigner.cs
- OdbcUtils.cs
- WorkItem.cs
- ReadOnlyHierarchicalDataSource.cs
- CodeCommentStatement.cs
- QueryRewriter.cs
- PerCallInstanceContextProvider.cs
- OleDbStruct.cs
- FormViewCommandEventArgs.cs
- Utils.cs
- ObjectItemAssemblyLoader.cs
- compensatingcollection.cs
- Context.cs
- ConnectionsZone.cs
- ColumnWidthChangedEvent.cs
- XmlSchemaGroupRef.cs
- BinHexEncoder.cs
- SqlParameterCollection.cs
- oledbmetadatacollectionnames.cs
- PreviewPrintController.cs
- MultiByteCodec.cs
- ComponentChangedEvent.cs
- SpAudioStreamWrapper.cs
- DynamicResourceExtensionConverter.cs
- NameScope.cs
- FormatConvertedBitmap.cs
- UInt16Converter.cs
- ContentPresenter.cs
- DateTimeConverter.cs
- RecommendedAsConfigurableAttribute.cs