Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / GraphicsContext.cs / 1 / GraphicsContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System; using System.Drawing.Drawing2D; using System.Diagnostics; ////// Contains information about the context of a Graphics object. /// internal class GraphicsContext : IDisposable { ////// The state that identifies the context. /// private int contextState; ////// The context's translate transform. /// private PointF transformOffset; ////// The context's clip region. /// private Region clipRegion; ////// The next context up the stack. /// private GraphicsContext nextContext; ////// The previous context down the stack. /// private GraphicsContext prevContext; ////// Flags that determines whether the context was created for a Graphics.Save() operation. /// This kind of contexts are cumulative across subsequent Save() calls so the top context /// info is cumulative. This is not the same for contexts created for a Graphics.BeginContainer() /// operation, in this case the new context information is reset. See Graphics.BeginContainer() /// and Graphics.Save() for more information. /// bool isCumulative; ////// Private constructor disallowed. /// private GraphicsContext() { } public GraphicsContext(Graphics g) { Matrix transform = g.Transform; if (!transform.IsIdentity) { float[] elements = transform.Elements; this.transformOffset.X = elements[4]; this.transformOffset.Y = elements[5]; } transform.Dispose(); Region clip = g.Clip; if (clip.IsInfinite(g)) { clip.Dispose(); } else { this.clipRegion = clip; } } ////// Disposes this and all contexts up the stack. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Disposes this and all contexts up the stack. /// public void Dispose(bool disposing) { if (this.nextContext != null) { // Dispose all contexts up the stack since they are relative to this one and its state will be invalid. this.nextContext.Dispose(); this.nextContext = null; } if (this.clipRegion != null) { this.clipRegion.Dispose(); this.clipRegion = null; } } ////// The state id representing the GraphicsContext. /// public int State { get { return this.contextState; } set { this.contextState = value; } } ////// The translate transform in the GraphicsContext. /// public PointF TransformOffset { get { return this.transformOffset; } } ////// The clipping region the GraphicsContext. /// public Region Clip { get { return this.clipRegion; } } ////// The next GraphicsContext object in the stack. /// public GraphicsContext Next { get { return this.nextContext; } set { this.nextContext = value; } } ////// The previous GraphicsContext object in the stack. /// public GraphicsContext Previous { get { return this.prevContext; } set { this.prevContext = value; } } ////// Determines whether this context is cumulative or not. See filed for more info. /// public bool IsCumulative { get { return this.isCumulative; } set { this.isCumulative = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
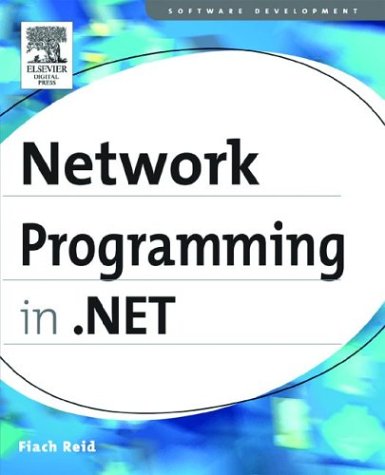
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UInt16.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- ReflectEventDescriptor.cs
- IsolationInterop.cs
- DefaultAsyncDataDispatcher.cs
- InputLangChangeRequestEvent.cs
- ValueSerializer.cs
- MsmqIntegrationBindingElement.cs
- CaseInsensitiveHashCodeProvider.cs
- CacheVirtualItemsEvent.cs
- HelloMessageApril2005.cs
- ConversionContext.cs
- TextTreeInsertElementUndoUnit.cs
- SqlDataSourceSummaryPanel.cs
- MachineSettingsSection.cs
- MultiPageTextView.cs
- baseshape.cs
- CommonRemoteMemoryBlock.cs
- PropertySourceInfo.cs
- NamespaceList.cs
- PresentationAppDomainManager.cs
- Thread.cs
- RoleService.cs
- CollectionBase.cs
- SqlTriggerAttribute.cs
- PassportAuthentication.cs
- MobileControlBuilder.cs
- SecurityRequiresReviewAttribute.cs
- _NegotiateClient.cs
- PathFigure.cs
- ImageAnimator.cs
- TypeDescriptionProvider.cs
- StreamUpdate.cs
- KeyboardNavigation.cs
- HttpContextWrapper.cs
- MatrixTransform.cs
- AddInDeploymentState.cs
- IResourceProvider.cs
- TrackBar.cs
- PersistenceTask.cs
- Calendar.cs
- CatchDesigner.xaml.cs
- NativeRecognizer.cs
- EntityException.cs
- FileChangeNotifier.cs
- SQLInt16Storage.cs
- InstancePersistence.cs
- SqlExpander.cs
- NetworkCredential.cs
- SmtpAuthenticationManager.cs
- RectAnimationClockResource.cs
- ObjectDataSourceDisposingEventArgs.cs
- NodeCounter.cs
- WebServiceData.cs
- CompositeTypefaceMetrics.cs
- VerificationAttribute.cs
- FocusWithinProperty.cs
- FilterQuery.cs
- CompositeDataBoundControl.cs
- SizeAnimation.cs
- ListViewItemMouseHoverEvent.cs
- DynamicResourceExtensionConverter.cs
- HandleCollector.cs
- VariantWrapper.cs
- HitTestFilterBehavior.cs
- SqlCommand.cs
- FixedSOMPageConstructor.cs
- GridItem.cs
- Propagator.cs
- Privilege.cs
- MarshalDirectiveException.cs
- QuerySubExprEliminator.cs
- ResourceReferenceKeyNotFoundException.cs
- InProcStateClientManager.cs
- CodeAttributeArgument.cs
- UITypeEditor.cs
- DataFormat.cs
- HtmlInputText.cs
- IpcChannelHelper.cs
- ACL.cs
- AuthenticationModulesSection.cs
- PolicyChain.cs
- BroadcastEventHelper.cs
- StatusBarPanel.cs
- ObjectDataSource.cs
- DataGridViewEditingControlShowingEventArgs.cs
- PersonalizationStateQuery.cs
- ToolStripGrip.cs
- QueuedDeliveryRequirementsMode.cs
- PagePropertiesChangingEventArgs.cs
- ScrollItemPattern.cs
- WebPartHelpVerb.cs
- CodeVariableDeclarationStatement.cs
- BCryptHashAlgorithm.cs
- DecimalAnimationUsingKeyFrames.cs
- ProcessProtocolHandler.cs
- EmptyEnumerable.cs
- HtmlMobileTextWriter.cs
- StdValidatorsAndConverters.cs
- TextSelectionHelper.cs