Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / StatusBarPanel.cs / 1305376 / StatusBarPanel.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; using System.Globalization; using System.Runtime.Versioning; ////// /// [ ToolboxItem(false), DesignTimeVisible(false), DefaultProperty("Text") ] public class StatusBarPanel : Component, ISupportInitialize { private const int DEFAULTWIDTH = 100; private const int DEFAULTMINWIDTH = 10; private const int PANELTEXTINSET = 3; private const int PANELGAP = 2; private string text = ""; private string name = ""; private string toolTipText = ""; private Icon icon = null; private HorizontalAlignment alignment = HorizontalAlignment.Left; private System.Windows.Forms.StatusBarPanelBorderStyle borderStyle = System.Windows.Forms.StatusBarPanelBorderStyle.Sunken; private StatusBarPanelStyle style = StatusBarPanelStyle.Text; // these are package scope so the parent can get at them. // private StatusBar parent = null; private int width = DEFAULTWIDTH; private int right = 0; private int minWidth = DEFAULTMINWIDTH; private int index = 0; private StatusBarPanelAutoSize autoSize = StatusBarPanelAutoSize.None; private bool initializing = false; private object userData; ////// Stores the ////// control panel's information. /// /// /// public StatusBarPanel() { } ////// Initializes a new default instance of the ///class. /// /// /// [ SRCategory(SR.CatAppearance), DefaultValue(HorizontalAlignment.Left), Localizable(true), SRDescription(SR.StatusBarPanelAlignmentDescr) ] public HorizontalAlignment Alignment { get { return alignment; } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)HorizontalAlignment.Left, (int)HorizontalAlignment.Center)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(HorizontalAlignment)); } if (alignment != value) { alignment = value; Realize(); } } } ////// Gets or sets the ////// property. /// /// /// /// [ SRCategory(SR.CatAppearance), DefaultValue(StatusBarPanelAutoSize.None), RefreshProperties(RefreshProperties.All), SRDescription(SR.StatusBarPanelAutoSizeDescr) ] public StatusBarPanelAutoSize AutoSize { get { return this.autoSize; } set { //valid values are 0x1 to 0x3 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StatusBarPanelAutoSize.None, (int)StatusBarPanelAutoSize.Contents)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(StatusBarPanelAutoSize)); } if (this.autoSize != value) { this.autoSize = value; UpdateSize(); } } } ////// Gets or sets the ////// property. /// /// /// /// [ SRCategory(SR.CatAppearance), DefaultValue(System.Windows.Forms.StatusBarPanelBorderStyle.Sunken), DispId(NativeMethods.ActiveX.DISPID_BORDERSTYLE), SRDescription(SR.StatusBarPanelBorderStyleDescr) ] public StatusBarPanelBorderStyle BorderStyle { get { return borderStyle; } set { //valid values are 0x1 to 0x3 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StatusBarPanelBorderStyle.None, (int)StatusBarPanelBorderStyle.Sunken)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(StatusBarPanelBorderStyle)); } if (this.borderStyle != value) { this.borderStyle = value; Realize(); if (Created) this.parent.Invalidate(); } } } ////// Gets or sets the ////// /// property. /// /// /// /// ///internal bool Created { get { return this.parent != null && this.parent.ArePanelsRealized(); } } /// /// /// [ SRCategory(SR.CatAppearance), DefaultValue(null), Localizable(true), SRDescription(SR.StatusBarPanelIconDescr) ] public Icon Icon { [ResourceExposure(ResourceScope.Machine)] get { // unfortunately we have no way of getting the icon from the control. return this.icon; } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] set { if (value != null && (((Icon)value).Height > SystemInformation.SmallIconSize.Height || ((Icon)value).Width > SystemInformation.SmallIconSize.Width)) { this.icon = new Icon(value, SystemInformation.SmallIconSize); } else { this.icon = value; } if (Created) { IntPtr handle = (this.icon == null) ? IntPtr.Zero : this.icon.Handle; this.parent.SendMessage(NativeMethods.SB_SETICON, (IntPtr)GetIndex(), handle); } UpdateSize(); if (Created) { this.parent.Invalidate(); } } } ////// Gets or sets the ////// property. /// /// /// /// internal int Index { get { return index; } set { index = value; } } ////// Expose index internally /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(DEFAULTMINWIDTH), Localizable(true), RefreshProperties(RefreshProperties.All), SRDescription(SR.StatusBarPanelMinWidthDescr) ] public int MinWidth { get { return this.minWidth; } set { if (value < 0) { throw new ArgumentOutOfRangeException("MinWidth", SR.GetString(SR.InvalidLowBoundArgumentEx, "MinWidth", value.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } if (value != this.minWidth) { this.minWidth = value; UpdateSize(); if (this.minWidth > this.Width) { Width = value; } } } } ////// Gets or sets the minimum width the ///can be within the /// control. /// /// /// /// [ SRCategory(SR.CatAppearance), Localizable(true), SRDescription(SR.StatusBarPanelNameDescr) ] public string Name { get { return WindowsFormsUtils.GetComponentName(this, name); } set { name = value; if(Site!= null) { Site.Name = name; } } } ////// Gets or sets the name of the panel. /// ////// /// [Browsable(false)] public StatusBar Parent { get { return parent; } } ////// Represents the ////// control which hosts the /// panel. /// /// /// /// internal StatusBar ParentInternal { set { parent = value; } } ////// Expose a direct setter for parent internally /// ////// /// internal int Right { get { return right; } set { right = value; } } ////// Expose right internally /// ////// /// [ SRCategory(SR.CatAppearance), DefaultValue(StatusBarPanelStyle.Text), SRDescription(SR.StatusBarPanelStyleDescr) ] public StatusBarPanelStyle Style { get { return style;} set { //valid values are 0x1 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StatusBarPanelStyle.Text, (int)StatusBarPanelStyle.OwnerDraw)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(StatusBarPanelStyle)); } if (this.style != value) { this.style = value; Realize(); if (Created) { this.parent.Invalidate(); } } } } ////// Gets or sets the style of the panel. /// /// ///[ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// [ SRCategory(SR.CatAppearance), Localizable(true), DefaultValue(""), SRDescription(SR.StatusBarPanelTextDescr) ] public string Text { get { if (text == null) { return ""; } else { return text; } } set { if (value == null) { value = ""; } if (!Text.Equals(value)) { if (value.Length == 0) { this.text = null; } else { this.text = value; } Realize(); UpdateSize(); } } } ////// Gets or sets the text of the panel. /// ////// /// [ SRCategory(SR.CatAppearance), Localizable(true), DefaultValue(""), SRDescription(SR.StatusBarPanelToolTipTextDescr) ] public string ToolTipText { get { if (this.toolTipText == null) { return ""; } else { return this.toolTipText; } } set { if (value == null) { value = ""; } if (!ToolTipText.Equals(value)) { if (value.Length == 0) { this.toolTipText = null; } else { this.toolTipText = value; } if (Created) { parent.UpdateTooltip(this); } } } } ////// Gets /// or sets the panel's tool tip text. /// ////// /// [ Localizable(true), SRCategory(SR.CatAppearance), DefaultValue(DEFAULTWIDTH), SRDescription(SR.StatusBarPanelWidthDescr) ] public int Width { get { return this.width; } set { if (!initializing && value < this.minWidth) throw new ArgumentOutOfRangeException("Width", SR.GetString(SR.WidthGreaterThanMinWidth)); this.width = value; UpdateSize(); } } ////// Gets or sets the width of the ///within the /// control. /// /// /// /// Handles tasks required when the control is being initialized. /// public void BeginInit() { initializing = true; } ////// /// /// protected override void Dispose(bool disposing) { if (disposing) { if (parent != null) { int index = GetIndex(); if (index != -1) { parent.Panels.RemoveAt(index); } } } base.Dispose(disposing); } ////// /// Called when initialization of the control is complete. /// public void EndInit() { initializing = false; if (Width < MinWidth) { Width = MinWidth; } } ////// /// /// Gets the width of the contents of the panel /// internal int GetContentsWidth(bool newPanel) { string text; if (newPanel) { if (this.text == null) text = ""; else text = this.text; } else text = Text; Graphics g = this.parent.CreateGraphicsInternal(); Size sz = Size.Ceiling(g.MeasureString(text, parent.Font)); if (this.icon != null) { sz.Width += this.icon.Size.Width + 5; } g.Dispose(); int width = sz.Width + SystemInformation.BorderSize.Width*2 + PANELTEXTINSET*2 + PANELGAP; return Math.Max(width, minWidth); } ////// /// /// Returns the index of the panel by making the parent control search /// for it within its list. /// private int GetIndex() { return index; } ////// /// /// Sets all the properties for this panel. /// internal void Realize() { if (Created) { string text; string sendText; int border = 0; if (this.text == null) { text = ""; } else { text = this.text; } HorizontalAlignment align = alignment; // Translate the alignment for Rtl apps // if (parent.RightToLeft == RightToLeft.Yes) { switch (align) { case HorizontalAlignment.Left: align = HorizontalAlignment.Right; break; case HorizontalAlignment.Right: align = HorizontalAlignment.Left; break; } } switch (align) { case HorizontalAlignment.Center: sendText = "\t" + text; break; case HorizontalAlignment.Right: sendText = "\t\t" + text; break; default: sendText = text; break; } switch (borderStyle) { case StatusBarPanelBorderStyle.None: border |= NativeMethods.SBT_NOBORDERS; break; case StatusBarPanelBorderStyle.Sunken: break; case StatusBarPanelBorderStyle.Raised: border |= NativeMethods.SBT_POPOUT; break; } switch (style) { case StatusBarPanelStyle.Text: break; case StatusBarPanelStyle.OwnerDraw: border |= NativeMethods.SBT_OWNERDRAW; break; } int wparam = GetIndex() | border; if (parent.RightToLeft == RightToLeft.Yes) { wparam |= NativeMethods.SBT_RTLREADING; } int result = (int) UnsafeNativeMethods.SendMessage(new HandleRef(parent, parent.Handle), NativeMethods.SB_SETTEXT, (IntPtr)wparam, sendText); if (result == 0) throw new InvalidOperationException(SR.GetString(SR.UnableToSetPanelText)); if (this.icon != null && style != StatusBarPanelStyle.OwnerDraw) { this.parent.SendMessage(NativeMethods.SB_SETICON, (IntPtr)GetIndex(), this.icon.Handle); } else { this.parent.SendMessage(NativeMethods.SB_SETICON, (IntPtr)GetIndex(), IntPtr.Zero); } if (style == StatusBarPanelStyle.OwnerDraw) { NativeMethods.RECT rect = new NativeMethods.RECT(); result = (int) UnsafeNativeMethods.SendMessage(new HandleRef(parent, parent.Handle), NativeMethods.SB_GETRECT, (IntPtr)GetIndex(), ref rect); if (result != 0) { this.parent.Invalidate(Rectangle.FromLTRB(rect.left, rect.top, rect.right, rect.bottom)); } } } } private void UpdateSize() { if (this.autoSize == StatusBarPanelAutoSize.Contents) { ApplyContentSizing(); } else { if (Created) { parent.DirtyLayout(); parent.PerformLayout(); } } } private void ApplyContentSizing() { if (this.autoSize == StatusBarPanelAutoSize.Contents && parent != null) { int newWidth = GetContentsWidth(false); if (newWidth != this.Width) { this.Width = newWidth; if (Created) { parent.DirtyLayout(); parent.PerformLayout(); } } } } ////// /// public override string ToString() { return "StatusBarPanel: {" + Text + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Retrieves a string that contains information about the /// panel. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; using System.Globalization; using System.Runtime.Versioning; ////// /// [ ToolboxItem(false), DesignTimeVisible(false), DefaultProperty("Text") ] public class StatusBarPanel : Component, ISupportInitialize { private const int DEFAULTWIDTH = 100; private const int DEFAULTMINWIDTH = 10; private const int PANELTEXTINSET = 3; private const int PANELGAP = 2; private string text = ""; private string name = ""; private string toolTipText = ""; private Icon icon = null; private HorizontalAlignment alignment = HorizontalAlignment.Left; private System.Windows.Forms.StatusBarPanelBorderStyle borderStyle = System.Windows.Forms.StatusBarPanelBorderStyle.Sunken; private StatusBarPanelStyle style = StatusBarPanelStyle.Text; // these are package scope so the parent can get at them. // private StatusBar parent = null; private int width = DEFAULTWIDTH; private int right = 0; private int minWidth = DEFAULTMINWIDTH; private int index = 0; private StatusBarPanelAutoSize autoSize = StatusBarPanelAutoSize.None; private bool initializing = false; private object userData; ////// Stores the ////// control panel's information. /// /// /// public StatusBarPanel() { } ////// Initializes a new default instance of the ///class. /// /// /// [ SRCategory(SR.CatAppearance), DefaultValue(HorizontalAlignment.Left), Localizable(true), SRDescription(SR.StatusBarPanelAlignmentDescr) ] public HorizontalAlignment Alignment { get { return alignment; } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)HorizontalAlignment.Left, (int)HorizontalAlignment.Center)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(HorizontalAlignment)); } if (alignment != value) { alignment = value; Realize(); } } } ////// Gets or sets the ////// property. /// /// /// /// [ SRCategory(SR.CatAppearance), DefaultValue(StatusBarPanelAutoSize.None), RefreshProperties(RefreshProperties.All), SRDescription(SR.StatusBarPanelAutoSizeDescr) ] public StatusBarPanelAutoSize AutoSize { get { return this.autoSize; } set { //valid values are 0x1 to 0x3 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StatusBarPanelAutoSize.None, (int)StatusBarPanelAutoSize.Contents)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(StatusBarPanelAutoSize)); } if (this.autoSize != value) { this.autoSize = value; UpdateSize(); } } } ////// Gets or sets the ////// property. /// /// /// /// [ SRCategory(SR.CatAppearance), DefaultValue(System.Windows.Forms.StatusBarPanelBorderStyle.Sunken), DispId(NativeMethods.ActiveX.DISPID_BORDERSTYLE), SRDescription(SR.StatusBarPanelBorderStyleDescr) ] public StatusBarPanelBorderStyle BorderStyle { get { return borderStyle; } set { //valid values are 0x1 to 0x3 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StatusBarPanelBorderStyle.None, (int)StatusBarPanelBorderStyle.Sunken)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(StatusBarPanelBorderStyle)); } if (this.borderStyle != value) { this.borderStyle = value; Realize(); if (Created) this.parent.Invalidate(); } } } ////// Gets or sets the ////// /// property. /// /// /// /// ///internal bool Created { get { return this.parent != null && this.parent.ArePanelsRealized(); } } /// /// /// [ SRCategory(SR.CatAppearance), DefaultValue(null), Localizable(true), SRDescription(SR.StatusBarPanelIconDescr) ] public Icon Icon { [ResourceExposure(ResourceScope.Machine)] get { // unfortunately we have no way of getting the icon from the control. return this.icon; } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] set { if (value != null && (((Icon)value).Height > SystemInformation.SmallIconSize.Height || ((Icon)value).Width > SystemInformation.SmallIconSize.Width)) { this.icon = new Icon(value, SystemInformation.SmallIconSize); } else { this.icon = value; } if (Created) { IntPtr handle = (this.icon == null) ? IntPtr.Zero : this.icon.Handle; this.parent.SendMessage(NativeMethods.SB_SETICON, (IntPtr)GetIndex(), handle); } UpdateSize(); if (Created) { this.parent.Invalidate(); } } } ////// Gets or sets the ////// property. /// /// /// /// internal int Index { get { return index; } set { index = value; } } ////// Expose index internally /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(DEFAULTMINWIDTH), Localizable(true), RefreshProperties(RefreshProperties.All), SRDescription(SR.StatusBarPanelMinWidthDescr) ] public int MinWidth { get { return this.minWidth; } set { if (value < 0) { throw new ArgumentOutOfRangeException("MinWidth", SR.GetString(SR.InvalidLowBoundArgumentEx, "MinWidth", value.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } if (value != this.minWidth) { this.minWidth = value; UpdateSize(); if (this.minWidth > this.Width) { Width = value; } } } } ////// Gets or sets the minimum width the ///can be within the /// control. /// /// /// /// [ SRCategory(SR.CatAppearance), Localizable(true), SRDescription(SR.StatusBarPanelNameDescr) ] public string Name { get { return WindowsFormsUtils.GetComponentName(this, name); } set { name = value; if(Site!= null) { Site.Name = name; } } } ////// Gets or sets the name of the panel. /// ////// /// [Browsable(false)] public StatusBar Parent { get { return parent; } } ////// Represents the ////// control which hosts the /// panel. /// /// /// /// internal StatusBar ParentInternal { set { parent = value; } } ////// Expose a direct setter for parent internally /// ////// /// internal int Right { get { return right; } set { right = value; } } ////// Expose right internally /// ////// /// [ SRCategory(SR.CatAppearance), DefaultValue(StatusBarPanelStyle.Text), SRDescription(SR.StatusBarPanelStyleDescr) ] public StatusBarPanelStyle Style { get { return style;} set { //valid values are 0x1 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StatusBarPanelStyle.Text, (int)StatusBarPanelStyle.OwnerDraw)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(StatusBarPanelStyle)); } if (this.style != value) { this.style = value; Realize(); if (Created) { this.parent.Invalidate(); } } } } ////// Gets or sets the style of the panel. /// /// ///[ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// [ SRCategory(SR.CatAppearance), Localizable(true), DefaultValue(""), SRDescription(SR.StatusBarPanelTextDescr) ] public string Text { get { if (text == null) { return ""; } else { return text; } } set { if (value == null) { value = ""; } if (!Text.Equals(value)) { if (value.Length == 0) { this.text = null; } else { this.text = value; } Realize(); UpdateSize(); } } } ////// Gets or sets the text of the panel. /// ////// /// [ SRCategory(SR.CatAppearance), Localizable(true), DefaultValue(""), SRDescription(SR.StatusBarPanelToolTipTextDescr) ] public string ToolTipText { get { if (this.toolTipText == null) { return ""; } else { return this.toolTipText; } } set { if (value == null) { value = ""; } if (!ToolTipText.Equals(value)) { if (value.Length == 0) { this.toolTipText = null; } else { this.toolTipText = value; } if (Created) { parent.UpdateTooltip(this); } } } } ////// Gets /// or sets the panel's tool tip text. /// ////// /// [ Localizable(true), SRCategory(SR.CatAppearance), DefaultValue(DEFAULTWIDTH), SRDescription(SR.StatusBarPanelWidthDescr) ] public int Width { get { return this.width; } set { if (!initializing && value < this.minWidth) throw new ArgumentOutOfRangeException("Width", SR.GetString(SR.WidthGreaterThanMinWidth)); this.width = value; UpdateSize(); } } ////// Gets or sets the width of the ///within the /// control. /// /// /// /// Handles tasks required when the control is being initialized. /// public void BeginInit() { initializing = true; } ////// /// /// protected override void Dispose(bool disposing) { if (disposing) { if (parent != null) { int index = GetIndex(); if (index != -1) { parent.Panels.RemoveAt(index); } } } base.Dispose(disposing); } ////// /// Called when initialization of the control is complete. /// public void EndInit() { initializing = false; if (Width < MinWidth) { Width = MinWidth; } } ////// /// /// Gets the width of the contents of the panel /// internal int GetContentsWidth(bool newPanel) { string text; if (newPanel) { if (this.text == null) text = ""; else text = this.text; } else text = Text; Graphics g = this.parent.CreateGraphicsInternal(); Size sz = Size.Ceiling(g.MeasureString(text, parent.Font)); if (this.icon != null) { sz.Width += this.icon.Size.Width + 5; } g.Dispose(); int width = sz.Width + SystemInformation.BorderSize.Width*2 + PANELTEXTINSET*2 + PANELGAP; return Math.Max(width, minWidth); } ////// /// /// Returns the index of the panel by making the parent control search /// for it within its list. /// private int GetIndex() { return index; } ////// /// /// Sets all the properties for this panel. /// internal void Realize() { if (Created) { string text; string sendText; int border = 0; if (this.text == null) { text = ""; } else { text = this.text; } HorizontalAlignment align = alignment; // Translate the alignment for Rtl apps // if (parent.RightToLeft == RightToLeft.Yes) { switch (align) { case HorizontalAlignment.Left: align = HorizontalAlignment.Right; break; case HorizontalAlignment.Right: align = HorizontalAlignment.Left; break; } } switch (align) { case HorizontalAlignment.Center: sendText = "\t" + text; break; case HorizontalAlignment.Right: sendText = "\t\t" + text; break; default: sendText = text; break; } switch (borderStyle) { case StatusBarPanelBorderStyle.None: border |= NativeMethods.SBT_NOBORDERS; break; case StatusBarPanelBorderStyle.Sunken: break; case StatusBarPanelBorderStyle.Raised: border |= NativeMethods.SBT_POPOUT; break; } switch (style) { case StatusBarPanelStyle.Text: break; case StatusBarPanelStyle.OwnerDraw: border |= NativeMethods.SBT_OWNERDRAW; break; } int wparam = GetIndex() | border; if (parent.RightToLeft == RightToLeft.Yes) { wparam |= NativeMethods.SBT_RTLREADING; } int result = (int) UnsafeNativeMethods.SendMessage(new HandleRef(parent, parent.Handle), NativeMethods.SB_SETTEXT, (IntPtr)wparam, sendText); if (result == 0) throw new InvalidOperationException(SR.GetString(SR.UnableToSetPanelText)); if (this.icon != null && style != StatusBarPanelStyle.OwnerDraw) { this.parent.SendMessage(NativeMethods.SB_SETICON, (IntPtr)GetIndex(), this.icon.Handle); } else { this.parent.SendMessage(NativeMethods.SB_SETICON, (IntPtr)GetIndex(), IntPtr.Zero); } if (style == StatusBarPanelStyle.OwnerDraw) { NativeMethods.RECT rect = new NativeMethods.RECT(); result = (int) UnsafeNativeMethods.SendMessage(new HandleRef(parent, parent.Handle), NativeMethods.SB_GETRECT, (IntPtr)GetIndex(), ref rect); if (result != 0) { this.parent.Invalidate(Rectangle.FromLTRB(rect.left, rect.top, rect.right, rect.bottom)); } } } } private void UpdateSize() { if (this.autoSize == StatusBarPanelAutoSize.Contents) { ApplyContentSizing(); } else { if (Created) { parent.DirtyLayout(); parent.PerformLayout(); } } } private void ApplyContentSizing() { if (this.autoSize == StatusBarPanelAutoSize.Contents && parent != null) { int newWidth = GetContentsWidth(false); if (newWidth != this.Width) { this.Width = newWidth; if (Created) { parent.DirtyLayout(); parent.PerformLayout(); } } } } ////// /// public override string ToString() { return "StatusBarPanel: {" + Text + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Retrieves a string that contains information about the /// panel. /// ///
Link Menu
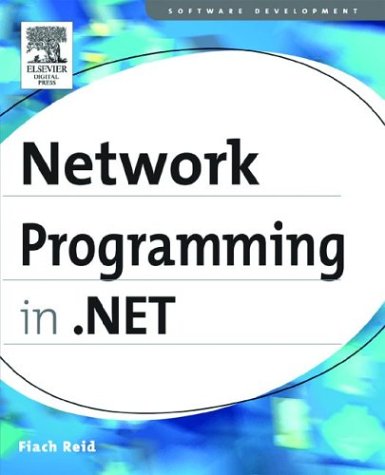
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmbeddedMailObjectsCollection.cs
- BackgroundWorker.cs
- LayoutUtils.cs
- TextContainerHelper.cs
- RuleRef.cs
- Int32Rect.cs
- ArgIterator.cs
- ThreadPool.cs
- Attachment.cs
- HttpWebRequest.cs
- _TransmitFileOverlappedAsyncResult.cs
- PathGradientBrush.cs
- ListViewHitTestInfo.cs
- DataBindingExpressionBuilder.cs
- ConfigurationLoader.cs
- UTF7Encoding.cs
- DatePicker.cs
- CookieProtection.cs
- WizardStepCollectionEditor.cs
- ConfigurationSectionCollection.cs
- HistoryEventArgs.cs
- Animatable.cs
- SoapFormatterSinks.cs
- DataPagerField.cs
- OleDbEnumerator.cs
- Boolean.cs
- ObjectParameterCollection.cs
- ProtocolsConfiguration.cs
- MetadataException.cs
- DatatypeImplementation.cs
- DataTrigger.cs
- DependentList.cs
- SqlBulkCopy.cs
- LayoutManager.cs
- SafeRightsManagementQueryHandle.cs
- TypeConverterHelper.cs
- TextBlock.cs
- EntityKey.cs
- DesignTableCollection.cs
- UseLicense.cs
- ObjectCloneHelper.cs
- SelectionGlyph.cs
- ActivationServices.cs
- ServiceObjectContainer.cs
- Cast.cs
- BitConverter.cs
- CodeStatementCollection.cs
- PointKeyFrameCollection.cs
- HtmlElement.cs
- EventToken.cs
- GenericUriParser.cs
- PassportPrincipal.cs
- HttpClientCertificate.cs
- BrowserCapabilitiesCodeGenerator.cs
- ConnectionString.cs
- CategoryGridEntry.cs
- CompilerError.cs
- UdpMessageProperty.cs
- StorageRoot.cs
- mactripleDES.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- PropertyFilterAttribute.cs
- x509utils.cs
- MetadataArtifactLoaderFile.cs
- CalendarButton.cs
- FontCollection.cs
- RegexTree.cs
- SpinLock.cs
- TextRunCacheImp.cs
- GeometryHitTestParameters.cs
- PublisherIdentityPermission.cs
- ProfilePropertyNameValidator.cs
- ListItemCollection.cs
- AsyncPostBackTrigger.cs
- HtmlForm.cs
- VisualTarget.cs
- BitmapPalettes.cs
- ListBoxItemAutomationPeer.cs
- GeneralTransform3D.cs
- ByeOperation11AsyncResult.cs
- SimpleTypesSurrogate.cs
- KeyBinding.cs
- HostingEnvironmentException.cs
- DesignerPerfEventProvider.cs
- EntryIndex.cs
- WindowsHyperlink.cs
- DATA_BLOB.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- SoapFormatter.cs
- BindingExpressionUncommonField.cs
- XmlSchemaComplexContentRestriction.cs
- DataControlImageButton.cs
- CompilerScopeManager.cs
- HelloMessage11.cs
- CmsInterop.cs
- RadioButtonBaseAdapter.cs
- DeadCharTextComposition.cs
- TemplatedControlDesigner.cs
- SessionIDManager.cs
- OutputScope.cs