Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / DesignerPerfEventProvider.cs / 1305376 / DesignerPerfEventProvider.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System; using System.Collections.Generic; using System.Text; using System.Diagnostics.Eventing; class DesignerPerfEventProvider { EventProvider provider = null; public DesignerPerfEventProvider() { try { this.provider = new EventProvider(new Guid("{B5697126-CBAF-4281-A983-7851DAF56454}")); } catch (PlatformNotSupportedException) { this.provider = null; } } public void WorkflowDesignerApplicationIdleAfterLoad() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerApplicationIdleAfterLoad); } } public void WorkflowDesignerDeserializeEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerDeserializeEnd); } } public void WorkflowDesignerDeserializeStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerDeserializeStart); } } public void WorkflowDesignerDrop() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerDrop); } } public void WorkflowDesignerIdleAfterDrop() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerIdleAfterDrop); } } public void WorkflowDesignerLoadComplete() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerLoadComplete); } } public void WorkflowDesignerLoadStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerLoadStart); } } public void WorkflowDesignerSerializeEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerSerializeEnd); } } public void WorkflowDesignerSerializeStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerSerializeStart); } } public void WorkflowDesignerExpressionEditorCompilationEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerExpressionEditorCompilationEnd); } } public void WorkflowDesignerExpressionEditorCompilationStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerExpressionEditorCompilationStart); } } public void WorkflowDesignerExpressionEditorLoaded() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerExpressionEditorLoaded); } } public void WorkflowDesignerExpressionEditorLoadStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerExpressionEditorLoadStart); } } public void WorkflowDesignerValidationEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerValidationEnd); } } public void WorkflowDesignerValidationStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerValidationStart); } } public void FlowchartDesignerLoadEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.FlowchartDesignerLoadEnd); } } public void FlowchartDesignerLoadStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.FlowchartDesignerLoadStart); } } public void FreeFormPanelMeasureEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.FreeFormPanelMeasureEnd); } } public void FreeFormPanelMeasureStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.FreeFormPanelMeasureStart); } } private bool IsEnabled() { bool isEnabled = false; if (this.provider != null) { isEnabled = this.provider.IsEnabled(); } return isEnabled; } private void WriteEventHelper(int eventId) { if (this.provider != null) { EventDescriptor descriptor = new EventDescriptor(eventId, 0, 0, 0, 0, 0, 0); this.provider.WriteEvent(ref descriptor); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System; using System.Collections.Generic; using System.Text; using System.Diagnostics.Eventing; class DesignerPerfEventProvider { EventProvider provider = null; public DesignerPerfEventProvider() { try { this.provider = new EventProvider(new Guid("{B5697126-CBAF-4281-A983-7851DAF56454}")); } catch (PlatformNotSupportedException) { this.provider = null; } } public void WorkflowDesignerApplicationIdleAfterLoad() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerApplicationIdleAfterLoad); } } public void WorkflowDesignerDeserializeEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerDeserializeEnd); } } public void WorkflowDesignerDeserializeStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerDeserializeStart); } } public void WorkflowDesignerDrop() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerDrop); } } public void WorkflowDesignerIdleAfterDrop() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerIdleAfterDrop); } } public void WorkflowDesignerLoadComplete() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerLoadComplete); } } public void WorkflowDesignerLoadStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerLoadStart); } } public void WorkflowDesignerSerializeEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerSerializeEnd); } } public void WorkflowDesignerSerializeStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerSerializeStart); } } public void WorkflowDesignerExpressionEditorCompilationEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerExpressionEditorCompilationEnd); } } public void WorkflowDesignerExpressionEditorCompilationStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerExpressionEditorCompilationStart); } } public void WorkflowDesignerExpressionEditorLoaded() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerExpressionEditorLoaded); } } public void WorkflowDesignerExpressionEditorLoadStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerExpressionEditorLoadStart); } } public void WorkflowDesignerValidationEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerValidationEnd); } } public void WorkflowDesignerValidationStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.WorkflowDesignerValidationStart); } } public void FlowchartDesignerLoadEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.FlowchartDesignerLoadEnd); } } public void FlowchartDesignerLoadStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.FlowchartDesignerLoadStart); } } public void FreeFormPanelMeasureEnd() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.FreeFormPanelMeasureEnd); } } public void FreeFormPanelMeasureStart() { if (this.IsEnabled()) { WriteEventHelper((int)DesignerPerfEvents.FreeFormPanelMeasureStart); } } private bool IsEnabled() { bool isEnabled = false; if (this.provider != null) { isEnabled = this.provider.IsEnabled(); } return isEnabled; } private void WriteEventHelper(int eventId) { if (this.provider != null) { EventDescriptor descriptor = new EventDescriptor(eventId, 0, 0, 0, 0, 0, 0); this.provider.WriteEvent(ref descriptor); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
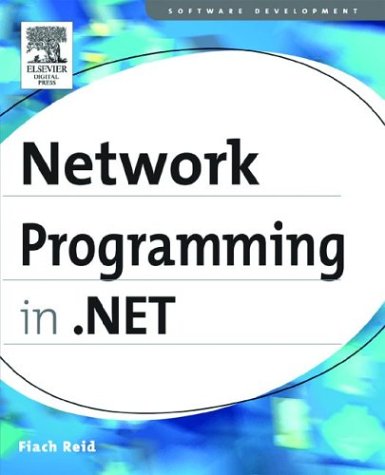
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AlphaSortedEnumConverter.cs
- ToolStripDropDownClosingEventArgs.cs
- SortedList.cs
- AccessDataSource.cs
- ItemDragEvent.cs
- CachedResourceDictionaryExtension.cs
- SamlAuthorizationDecisionStatement.cs
- TimeoutValidationAttribute.cs
- RenderDataDrawingContext.cs
- SHA512.cs
- _Semaphore.cs
- HashAlgorithm.cs
- BidPrivateBase.cs
- BamlResourceSerializer.cs
- GeometryHitTestResult.cs
- PropertyOrder.cs
- WindowsStatic.cs
- UpdateCommand.cs
- StorageMappingItemLoader.cs
- Drawing.cs
- ProgressiveCrcCalculatingStream.cs
- brushes.cs
- SpellerError.cs
- UriScheme.cs
- SqlSupersetValidator.cs
- TextDecorationCollection.cs
- TypedTableBaseExtensions.cs
- DataControlPagerLinkButton.cs
- RelationalExpressions.cs
- Utility.cs
- PixelFormat.cs
- LicenseContext.cs
- JsonFormatReaderGenerator.cs
- EncryptedData.cs
- DocumentReferenceCollection.cs
- Compiler.cs
- GridViewSortEventArgs.cs
- ContentPathSegment.cs
- AdvancedBindingPropertyDescriptor.cs
- HebrewNumber.cs
- xdrvalidator.cs
- Overlapped.cs
- RuleInfoComparer.cs
- DesignerExtenders.cs
- Condition.cs
- InternalPermissions.cs
- TextBlock.cs
- StorageAssociationTypeMapping.cs
- AlternateView.cs
- SpellCheck.cs
- ProviderCollection.cs
- XmlWhitespace.cs
- SoapSchemaImporter.cs
- AnnotationHighlightLayer.cs
- RegistrySecurity.cs
- ToolStripComboBox.cs
- ObjectListCommandCollection.cs
- DataGridState.cs
- SqlLiftIndependentRowExpressions.cs
- CodeMemberMethod.cs
- BuildTopDownAttribute.cs
- StateDesigner.Helpers.cs
- WrappedIUnknown.cs
- HttpHandlersSection.cs
- Translator.cs
- RtfToXamlLexer.cs
- MexNamedPipeBindingElement.cs
- JsonQueryStringConverter.cs
- SHA1.cs
- XmlSerializerVersionAttribute.cs
- SamlSecurityTokenAuthenticator.cs
- StringAnimationUsingKeyFrames.cs
- GridViewColumnCollectionChangedEventArgs.cs
- FatalException.cs
- WebPartUtil.cs
- ZipIOModeEnforcingStream.cs
- DiscoveryVersionConverter.cs
- NameObjectCollectionBase.cs
- DataGridViewHeaderCell.cs
- StoryFragments.cs
- XmlSerializationReader.cs
- UIInitializationException.cs
- basenumberconverter.cs
- ListViewSortEventArgs.cs
- RuleCache.cs
- RegexWorker.cs
- SystemUdpStatistics.cs
- IDataContractSurrogate.cs
- CfgSemanticTag.cs
- Point4D.cs
- WebControlAdapter.cs
- PrintPageEvent.cs
- NonBatchDirectoryCompiler.cs
- SmtpCommands.cs
- DrawingDrawingContext.cs
- PeoplePickerWrapper.cs
- CallTemplateAction.cs
- WebHttpBindingCollectionElement.cs
- MatrixIndependentAnimationStorage.cs
- RadialGradientBrush.cs