Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Imaging / PropVariant.cs / 1 / PropVariant.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved // // File: PropVariant.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.Windows.Media.Imaging; using System.Windows.Media.Composition; using System.Text; using MS.Internal.PresentationCore; using System.Diagnostics.CodeAnalysis; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings // // This class wraps a PROPVARIANT type for interop with the unmanaged metadata APIs. Only // the capabilities used by this API are supported (so for example, there is no SAFEARRAY, IDispatch, // general VT_UNKNOWN support, etc.) // // The types are mapped to C# types as follows: // // byte <=> VT_UI1 // sbyte <=> VT_I1 // char <=> VT_LPSTR (size 1) // ushort <=> VT_UI2 // short <=> VT_I2 // String <=> VT_LPWSTR // uint <=> VT_UI4 // int <=> VT_I4 // UInt64 <=> VT_UI8 // Int64 <=> VT_I8 // float <=> VT_R4 // double <=> VT_R8 // Guid <=> VT_CLSID // bool <=> VT_BOOL // BitmapMetadata <=> VT_UNKNOWN (IWICMetadataQueryReader) // BitmapMetadataBlob <=> VT_BLOB // // For array types: // // byte[] <=> VT_UI1|VT_VECTOR // sbyte[] <=> VT_I1|VT_VECTOR // char[] <=> VT_LPSTR (size is length of array - treated as ASCII string) - read back is String, use ToCharArray(). // char[][] <=> VT_LPSTR|VT_VECTOR (array of ASCII strings) // ushort[] <=> VT_UI2|VT_VECTOR // short[] <=> VT_I2|VT_VECTOR // String[] <=> VT_LPWSTR|VT_VECTOR // uint[] <=> VT_UI4|VT_VECTOR // int[] <=> VT_I4|VT_VECTOR // UInt64[] <=> VT_UI8|VT_VECTOR // Int64[] <=> VT_I8|VT_VECTOR // float[] <=> VT_R4|VT_VECTOR // double[] <=> VT_R8|VT_VECTOR // Guid[] <=> VT_CLSID|VT_VECTOR // bool[] <=> VT_BOOL|VT_VECTOR // namespace System.Windows.Media.Imaging { #region PropVariant [StructLayout(LayoutKind.Sequential, Pack=0)] internal struct PROPARRAY { internal UInt32 cElems; internal IntPtr pElems; } [StructLayout(LayoutKind.Explicit, Pack=1)] internal struct PROPVARIANT { [FieldOffset(0)] internal ushort varType; [FieldOffset(2)] internal ushort wReserved1; [FieldOffset(4)] internal ushort wReserved2; [FieldOffset(6)] internal ushort wReserved3; [FieldOffset(8)] internal byte bVal; [FieldOffset(8)] internal sbyte cVal; [FieldOffset(8)] internal ushort uiVal; [FieldOffset(8)] internal short iVal; [FieldOffset(8)] internal UInt32 uintVal; [FieldOffset(8)] internal Int32 intVal; [FieldOffset(8)] internal UInt64 ulVal; [FieldOffset(8)] internal Int64 lVal; [FieldOffset(8)] internal float fltVal; [FieldOffset(8)] internal double dblVal; [FieldOffset(8)] internal short boolVal; [FieldOffset(8)] internal IntPtr pclsidVal; //this is for GUID ID pointer [FieldOffset(8)] internal IntPtr pszVal; //this is for ansi string pointer [FieldOffset(8)] internal IntPtr pwszVal; //this is for Unicode string pointer [FieldOffset(8)] internal IntPtr punkVal; //this is for punkVal (interface pointer) [FieldOffset(8)] internal PROPARRAY ca; [FieldOffset(8)] internal System.Runtime.InteropServices.ComTypes.FILETIME filetime; ////// CopyBytes - Poor man's mem copy. Copies cbData from pbFrom to pbTo. /// /// byte* pointing to the "to" array. /// int - count of bytes of receiving buffer. /// byte* pointing to the "from" array. /// int - count of bytes to copy from buffer. ////// Critical - Accesses unmanaged memory for copying /// [SecurityCritical] private static unsafe void CopyBytes( byte* pbTo, int cbTo, byte* pbFrom, int cbFrom ) { if (cbFrom>cbTo) { throw new InvalidOperationException(SR.Get(SRID.Image_InsufficientBufferSize)); } byte* pCurFrom = (byte*)pbFrom; byte* pCurTo = (byte*)pbTo; for (int i = 0; i < cbFrom; i++) { pCurTo[i] = pCurFrom[i]; } } ////// Critical -Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] internal void InitVector(Array array, Type type, VarEnum varEnum) { Init(array, type, varEnum | VarEnum.VT_VECTOR); } ////// Critical -Accesses unmanaged code and structure is overlapping in memory /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] internal void Init(Array array, Type type, VarEnum vt) { varType = (ushort) vt; ca.cElems = 0; ca.pElems = IntPtr.Zero; int length = array.Length; if (length > 0) { long size = Marshal.SizeOf(type) * length; IntPtr destPtr =IntPtr.Zero; GCHandle handle = new GCHandle(); try { destPtr = Marshal.AllocCoTaskMem((int) size); handle = GCHandle.Alloc(array, GCHandleType.Pinned); unsafe { CopyBytes((byte *) destPtr, (int)size, (byte *)handle.AddrOfPinnedObject(), (int)size); } ca.cElems = (uint)length; ca.pElems = destPtr; destPtr = IntPtr.Zero; } finally { if (handle.IsAllocated) { handle.Free(); } if (destPtr != IntPtr.Zero) { Marshal.FreeCoTaskMem(destPtr); } } } } ////// Critical -Accesses unmanaged code and structure is overlapping in memory /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] internal void Init(String[] value, bool fAscii) { varType = (ushort) (fAscii ? VarEnum.VT_LPSTR : VarEnum.VT_LPWSTR); varType |= (ushort) VarEnum.VT_VECTOR; ca.cElems = 0; ca.pElems = IntPtr.Zero; int length = value.Length; if (length > 0) { IntPtr destPtr = IntPtr.Zero; int sizeIntPtr = 0; unsafe { sizeIntPtr = sizeof(IntPtr); } long size = sizeIntPtr * length; int index = 0; try { IntPtr pString = IntPtr.Zero; destPtr = Marshal.AllocCoTaskMem((int)size); for (index=0; index/// Critical -Accesses unmanaged code and structure is overlapping in memory /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] internal void Init(object value) { if (value == null) { varType = (ushort)VarEnum.VT_EMPTY; } else if (value is Array) { Type type = value.GetType(); if (type == typeof(sbyte[])) { InitVector(value as Array, typeof(sbyte), VarEnum.VT_I1); } else if (type == typeof(byte[])) { InitVector(value as Array, typeof(byte), VarEnum.VT_UI1); } else if (value is char[]) { varType = (ushort) VarEnum.VT_LPSTR; pszVal = Marshal.StringToCoTaskMemAnsi(new String(value as char[])); } else if (value is char[][]) { char[][] charArray = value as char[][]; String[] strArray = new String[charArray.GetLength(0)]; for (int i=0; i /// Critical -Accesses unmanaged code and structure is overlapping in memory /// TreatAsSafe - there are no inputs /// [SecurityCritical, SecurityTreatAsSafe] internal void Clear() { VarEnum vt = (VarEnum) varType; if ((vt & VarEnum.VT_VECTOR) != 0 || vt == VarEnum.VT_BLOB) { if (ca.pElems != IntPtr.Zero) { vt = vt & ~VarEnum.VT_VECTOR; if (vt == VarEnum.VT_UNKNOWN) { IntPtr punkPtr = ca.pElems; int sizeIntPtr = 0; unsafe { sizeIntPtr = sizeof(IntPtr); } for (uint i=0; i /// Critical -Accesses unmanaged code and structure is overlapping in memory /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] internal object ToObject(object syncObject) { VarEnum vt = (VarEnum) varType; if ((vt & VarEnum.VT_VECTOR) != 0) { switch (vt & (~VarEnum.VT_VECTOR)) { case VarEnum.VT_EMPTY: return null; case VarEnum.VT_I1: { sbyte[] array = new sbyte[ca.cElems]; for (int i=0; i /// Critical - Accesses a structure that contains overlapping data /// TreatAsSafe - there are no inputs /// internal bool RequiresSyncObject { [SecurityCritical, SecurityTreatAsSafe] get { return (varType == (ushort) VarEnum.VT_UNKNOWN); } } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
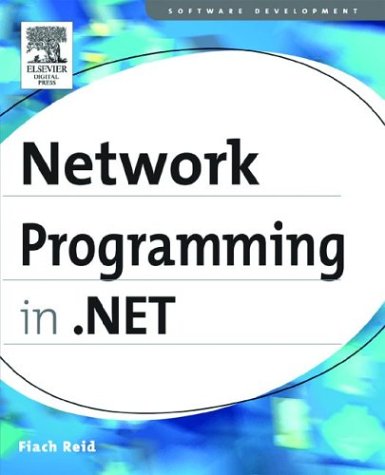
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyDescriptorGridEntry.cs
- StorageModelBuildProvider.cs
- ExtensibleClassFactory.cs
- FloatSumAggregationOperator.cs
- AssertFilter.cs
- DriveInfo.cs
- ProtocolsConfigurationEntry.cs
- ExpressionsCollectionEditor.cs
- ArrayWithOffset.cs
- Collection.cs
- RuleRefElement.cs
- EmptyStringExpandableObjectConverter.cs
- WsdlBuildProvider.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- HandlerBase.cs
- ParallelDesigner.cs
- DynamicMethod.cs
- FormViewPageEventArgs.cs
- StickyNoteContentControl.cs
- CDSsyncETWBCLProvider.cs
- MimeMapping.cs
- Single.cs
- Util.cs
- IndividualDeviceConfig.cs
- SmtpMail.cs
- MsmqIntegrationChannelFactory.cs
- DependencyStoreSurrogate.cs
- PkcsMisc.cs
- EnumerableCollectionView.cs
- CleanUpVirtualizedItemEventArgs.cs
- ReadOnlyHierarchicalDataSource.cs
- ClickablePoint.cs
- EpmTargetTree.cs
- RSAOAEPKeyExchangeFormatter.cs
- TableCell.cs
- WindowsFont.cs
- ProfilePropertyMetadata.cs
- ManifestResourceInfo.cs
- HtmlInputRadioButton.cs
- XmlSchemaAnyAttribute.cs
- ColorBlend.cs
- Tablet.cs
- SecurityKeyIdentifierClause.cs
- InkSerializer.cs
- XmlNode.cs
- CustomErrorsSection.cs
- MediaEntryAttribute.cs
- StateWorkerRequest.cs
- ExceptionUtil.cs
- SequentialUshortCollection.cs
- PlainXmlDeserializer.cs
- StatusBar.cs
- BaseUriHelper.cs
- EnterpriseServicesHelper.cs
- X509Certificate2Collection.cs
- RealizationDrawingContextWalker.cs
- FixedSOMPage.cs
- ErrorHandler.cs
- TypeBuilder.cs
- ServiceActivationException.cs
- HttpDictionary.cs
- SqlTriggerContext.cs
- WorkflowPrinting.cs
- ImageDrawing.cs
- SettingsSavedEventArgs.cs
- WinHttpWebProxyFinder.cs
- ETagAttribute.cs
- DefaultObjectMappingItemCollection.cs
- MatrixUtil.cs
- InputLanguage.cs
- DrawingCollection.cs
- SelectorItemAutomationPeer.cs
- HScrollBar.cs
- BaseCodeDomTreeGenerator.cs
- RelativeSource.cs
- ProxyFragment.cs
- AppDomainAttributes.cs
- CheckBoxStandardAdapter.cs
- UserPersonalizationStateInfo.cs
- ISO2022Encoding.cs
- ClientType.cs
- SortQueryOperator.cs
- ObjectAnimationUsingKeyFrames.cs
- ToolBarButton.cs
- WindowsPrincipal.cs
- TemplateBindingExpressionConverter.cs
- SystemUnicastIPAddressInformation.cs
- DoubleLinkList.cs
- SchemaTypeEmitter.cs
- PeerConnector.cs
- WebPartConnectionsCloseVerb.cs
- StringTraceRecord.cs
- HtmlHead.cs
- RequestCachingSection.cs
- CodeEntryPointMethod.cs
- ChtmlTextWriter.cs
- Point3DConverter.cs
- StateFinalizationDesigner.cs
- DefaultValueAttribute.cs
- ImageField.cs