Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Animation / TimelineGroup.cs / 1 / TimelineGroup.cs
//---------------------------------------------------------------------------- //// Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// This class represents base class for Timelines that have functionality /// related to having Children. /// [ContentProperty("Children")] public abstract partial class TimelineGroup : Timeline, IAddChild { #region Constructors ////// Creates a TimelineGroup with default properties. /// protected TimelineGroup() : base() { } ////// Creates a TimelineGroup with the specified BeginTime. /// /// /// The scheduled BeginTime for this TimelineGroup. /// protected TimelineGroup(NullablebeginTime) : base(beginTime) { } /// /// Creates a TimelineGroup with the specified begin time and duration. /// /// /// The scheduled BeginTime for this TimelineGroup. /// /// /// The simple Duration of this TimelineGroup. /// protected TimelineGroup(NullablebeginTime, Duration duration) : base(beginTime, duration) { } /// /// Creates a TimelineGroup with the specified BeginTime, Duration and RepeatBehavior. /// /// /// The scheduled BeginTime for this TimelineGroup. /// /// /// The simple Duration of this TimelineGroup. /// /// /// The RepeatBehavior for this TimelineGroup. /// protected TimelineGroup(NullablebeginTime, Duration duration, RepeatBehavior repeatBehavior) : base(beginTime, duration, repeatBehavior) { } #endregion #region Timeline /// /// /// ///protected internal override Clock AllocateClock() { return new ClockGroup(this); } /// /// Creates a new ClockGroup using this TimelineGroup. /// ///A new ClockGroup. new public ClockGroup CreateClock() { return (ClockGroup)base.CreateClock(); } #endregion #region IAddChild interface ////// Adds a child object to this TimelineGroup. /// /// /// The child object to add. /// ////// A Timeline only accepts another Timeline (or derived class) as /// a child. /// void IAddChild.AddChild(object child) { WritePreamble(); if (child == null) { throw new ArgumentNullException("child"); } AddChild(child); WritePostscript(); } ////// This method performs the core functionality of the AddChild() /// method on the IAddChild interface. For a Timeline this means /// determining adding the child parameter to the Children collection /// if it's a Timeline. /// ////// This method is the only core implementation. It does not call /// WritePreamble() or WritePostscript(). It also doesn't throw an /// ArgumentNullException if the child parameter is null. These tasks /// are performed by the interface implementation. Therefore, it's OK /// for a derived class to override this method and call the base /// class implementation only if they determine that it's the right /// course of action. The derived class can rely on Timeline's /// implementation of IAddChild.AddChild or implement their own /// following the Freezable pattern since that would be a public /// method. /// /// An object representing the child that /// should be added. If this is a Timeline it will be added to the /// Children collection; otherwise an exception will be thrown. ///The child parameter is not a /// Timeline. [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void AddChild(object child) { Timeline timelineChild = child as Timeline; if (timelineChild == null) { throw new ArgumentException(SR.Get(SRID.Timing_ChildMustBeTimeline), "child"); } else { Children.Add(timelineChild); } } ////// Adds a text string as a child of this Timeline. /// /// /// The text to add. /// ////// A Timeline does not accept text as a child, so this method will /// raise an InvalididOperationException unless a derived class has /// overridden the behavior to add text. /// ///The childText parameter is /// null. void IAddChild.AddText(string childText) { WritePreamble(); if (childText == null) { throw new ArgumentNullException("childText"); } AddText(childText); WritePostscript(); } ////// This method performs the core functionality of the AddText() /// method on the IAddChild interface. For a Timeline this means /// throwing and InvalidOperationException because it doesn't /// support adding text. /// ////// This method is the only core implementation. It does not call /// WritePreamble() or WritePostscript(). It also doesn't throw an /// ArgumentNullException if the childText parameter is null. These tasks /// are performed by the interface implementation. Therefore, it's OK /// for a derived class to override this method and call the base /// class implementation only if they determine that it's the right /// course of action. The derived class can rely on Timeline's /// implementation of IAddChild.AddChild or implement their own /// following the Freezable pattern since that would be a public /// method. /// /// A string representing the child text that /// should be added. If this is a Timeline an exception will be /// thrown. ///Timelines have no way /// of adding text. [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void AddText(string childText) { throw new InvalidOperationException(SR.Get(SRID.Timing_NoTextChildren)); } #endregion // IAddChild interface } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
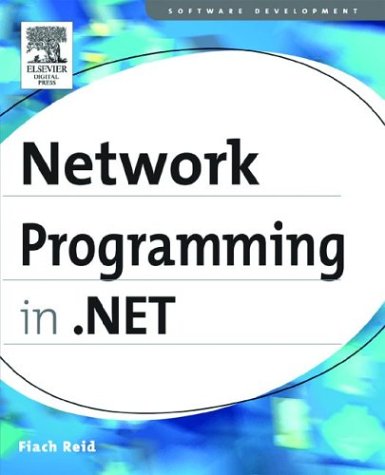
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MessageBox.cs
- Propagator.Evaluator.cs
- CodeTypeReferenceCollection.cs
- ElementProxy.cs
- ValidatorCollection.cs
- LayoutInformation.cs
- BinHexEncoding.cs
- Typeface.cs
- SimpleWebHandlerParser.cs
- SafeCryptHandles.cs
- SafeSecurityHelper.cs
- OleDbRowUpdatedEvent.cs
- MulticastDelegate.cs
- XmlNodeChangedEventManager.cs
- GridPattern.cs
- EntityDataSourceUtil.cs
- BindingNavigator.cs
- GridItemProviderWrapper.cs
- ChangePasswordAutoFormat.cs
- ErrorWrapper.cs
- ToolboxItemCollection.cs
- Wizard.cs
- HtmlHead.cs
- Literal.cs
- PropertyTab.cs
- TypeElementCollection.cs
- StyleReferenceConverter.cs
- WebResourceAttribute.cs
- RequestTimeoutManager.cs
- ControlPropertyNameConverter.cs
- StandardBindingElement.cs
- ACE.cs
- OSFeature.cs
- CharKeyFrameCollection.cs
- FlatButtonAppearance.cs
- ResourceDisplayNameAttribute.cs
- PerfService.cs
- HtmlTableCell.cs
- XsltInput.cs
- EllipticalNodeOperations.cs
- HttpListenerTimeoutManager.cs
- CultureInfo.cs
- ButtonBaseAutomationPeer.cs
- Pair.cs
- RichTextBox.cs
- HighlightVisual.cs
- DataSourceHelper.cs
- NetCodeGroup.cs
- ADMembershipProvider.cs
- NameScope.cs
- PathFigureCollectionValueSerializer.cs
- Matrix3DValueSerializer.cs
- OutputCacheModule.cs
- ContentPosition.cs
- SHA1Managed.cs
- SqlInternalConnection.cs
- ConvertEvent.cs
- StringWriter.cs
- WorkflowViewService.cs
- CodeNamespaceImport.cs
- TraceHelpers.cs
- DrawingImage.cs
- DataBinder.cs
- IncomingWebRequestContext.cs
- TcpTransportElement.cs
- MemberInitExpression.cs
- Suspend.cs
- SchemaNotation.cs
- DbConvert.cs
- ColumnMapCopier.cs
- SubMenuStyleCollectionEditor.cs
- Animatable.cs
- DeleteBookmarkScope.cs
- DesignerLoader.cs
- DataObjectCopyingEventArgs.cs
- DataServiceClientException.cs
- DataTrigger.cs
- Trigger.cs
- CompatibleIComparer.cs
- ReadOnlyCollection.cs
- ObfuscateAssemblyAttribute.cs
- SqlFileStream.cs
- ScopedKnownTypes.cs
- BrowserDefinitionCollection.cs
- PerformanceCounterManager.cs
- WebPartUtil.cs
- StyleSheetDesigner.cs
- CancelEventArgs.cs
- GlobalItem.cs
- ColumnMapCopier.cs
- TextElementAutomationPeer.cs
- LocalBuilder.cs
- PropertyStore.cs
- ComponentResourceKey.cs
- AsmxEndpointPickerExtension.cs
- PersistChildrenAttribute.cs
- ByteStack.cs
- BindStream.cs
- XmlSchemaComplexType.cs
- HitTestParameters.cs