Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Primitives / DataGridRowsPresenter.cs / 1305600 / DataGridRowsPresenter.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Threading; using MS.Internal; namespace System.Windows.Controls.Primitives { ////// Panel that lays out individual rows top to bottom. /// public class DataGridRowsPresenter : VirtualizingStackPanel { #region Scroll Methods ////// Calls the protected method BringIndexIntoView. /// /// The index of the row to scroll into view. ////// BringIndexIntoView should be callable either from the ItemsControl /// or directly on the panel. This was not done in WPF, so we are /// building this internally for the DataGrid. However, if a public /// way of calling BringIndexIntoView becomes a reality, then /// this method is no longer needed. /// internal void InternalBringIndexIntoView(int index) { BringIndexIntoView(index); } ////// This method is invoked when the IsItemsHost property changes. /// /// The old value of the IsItemsHost property. /// The new value of the IsItemsHost property. protected override void OnIsItemsHostChanged(bool oldIsItemsHost, bool newIsItemsHost) { base.OnIsItemsHostChanged(oldIsItemsHost, newIsItemsHost); if (newIsItemsHost) { DataGrid dataGrid = Owner; if (dataGrid != null) { // ItemsHost should be the "root" element which has // IsItemsHost = true on it. In the case of grouping, // IsItemsHost is true on all panels which are generating // content. Thus, we care only about the panel which // is generating content for the ItemsControl. IItemContainerGenerator generator = dataGrid.ItemContainerGenerator as IItemContainerGenerator; if (generator != null && generator == generator.GetItemContainerGeneratorForPanel(this)) { dataGrid.InternalItemsHost = this; } } } else { // No longer the items host, clear out the property on the DataGrid if ((_owner != null) && (_owner.InternalItemsHost == this)) { _owner.InternalItemsHost = null; } _owner = null; } } ////// Override of ViewportSizeChanged method which enqueues a dispatcher operation to redistribute /// the width among columns. /// /// viewport size before the change /// viewport size after the change protected override void OnViewportSizeChanged(Size oldViewportSize, Size newViewportSize) { DataGrid dataGrid = Owner; if (dataGrid != null) { ScrollContentPresenter scrollContentPresenter = dataGrid.InternalScrollContentPresenter; if (scrollContentPresenter == null || scrollContentPresenter.CanContentScroll) { dataGrid.OnViewportSizeChanged(oldViewportSize, newViewportSize); } } } #endregion #region Column Virtualization ////// Measure /// protected override Size MeasureOverride(Size constraint) { _availableSize = constraint; return base.MeasureOverride(constraint); } ////// Property which returns the last measure input size /// internal Size AvailableSize { get { return _availableSize; } } #endregion #region Row Virtualization ////// Override method to suppress the cleanup of rows /// with validation errors. /// /// protected override void OnCleanUpVirtualizedItem(CleanUpVirtualizedItemEventArgs e) { base.OnCleanUpVirtualizedItem(e); if (e.UIElement != null && Validation.GetHasError(e.UIElement)) { e.Cancel = true; } } #endregion #region Helpers internal DataGrid Owner { get { if (_owner == null) { _owner = ItemsControl.GetItemsOwner(this) as DataGrid; } return _owner; } } #endregion #region Data private DataGrid _owner; private Size _availableSize; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
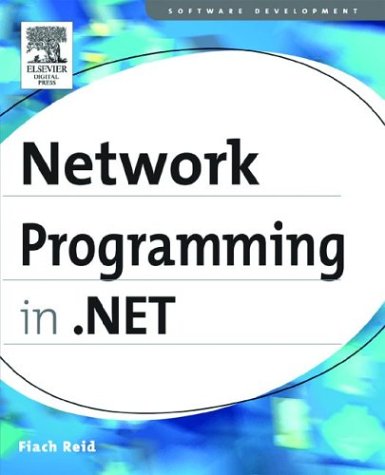
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FamilyTypeface.cs
- SrgsToken.cs
- RepeaterItem.cs
- WebBrowserUriTypeConverter.cs
- EntityKey.cs
- ToolStripContainer.cs
- ACE.cs
- SetterBase.cs
- ChannelManager.cs
- BitmapEffectInput.cs
- DataBoundControlHelper.cs
- DataTableClearEvent.cs
- Conditional.cs
- FontNamesConverter.cs
- NonParentingControl.cs
- URLString.cs
- InvalidProgramException.cs
- FixedSOMElement.cs
- EdmTypeAttribute.cs
- DependsOnAttribute.cs
- Root.cs
- ReliableMessagingVersion.cs
- ExpressionBuilderContext.cs
- LockCookie.cs
- AnnotationObservableCollection.cs
- GeometryGroup.cs
- ArrayConverter.cs
- ModelFunctionTypeElement.cs
- GACMembershipCondition.cs
- Char.cs
- ResourceDescriptionAttribute.cs
- ClientRuntime.cs
- UnauthorizedWebPart.cs
- EventRoute.cs
- SmtpDigestAuthenticationModule.cs
- SingleConverter.cs
- TextElementCollection.cs
- ConstructorBuilder.cs
- TableRowGroup.cs
- LeaseManager.cs
- _emptywebproxy.cs
- TextEditorThreadLocalStore.cs
- TextBox.cs
- LabelInfo.cs
- DataViewListener.cs
- StorageComplexPropertyMapping.cs
- QilFunction.cs
- DataListItemCollection.cs
- SiteMapNodeItem.cs
- OwnerDrawPropertyBag.cs
- arabicshape.cs
- ValidatingPropertiesEventArgs.cs
- DataPointer.cs
- WebServiceEnumData.cs
- cryptoapiTransform.cs
- StorageEntityContainerMapping.cs
- SchemaMerger.cs
- WebServiceHandler.cs
- LinqDataSourceStatusEventArgs.cs
- LinqDataSourceInsertEventArgs.cs
- Size3D.cs
- DataGridViewCellPaintingEventArgs.cs
- NotifyIcon.cs
- HighlightComponent.cs
- ConnectivityStatus.cs
- Assembly.cs
- HashAlgorithm.cs
- CodeCompileUnit.cs
- OletxTransactionManager.cs
- FileInfo.cs
- MessageSecurityOverTcpElement.cs
- WebPartExportVerb.cs
- WebPartPersonalization.cs
- StreamingContext.cs
- InputLanguageEventArgs.cs
- TimeSpan.cs
- MultitargetUtil.cs
- FontFamilyConverter.cs
- CursorConverter.cs
- MetafileHeaderWmf.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- XpsFixedPageReaderWriter.cs
- RowType.cs
- EndPoint.cs
- InteropBitmapSource.cs
- WebZone.cs
- CustomErrorCollection.cs
- MenuItemBinding.cs
- BamlMapTable.cs
- CngUIPolicy.cs
- ProxyAttribute.cs
- PrintPageEvent.cs
- DataSourceGeneratorException.cs
- ListViewItemSelectionChangedEvent.cs
- MetadataPropertyCollection.cs
- AppSettingsExpressionBuilder.cs
- SoapHttpTransportImporter.cs
- TokenFactoryBase.cs
- JapaneseLunisolarCalendar.cs
- TabControlDesigner.cs