Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / DataGridColumnFloatingHeader.cs / 1305600 / DataGridColumnFloatingHeader.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Controls.Primitives; using System.Windows.Media; using MS.Internal; namespace System.Windows.Controls { ////// The control which would be used to indicate the drag during column header drag-drop /// [TemplatePart(Name = "PART_VisualBrushCanvas", Type = typeof(Canvas))] internal class DataGridColumnFloatingHeader : Control { #region Constructors static DataGridColumnFloatingHeader() { DefaultStyleKeyProperty.OverrideMetadata( typeof(DataGridColumnFloatingHeader), new FrameworkPropertyMetadata(DataGridColumnHeader.ColumnFloatingHeaderStyleKey)); WidthProperty.OverrideMetadata( typeof(DataGridColumnFloatingHeader), new FrameworkPropertyMetadata(new PropertyChangedCallback(OnWidthChanged), new CoerceValueCallback(OnCoerceWidth))); HeightProperty.OverrideMetadata( typeof(DataGridColumnFloatingHeader), new FrameworkPropertyMetadata(new PropertyChangedCallback(OnHeightChanged), new CoerceValueCallback(OnCoerceHeight))); } #endregion #region Static Methods private static void OnWidthChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DataGridColumnFloatingHeader header = (DataGridColumnFloatingHeader)d; double width = (double)e.NewValue; if (header._visualBrushCanvas != null && !DoubleUtil.IsNaN(width)) { VisualBrush brush = header._visualBrushCanvas.Background as VisualBrush; if (brush != null) { Rect viewBox = brush.Viewbox; brush.Viewbox = new Rect(viewBox.X, viewBox.Y, width - header.GetVisualCanvasMarginX(), viewBox.Height); } } } private static object OnCoerceWidth(DependencyObject d, object baseValue) { double width = (double)baseValue; DataGridColumnFloatingHeader header = (DataGridColumnFloatingHeader)d; if (header._referenceHeader != null && DoubleUtil.IsNaN(width)) { return header._referenceHeader.ActualWidth + header.GetVisualCanvasMarginX(); } return baseValue; } private static void OnHeightChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DataGridColumnFloatingHeader header = (DataGridColumnFloatingHeader)d; double height = (double)e.NewValue; if (header._visualBrushCanvas != null && !DoubleUtil.IsNaN(height)) { VisualBrush brush = header._visualBrushCanvas.Background as VisualBrush; if (brush != null) { Rect viewBox = brush.Viewbox; brush.Viewbox = new Rect(viewBox.X, viewBox.Y, viewBox.Width, height - header.GetVisualCanvasMarginY()); } } } private static object OnCoerceHeight(DependencyObject d, object baseValue) { double height = (double)baseValue; DataGridColumnFloatingHeader header = (DataGridColumnFloatingHeader)d; if (header._referenceHeader != null && DoubleUtil.IsNaN(height)) { return header._referenceHeader.ActualHeight + header.GetVisualCanvasMarginY(); } return baseValue; } #endregion #region Methods and Properties public override void OnApplyTemplate() { base.OnApplyTemplate(); _visualBrushCanvas = GetTemplateChild(VisualBrushCanvasTemplateName) as Canvas; UpdateVisualBrush(); } internal DataGridColumnHeader ReferenceHeader { get { return _referenceHeader; } set { _referenceHeader = value; } } private void UpdateVisualBrush() { if (_referenceHeader != null && _visualBrushCanvas != null) { VisualBrush visualBrush = new VisualBrush(_referenceHeader); visualBrush.ViewboxUnits = BrushMappingMode.Absolute; double width = Width; if (DoubleUtil.IsNaN(width)) { width = _referenceHeader.ActualWidth; } else { width = width - GetVisualCanvasMarginX(); } double height = Height; if (DoubleUtil.IsNaN(height)) { height = _referenceHeader.ActualHeight; } else { height = height - GetVisualCanvasMarginY(); } Vector offset = VisualTreeHelper.GetOffset(_referenceHeader); visualBrush.Viewbox = new Rect(offset.X, offset.Y, width, height); _visualBrushCanvas.Background = visualBrush; } } internal void ClearHeader() { _referenceHeader = null; if (_visualBrushCanvas != null) { _visualBrushCanvas.Background = null; } } private double GetVisualCanvasMarginX() { double delta = 0; if (_visualBrushCanvas != null) { Thickness margin = _visualBrushCanvas.Margin; delta += margin.Left; delta += margin.Right; } return delta; } private double GetVisualCanvasMarginY() { double delta = 0; if (_visualBrushCanvas != null) { Thickness margin = _visualBrushCanvas.Margin; delta += margin.Top; delta += margin.Bottom; } return delta; } #endregion #region Data private DataGridColumnHeader _referenceHeader; private const string VisualBrushCanvasTemplateName = "PART_VisualBrushCanvas"; private Canvas _visualBrushCanvas; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
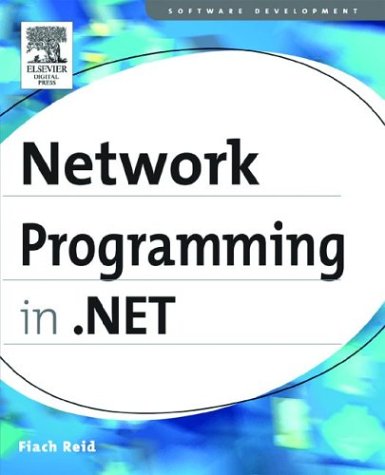
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Wow64ConfigurationLoader.cs
- XmlNodeList.cs
- WebHttpEndpointElement.cs
- StreamAsIStream.cs
- PrintPageEvent.cs
- ContentFileHelper.cs
- COM2IPerPropertyBrowsingHandler.cs
- DBConcurrencyException.cs
- BasicHttpBinding.cs
- Int16Storage.cs
- IsolatedStorageFileStream.cs
- FixedFindEngine.cs
- GB18030Encoding.cs
- DSACryptoServiceProvider.cs
- AuthorizationRule.cs
- SqlTransaction.cs
- PageWrapper.cs
- LicenseProviderAttribute.cs
- MbpInfo.cs
- RulePatternOps.cs
- FontFamilyIdentifier.cs
- ProjectionPruner.cs
- WhitespaceRule.cs
- MarkupCompilePass1.cs
- WeakHashtable.cs
- SafeFileMappingHandle.cs
- Win32Interop.cs
- AQNBuilder.cs
- AnnouncementEndpoint.cs
- AttributeUsageAttribute.cs
- InheritedPropertyChangedEventArgs.cs
- WindowsEditBoxRange.cs
- KeyValuePairs.cs
- mda.cs
- TemplateEditingFrame.cs
- Publisher.cs
- MetadataArtifactLoaderResource.cs
- XmlTextReaderImpl.cs
- TemplateNameScope.cs
- OleDbSchemaGuid.cs
- SqlInternalConnectionTds.cs
- GregorianCalendarHelper.cs
- DataExpression.cs
- DefaultValueAttribute.cs
- XmlCharacterData.cs
- GroupBox.cs
- SqlClientWrapperSmiStreamChars.cs
- nulltextnavigator.cs
- SelectionService.cs
- CodeValidator.cs
- ScriptResourceInfo.cs
- ControlCachePolicy.cs
- Crc32.cs
- DispatcherTimer.cs
- LogManagementAsyncResult.cs
- IdentityReference.cs
- VisualTreeUtils.cs
- BuildProviderCollection.cs
- ConditionalAttribute.cs
- SqlPersonalizationProvider.cs
- OutputCacheProfile.cs
- BaseCollection.cs
- EventEntry.cs
- ChildTable.cs
- CookieHandler.cs
- ProfileService.cs
- ProjectionCamera.cs
- FileChangesMonitor.cs
- HealthMonitoringSectionHelper.cs
- TTSEngineTypes.cs
- SplitContainer.cs
- TraceUtils.cs
- __Filters.cs
- Msec.cs
- CompensationHandlingFilter.cs
- AppDomainShutdownMonitor.cs
- SystemColors.cs
- Line.cs
- SqlCacheDependency.cs
- LabelDesigner.cs
- StyleSheet.cs
- Condition.cs
- TemplateEditingVerb.cs
- PageThemeCodeDomTreeGenerator.cs
- ImplicitInputBrush.cs
- RawUIStateInputReport.cs
- TriggerCollection.cs
- SR.Designer.cs
- SafeNativeMethods.cs
- MsmqIntegrationSecurityElement.cs
- PathGeometry.cs
- Scene3D.cs
- DependencyObject.cs
- Imaging.cs
- XmlObjectSerializerWriteContextComplex.cs
- Point3D.cs
- EpmSyndicationContentSerializer.cs
- ConfigurationManagerInternal.cs
- TaskResultSetter.cs
- ContractInferenceHelper.cs