Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusPlugin.cs / 1305600 / StylusPlugin.cs
// #define TRACE using System; using System.Diagnostics; using System.Collections; using System.Collections.Specialized; using System.Security.Permissions; using System.Windows.Threading; using System.Windows; using System.Windows.Input; using System.Windows.Media; using MS.Win32; // for *NativeMethods namespace System.Windows.Input.StylusPlugIns { ///////////////////////////////////////////////////////////////////// ////// [TBS] /// public abstract class StylusPlugIn { ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected StylusPlugIn() { } ///////////////////////////////////////////////////////////////////// // (in Dispatcher) internal void Added(StylusPlugInCollection plugInCollection) { _pic = plugInCollection; OnAdded(); InvalidateIsActiveForInput(); // Make sure we fire OnIsActivateForInputChanged. } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnAdded() { } ///////////////////////////////////////////////////////////////////// // (in Dispatcher) internal void Removed() { // Make sure we fire OnIsActivateForInputChanged if we need to. if (_activeForInput) { InvalidateIsActiveForInput(); } OnRemoved(); _pic = null; } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnRemoved() { } ///////////////////////////////////////////////////////////////////// // (in RTI Dispatcher) internal void StylusEnterLeave(bool isEnter, RawStylusInput rawStylusInput, bool confirmed) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { if (isEnter) OnStylusEnter(rawStylusInput, confirmed); else OnStylusLeave(rawStylusInput, confirmed); } } ///////////////////////////////////////////////////////////////////// // (in RTI Dispatcher) internal void RawStylusInput(RawStylusInput rawStylusInput) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { switch( rawStylusInput.Report.Actions ) { case RawStylusActions.Down: OnStylusDown(rawStylusInput); break; case RawStylusActions.Move: OnStylusMove(rawStylusInput); break; case RawStylusActions.Up: OnStylusUp(rawStylusInput); break; } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusEnter(RawStylusInput rawStylusInput, bool confirmed) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusLeave(RawStylusInput rawStylusInput, bool confirmed) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusDown(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusMove(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusUp(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// // (on app Dispatcher) internal void FireCustomData(object callbackData, RawStylusActions action, bool targetVerified) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { switch (action) { case RawStylusActions.Down: OnStylusDownProcessed(callbackData, targetVerified); break; case RawStylusActions.Move: OnStylusMoveProcessed(callbackData, targetVerified); break; case RawStylusActions.Up: OnStylusUpProcessed(callbackData, targetVerified); break; } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusDownProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusMoveProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusUpProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - both Dispatchers /// public UIElement Element { get { return (_pic != null) ? _pic.Element : null; } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - both Dispatchers /// public Rect ElementBounds { get { return (_pic != null) ? _pic.Rect : new Rect(); } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - get - both Dispatchers, set Dispatcher /// public bool Enabled { get // both Dispatchers { return __enabled; } set // on Dispatcher { // Verify we are on the proper thread. if (_pic != null) { _pic.Element.VerifyAccess(); } if (value != __enabled) { // If we are currently active for input we need to lock before input before // changing so we don't get input coming in before event is fired. if (_pic != null && _pic.IsActiveForInput) { // Make sure lock() doesn't cause reentrancy. using(_pic.Element.Dispatcher.DisableProcessing()) { lock(_pic.PenContextsSyncRoot) { // Make sure we fire the OnEnabledChanged event in the proper order // depending on whether we are going active or inactive so you don't // get input events after going inactive or before going active. __enabled = value; if (value == false) { // Make sure we fire OnIsActivateForInputChanged if we need to. InvalidateIsActiveForInput(); OnEnabledChanged(); } else { OnEnabledChanged(); InvalidateIsActiveForInput(); } } } } else { __enabled = value; if (value == false) { // Make sure we fire OnIsActivateForInputChanged if we need to. InvalidateIsActiveForInput(); OnEnabledChanged(); } else { OnEnabledChanged(); InvalidateIsActiveForInput(); } } } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnEnabledChanged() { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - app Dispatcher /// internal void InvalidateIsActiveForInput() { bool newIsActive = (_pic != null) ? (Enabled && _pic.Contains(this) && _pic.IsActiveForInput) : false; if (newIsActive != _activeForInput) { _activeForInput = newIsActive; OnIsActiveForInputChanged(); } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - get - both Dispatchers /// public bool IsActiveForInput { get // both Dispatchers { return _activeForInput; } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnIsActiveForInputChanged() { } // Enabled state is local to this plugin so we just use volatile versus creating a lock // around it since we just read it from multiple thread and write from one. volatile bool __enabled = true; bool _activeForInput = false; StylusPlugInCollection _pic = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
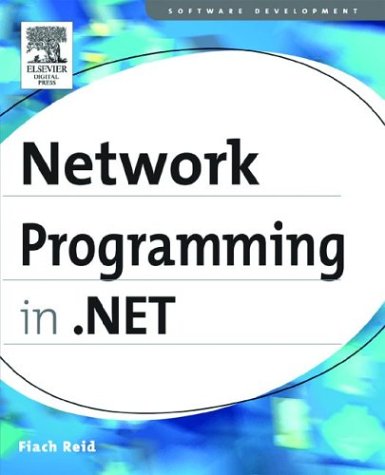
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaFacet.cs
- DelegateArgumentValue.cs
- SqlWorkflowInstanceStoreLock.cs
- CodeExpressionStatement.cs
- TerminatorSinks.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- DataTableNewRowEvent.cs
- VectorAnimationBase.cs
- Int16AnimationUsingKeyFrames.cs
- GridViewEditEventArgs.cs
- WebResourceAttribute.cs
- XmlMembersMapping.cs
- Rules.cs
- Privilege.cs
- FakeModelPropertyImpl.cs
- ApplicationBuildProvider.cs
- ResXResourceWriter.cs
- SchemaElementLookUpTable.cs
- ToolStripSplitButton.cs
- _HTTPDateParse.cs
- AspNetRouteServiceHttpHandler.cs
- ListViewSortEventArgs.cs
- CodeDOMUtility.cs
- CustomAttributeFormatException.cs
- MatrixConverter.cs
- XPathPatternParser.cs
- SmtpFailedRecipientException.cs
- PointHitTestParameters.cs
- MenuItem.cs
- StringToken.cs
- ControlEvent.cs
- DataRowCollection.cs
- RangeBaseAutomationPeer.cs
- RotateTransform3D.cs
- Fonts.cs
- WebEvents.cs
- UnauthorizedWebPart.cs
- CategoryAttribute.cs
- SafeRegistryHandle.cs
- EnvelopedPkcs7.cs
- __ComObject.cs
- EdgeModeValidation.cs
- PermissionSet.cs
- SubqueryRules.cs
- XmlLinkedNode.cs
- SvcMapFileSerializer.cs
- PeerToPeerException.cs
- DataGridViewCellEventArgs.cs
- Visual.cs
- PlatformNotSupportedException.cs
- DrawingGroup.cs
- SystemWebCachingSectionGroup.cs
- ViewStateChangedEventArgs.cs
- DeclaredTypeElementCollection.cs
- StylusPointPropertyInfo.cs
- TransformPattern.cs
- CompiledQuery.cs
- PropertyFilterAttribute.cs
- BrushConverter.cs
- OleDbConnectionFactory.cs
- RegexCompilationInfo.cs
- QuadraticBezierSegment.cs
- WebExceptionStatus.cs
- TransformGroup.cs
- DataServiceQueryProvider.cs
- FloaterParagraph.cs
- VectorConverter.cs
- CodeComment.cs
- OleDbCommandBuilder.cs
- RichTextBoxContextMenu.cs
- SerializationStore.cs
- SplineKeyFrames.cs
- WebPartTransformerAttribute.cs
- DataGridAutoGeneratingColumnEventArgs.cs
- LinkClickEvent.cs
- BindingExpressionBase.cs
- UnsafeNativeMethods.cs
- CryptoApi.cs
- OracleInfoMessageEventArgs.cs
- MetadataItemEmitter.cs
- DefaultParameterValueAttribute.cs
- ManagedFilter.cs
- OleDbErrorCollection.cs
- ProfileEventArgs.cs
- Substitution.cs
- remotingproxy.cs
- PreservationFileReader.cs
- PointCollection.cs
- ISessionStateStore.cs
- FontSourceCollection.cs
- QilParameter.cs
- XmlEnumAttribute.cs
- DbConnectionStringCommon.cs
- VisualStyleRenderer.cs
- ClassHandlersStore.cs
- ToolStripMenuItemDesigner.cs
- BinHexDecoder.cs
- TraceXPathNavigator.cs
- RadioButtonAutomationPeer.cs
- CmsUtils.cs