Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Data / System / Data / OleDb / OleDbConnectionFactory.cs / 1 / OleDbConnectionFactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.OleDb { using System; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; using System.Collections.Specialized; using System.Configuration; using System.IO; sealed internal class OleDbConnectionFactory : DbConnectionFactory { private OleDbConnectionFactory() : base() {} // At this time, the OleDb Managed Provider doesn't have any connection pool // counters because we'd only confuse people with "non-pooled" connections // that are actually being pooled by the native pooler. private const string _metaDataXml = ":MetaDataXml"; private const string _defaultMetaDataXml = "defaultMetaDataXml"; public static readonly OleDbConnectionFactory SingletonInstance = new OleDbConnectionFactory(); override public DbProviderFactory ProviderFactory { get { return OleDbFactory.Instance; } } override protected DbConnectionInternal CreateConnection(DbConnectionOptions options, object poolGroupProviderInfo, DbConnectionPool pool, DbConnection owningObject) { DbConnectionInternal result = new OleDbConnectionInternal((OleDbConnectionString)options, (OleDbConnection)owningObject); return result; } protected override DbConnectionOptions CreateConnectionOptions(string connectionString, DbConnectionOptions previous) { Debug.Assert(!ADP.IsEmpty(connectionString), "null connectionString"); OleDbConnectionString result = new OleDbConnectionString(connectionString, (null != previous)); return result; } override protected DbMetaDataFactory CreateMetaDataFactory(DbConnectionInternal internalConnection, out bool cacheMetaDataFactory){ Debug.Assert (internalConnection != null,"internalConnection may not be null."); cacheMetaDataFactory = false; OleDbConnectionInternal oleDbInternalConnection = (OleDbConnectionInternal) internalConnection; OleDbConnection oleDbOuterConnection = oleDbInternalConnection.Connection; Debug.Assert(oleDbOuterConnection != null,"outer connection may not be null."); NameValueCollection settings = (NameValueCollection)PrivilegedConfigurationManager.GetSection("system.data.oledb"); Stream XMLStream =null; String providerFileName = oleDbOuterConnection.GetDataSourcePropertyValue(OleDbPropertySetGuid.DataSourceInfo,ODB.DBPROP_PROVIDERFILENAME) as string; if (settings != null){ string [] values = null; string metaDataXML = null; // first try to get the provider specific xml // if providerfilename is not supported we can't build the settings key needed to // get the provider specific XML path if (providerFileName != null){ metaDataXML = providerFileName + _metaDataXml; values = settings.GetValues(metaDataXML); } // if we did not find provider specific xml see if there is new default xml if (values == null) { metaDataXML =_defaultMetaDataXml; values = settings.GetValues(metaDataXML); } // If there is new XML get it if (values != null) { XMLStream = ADP.GetXmlStreamFromValues(values,metaDataXML); } } // if the xml was not obtained from machine.config use the embedded XML resource if (XMLStream == null) { XMLStream = System.Reflection.Assembly.GetExecutingAssembly().GetManifestResourceStream("System.Data.OleDb.OleDbMetaData.xml"); cacheMetaDataFactory = true; } Debug.Assert (XMLStream != null,"XMLstream may not be null."); // using the ServerVersion as the NormalizedServerVersion. Doing this for two reasons // 1) The Spec for DBPROP_DBMSVER normalizes the ServerVersion // 2) for OLE DB its the only game in town return new OleDbMetaDataFactory (XMLStream, oleDbInternalConnection.ServerVersion, oleDbInternalConnection.ServerVersion, oleDbInternalConnection.GetSchemaRowsetInformation()); } override protected DbConnectionPoolGroupOptions CreateConnectionPoolGroupOptions(DbConnectionOptions connectionOptions) { return null; } override internal DbConnectionPoolGroupProviderInfo CreateConnectionPoolGroupProviderInfo (DbConnectionOptions connectionOptions) { return new OleDbConnectionPoolGroupProviderInfo(); } override internal DbConnectionPoolGroup GetConnectionPoolGroup(DbConnection connection) { OleDbConnection c = (connection as OleDbConnection); if (null != c) { return c.PoolGroup; } return null; } override internal DbConnectionInternal GetInnerConnection(DbConnection connection) { OleDbConnection c = (connection as OleDbConnection); if (null != c) { return c.InnerConnection; } return null; } override protected int GetObjectId(DbConnection connection) { OleDbConnection c = (connection as OleDbConnection); if (null != c) { return c.ObjectID; } return 0; } override internal void PermissionDemand(DbConnection outerConnection) { OleDbConnection c = (outerConnection as OleDbConnection); if (null != c) { c.PermissionDemand(); } } override internal void SetConnectionPoolGroup(DbConnection outerConnection, DbConnectionPoolGroup poolGroup) { OleDbConnection c = (outerConnection as OleDbConnection); if (null != c) { c.PoolGroup = poolGroup; } } override internal void SetInnerConnectionEvent(DbConnection owningObject, DbConnectionInternal to) { OleDbConnection c = (owningObject as OleDbConnection); if (null != c) { c.SetInnerConnectionEvent(to); } } override internal bool SetInnerConnectionFrom(DbConnection owningObject, DbConnectionInternal to, DbConnectionInternal from) { OleDbConnection c = (owningObject as OleDbConnection); if (null != c) { return c.SetInnerConnectionFrom(to, from); } return false; } override internal void SetInnerConnectionTo(DbConnection owningObject, DbConnectionInternal to) { OleDbConnection c = (owningObject as OleDbConnection); if (null != c) { c.SetInnerConnectionTo(to); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.OleDb { using System; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; using System.Collections.Specialized; using System.Configuration; using System.IO; sealed internal class OleDbConnectionFactory : DbConnectionFactory { private OleDbConnectionFactory() : base() {} // At this time, the OleDb Managed Provider doesn't have any connection pool // counters because we'd only confuse people with "non-pooled" connections // that are actually being pooled by the native pooler. private const string _metaDataXml = ":MetaDataXml"; private const string _defaultMetaDataXml = "defaultMetaDataXml"; public static readonly OleDbConnectionFactory SingletonInstance = new OleDbConnectionFactory(); override public DbProviderFactory ProviderFactory { get { return OleDbFactory.Instance; } } override protected DbConnectionInternal CreateConnection(DbConnectionOptions options, object poolGroupProviderInfo, DbConnectionPool pool, DbConnection owningObject) { DbConnectionInternal result = new OleDbConnectionInternal((OleDbConnectionString)options, (OleDbConnection)owningObject); return result; } protected override DbConnectionOptions CreateConnectionOptions(string connectionString, DbConnectionOptions previous) { Debug.Assert(!ADP.IsEmpty(connectionString), "null connectionString"); OleDbConnectionString result = new OleDbConnectionString(connectionString, (null != previous)); return result; } override protected DbMetaDataFactory CreateMetaDataFactory(DbConnectionInternal internalConnection, out bool cacheMetaDataFactory){ Debug.Assert (internalConnection != null,"internalConnection may not be null."); cacheMetaDataFactory = false; OleDbConnectionInternal oleDbInternalConnection = (OleDbConnectionInternal) internalConnection; OleDbConnection oleDbOuterConnection = oleDbInternalConnection.Connection; Debug.Assert(oleDbOuterConnection != null,"outer connection may not be null."); NameValueCollection settings = (NameValueCollection)PrivilegedConfigurationManager.GetSection("system.data.oledb"); Stream XMLStream =null; String providerFileName = oleDbOuterConnection.GetDataSourcePropertyValue(OleDbPropertySetGuid.DataSourceInfo,ODB.DBPROP_PROVIDERFILENAME) as string; if (settings != null){ string [] values = null; string metaDataXML = null; // first try to get the provider specific xml // if providerfilename is not supported we can't build the settings key needed to // get the provider specific XML path if (providerFileName != null){ metaDataXML = providerFileName + _metaDataXml; values = settings.GetValues(metaDataXML); } // if we did not find provider specific xml see if there is new default xml if (values == null) { metaDataXML =_defaultMetaDataXml; values = settings.GetValues(metaDataXML); } // If there is new XML get it if (values != null) { XMLStream = ADP.GetXmlStreamFromValues(values,metaDataXML); } } // if the xml was not obtained from machine.config use the embedded XML resource if (XMLStream == null) { XMLStream = System.Reflection.Assembly.GetExecutingAssembly().GetManifestResourceStream("System.Data.OleDb.OleDbMetaData.xml"); cacheMetaDataFactory = true; } Debug.Assert (XMLStream != null,"XMLstream may not be null."); // using the ServerVersion as the NormalizedServerVersion. Doing this for two reasons // 1) The Spec for DBPROP_DBMSVER normalizes the ServerVersion // 2) for OLE DB its the only game in town return new OleDbMetaDataFactory (XMLStream, oleDbInternalConnection.ServerVersion, oleDbInternalConnection.ServerVersion, oleDbInternalConnection.GetSchemaRowsetInformation()); } override protected DbConnectionPoolGroupOptions CreateConnectionPoolGroupOptions(DbConnectionOptions connectionOptions) { return null; } override internal DbConnectionPoolGroupProviderInfo CreateConnectionPoolGroupProviderInfo (DbConnectionOptions connectionOptions) { return new OleDbConnectionPoolGroupProviderInfo(); } override internal DbConnectionPoolGroup GetConnectionPoolGroup(DbConnection connection) { OleDbConnection c = (connection as OleDbConnection); if (null != c) { return c.PoolGroup; } return null; } override internal DbConnectionInternal GetInnerConnection(DbConnection connection) { OleDbConnection c = (connection as OleDbConnection); if (null != c) { return c.InnerConnection; } return null; } override protected int GetObjectId(DbConnection connection) { OleDbConnection c = (connection as OleDbConnection); if (null != c) { return c.ObjectID; } return 0; } override internal void PermissionDemand(DbConnection outerConnection) { OleDbConnection c = (outerConnection as OleDbConnection); if (null != c) { c.PermissionDemand(); } } override internal void SetConnectionPoolGroup(DbConnection outerConnection, DbConnectionPoolGroup poolGroup) { OleDbConnection c = (outerConnection as OleDbConnection); if (null != c) { c.PoolGroup = poolGroup; } } override internal void SetInnerConnectionEvent(DbConnection owningObject, DbConnectionInternal to) { OleDbConnection c = (owningObject as OleDbConnection); if (null != c) { c.SetInnerConnectionEvent(to); } } override internal bool SetInnerConnectionFrom(DbConnection owningObject, DbConnectionInternal to, DbConnectionInternal from) { OleDbConnection c = (owningObject as OleDbConnection); if (null != c) { return c.SetInnerConnectionFrom(to, from); } return false; } override internal void SetInnerConnectionTo(DbConnection owningObject, DbConnectionInternal to) { OleDbConnection c = (owningObject as OleDbConnection); if (null != c) { c.SetInnerConnectionTo(to); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
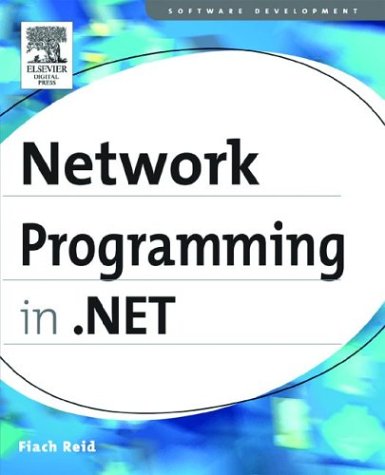
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OracleParameter.cs
- EventHandlers.cs
- CodeDOMUtility.cs
- ItemCollectionEditor.cs
- SelectionManager.cs
- UriParserTemplates.cs
- SqlBulkCopyColumnMappingCollection.cs
- HtmlInputImage.cs
- CharacterString.cs
- TypeUtil.cs
- StylusCollection.cs
- SystemWebSectionGroup.cs
- SafeProcessHandle.cs
- LinkLabel.cs
- Vector3DAnimationBase.cs
- XMLSchema.cs
- QuerySettings.cs
- SecurityTokenTypes.cs
- MessageSecurityOverMsmq.cs
- BuildProvidersCompiler.cs
- URL.cs
- SiteOfOriginPart.cs
- GlyphInfoList.cs
- ComplexTypeEmitter.cs
- PropertyConverter.cs
- HttpPostClientProtocol.cs
- DataKey.cs
- ItemCheckedEvent.cs
- XNodeSchemaApplier.cs
- ResourceReader.cs
- CodeSubDirectoriesCollection.cs
- RootAction.cs
- MimeWriter.cs
- CachedFontFamily.cs
- PrintDialog.cs
- ParamArrayAttribute.cs
- TraceSwitch.cs
- InheritedPropertyDescriptor.cs
- ExpressionEditorAttribute.cs
- BaseDataList.cs
- SignedXml.cs
- NativeMethods.cs
- TreeNodeStyleCollection.cs
- CompensationToken.cs
- DataGrid.cs
- SqlHelper.cs
- XmlHierarchyData.cs
- StaticDataManager.cs
- CompilationRelaxations.cs
- ThicknessAnimationBase.cs
- WhiteSpaceTrimStringConverter.cs
- FlowDocumentReaderAutomationPeer.cs
- ColorConvertedBitmap.cs
- Divide.cs
- WebPartAddingEventArgs.cs
- TreeNodeMouseHoverEvent.cs
- PermissionListSet.cs
- ByteStreamGeometryContext.cs
- RelationshipConstraintValidator.cs
- ConfigXmlDocument.cs
- WorkflowIdleElement.cs
- ListViewSelectEventArgs.cs
- TextEditorContextMenu.cs
- SqlBulkCopy.cs
- CachedTypeface.cs
- ContextMenuAutomationPeer.cs
- NamespaceList.cs
- AssemblyLoader.cs
- WebPartZone.cs
- HtmlFormWrapper.cs
- SelectionPatternIdentifiers.cs
- SimpleLine.cs
- NumberFormatInfo.cs
- MsdtcWrapper.cs
- PresentationAppDomainManager.cs
- ColorDialog.cs
- SponsorHelper.cs
- AuthenticationConfig.cs
- Italic.cs
- ImageCodecInfoPrivate.cs
- PreparingEnlistment.cs
- PaperSource.cs
- DataGridColumnEventArgs.cs
- XmlNode.cs
- UnsafeNativeMethodsCLR.cs
- ListManagerBindingsCollection.cs
- XmlSchemaAttribute.cs
- MetadataArtifactLoaderFile.cs
- MarginCollapsingState.cs
- PersonalizableAttribute.cs
- HyperLink.cs
- WindowsIPAddress.cs
- XslAstAnalyzer.cs
- WindowsListView.cs
- DesignerAutoFormatCollection.cs
- ResourceKey.cs
- DBAsyncResult.cs
- Stackframe.cs
- ChtmlTextWriter.cs
- FontWeightConverter.cs