Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / DataServiceQueryProvider.cs / 1625574 / DataServiceQueryProvider.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Query Provider for Linq to URI translatation // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; #endregion Namespaces. ////// QueryProvider implementation /// internal sealed class DataServiceQueryProvider : IQueryProvider { ///DataServiceContext for query provider internal readonly DataServiceContext Context; ///Constructs a query provider based on the context passed in /// The context for the query provider internal DataServiceQueryProvider(DataServiceContext context) { this.Context = context; } #region IQueryProvider implementation ///Factory method for creating DataServiceOrderedQuery based on expression /// The expression for the new query ///new DataServiceQuery [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1062:Validate arguments of public methods", MessageId = "0", Justification = "parameters are validated against null via CheckArgumentNull")] public IQueryable CreateQuery(Expression expression) { Util.CheckArgumentNull(expression, "expression"); Type et = TypeSystem.GetElementType(expression.Type); Type qt = typeof(DataServiceQuery<>.DataServiceOrderedQuery).MakeGenericType(et); object[] args = new object[] { expression, this }; ConstructorInfo ci = qt.GetConstructor( BindingFlags.NonPublic | BindingFlags.Instance, null, new Type[] { typeof(Expression), typeof(DataServiceQueryProvider) }, null); return (IQueryable)Util.ConstructorInvoke(ci, args); } ///Factory method for creating DataServiceOrderedQuery based on expression ///generic type /// The expression for the new query ///new DataServiceQuery public IQueryableCreateQuery (Expression expression) { Util.CheckArgumentNull(expression, "expression"); return new DataServiceQuery .DataServiceOrderedQuery(expression, this); } /// Creates and executes a DataServiceQuery based on the passed in expression /// The expression for the new query ///the results [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1062:Validate arguments of public methods", MessageId = "0", Justification = "parameters are validated against null via CheckArgumentNull")] [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining | System.Runtime.CompilerServices.MethodImplOptions.NoOptimization)] public object Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); MethodInfo mi = typeof(DataServiceQueryProvider).GetMethod("ReturnSingleton", BindingFlags.NonPublic | BindingFlags.Instance); return mi.MakeGenericMethod(expression.Type).Invoke(this, new object[] { expression }); } ///Creates and executes a DataServiceQuery based on the passed in expression ///generic type /// The expression for the new query ///the results public TResult Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); return ReturnSingleton (expression); } #endregion /// Creates and executes a DataServiceQuery based on the passed in expression which results a single value ///generic type /// The expression for the new query ///single valued results internal TElement ReturnSingleton(Expression expression) { IQueryable query = new DataServiceQuery .DataServiceOrderedQuery(expression, this); MethodCallExpression mce = expression as MethodCallExpression; Debug.Assert(mce != null, "mce != null"); SequenceMethod sequenceMethod; if (ReflectionUtil.TryIdentifySequenceMethod(mce.Method, out sequenceMethod)) { switch (sequenceMethod) { case SequenceMethod.Single: return query.AsEnumerable().Single(); case SequenceMethod.SingleOrDefault: return query.AsEnumerable().SingleOrDefault(); case SequenceMethod.First: return query.AsEnumerable().First(); case SequenceMethod.FirstOrDefault: return query.AsEnumerable().FirstOrDefault(); #if !ASTORIA_LIGHT case SequenceMethod.LongCount: case SequenceMethod.Count: return (TElement)Convert.ChangeType(((DataServiceQuery )query).GetQuerySetCount(this.Context), typeof(TElement), System.Globalization.CultureInfo.InvariantCulture.NumberFormat); #endif default: throw Error.MethodNotSupported(mce); } } // Should never get here - should be caught by expression compiler. Debug.Assert(false, "Not supported singleton operator not caught by Resource Binder"); throw Error.MethodNotSupported(mce); } /// Builds the Uri for the expression passed in. /// The expression to translate into a Uri ///Query components internal QueryComponents Translate(Expression e) { Uri uri; Version version; bool addTrailingParens = false; DictionarynormalizerRewrites = null; // short cut analysis if just a resource set // note - to be backwards compatible with V1, will only append trailing () for queries // that include more then just a resource set. if (!(e is ResourceSetExpression)) { normalizerRewrites = new Dictionary (ReferenceEqualityComparer .Instance); e = Evaluator.PartialEval(e); e = ExpressionNormalizer.Normalize(e, normalizerRewrites); e = ResourceBinder.Bind(e); addTrailingParens = true; } UriWriter.Translate(this.Context, addTrailingParens, e, out uri, out version); ResourceExpression re = e as ResourceExpression; Type lastSegmentType = re.Projection == null ? re.ResourceType : re.Projection.Selector.Parameters[0].Type; LambdaExpression selector = re.Projection == null ? null : re.Projection.Selector; return new QueryComponents(uri, version, lastSegmentType, selector, normalizerRewrites); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// Query Provider for Linq to URI translatation // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; #endregion Namespaces. ////// QueryProvider implementation /// internal sealed class DataServiceQueryProvider : IQueryProvider { ///DataServiceContext for query provider internal readonly DataServiceContext Context; ///Constructs a query provider based on the context passed in /// The context for the query provider internal DataServiceQueryProvider(DataServiceContext context) { this.Context = context; } #region IQueryProvider implementation ///Factory method for creating DataServiceOrderedQuery based on expression /// The expression for the new query ///new DataServiceQuery [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1062:Validate arguments of public methods", MessageId = "0", Justification = "parameters are validated against null via CheckArgumentNull")] public IQueryable CreateQuery(Expression expression) { Util.CheckArgumentNull(expression, "expression"); Type et = TypeSystem.GetElementType(expression.Type); Type qt = typeof(DataServiceQuery<>.DataServiceOrderedQuery).MakeGenericType(et); object[] args = new object[] { expression, this }; ConstructorInfo ci = qt.GetConstructor( BindingFlags.NonPublic | BindingFlags.Instance, null, new Type[] { typeof(Expression), typeof(DataServiceQueryProvider) }, null); return (IQueryable)Util.ConstructorInvoke(ci, args); } ///Factory method for creating DataServiceOrderedQuery based on expression ///generic type /// The expression for the new query ///new DataServiceQuery public IQueryableCreateQuery (Expression expression) { Util.CheckArgumentNull(expression, "expression"); return new DataServiceQuery .DataServiceOrderedQuery(expression, this); } /// Creates and executes a DataServiceQuery based on the passed in expression /// The expression for the new query ///the results [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1062:Validate arguments of public methods", MessageId = "0", Justification = "parameters are validated against null via CheckArgumentNull")] [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining | System.Runtime.CompilerServices.MethodImplOptions.NoOptimization)] public object Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); MethodInfo mi = typeof(DataServiceQueryProvider).GetMethod("ReturnSingleton", BindingFlags.NonPublic | BindingFlags.Instance); return mi.MakeGenericMethod(expression.Type).Invoke(this, new object[] { expression }); } ///Creates and executes a DataServiceQuery based on the passed in expression ///generic type /// The expression for the new query ///the results public TResult Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); return ReturnSingleton (expression); } #endregion /// Creates and executes a DataServiceQuery based on the passed in expression which results a single value ///generic type /// The expression for the new query ///single valued results internal TElement ReturnSingleton(Expression expression) { IQueryable query = new DataServiceQuery .DataServiceOrderedQuery(expression, this); MethodCallExpression mce = expression as MethodCallExpression; Debug.Assert(mce != null, "mce != null"); SequenceMethod sequenceMethod; if (ReflectionUtil.TryIdentifySequenceMethod(mce.Method, out sequenceMethod)) { switch (sequenceMethod) { case SequenceMethod.Single: return query.AsEnumerable().Single(); case SequenceMethod.SingleOrDefault: return query.AsEnumerable().SingleOrDefault(); case SequenceMethod.First: return query.AsEnumerable().First(); case SequenceMethod.FirstOrDefault: return query.AsEnumerable().FirstOrDefault(); #if !ASTORIA_LIGHT case SequenceMethod.LongCount: case SequenceMethod.Count: return (TElement)Convert.ChangeType(((DataServiceQuery )query).GetQuerySetCount(this.Context), typeof(TElement), System.Globalization.CultureInfo.InvariantCulture.NumberFormat); #endif default: throw Error.MethodNotSupported(mce); } } // Should never get here - should be caught by expression compiler. Debug.Assert(false, "Not supported singleton operator not caught by Resource Binder"); throw Error.MethodNotSupported(mce); } /// Builds the Uri for the expression passed in. /// The expression to translate into a Uri ///Query components internal QueryComponents Translate(Expression e) { Uri uri; Version version; bool addTrailingParens = false; DictionarynormalizerRewrites = null; // short cut analysis if just a resource set // note - to be backwards compatible with V1, will only append trailing () for queries // that include more then just a resource set. if (!(e is ResourceSetExpression)) { normalizerRewrites = new Dictionary (ReferenceEqualityComparer .Instance); e = Evaluator.PartialEval(e); e = ExpressionNormalizer.Normalize(e, normalizerRewrites); e = ResourceBinder.Bind(e); addTrailingParens = true; } UriWriter.Translate(this.Context, addTrailingParens, e, out uri, out version); ResourceExpression re = e as ResourceExpression; Type lastSegmentType = re.Projection == null ? re.ResourceType : re.Projection.Selector.Parameters[0].Type; LambdaExpression selector = re.Projection == null ? null : re.Projection.Selector; return new QueryComponents(uri, version, lastSegmentType, selector, normalizerRewrites); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
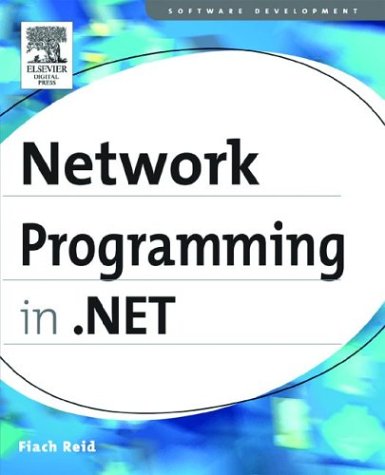
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeViewImageIndexConverter.cs
- Closure.cs
- ContentElementAutomationPeer.cs
- EmptyStringExpandableObjectConverter.cs
- fixedPageContentExtractor.cs
- ReliableChannelBinder.cs
- MultiDataTrigger.cs
- AnonymousIdentificationModule.cs
- OpCopier.cs
- OleDbCommand.cs
- TextPattern.cs
- DataServiceContext.cs
- FocusTracker.cs
- DataTableNewRowEvent.cs
- SinglePhaseEnlistment.cs
- CodeTypeDeclaration.cs
- ActivityInstance.cs
- UrlMappingsModule.cs
- Wildcard.cs
- BaseValidator.cs
- ToolboxItemWrapper.cs
- SafeNativeMethods.cs
- ApplicationInterop.cs
- FixUpCollection.cs
- ConnectionPointCookie.cs
- KeyEventArgs.cs
- FixedElement.cs
- DropDownList.cs
- ConfigLoader.cs
- MenuCommand.cs
- CompilerInfo.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- ExpressionBuilderContext.cs
- ShortcutKeysEditor.cs
- ObjectStateFormatter.cs
- InstancePersistenceContext.cs
- DoubleUtil.cs
- SqlRewriteScalarSubqueries.cs
- CounterCreationData.cs
- XmlEnumAttribute.cs
- Method.cs
- DocumentSchemaValidator.cs
- NameNode.cs
- CodeVariableReferenceExpression.cs
- BindingsCollection.cs
- DomainUpDown.cs
- HttpCachePolicyBase.cs
- NamespaceList.cs
- CodeDirectiveCollection.cs
- MaterialCollection.cs
- KeyboardNavigation.cs
- XmlSerializerFactory.cs
- BamlLocalizableResourceKey.cs
- Int64Storage.cs
- HttpHandlerActionCollection.cs
- StrongNamePublicKeyBlob.cs
- CodeThrowExceptionStatement.cs
- InputEventArgs.cs
- ContentPlaceHolder.cs
- ProvidersHelper.cs
- TextProviderWrapper.cs
- SequenceFullException.cs
- DataReceivedEventArgs.cs
- ContentType.cs
- BasePattern.cs
- Rotation3DAnimationBase.cs
- CultureInfoConverter.cs
- EventData.cs
- StreamInfo.cs
- InputReport.cs
- TimelineClockCollection.cs
- ParameterToken.cs
- DataBindEngine.cs
- DataGridRelationshipRow.cs
- PreservationFileWriter.cs
- MessageBox.cs
- CatalogZoneAutoFormat.cs
- Atom10FormatterFactory.cs
- WorkflowApplicationIdleEventArgs.cs
- WebPart.cs
- HttpCacheVaryByContentEncodings.cs
- AdRotator.cs
- StreamReader.cs
- DataStreams.cs
- OpCodes.cs
- ListDictionaryInternal.cs
- DataServiceBuildProvider.cs
- TypeHelpers.cs
- WindowCollection.cs
- SecondaryViewProvider.cs
- ListViewPagedDataSource.cs
- PrintingPermissionAttribute.cs
- MouseOverProperty.cs
- HtmlShimManager.cs
- RequiredAttributeAttribute.cs
- TdsEnums.cs
- XmlSchemaSimpleType.cs
- TextWriter.cs
- ServiceBehaviorElement.cs
- LicenseContext.cs