Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Ink / Quad.cs / 1305600 / Quad.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { ////// A helper structure used in StrokeNode and StrokeNodeOperation implementations /// to store endpoints of the quad connecting two nodes of a stroke. /// The vertices of a quad are supposed to be clockwise with points A and D located /// on the begin node and B and C on the end one. /// internal struct Quad { #region Statics private static Quad s_empty = new Quad(new Point(0, 0), new Point(0, 0), new Point(0, 0), new Point(0, 0)); #endregion #region API ///Returns the static object representing an empty (unitialized) quad internal static Quad Empty { get { return s_empty; } } ///Constructor internal Quad(Point a, Point b, Point c, Point d) { _A = a; _B = b; _C = c; _D = d; } ///The A vertex of the quad internal Point A { get { return _A; } set { _A = value; } } ///The B vertex of the quad internal Point B { get { return _B; } set { _B = value; } } ///The C vertex of the quad internal Point C { get { return _C; } set { _C = value; } } ///The D vertex of the quad internal Point D { get { return _D; } set { _D = value; } } // Returns quad's vertex by index where A is of the index 0, B - is 1, etc internal Point this[int index] { get { switch (index) { case 0: return _A; case 1: return _B; case 2: return _C; case 3: return _D; default: throw new IndexOutOfRangeException("index"); } } } ///Tells whether the quad is invalid (empty) internal bool IsEmpty { get { return (_A == _B) && (_C == _D); } } internal void GetPoints(ListpointBuffer) { pointBuffer.Add(_A); pointBuffer.Add(_B); pointBuffer.Add(_C); pointBuffer.Add(_D); } /// Returns the bounds of the quad internal Rect Bounds { get { return IsEmpty ? Rect.Empty : Rect.Union(new Rect(_A, _B), new Rect(_C, _D)); } } #endregion #region Fields private Point _A; private Point _B; private Point _C; private Point _D; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
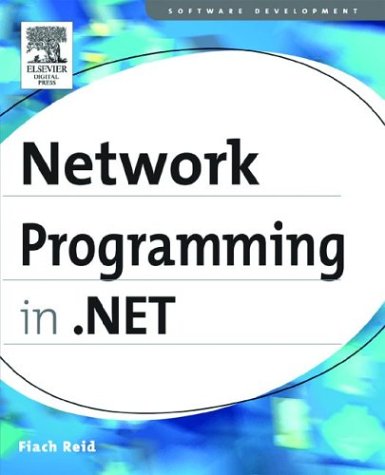
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlUTF8TextReader.cs
- TypographyProperties.cs
- HttpServerUtilityWrapper.cs
- XmlImplementation.cs
- GuidelineCollection.cs
- EntryPointNotFoundException.cs
- ExeConfigurationFileMap.cs
- HybridDictionary.cs
- CustomLineCap.cs
- DependencyPropertyValueSerializer.cs
- NameTable.cs
- DataControlImageButton.cs
- MulticastOption.cs
- XmlAnyElementAttribute.cs
- WebBrowser.cs
- AdobeCFFWrapper.cs
- RunClient.cs
- InstallerTypeAttribute.cs
- EventLog.cs
- DetailsViewDeletedEventArgs.cs
- RegexWriter.cs
- ConfigUtil.cs
- BrowsableAttribute.cs
- TextEndOfSegment.cs
- InputGestureCollection.cs
- UpdateException.cs
- GridViewColumn.cs
- SqlConnectionFactory.cs
- MultipartIdentifier.cs
- EventListenerClientSide.cs
- CancellationHandlerDesigner.cs
- HttpProfileGroupBase.cs
- BitmapCacheBrush.cs
- DataSourceGeneratorException.cs
- WindowsListView.cs
- AuthStoreRoleProvider.cs
- ProcessInfo.cs
- CharacterBuffer.cs
- DetailsViewPageEventArgs.cs
- MetadataImporterQuotas.cs
- ImportException.cs
- BitmapCacheBrush.cs
- GeometryHitTestResult.cs
- DataPagerField.cs
- AsyncResult.cs
- IgnoreSection.cs
- Rect.cs
- ITextView.cs
- SID.cs
- FormsAuthenticationModule.cs
- WpfGeneratedKnownProperties.cs
- DummyDataSource.cs
- FixedSOMGroup.cs
- selecteditemcollection.cs
- MethodToken.cs
- PenCursorManager.cs
- SafeBitVector32.cs
- DataListDesigner.cs
- TypedTableGenerator.cs
- WebBrowserNavigatingEventHandler.cs
- CompositeCollection.cs
- TextRange.cs
- SaveFileDialog.cs
- TerminatorSinks.cs
- MethodBody.cs
- ControlCollection.cs
- precedingsibling.cs
- ControlEvent.cs
- ParseNumbers.cs
- ConfigsHelper.cs
- DSACryptoServiceProvider.cs
- ClickablePoint.cs
- AsyncResult.cs
- dbenumerator.cs
- IPipelineRuntime.cs
- SoapEnumAttribute.cs
- ExpressionPrinter.cs
- TextWriter.cs
- TextStore.cs
- Propagator.JoinPropagator.cs
- grammarelement.cs
- DataControlPagerLinkButton.cs
- DispatchChannelSink.cs
- AxisAngleRotation3D.cs
- BigInt.cs
- XmlDataImplementation.cs
- RadioButtonBaseAdapter.cs
- WeakEventManager.cs
- ColorTransform.cs
- ComUdtElementCollection.cs
- CodeNamespaceImportCollection.cs
- HttpProfileBase.cs
- HealthMonitoringSectionHelper.cs
- UnsafeNativeMethodsPenimc.cs
- ContextBase.cs
- QueryInterceptorAttribute.cs
- NotSupportedException.cs
- IPEndPoint.cs
- MediaElement.cs
- GatewayDefinition.cs