Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Query / InternalTrees / Rule.cs / 1305376 / Rule.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; namespace System.Data.Query.InternalTrees { ////// A Rule - more specifically, a transformation rule - describes an action that is to /// be taken when a specific kind of subtree is found in the tree /// internal abstract class Rule { ////// The "callback" function for each rule. /// Every callback function must return true if the subtree has /// been modified (or a new subtree has been returned); and must return false /// otherwise. If the root of the subtree has not changed, but some internal details /// of the subtree have changed, it is the responsibility of the rule to update any /// local bookkeeping information. /// /// The rule processing context /// the subtree to operate on /// possibly transformed subtree ///transformation status - true, if there was some change; false otherwise internal delegate bool ProcessNodeDelegate(RuleProcessingContext context, Node subTree, out Node newSubTree); #region private state private ProcessNodeDelegate m_nodeDelegate; private OpType m_opType; #endregion #region Constructors ////// Basic constructor /// /// The OpType we're interested in processing /// The callback to invoke protected Rule(OpType opType, ProcessNodeDelegate nodeProcessDelegate) { Debug.Assert(nodeProcessDelegate != null, "null process delegate"); Debug.Assert(opType != OpType.NotValid, "bad OpType"); Debug.Assert(opType != OpType.Leaf, "bad OpType - Leaf"); m_opType = opType; m_nodeDelegate = nodeProcessDelegate; } #endregion #region protected methods #endregion #region public methods ////// Does the rule match the current node? /// /// the node in question ///true, if a match was found internal abstract bool Match(Node node); ////// We need to invoke the specified callback on the subtree in question - but only /// if the match succeeds /// /// Current rule processing context /// The node (subtree) to process /// the (possibly) modified subtree ///true, if the subtree was modified internal bool Apply(RuleProcessingContext ruleProcessingContext, Node node, out Node newNode) { // invoke the real callback return m_nodeDelegate(ruleProcessingContext, node, out newNode); } ////// The OpType we're interested in transforming /// internal OpType RuleOpType { get { return m_opType; } } #if DEBUG ////// The method name for the rule /// internal string MethodName { get { return m_nodeDelegate.Method.Name; } } #endif #endregion } ////// A SimpleRule is a rule that specifies a specific OpType to look for, and an /// appropriate action to take when such an Op is identified /// internal sealed class SimpleRule : Rule { #region private state #endregion #region constructors ////// Basic constructor. /// /// The OpType we're interested in /// The callback to invoke when we see such an Op internal SimpleRule(OpType opType, ProcessNodeDelegate processDelegate) : base(opType, processDelegate) { } #endregion #region overriden methods internal override bool Match(Node node) { return node.Op.OpType == this.RuleOpType; } #endregion } ////// A PatternMatchRule allows for a pattern to be specified to identify interesting /// subtrees, rather than just an OpType /// internal sealed class PatternMatchRule: Rule { #region private state private Node m_pattern; #endregion #region constructors ////// Basic constructor /// /// The pattern to look for /// The callback to invoke when such a pattern is identified internal PatternMatchRule(Node pattern, ProcessNodeDelegate processDelegate) : base(pattern.Op.OpType, processDelegate) { Debug.Assert(pattern != null, "null pattern"); Debug.Assert(pattern.Op != null, "null pattern Op"); m_pattern = pattern; } #endregion #region private methods private bool Match(Node pattern, Node original) { if (pattern.Op.OpType == OpType.Leaf) return true; if (pattern.Op.OpType != original.Op.OpType) return false; if (pattern.Children.Count != original.Children.Count) return false; for (int i = 0; i < pattern.Children.Count; i++) if (!Match(pattern.Children[i], original.Children[i])) return false; return true; } #endregion #region overridden methods internal override bool Match(Node node) { return Match(m_pattern, node); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
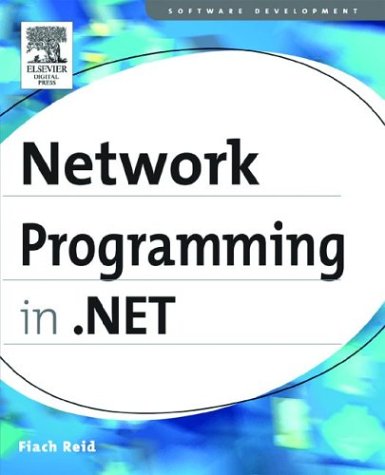
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeUtil.cs
- IItemProperties.cs
- WebPartConnectionsDisconnectVerb.cs
- MessageSecurityProtocol.cs
- ConfigUtil.cs
- DiscoveryDocumentSerializer.cs
- WebBrowser.cs
- GZipStream.cs
- DataObjectMethodAttribute.cs
- CompressStream.cs
- PrimitiveXmlSerializers.cs
- IndexedEnumerable.cs
- HandlerFactoryWrapper.cs
- RSAPKCS1SignatureDeformatter.cs
- UIServiceHelper.cs
- IntegerValidator.cs
- Vector3DCollection.cs
- UserUseLicenseDictionaryLoader.cs
- PenContext.cs
- AmbientValueAttribute.cs
- ApplicationManager.cs
- SoapBinding.cs
- DataGridViewComponentPropertyGridSite.cs
- ContextMenuStrip.cs
- ImageListImageEditor.cs
- Border.cs
- XPathScanner.cs
- ObjRef.cs
- DictionaryKeyPropertyAttribute.cs
- TagPrefixInfo.cs
- EditorPartChrome.cs
- SplitterPanel.cs
- AppSecurityManager.cs
- Container.cs
- DbDataReader.cs
- DataSourceProvider.cs
- GroupAggregateExpr.cs
- Overlapped.cs
- CustomPeerResolverService.cs
- ApplicationBuildProvider.cs
- DefaultSection.cs
- MembershipUser.cs
- FillBehavior.cs
- StructuralObject.cs
- SettingsSavedEventArgs.cs
- LambdaCompiler.Statements.cs
- SqlCachedBuffer.cs
- Compiler.cs
- _emptywebproxy.cs
- URL.cs
- WorkflowDefinitionDispenser.cs
- FontStretch.cs
- ResXBuildProvider.cs
- InputLanguageManager.cs
- CodeGenerator.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- XsdBuildProvider.cs
- StringAnimationBase.cs
- XPathEmptyIterator.cs
- SoapReflectionImporter.cs
- ContextQuery.cs
- QilPatternVisitor.cs
- PointCollectionValueSerializer.cs
- RowToFieldTransformer.cs
- PopOutPanel.cs
- TraceData.cs
- FontFamily.cs
- filewebresponse.cs
- WmlTextBoxAdapter.cs
- EventLogStatus.cs
- SecureStringHasher.cs
- NumberAction.cs
- querybuilder.cs
- InternalBufferOverflowException.cs
- FolderLevelBuildProviderAppliesToAttribute.cs
- WebPartCollection.cs
- PlatformCulture.cs
- ParseChildrenAsPropertiesAttribute.cs
- EventLogLink.cs
- HashCodeCombiner.cs
- InvariantComparer.cs
- ElementsClipboardData.cs
- TargetPerspective.cs
- DateTimeStorage.cs
- Cursors.cs
- SerializationInfo.cs
- ConnectionsZone.cs
- AutoResetEvent.cs
- DictationGrammar.cs
- ToolStripLabel.cs
- RenderData.cs
- TextSpanModifier.cs
- SystemIPGlobalProperties.cs
- TdsParameterSetter.cs
- InlineUIContainer.cs
- IsolationInterop.cs
- SQLDouble.cs
- MonthCalendar.cs
- MessageEncoder.cs
- ModifierKeysConverter.cs