Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / DoubleSumAggregationOperator.cs / 1305376 / DoubleSumAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // DoubleSumAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for doubles. /// internal sealed class DoubleSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal DoubleSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. double sum = 0.0; while (enumerator.MoveNext()) { checked { sum += enumerator.Current; } } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new DoubleSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class DoubleSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal DoubleSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref double currentElement) { double element = default(double); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. double tempSum = 0.0; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); tempSum += element; } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = tempSum; return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
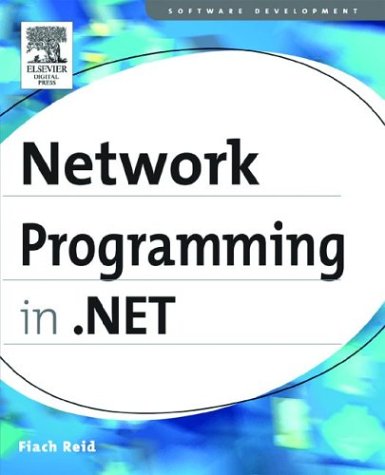
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLDecimal.cs
- BlobPersonalizationState.cs
- TypeRefElement.cs
- HTMLTagNameToTypeMapper.cs
- ProfileSettings.cs
- StoragePropertyMapping.cs
- EpmAttributeNameBuilder.cs
- JsonFormatGeneratorStatics.cs
- RegexCaptureCollection.cs
- CustomCredentialPolicy.cs
- BevelBitmapEffect.cs
- TextBreakpoint.cs
- MatrixTransform.cs
- SystemFonts.cs
- UserControlBuildProvider.cs
- TdsParserHelperClasses.cs
- QilInvoke.cs
- DataReaderContainer.cs
- Polyline.cs
- Propagator.ExtentPlaceholderCreator.cs
- CountdownEvent.cs
- DiscardableAttribute.cs
- XmlSchemaSimpleTypeList.cs
- TypeConverterHelper.cs
- DataBindingHandlerAttribute.cs
- NonClientArea.cs
- SQLSingleStorage.cs
- EntityDataSourceState.cs
- _ListenerRequestStream.cs
- FileSecurity.cs
- counter.cs
- InputMethod.cs
- documentation.cs
- HierarchicalDataTemplate.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- ObjectNotFoundException.cs
- TextSpan.cs
- _IPv6Address.cs
- SmiMetaDataProperty.cs
- PasswordRecoveryAutoFormat.cs
- TextRunTypographyProperties.cs
- ClickablePoint.cs
- DynamicEndpointElement.cs
- BasicExpandProvider.cs
- LinkedDataMemberFieldEditor.cs
- BaseValidatorDesigner.cs
- DecimalConverter.cs
- SendKeys.cs
- IndicFontClient.cs
- InsufficientExecutionStackException.cs
- XPathBinder.cs
- DependencyProperty.cs
- GeneralTransform3D.cs
- DataConnectionHelper.cs
- NameTable.cs
- TransformerInfoCollection.cs
- ScrollBar.cs
- WebPartDescription.cs
- UserUseLicenseDictionaryLoader.cs
- SoapAttributeOverrides.cs
- PrefixHandle.cs
- OracleConnectionFactory.cs
- ObjectDataSourceSelectingEventArgs.cs
- CodeMemberProperty.cs
- ConsoleTraceListener.cs
- WpfSharedXamlSchemaContext.cs
- BitArray.cs
- DataGridViewHitTestInfo.cs
- ScriptComponentDescriptor.cs
- WebWorkflowRole.cs
- WebBrowserNavigatingEventHandler.cs
- XmlAutoDetectWriter.cs
- WpfXamlMember.cs
- HttpListenerRequest.cs
- NameSpaceEvent.cs
- DynamicMethod.cs
- TextServicesManager.cs
- SafeEventLogWriteHandle.cs
- ExpressionBuilder.cs
- AttributeEmitter.cs
- TextTreeTextNode.cs
- odbcmetadatacollectionnames.cs
- Token.cs
- LocalBuilder.cs
- CodeEntryPointMethod.cs
- RuleEngine.cs
- StylusTip.cs
- WebPartsPersonalization.cs
- SafeFileMappingHandle.cs
- XPathDocumentIterator.cs
- HwndProxyElementProvider.cs
- XPathPatternBuilder.cs
- ProviderBase.cs
- CorrelationManager.cs
- DataRowCollection.cs
- ProvidePropertyAttribute.cs
- XmlIgnoreAttribute.cs
- CompilationSection.cs
- VarRemapper.cs
- ExpressionTable.cs