Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Reflection / Emit / EventBuilder.cs / 1305376 / EventBuilder.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: EventBuilder ** **[....] ** ** ** Eventbuilder is for client to define eevnts for a class ** ** ===========================================================*/ namespace System.Reflection.Emit { using System; using System.Reflection; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Diagnostics.Contracts; // // A EventBuilder is always associated with a TypeBuilder. The TypeBuilder.DefineEvent // method will return a new EventBuilder to a client. // [HostProtection(MayLeakOnAbort = true)] [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_EventBuilder))] [System.Runtime.InteropServices.ComVisible(true)] public sealed class EventBuilder : _EventBuilder { // Make a private constructor so these cannot be constructed externally. private EventBuilder() {} // Constructs a EventBuilder. // internal EventBuilder( ModuleBuilder mod, // the module containing this EventBuilder String name, // Event name EventAttributes attr, // event attribute such as Public, Private, and Protected defined above //int eventType, // event type TypeBuilder type, // containing type EventToken evToken) { m_name = name; m_module = mod; m_attributes = attr; m_evToken = evToken; m_type = type; } // Return the Token for this event within the TypeBuilder that the // event is defined within. public EventToken GetEventToken() { return m_evToken; } [System.Security.SecurityCritical] // auto-generated private void SetMethodSemantics(MethodBuilder mdBuilder, MethodSemanticsAttributes semantics) { if (mdBuilder == null) { throw new ArgumentNullException("mdBuilder"); } Contract.EndContractBlock(); m_type.ThrowIfCreated(); TypeBuilder.DefineMethodSemantics( m_module.GetNativeHandle(), m_evToken.Token, semantics, mdBuilder.GetToken().Token); } [System.Security.SecuritySafeCritical] // auto-generated public void SetAddOnMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.AddOn); } [System.Security.SecuritySafeCritical] // auto-generated public void SetRemoveOnMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.RemoveOn); } [System.Security.SecuritySafeCritical] // auto-generated public void SetRaiseMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.Fire); } [System.Security.SecuritySafeCritical] // auto-generated public void AddOtherMethod(MethodBuilder mdBuilder) { SetMethodSemantics(mdBuilder, MethodSemanticsAttributes.Other); } // Use this function if client decides to form the custom attribute blob themselves [System.Security.SecuritySafeCritical] // auto-generated [System.Runtime.InteropServices.ComVisible(true)] public void SetCustomAttribute(ConstructorInfo con, byte[] binaryAttribute) { if (con == null) throw new ArgumentNullException("con"); if (binaryAttribute == null) throw new ArgumentNullException("binaryAttribute"); Contract.EndContractBlock(); m_type.ThrowIfCreated(); TypeBuilder.DefineCustomAttribute( m_module, m_evToken.Token, m_module.GetConstructorToken(con).Token, binaryAttribute, false, false); } // Use this function if client wishes to build CustomAttribute using CustomAttributeBuilder [System.Security.SecuritySafeCritical] // auto-generated public void SetCustomAttribute(CustomAttributeBuilder customBuilder) { if (customBuilder == null) { throw new ArgumentNullException("customBuilder"); } Contract.EndContractBlock(); m_type.ThrowIfCreated(); customBuilder.CreateCustomAttribute(m_module, m_evToken.Token); } void _EventBuilder.GetTypeInfoCount(out uint pcTInfo) { throw new NotImplementedException(); } void _EventBuilder.GetTypeInfo(uint iTInfo, uint lcid, IntPtr ppTInfo) { throw new NotImplementedException(); } void _EventBuilder.GetIDsOfNames([In] ref Guid riid, IntPtr rgszNames, uint cNames, uint lcid, IntPtr rgDispId) { throw new NotImplementedException(); } void _EventBuilder.Invoke(uint dispIdMember, [In] ref Guid riid, uint lcid, short wFlags, IntPtr pDispParams, IntPtr pVarResult, IntPtr pExcepInfo, IntPtr puArgErr) { throw new NotImplementedException(); } // These are package private so that TypeBuilder can access them. private String m_name; // The name of the event private EventToken m_evToken; // The token of this event private ModuleBuilder m_module; private EventAttributes m_attributes; private TypeBuilder m_type; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
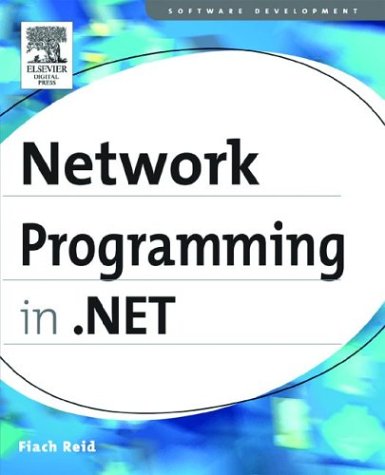
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScaleTransform.cs
- OptimisticConcurrencyException.cs
- ToolStripPanelRow.cs
- PasswordRecovery.cs
- objectquery_tresulttype.cs
- TableCellsCollectionEditor.cs
- BooleanFacetDescriptionElement.cs
- Baml2006Reader.cs
- SR.Designer.cs
- ValidationRule.cs
- HttpHandler.cs
- TreeNodeBindingCollection.cs
- FilterException.cs
- TableItemStyle.cs
- CommandExpr.cs
- OuterGlowBitmapEffect.cs
- ResponseStream.cs
- ClientApiGenerator.cs
- FieldAccessException.cs
- ResourcesChangeInfo.cs
- StylusPoint.cs
- GeneralTransform2DTo3D.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- ISAPIWorkerRequest.cs
- ContentType.cs
- SymbolMethod.cs
- DbConnectionInternal.cs
- _LazyAsyncResult.cs
- FormatterServices.cs
- CheckBoxList.cs
- SapiRecognizer.cs
- TableRowCollection.cs
- _ListenerAsyncResult.cs
- XPathChildIterator.cs
- SplitterEvent.cs
- RegionData.cs
- BasicSecurityProfileVersion.cs
- PersonalizationDictionary.cs
- Int32Storage.cs
- Overlapped.cs
- ScalarOps.cs
- DataListDesigner.cs
- HttpCookiesSection.cs
- MetricEntry.cs
- TextElementEditingBehaviorAttribute.cs
- RemoteWebConfigurationHostStream.cs
- ExtensionQuery.cs
- Thread.cs
- ellipse.cs
- TypeLoadException.cs
- TagElement.cs
- StrokeDescriptor.cs
- WebPartVerbCollection.cs
- WeakEventManager.cs
- Parameter.cs
- BitmapFrameEncode.cs
- DeviceContext.cs
- __Filters.cs
- Geometry.cs
- SqlNode.cs
- ReservationNotFoundException.cs
- CryptoHelper.cs
- XPathPatternParser.cs
- Span.cs
- DispatchWrapper.cs
- ConstraintStruct.cs
- SerializationTrace.cs
- VisualStyleRenderer.cs
- UidManager.cs
- SplitterCancelEvent.cs
- Validator.cs
- EdmType.cs
- EntityCommand.cs
- SiteMapDataSource.cs
- WebPartManagerInternals.cs
- __Error.cs
- XmlILIndex.cs
- OracleLob.cs
- XsltException.cs
- ApplicationException.cs
- BitmapEffectvisualstate.cs
- ChannelFactory.cs
- TypeResolvingOptions.cs
- ListSourceHelper.cs
- RootBrowserWindowAutomationPeer.cs
- ProtocolInformationWriter.cs
- UpdateCommandGenerator.cs
- SafeFileMapViewHandle.cs
- SymbolPair.cs
- ToolTipAutomationPeer.cs
- PhysicalOps.cs
- ExpressionList.cs
- BadImageFormatException.cs
- ResourceDescriptionAttribute.cs
- IsolatedStorage.cs
- ResourceReader.cs
- KeyPressEvent.cs
- AssociationTypeEmitter.cs
- DoubleAnimationBase.cs
- _FixedSizeReader.cs