Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / ValueExpressions.cs / 1305376 / ValueExpressions.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Represents a constant value. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbConstantExpression : DbExpression { private readonly PrimitiveTypeKind _constantKind; private readonly object _value; internal DbConstantExpression(TypeUsage resultType, object value) : base(DbExpressionKind.Constant, resultType) { Debug.Assert(value != null, "DbConstantExpression value cannot be null"); Debug.Assert(TypeSemantics.IsPrimitiveType(resultType), "DbConstantExpression must have a primitive value"); this._constantKind = TypeHelpers.GetEdmType(resultType).PrimitiveTypeKind; if (this._constantKind == PrimitiveTypeKind.Binary) { // DevDiv#480416: DbConstantExpression with a binary value is not fully immutable // this._value = ((byte[])value).Clone(); } else { this._value = value; } } /// /// Provides direct access to the constant value, even for byte[] constants. /// ///The object value contained by this constant expression, not a copy. internal object GetValue() { return this._value; } ////// Gets the constant value. /// public object Value { get { // DevDiv#480416: DbConstantExpression with a binary value is not fully immutable // if (this._constantKind == PrimitiveTypeKind.Binary) { return ((byte[])_value).Clone(); } else { return _value; } } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents null. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbNullExpression : DbExpression { internal DbNullExpression(TypeUsage type) : base(DbExpressionKind.Null, type) { } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a reference to a variable that is currently in scope. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbVariableReferenceExpression : DbExpression { private readonly string _name; internal DbVariableReferenceExpression(TypeUsage type, string name) : base(DbExpressionKind.VariableReference, type) { Debug.Assert(name != null, "DbVariableReferenceExpression Name cannot be null"); this._name = name; } ////// Gets the name of the referenced variable. /// public string VariableName { get { return _name; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a reference to a parameter declared on the command tree that contains this expression. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbParameterReferenceExpression : DbExpression { private readonly string _name; internal DbParameterReferenceExpression(TypeUsage type, string name) : base(DbExpressionKind.ParameterReference, type) { Debug.Assert(DbCommandTree.IsValidParameterName(name), "DbParameterReferenceExpression name should be valid"); this._name = name; } ////// Gets the name of the referenced parameter. /// public string ParameterName { get { return _name; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of a static or instance property. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbPropertyExpression : DbExpression { private readonly EdmMember _property; private readonly DbExpression _instance; internal DbPropertyExpression(TypeUsage resultType, EdmMember property, DbExpression instance) : base(DbExpressionKind.Property, resultType) { Debug.Assert(property != null, "DbPropertyExpression property cannot be null"); Debug.Assert(instance != null, "DbPropertyExpression instance cannot be null"); Debug.Assert(Helper.IsEdmProperty(property) || Helper.IsRelationshipEndMember(property) || Helper.IsNavigationProperty(property), "DbExpression property must be a property, navigation property, or relationship end"); this._property = property; this._instance = instance; } ////// Gets the property metadata for the property to retrieve. /// public EdmMember Property { get { return _property; } } ////// Gets the public DbExpression Instance { get { return _instance; } } ///that defines the instance from which the property should be retrieved. /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// /// Creates a new KeyValuePair<string, DbExpression> based on this property expression. /// The string key will be the name of the referenced property, while the DbExpression value will be the property expression itself. /// ///A new KeyValuePair<string, DbExpression> with key and value derived from the DbPropertyExpression public KeyValuePairToKeyValuePair() { return new KeyValuePair (this.Property.Name, this); } public static implicit operator KeyValuePair (DbPropertyExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return value.ToKeyValuePair(); } } /// /// Represents the invocation of a function. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbFunctionExpression : DbExpression { private readonly EdmFunction _functionInfo; private readonly DbExpressionList _arguments; internal DbFunctionExpression(TypeUsage resultType, EdmFunction function, DbExpressionList arguments) : base(DbExpressionKind.Function, resultType) { Debug.Assert(function != null, "DbFunctionExpression function cannot be null"); Debug.Assert(arguments != null, "DbFunctionExpression arguments cannot be null"); Debug.Assert(object.ReferenceEquals(resultType, function.ReturnParameter.TypeUsage), "DbFunctionExpression result type must be function return type"); this._functionInfo = function; this._arguments = arguments; } ////// Gets the metadata for the function to invoke. /// public EdmFunction Function { get { return _functionInfo; } } ////// Gets an public IListlist that provides the arguments to the function. /// Arguments { get { return this._arguments; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the application of a Lambda function. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbLambdaExpression : DbExpression { private readonly DbLambda _lambda; private readonly DbExpressionList _arguments; internal DbLambdaExpression(TypeUsage resultType, DbLambda lambda, DbExpressionList args) : base(DbExpressionKind.Lambda, resultType) { Debug.Assert(lambda != null, "DbLambdaExpression lambda cannot be null"); Debug.Assert(args != null, "DbLambdaExpression arguments cannot be null"); Debug.Assert(object.ReferenceEquals(resultType, lambda.Body.ResultType), "DbLambdaExpression result type must be Lambda body result type"); Debug.Assert(lambda.Variables.Count == args.Count, "DbLambdaExpression argument count does not match Lambda parameter count"); this._lambda = lambda; this._arguments = args; } ////// Gets the public DbLambda Lambda { get { return _lambda; } } ///representing the Lambda function applied by this expression. /// /// Gets a public IListlist that provides the arguments to which the Lambda function should be applied. /// Arguments { get { return this._arguments; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } #if METHOD_EXPRESSION /// /// Represents the invocation of a method. /// public sealed class MethodExpression : Expression { MethodMetadata m_methodInfo; IListm_args; ExpressionLink m_inst; internal MethodExpression(CommandTree cmdTree, MethodMetadata methodInfo, IList args, Expression instance) : base(cmdTree, ExpressionKind.Method) { // // Ensure that the property metadata is non-null and from the same metadata workspace and dataspace as the command tree. // CommandTreeTypeHelper.CheckMember(methodInfo, "method", "methodInfo"); // // Methods that return void are not allowed // if (cmdTree.TypeHelper.IsNullOrNullType(methodInfo.Type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Method_VoidResultInvalid, "methodInfo"); } if (null == args) { throw EntityUtil.ArgumentNull("args"); } this.m_inst = new ExpressionLink("Instance", cmdTree); // // Validate the instance // if (methodInfo.IsStatic) { if (instance != null) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Method_InstanceInvalidForStatic, "instance"); } } else { if (null == instance) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Method_InstanceRequiredForInstance, "instance"); } this.m_inst.SetExpectedType(methodInfo.DefiningType); this.m_inst.InitializeValue(instance); } // // Validate the arguments // m_args = new ExpressionList("Arguments", cmdTree, methodInfo.Parameters, args); m_methodInfo = methodInfo; this.ResultType = methodInfo.Type; } /// /// Gets the metadata for the method to invoke. /// public MethodMetadata Method { get { return m_methodInfo; } } ////// Gets the expressions that provide the arguments to the method. /// public IListArguments { get { return m_args; } } /// /// Gets or sets an ///that defines the instance on which the method should be invoked. Must be null for instance methods. /// For static properties, Instance
will always be null, and attempting to set a new value will result /// in. /// The expression is null ///The method is static ////// The expression is not associated with the MethodExpression's command tree, /// or its result type is not equal or promotable to the type that defines the method /// public Expression Instance { get { return m_inst.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { if (this.m_methodInfo.IsStatic) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.Cqt_Method_InstanceInvalidForStatic); } this.m_inst.Expression = value; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of ExpressionVisitor. ///public override void Accept(ExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed ExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } #endif /// /// Represents the navigation of a (composition or association) relationship given the 'from' role, the 'to' role and an instance of the from role /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbRelationshipNavigationExpression : DbExpression { private readonly RelationshipType _relation; private readonly RelationshipEndMember _fromRole; private readonly RelationshipEndMember _toRole; private readonly DbExpression _from; internal DbRelationshipNavigationExpression( TypeUsage resultType, RelationshipType relType, RelationshipEndMember fromEnd, RelationshipEndMember toEnd, DbExpression navigateFrom) : base(DbExpressionKind.RelationshipNavigation, resultType) { Debug.Assert(relType != null, "DbRelationshipNavigationExpression relationship type cannot be null"); Debug.Assert(fromEnd != null, "DbRelationshipNavigationExpression 'from' end cannot be null"); Debug.Assert(toEnd != null, "DbRelationshipNavigationExpression 'to' end cannot be null"); Debug.Assert(navigateFrom != null, "DbRelationshipNavigationExpression navigation source cannot be null"); this._relation = relType; this._fromRole = fromEnd; this._toRole = toEnd; this._from = navigateFrom; } ////// Gets the metadata for the relationship over which navigation occurs /// public RelationshipType Relationship { get { return _relation; } } ////// Gets the metadata for the relationship end to navigate from /// public RelationshipEndMember NavigateFrom { get { return _fromRole; } } ////// Gets the metadata for the relationship end to navigate to /// public RelationshipEndMember NavigateTo { get { return _toRole; } } ////// Gets the public DbExpression NavigationSource { get { return _from; } } ///that specifies the instance of the 'from' relationship end from which navigation should occur. /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Encapsulates the result (represented as a Ref to the resulting Entity) of navigating from /// the specified source end of a relationship to the specified target end. This class is intended /// for use only with [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] internal sealed class DbRelatedEntityRef { private readonly RelationshipEndMember _sourceEnd; private readonly RelationshipEndMember _targetEnd; private readonly DbExpression _targetEntityRef; internal DbRelatedEntityRef(RelationshipEndMember sourceEnd, RelationshipEndMember targetEnd, DbExpression targetEntityRef) { // Validate that the specified relationship ends are: // 1. Non-null // 2. From the same metadata workspace as that used by the command tree EntityUtil.CheckArgumentNull(sourceEnd, "sourceEnd"); EntityUtil.CheckArgumentNull(targetEnd, "targetEnd"); // Validate that the specified target entity ref is: // 1. Non-null EntityUtil.CheckArgumentNull(targetEntityRef, "targetEntityRef"); // Validate that the specified source and target ends are: // 1. Declared by the same relationship type if (!object.ReferenceEquals(sourceEnd.DeclaringType, targetEnd.DeclaringType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEndFromDifferentRelationship, "targetEnd"); } // 2. Not the same end if (object.ReferenceEquals(sourceEnd, targetEnd)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEndSameAsSourceEnd, "targetEnd"); } // Validate that the specified target end has multiplicity of at most one if (targetEnd.RelationshipMultiplicity != RelationshipMultiplicity.One && targetEnd.RelationshipMultiplicity != RelationshipMultiplicity.ZeroOrOne) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEndMustBeAtMostOne, "targetEnd"); } // Validate that the specified target entity ref actually has a ref result type if (!TypeSemantics.IsReferenceType(targetEntityRef.ResultType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEntityNotRef, "targetEntityRef"); } // Validate that the specified target entity is of a type that can be reached by navigating to the specified relationship end EntityTypeBase endType = TypeHelpers.GetEdmType, where an 'owning' instance of that class /// represents the source Entity involved in the relationship navigation. /// Instances of DbRelatedEntityRef may be specified when creating a that /// constructs an Entity, allowing information about Entities that are related to the newly constructed Entity to be captured. /// (targetEnd.TypeUsage).ElementType; EntityTypeBase targetType = TypeHelpers.GetEdmType (targetEntityRef.ResultType).ElementType; // if (!endType.EdmEquals(targetType) && !TypeSemantics.IsSubTypeOf(targetType, endType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEntityNotCompatible, "targetEntityRef"); } // Validation succeeded, initialize state _targetEntityRef = targetEntityRef; _targetEnd = targetEnd; _sourceEnd = sourceEnd; } /// /// Retrieves the 'source' end of the relationship navigation satisfied by this related entity Ref /// internal RelationshipEndMember SourceEnd { get { return _sourceEnd; } } ////// Retrieves the 'target' end of the relationship navigation satisfied by this related entity Ref /// internal RelationshipEndMember TargetEnd { get { return _targetEnd; } } ////// Retrieves the entity Ref that is the result of navigating from the source to the target end of this related entity Ref /// internal DbExpression TargetEntityReference { get { return _targetEntityRef; } } } ////// Represents the construction of a new instance of a given type, including set and record types. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbNewInstanceExpression : DbExpression { private readonly DbExpressionList _elements; private readonly System.Collections.ObjectModel.ReadOnlyCollection_relatedEntityRefs; internal DbNewInstanceExpression(TypeUsage type, DbExpressionList args) : base(DbExpressionKind.NewInstance, type) { Debug.Assert(args != null, "DbNewInstanceExpression arguments cannot be null"); Debug.Assert(args.Count > 0 || TypeSemantics.IsCollectionType(type), "DbNewInstanceExpression requires at least one argument when not creating an empty collection"); this._elements = args; } internal DbNewInstanceExpression(TypeUsage resultType, DbExpressionList attributeValues, System.Collections.ObjectModel.ReadOnlyCollection relationships) : this(resultType, attributeValues) { Debug.Assert(TypeSemantics.IsEntityType(resultType), "An entity type is required to create a NewEntityWithRelationships expression"); Debug.Assert(relationships != null, "Related entity ref collection cannot be null"); this._relatedEntityRefs = (relationships.Count > 0 ? relationships : null); } /// /// Gets an public IListlist that provides the property/column values or set elements for the new instance. /// Arguments { get { return _elements; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } internal bool HasRelatedEntityReferences { get { return (_relatedEntityRefs != null); } } /// /// Gets the related entity references (if any) for an entity constructor. /// May be null if no related entities were specified - use the internal System.Collections.ObjectModel.ReadOnlyCollectionproperty to determine this. /// RelatedEntityReferences { get { return _relatedEntityRefs; } } } /// /// Represents a (strongly typed) reference to a specific instance within a given entity set. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbRefExpression : DbUnaryExpression { private readonly EntitySet _entitySet; internal DbRefExpression(TypeUsage refResultType, EntitySet entitySet, DbExpression refKeys) : base(DbExpressionKind.Ref, refResultType, refKeys) { Debug.Assert(TypeSemantics.IsReferenceType(refResultType), "DbRefExpression requires a reference result type"); this._entitySet = entitySet; } ////// Gets the metadata for the entity set that contains the instance. /// public EntitySet EntitySet { get { return _entitySet; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of a given entity using the specified Ref. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Deref"), System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbDerefExpression : DbUnaryExpression { internal DbDerefExpression(TypeUsage entityResultType, DbExpression refExpr) : base(DbExpressionKind.Deref, entityResultType, refExpr) { Debug.Assert(TypeSemantics.IsEntityType(entityResultType), "DbDerefExpression requires an entity result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a 'scan' of all elements of a given entity set. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbScanExpression : DbExpression { private readonly EntitySetBase _targetSet; internal DbScanExpression(TypeUsage collectionOfEntityType, EntitySetBase entitySet) : base(DbExpressionKind.Scan, collectionOfEntityType) { Debug.Assert(entitySet != null, "DbScanExpression entity set cannot be null"); this._targetSet = entitySet; } ////// Gets the metadata for the referenced entity or relationship set. /// public EntitySetBase Target { get { return _targetSet; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Represents a constant value. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbConstantExpression : DbExpression { private readonly PrimitiveTypeKind _constantKind; private readonly object _value; internal DbConstantExpression(TypeUsage resultType, object value) : base(DbExpressionKind.Constant, resultType) { Debug.Assert(value != null, "DbConstantExpression value cannot be null"); Debug.Assert(TypeSemantics.IsPrimitiveType(resultType), "DbConstantExpression must have a primitive value"); this._constantKind = TypeHelpers.GetEdmType(resultType).PrimitiveTypeKind; if (this._constantKind == PrimitiveTypeKind.Binary) { // DevDiv#480416: DbConstantExpression with a binary value is not fully immutable // this._value = ((byte[])value).Clone(); } else { this._value = value; } } /// /// Provides direct access to the constant value, even for byte[] constants. /// ///The object value contained by this constant expression, not a copy. internal object GetValue() { return this._value; } ////// Gets the constant value. /// public object Value { get { // DevDiv#480416: DbConstantExpression with a binary value is not fully immutable // if (this._constantKind == PrimitiveTypeKind.Binary) { return ((byte[])_value).Clone(); } else { return _value; } } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents null. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbNullExpression : DbExpression { internal DbNullExpression(TypeUsage type) : base(DbExpressionKind.Null, type) { } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a reference to a variable that is currently in scope. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbVariableReferenceExpression : DbExpression { private readonly string _name; internal DbVariableReferenceExpression(TypeUsage type, string name) : base(DbExpressionKind.VariableReference, type) { Debug.Assert(name != null, "DbVariableReferenceExpression Name cannot be null"); this._name = name; } ////// Gets the name of the referenced variable. /// public string VariableName { get { return _name; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a reference to a parameter declared on the command tree that contains this expression. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbParameterReferenceExpression : DbExpression { private readonly string _name; internal DbParameterReferenceExpression(TypeUsage type, string name) : base(DbExpressionKind.ParameterReference, type) { Debug.Assert(DbCommandTree.IsValidParameterName(name), "DbParameterReferenceExpression name should be valid"); this._name = name; } ////// Gets the name of the referenced parameter. /// public string ParameterName { get { return _name; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of a static or instance property. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbPropertyExpression : DbExpression { private readonly EdmMember _property; private readonly DbExpression _instance; internal DbPropertyExpression(TypeUsage resultType, EdmMember property, DbExpression instance) : base(DbExpressionKind.Property, resultType) { Debug.Assert(property != null, "DbPropertyExpression property cannot be null"); Debug.Assert(instance != null, "DbPropertyExpression instance cannot be null"); Debug.Assert(Helper.IsEdmProperty(property) || Helper.IsRelationshipEndMember(property) || Helper.IsNavigationProperty(property), "DbExpression property must be a property, navigation property, or relationship end"); this._property = property; this._instance = instance; } ////// Gets the property metadata for the property to retrieve. /// public EdmMember Property { get { return _property; } } ////// Gets the public DbExpression Instance { get { return _instance; } } ///that defines the instance from which the property should be retrieved. /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// /// Creates a new KeyValuePair<string, DbExpression> based on this property expression. /// The string key will be the name of the referenced property, while the DbExpression value will be the property expression itself. /// ///A new KeyValuePair<string, DbExpression> with key and value derived from the DbPropertyExpression public KeyValuePairToKeyValuePair() { return new KeyValuePair (this.Property.Name, this); } public static implicit operator KeyValuePair (DbPropertyExpression value) { EntityUtil.CheckArgumentNull(value, "value"); return value.ToKeyValuePair(); } } /// /// Represents the invocation of a function. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbFunctionExpression : DbExpression { private readonly EdmFunction _functionInfo; private readonly DbExpressionList _arguments; internal DbFunctionExpression(TypeUsage resultType, EdmFunction function, DbExpressionList arguments) : base(DbExpressionKind.Function, resultType) { Debug.Assert(function != null, "DbFunctionExpression function cannot be null"); Debug.Assert(arguments != null, "DbFunctionExpression arguments cannot be null"); Debug.Assert(object.ReferenceEquals(resultType, function.ReturnParameter.TypeUsage), "DbFunctionExpression result type must be function return type"); this._functionInfo = function; this._arguments = arguments; } ////// Gets the metadata for the function to invoke. /// public EdmFunction Function { get { return _functionInfo; } } ////// Gets an public IListlist that provides the arguments to the function. /// Arguments { get { return this._arguments; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the application of a Lambda function. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbLambdaExpression : DbExpression { private readonly DbLambda _lambda; private readonly DbExpressionList _arguments; internal DbLambdaExpression(TypeUsage resultType, DbLambda lambda, DbExpressionList args) : base(DbExpressionKind.Lambda, resultType) { Debug.Assert(lambda != null, "DbLambdaExpression lambda cannot be null"); Debug.Assert(args != null, "DbLambdaExpression arguments cannot be null"); Debug.Assert(object.ReferenceEquals(resultType, lambda.Body.ResultType), "DbLambdaExpression result type must be Lambda body result type"); Debug.Assert(lambda.Variables.Count == args.Count, "DbLambdaExpression argument count does not match Lambda parameter count"); this._lambda = lambda; this._arguments = args; } ////// Gets the public DbLambda Lambda { get { return _lambda; } } ///representing the Lambda function applied by this expression. /// /// Gets a public IListlist that provides the arguments to which the Lambda function should be applied. /// Arguments { get { return this._arguments; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } #if METHOD_EXPRESSION /// /// Represents the invocation of a method. /// public sealed class MethodExpression : Expression { MethodMetadata m_methodInfo; IListm_args; ExpressionLink m_inst; internal MethodExpression(CommandTree cmdTree, MethodMetadata methodInfo, IList args, Expression instance) : base(cmdTree, ExpressionKind.Method) { // // Ensure that the property metadata is non-null and from the same metadata workspace and dataspace as the command tree. // CommandTreeTypeHelper.CheckMember(methodInfo, "method", "methodInfo"); // // Methods that return void are not allowed // if (cmdTree.TypeHelper.IsNullOrNullType(methodInfo.Type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Method_VoidResultInvalid, "methodInfo"); } if (null == args) { throw EntityUtil.ArgumentNull("args"); } this.m_inst = new ExpressionLink("Instance", cmdTree); // // Validate the instance // if (methodInfo.IsStatic) { if (instance != null) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Method_InstanceInvalidForStatic, "instance"); } } else { if (null == instance) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Method_InstanceRequiredForInstance, "instance"); } this.m_inst.SetExpectedType(methodInfo.DefiningType); this.m_inst.InitializeValue(instance); } // // Validate the arguments // m_args = new ExpressionList("Arguments", cmdTree, methodInfo.Parameters, args); m_methodInfo = methodInfo; this.ResultType = methodInfo.Type; } /// /// Gets the metadata for the method to invoke. /// public MethodMetadata Method { get { return m_methodInfo; } } ////// Gets the expressions that provide the arguments to the method. /// public IListArguments { get { return m_args; } } /// /// Gets or sets an ///that defines the instance on which the method should be invoked. Must be null for instance methods. /// For static properties, Instance
will always be null, and attempting to set a new value will result /// in. /// The expression is null ///The method is static ////// The expression is not associated with the MethodExpression's command tree, /// or its result type is not equal or promotable to the type that defines the method /// public Expression Instance { get { return m_inst.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { if (this.m_methodInfo.IsStatic) { throw EntityUtil.NotSupported(System.Data.Entity.Strings.Cqt_Method_InstanceInvalidForStatic); } this.m_inst.Expression = value; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of ExpressionVisitor. ///public override void Accept(ExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed ExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } #endif /// /// Represents the navigation of a (composition or association) relationship given the 'from' role, the 'to' role and an instance of the from role /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbRelationshipNavigationExpression : DbExpression { private readonly RelationshipType _relation; private readonly RelationshipEndMember _fromRole; private readonly RelationshipEndMember _toRole; private readonly DbExpression _from; internal DbRelationshipNavigationExpression( TypeUsage resultType, RelationshipType relType, RelationshipEndMember fromEnd, RelationshipEndMember toEnd, DbExpression navigateFrom) : base(DbExpressionKind.RelationshipNavigation, resultType) { Debug.Assert(relType != null, "DbRelationshipNavigationExpression relationship type cannot be null"); Debug.Assert(fromEnd != null, "DbRelationshipNavigationExpression 'from' end cannot be null"); Debug.Assert(toEnd != null, "DbRelationshipNavigationExpression 'to' end cannot be null"); Debug.Assert(navigateFrom != null, "DbRelationshipNavigationExpression navigation source cannot be null"); this._relation = relType; this._fromRole = fromEnd; this._toRole = toEnd; this._from = navigateFrom; } ////// Gets the metadata for the relationship over which navigation occurs /// public RelationshipType Relationship { get { return _relation; } } ////// Gets the metadata for the relationship end to navigate from /// public RelationshipEndMember NavigateFrom { get { return _fromRole; } } ////// Gets the metadata for the relationship end to navigate to /// public RelationshipEndMember NavigateTo { get { return _toRole; } } ////// Gets the public DbExpression NavigationSource { get { return _from; } } ///that specifies the instance of the 'from' relationship end from which navigation should occur. /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Encapsulates the result (represented as a Ref to the resulting Entity) of navigating from /// the specified source end of a relationship to the specified target end. This class is intended /// for use only with [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] internal sealed class DbRelatedEntityRef { private readonly RelationshipEndMember _sourceEnd; private readonly RelationshipEndMember _targetEnd; private readonly DbExpression _targetEntityRef; internal DbRelatedEntityRef(RelationshipEndMember sourceEnd, RelationshipEndMember targetEnd, DbExpression targetEntityRef) { // Validate that the specified relationship ends are: // 1. Non-null // 2. From the same metadata workspace as that used by the command tree EntityUtil.CheckArgumentNull(sourceEnd, "sourceEnd"); EntityUtil.CheckArgumentNull(targetEnd, "targetEnd"); // Validate that the specified target entity ref is: // 1. Non-null EntityUtil.CheckArgumentNull(targetEntityRef, "targetEntityRef"); // Validate that the specified source and target ends are: // 1. Declared by the same relationship type if (!object.ReferenceEquals(sourceEnd.DeclaringType, targetEnd.DeclaringType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEndFromDifferentRelationship, "targetEnd"); } // 2. Not the same end if (object.ReferenceEquals(sourceEnd, targetEnd)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEndSameAsSourceEnd, "targetEnd"); } // Validate that the specified target end has multiplicity of at most one if (targetEnd.RelationshipMultiplicity != RelationshipMultiplicity.One && targetEnd.RelationshipMultiplicity != RelationshipMultiplicity.ZeroOrOne) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEndMustBeAtMostOne, "targetEnd"); } // Validate that the specified target entity ref actually has a ref result type if (!TypeSemantics.IsReferenceType(targetEntityRef.ResultType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEntityNotRef, "targetEntityRef"); } // Validate that the specified target entity is of a type that can be reached by navigating to the specified relationship end EntityTypeBase endType = TypeHelpers.GetEdmType, where an 'owning' instance of that class /// represents the source Entity involved in the relationship navigation. /// Instances of DbRelatedEntityRef may be specified when creating a that /// constructs an Entity, allowing information about Entities that are related to the newly constructed Entity to be captured. /// (targetEnd.TypeUsage).ElementType; EntityTypeBase targetType = TypeHelpers.GetEdmType (targetEntityRef.ResultType).ElementType; // if (!endType.EdmEquals(targetType) && !TypeSemantics.IsSubTypeOf(targetType, endType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_RelatedEntityRef_TargetEntityNotCompatible, "targetEntityRef"); } // Validation succeeded, initialize state _targetEntityRef = targetEntityRef; _targetEnd = targetEnd; _sourceEnd = sourceEnd; } /// /// Retrieves the 'source' end of the relationship navigation satisfied by this related entity Ref /// internal RelationshipEndMember SourceEnd { get { return _sourceEnd; } } ////// Retrieves the 'target' end of the relationship navigation satisfied by this related entity Ref /// internal RelationshipEndMember TargetEnd { get { return _targetEnd; } } ////// Retrieves the entity Ref that is the result of navigating from the source to the target end of this related entity Ref /// internal DbExpression TargetEntityReference { get { return _targetEntityRef; } } } ////// Represents the construction of a new instance of a given type, including set and record types. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbNewInstanceExpression : DbExpression { private readonly DbExpressionList _elements; private readonly System.Collections.ObjectModel.ReadOnlyCollection_relatedEntityRefs; internal DbNewInstanceExpression(TypeUsage type, DbExpressionList args) : base(DbExpressionKind.NewInstance, type) { Debug.Assert(args != null, "DbNewInstanceExpression arguments cannot be null"); Debug.Assert(args.Count > 0 || TypeSemantics.IsCollectionType(type), "DbNewInstanceExpression requires at least one argument when not creating an empty collection"); this._elements = args; } internal DbNewInstanceExpression(TypeUsage resultType, DbExpressionList attributeValues, System.Collections.ObjectModel.ReadOnlyCollection relationships) : this(resultType, attributeValues) { Debug.Assert(TypeSemantics.IsEntityType(resultType), "An entity type is required to create a NewEntityWithRelationships expression"); Debug.Assert(relationships != null, "Related entity ref collection cannot be null"); this._relatedEntityRefs = (relationships.Count > 0 ? relationships : null); } /// /// Gets an public IListlist that provides the property/column values or set elements for the new instance. /// Arguments { get { return _elements; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } internal bool HasRelatedEntityReferences { get { return (_relatedEntityRefs != null); } } /// /// Gets the related entity references (if any) for an entity constructor. /// May be null if no related entities were specified - use the internal System.Collections.ObjectModel.ReadOnlyCollectionproperty to determine this. /// RelatedEntityReferences { get { return _relatedEntityRefs; } } } /// /// Represents a (strongly typed) reference to a specific instance within a given entity set. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbRefExpression : DbUnaryExpression { private readonly EntitySet _entitySet; internal DbRefExpression(TypeUsage refResultType, EntitySet entitySet, DbExpression refKeys) : base(DbExpressionKind.Ref, refResultType, refKeys) { Debug.Assert(TypeSemantics.IsReferenceType(refResultType), "DbRefExpression requires a reference result type"); this._entitySet = entitySet; } ////// Gets the metadata for the entity set that contains the instance. /// public EntitySet EntitySet { get { return _entitySet; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of a given entity using the specified Ref. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Deref"), System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbDerefExpression : DbUnaryExpression { internal DbDerefExpression(TypeUsage entityResultType, DbExpression refExpr) : base(DbExpressionKind.Deref, entityResultType, refExpr) { Debug.Assert(TypeSemantics.IsEntityType(entityResultType), "DbDerefExpression requires an entity result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a 'scan' of all elements of a given entity set. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbScanExpression : DbExpression { private readonly EntitySetBase _targetSet; internal DbScanExpression(TypeUsage collectionOfEntityType, EntitySetBase entitySet) : base(DbExpressionKind.Scan, collectionOfEntityType) { Debug.Assert(entitySet != null, "DbScanExpression entity set cannot be null"); this._targetSet = entitySet; } ////// Gets the metadata for the referenced entity or relationship set. /// public EntitySetBase Target { get { return _targetSet; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
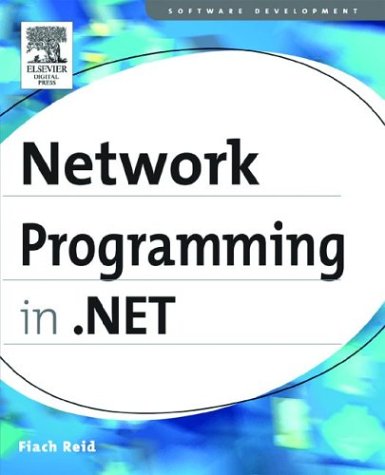
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MsmqBindingBase.cs
- ProtocolsConfigurationHandler.cs
- PixelShader.cs
- AttributeQuery.cs
- PagerSettings.cs
- OracleCommand.cs
- ToolStripOverflow.cs
- bindurihelper.cs
- TableParagraph.cs
- COM2Enum.cs
- IgnoreDeviceFilterElement.cs
- SafeMemoryMappedViewHandle.cs
- DesignerObject.cs
- HtmlToClrEventProxy.cs
- AgileSafeNativeMemoryHandle.cs
- CompilerResults.cs
- BamlRecordWriter.cs
- SubpageParagraph.cs
- WorkflowExecutor.cs
- _ScatterGatherBuffers.cs
- ControlCommandSet.cs
- CodeMethodReturnStatement.cs
- ConstrainedGroup.cs
- SchemaSetCompiler.cs
- Switch.cs
- Errors.cs
- RegexStringValidator.cs
- ReaderOutput.cs
- PropertySegmentSerializationProvider.cs
- SrgsText.cs
- ByteConverter.cs
- Clause.cs
- VisualTreeHelper.cs
- ReflectEventDescriptor.cs
- SingleResultAttribute.cs
- TranslateTransform3D.cs
- BitmapFrameEncode.cs
- WebExceptionStatus.cs
- SqlCachedBuffer.cs
- VisualStateChangedEventArgs.cs
- NamespaceDecl.cs
- CookieProtection.cs
- SchemaLookupTable.cs
- AdornerDecorator.cs
- FileDialog.cs
- ServerValidateEventArgs.cs
- UserValidatedEventArgs.cs
- CompiledXpathExpr.cs
- SqlDataSourceFilteringEventArgs.cs
- SmtpFailedRecipientsException.cs
- ComPlusInstanceContextInitializer.cs
- Error.cs
- TemplateColumn.cs
- Collection.cs
- followingquery.cs
- HttpFileCollectionWrapper.cs
- RijndaelManagedTransform.cs
- ThousandthOfEmRealDoubles.cs
- ExpressionTextBox.xaml.cs
- FlowNode.cs
- XmlSchemaElement.cs
- CodeGotoStatement.cs
- HtmlInputText.cs
- XmlNamespaceDeclarationsAttribute.cs
- Color.cs
- IConvertible.cs
- CancellationState.cs
- PeerCollaborationPermission.cs
- MultiDataTrigger.cs
- BoundingRectTracker.cs
- OleDbWrapper.cs
- PathFigure.cs
- PassportAuthenticationModule.cs
- LineInfo.cs
- HtmlListAdapter.cs
- RtfControls.cs
- ValidatedControlConverter.cs
- SelectedCellsChangedEventArgs.cs
- RecordsAffectedEventArgs.cs
- XPathQilFactory.cs
- ListViewDeleteEventArgs.cs
- StrongNameSignatureInformation.cs
- HttpModulesSection.cs
- XmlNamespaceDeclarationsAttribute.cs
- AuthenticationConfig.cs
- TextLine.cs
- KeyNotFoundException.cs
- IPHostEntry.cs
- MimePart.cs
- SqlReferenceCollection.cs
- ScrollData.cs
- DesigntimeLicenseContext.cs
- Shape.cs
- RenderOptions.cs
- WaitHandleCannotBeOpenedException.cs
- HWStack.cs
- TextControlDesigner.cs
- WS2007HttpBindingElement.cs
- SrgsGrammar.cs
- DataStreams.cs