Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / RenderOptions.cs / 1 / RenderOptions.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The RenderOptions class provides definitions of attached properties // which will control rendering. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Windows.Media { ////// RenderOptions - /// The RenderOptions class provides definitions of attached properties /// which will control rendering. /// public static class RenderOptions { // // EdgeMode // ////// EdgeModeProperty - Enum which descibes the manner in which we render edges of non-text primitives. /// public static readonly DependencyProperty EdgeModeProperty = DependencyProperty.RegisterAttached("EdgeMode", typeof(EdgeMode), typeof(RenderOptions), new UIPropertyMetadata(EdgeMode.Unspecified), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsEdgeModeValid)); ////// Reads the attached property EdgeMode from the given object. /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static EdgeMode GetEdgeMode(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (EdgeMode)target.GetValue(EdgeModeProperty); } ////// Writes the attached property EdgeMode to the given object. /// public static void SetEdgeMode(DependencyObject target, EdgeMode edgeMode) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(EdgeModeProperty, edgeMode); } // // BitmapScaling // ////// BitmapScalingModeProperty - Enum which descibes the manner in which we scale the images. /// public static readonly DependencyProperty BitmapScalingModeProperty = DependencyProperty.RegisterAttached("BitmapScalingMode", typeof(BitmapScalingMode), typeof(RenderOptions), new UIPropertyMetadata(BitmapScalingMode.Unspecified), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsBitmapScalingModeValid)); ////// Reads the attached property BitmapScalingMode from the given object. /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static BitmapScalingMode GetBitmapScalingMode(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (BitmapScalingMode)target.GetValue(BitmapScalingModeProperty); } ////// Writes the attached property BitmapScalingMode to the given object. /// public static void SetBitmapScalingMode(DependencyObject target, BitmapScalingMode bitmapScalingMode) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(BitmapScalingModeProperty, bitmapScalingMode); } // // CachingHint // ////// CachingHintProperty - Hints the rendering engine that rendered content should be cached /// when possible. This is currently supported on TileBrush. /// public static readonly DependencyProperty CachingHintProperty = DependencyProperty.RegisterAttached("CachingHint", typeof(CachingHint), typeof(RenderOptions), new UIPropertyMetadata(CachingHint.Unspecified), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsCachingHintValid)); ////// Reads the attached property CachingHint from the given object. /// [AttachedPropertyBrowsableForType(typeof(TileBrush))] public static CachingHint GetCachingHint(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (CachingHint)target.GetValue(CachingHintProperty); } ////// Writes the attached property CachingHint to the given object. /// public static void SetCachingHint(DependencyObject target, CachingHint cachingHint) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CachingHintProperty, cachingHint); } // // CacheInvalidationThresholdMinimum // ////// CacheInvalidationThresholdMinimum - /// public static readonly DependencyProperty CacheInvalidationThresholdMinimumProperty = DependencyProperty.RegisterAttached("CacheInvalidationThresholdMinimum", typeof(double), typeof(RenderOptions), new UIPropertyMetadata(0.707), /* ValidateValueCallback */ null); ////// Reads the attached property CacheInvalidationThresholdMinimum from the given object. /// [AttachedPropertyBrowsableForType(typeof(TileBrush))] public static double GetCacheInvalidationThresholdMinimum(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (double)target.GetValue(CacheInvalidationThresholdMinimumProperty); } ////// Writes the attached property CacheInvalidationThresholdMinimum to the given object. /// public static void SetCacheInvalidationThresholdMinimum(DependencyObject target, double cacheInvalidationThresholdMinimum) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CacheInvalidationThresholdMinimumProperty, cacheInvalidationThresholdMinimum); } // // CacheInvalidationThresholdMaximum // ////// CacheInvalidationThresholdMaximum - /// public static readonly DependencyProperty CacheInvalidationThresholdMaximumProperty = DependencyProperty.RegisterAttached("CacheInvalidationThresholdMaximum", typeof(double), typeof(RenderOptions), new UIPropertyMetadata(1.414), /* ValidateValueCallback */ null); ////// Reads the attached property CacheInvalidationThresholdMaximum from the given object. /// [AttachedPropertyBrowsableForType(typeof(TileBrush))] public static double GetCacheInvalidationThresholdMaximum(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (double)target.GetValue(CacheInvalidationThresholdMaximumProperty); } ////// Writes the attached property CacheInvalidationThresholdMaximum to the given object. /// public static void SetCacheInvalidationThresholdMaximum(DependencyObject target, double cacheInvalidationThresholdMaximum) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CacheInvalidationThresholdMaximumProperty, cacheInvalidationThresholdMaximum); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // The RenderOptions class provides definitions of attached properties // which will control rendering. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Windows.Media { ////// RenderOptions - /// The RenderOptions class provides definitions of attached properties /// which will control rendering. /// public static class RenderOptions { // // EdgeMode // ////// EdgeModeProperty - Enum which descibes the manner in which we render edges of non-text primitives. /// public static readonly DependencyProperty EdgeModeProperty = DependencyProperty.RegisterAttached("EdgeMode", typeof(EdgeMode), typeof(RenderOptions), new UIPropertyMetadata(EdgeMode.Unspecified), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsEdgeModeValid)); ////// Reads the attached property EdgeMode from the given object. /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static EdgeMode GetEdgeMode(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (EdgeMode)target.GetValue(EdgeModeProperty); } ////// Writes the attached property EdgeMode to the given object. /// public static void SetEdgeMode(DependencyObject target, EdgeMode edgeMode) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(EdgeModeProperty, edgeMode); } // // BitmapScaling // ////// BitmapScalingModeProperty - Enum which descibes the manner in which we scale the images. /// public static readonly DependencyProperty BitmapScalingModeProperty = DependencyProperty.RegisterAttached("BitmapScalingMode", typeof(BitmapScalingMode), typeof(RenderOptions), new UIPropertyMetadata(BitmapScalingMode.Unspecified), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsBitmapScalingModeValid)); ////// Reads the attached property BitmapScalingMode from the given object. /// [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static BitmapScalingMode GetBitmapScalingMode(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (BitmapScalingMode)target.GetValue(BitmapScalingModeProperty); } ////// Writes the attached property BitmapScalingMode to the given object. /// public static void SetBitmapScalingMode(DependencyObject target, BitmapScalingMode bitmapScalingMode) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(BitmapScalingModeProperty, bitmapScalingMode); } // // CachingHint // ////// CachingHintProperty - Hints the rendering engine that rendered content should be cached /// when possible. This is currently supported on TileBrush. /// public static readonly DependencyProperty CachingHintProperty = DependencyProperty.RegisterAttached("CachingHint", typeof(CachingHint), typeof(RenderOptions), new UIPropertyMetadata(CachingHint.Unspecified), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsCachingHintValid)); ////// Reads the attached property CachingHint from the given object. /// [AttachedPropertyBrowsableForType(typeof(TileBrush))] public static CachingHint GetCachingHint(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (CachingHint)target.GetValue(CachingHintProperty); } ////// Writes the attached property CachingHint to the given object. /// public static void SetCachingHint(DependencyObject target, CachingHint cachingHint) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CachingHintProperty, cachingHint); } // // CacheInvalidationThresholdMinimum // ////// CacheInvalidationThresholdMinimum - /// public static readonly DependencyProperty CacheInvalidationThresholdMinimumProperty = DependencyProperty.RegisterAttached("CacheInvalidationThresholdMinimum", typeof(double), typeof(RenderOptions), new UIPropertyMetadata(0.707), /* ValidateValueCallback */ null); ////// Reads the attached property CacheInvalidationThresholdMinimum from the given object. /// [AttachedPropertyBrowsableForType(typeof(TileBrush))] public static double GetCacheInvalidationThresholdMinimum(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (double)target.GetValue(CacheInvalidationThresholdMinimumProperty); } ////// Writes the attached property CacheInvalidationThresholdMinimum to the given object. /// public static void SetCacheInvalidationThresholdMinimum(DependencyObject target, double cacheInvalidationThresholdMinimum) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CacheInvalidationThresholdMinimumProperty, cacheInvalidationThresholdMinimum); } // // CacheInvalidationThresholdMaximum // ////// CacheInvalidationThresholdMaximum - /// public static readonly DependencyProperty CacheInvalidationThresholdMaximumProperty = DependencyProperty.RegisterAttached("CacheInvalidationThresholdMaximum", typeof(double), typeof(RenderOptions), new UIPropertyMetadata(1.414), /* ValidateValueCallback */ null); ////// Reads the attached property CacheInvalidationThresholdMaximum from the given object. /// [AttachedPropertyBrowsableForType(typeof(TileBrush))] public static double GetCacheInvalidationThresholdMaximum(DependencyObject target) { if (target == null) { throw new ArgumentNullException("target"); } return (double)target.GetValue(CacheInvalidationThresholdMaximumProperty); } ////// Writes the attached property CacheInvalidationThresholdMaximum to the given object. /// public static void SetCacheInvalidationThresholdMaximum(DependencyObject target, double cacheInvalidationThresholdMaximum) { if (target == null) { throw new ArgumentNullException("target"); } target.SetValue(CacheInvalidationThresholdMaximumProperty, cacheInvalidationThresholdMaximum); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
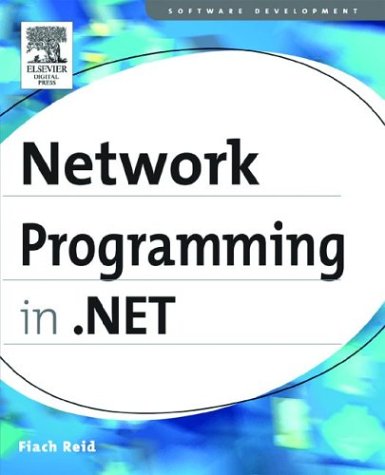
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartUtil.cs
- XPathScanner.cs
- Inflater.cs
- HostingPreferredMapPath.cs
- WebBrowserContainer.cs
- HttpResponse.cs
- ReadOnlyObservableCollection.cs
- XmlDocumentViewSchema.cs
- Property.cs
- DateTimeFormatInfo.cs
- ExclusiveTcpListener.cs
- WindowsStatusBar.cs
- InternalTypeHelper.cs
- LocalFileSettingsProvider.cs
- VideoDrawing.cs
- ButtonFlatAdapter.cs
- XmlLinkedNode.cs
- Directory.cs
- ColumnPropertiesGroup.cs
- CommonDialog.cs
- TypedLocationWrapper.cs
- MergablePropertyAttribute.cs
- StateRuntime.cs
- versioninfo.cs
- OdbcTransaction.cs
- RijndaelManaged.cs
- DesignerRegionMouseEventArgs.cs
- DynamicResourceExtensionConverter.cs
- RelationshipType.cs
- EditorAttribute.cs
- DbModificationClause.cs
- ManipulationDeltaEventArgs.cs
- GetMemberBinder.cs
- LoginViewDesigner.cs
- TableLayoutPanelCellPosition.cs
- PtsHost.cs
- VBIdentifierDesigner.xaml.cs
- EntityCommand.cs
- ObjectSet.cs
- Misc.cs
- Type.cs
- FtpCachePolicyElement.cs
- RuleSettingsCollection.cs
- PeerNameRegistration.cs
- ConfigurationSettings.cs
- DataGrid.cs
- TextTreeInsertUndoUnit.cs
- TypeUtil.cs
- BitmapSourceSafeMILHandle.cs
- Odbc32.cs
- NameValueCollection.cs
- SearchForVirtualItemEventArgs.cs
- QuaternionAnimationBase.cs
- XmlLangPropertyAttribute.cs
- HighContrastHelper.cs
- SizeAnimationUsingKeyFrames.cs
- UIElementParaClient.cs
- DiagnosticTraceSource.cs
- ErrorView.xaml.cs
- HtmlInputRadioButton.cs
- FileLevelControlBuilderAttribute.cs
- ConnectionStringSettingsCollection.cs
- HtmlForm.cs
- RegisteredHiddenField.cs
- ReverseInheritProperty.cs
- Message.cs
- UTF32Encoding.cs
- Material.cs
- PrinterSettings.cs
- BindingValueChangedEventArgs.cs
- ConfigurationSection.cs
- SecurityTokenException.cs
- GetLedgerEntryForRecipientRequest.cs
- OdbcUtils.cs
- MessagePropertyVariants.cs
- DesignerEditorPartChrome.cs
- RegexCaptureCollection.cs
- ResourceBinder.cs
- TemplatePagerField.cs
- Comparer.cs
- ScriptManagerProxy.cs
- MimeMapping.cs
- ProcessInfo.cs
- ConsoleEntryPoint.cs
- UnitySerializationHolder.cs
- NavigationCommands.cs
- MemberMaps.cs
- ShellProvider.cs
- XmlDocumentSurrogate.cs
- SiteMapHierarchicalDataSourceView.cs
- Knowncolors.cs
- DrawingCollection.cs
- ConsoleKeyInfo.cs
- XmlSerializerNamespaces.cs
- TypeDependencyAttribute.cs
- UndirectedGraph.cs
- DbLambda.cs
- EastAsianLunisolarCalendar.cs
- DataListCommandEventArgs.cs
- SqlXml.cs