Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / ProcessActivityTreeOptions.cs / 1305376 / ProcessActivityTreeOptions.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System.Collections.Generic; using System.Activities.Validation; using System.Runtime; class ProcessActivityTreeOptions { static ProcessActivityTreeOptions validationOptions; static ProcessActivityTreeOptions singleLevelValidationOptions; static ProcessActivityTreeOptions fullCachingOptions; static ProcessActivityTreeOptions finishCachingSubtreeOptionsWithCreateEmptyBindings; static ProcessActivityTreeOptions finishCachingSubtreeOptionsWithoutCreateEmptyBindings; ProcessActivityTreeOptions() { } public static ProcessActivityTreeOptions FullCachingOptions { get { if (fullCachingOptions == null) { fullCachingOptions = new ProcessActivityTreeOptions { SkipIfCached = true, CreateEmptyBindings = true, OnlyCallCallbackForDeclarations = true }; } return fullCachingOptions; } } public static ProcessActivityTreeOptions ValidationOptions { get { if (validationOptions == null) { validationOptions = new ProcessActivityTreeOptions { SkipPrivateChildren = false, // We don't want to interfere with activities doing null-checks // by creating empty bindings. CreateEmptyBindings = false }; } return validationOptions; } } static ProcessActivityTreeOptions SingleLevelValidationOptions { get { if (singleLevelValidationOptions == null) { singleLevelValidationOptions = new ProcessActivityTreeOptions { SkipPrivateChildren = false, // We don't want to interfere with activities doing null-checks // by creating empty bindings. CreateEmptyBindings = false, OnlyVisitSingleLevel = true }; } return singleLevelValidationOptions; } } static ProcessActivityTreeOptions FinishCachingSubtreeOptionsWithoutCreateEmptyBindings { get { if (finishCachingSubtreeOptionsWithoutCreateEmptyBindings == null) { // We don't want to run constraints and we only want to hit // the public path. finishCachingSubtreeOptionsWithoutCreateEmptyBindings = new ProcessActivityTreeOptions { SkipConstraints = true, StoreTempViolations = true }; } return finishCachingSubtreeOptionsWithoutCreateEmptyBindings; } } static ProcessActivityTreeOptions FinishCachingSubtreeOptionsWithCreateEmptyBindings { get { if (finishCachingSubtreeOptionsWithCreateEmptyBindings == null) { // We don't want to run constraints and we only want to hit // the public path. finishCachingSubtreeOptionsWithCreateEmptyBindings = new ProcessActivityTreeOptions { SkipConstraints = true, CreateEmptyBindings = true, StoreTempViolations = true }; } return finishCachingSubtreeOptionsWithCreateEmptyBindings; } } public static ProcessActivityTreeOptions GetFinishCachingSubtreeOptions(ProcessActivityTreeOptions originalOptions) { if (originalOptions.CreateEmptyBindings) { return ProcessActivityTreeOptions.FinishCachingSubtreeOptionsWithCreateEmptyBindings; } else { return ProcessActivityTreeOptions.FinishCachingSubtreeOptionsWithoutCreateEmptyBindings; } } public static ProcessActivityTreeOptions GetValidationOptions(ValidationSettings settings) { if (settings.SingleLevel) { return ProcessActivityTreeOptions.SingleLevelValidationOptions; } else { return ProcessActivityTreeOptions.ValidationOptions; } } public bool SkipIfCached { get; private set; } public bool CreateEmptyBindings { get; private set; } public bool SkipPrivateChildren { get; private set; } public bool OnlyCallCallbackForDeclarations { get; private set; } public bool SkipConstraints { get; private set; } public bool OnlyVisitSingleLevel { get; private set; } public bool StoreTempViolations { get; private set; } public bool IsRuntimeReadyOptions { get { // We don't really support progressive caching at runtime so we only set ourselves // as runtime ready if we cached the whole workflow and created empty bindings. // In order to support progressive caching we need to deal with the following // issues: // * We need a mechanism for supporting activities which supply extensions // * We need to understand when we haven't created empty bindings so that // we can progressively create them return !this.SkipPrivateChildren && this.CreateEmptyBindings; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
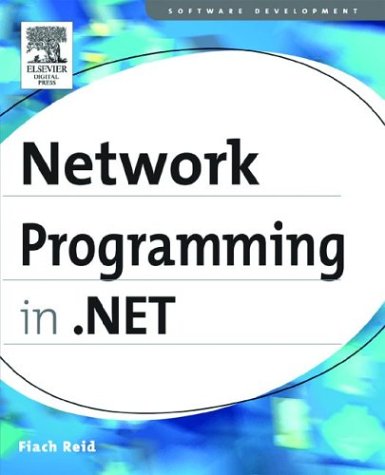
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsSolidBrush.cs
- ScopedMessagePartSpecification.cs
- ReaderOutput.cs
- Rijndael.cs
- XmlLanguageConverter.cs
- ToolBar.cs
- _BufferOffsetSize.cs
- XmlAnyElementAttribute.cs
- RootBrowserWindowProxy.cs
- CodeLinePragma.cs
- RenderTargetBitmap.cs
- MouseActionValueSerializer.cs
- Emitter.cs
- SystemBrushes.cs
- SynchronizedPool.cs
- EdmSchemaAttribute.cs
- TimersDescriptionAttribute.cs
- PaperSource.cs
- TemplateNameScope.cs
- TraceHandlerErrorFormatter.cs
- FontDifferentiator.cs
- TabControlCancelEvent.cs
- QualificationDataItem.cs
- ExpressionBuilder.cs
- indexingfiltermarshaler.cs
- EventLogger.cs
- Simplifier.cs
- HttpGetServerProtocol.cs
- Column.cs
- TogglePattern.cs
- DataContractAttribute.cs
- CollectionView.cs
- XD.cs
- Lasso.cs
- SpecularMaterial.cs
- ModelTreeEnumerator.cs
- XmlSchemaSimpleType.cs
- SortQuery.cs
- hresults.cs
- TextEditorCharacters.cs
- InternalControlCollection.cs
- XXXInfos.cs
- Win32KeyboardDevice.cs
- BevelBitmapEffect.cs
- BrowserCapabilitiesCodeGenerator.cs
- XPathChildIterator.cs
- SqlFunctionAttribute.cs
- XmlElementAttribute.cs
- RtfToXamlLexer.cs
- ColumnClickEvent.cs
- SafeCloseHandleCritical.cs
- ErrorFormatter.cs
- SqlNodeAnnotation.cs
- ChangeNode.cs
- PenLineJoinValidation.cs
- MediaTimeline.cs
- SQLBytes.cs
- WaitHandleCannotBeOpenedException.cs
- FormatterServices.cs
- DataGridViewButtonCell.cs
- SafeProcessHandle.cs
- ArrangedElementCollection.cs
- InstanceCreationEditor.cs
- TreeNodeBindingCollection.cs
- ICspAsymmetricAlgorithm.cs
- TextServicesManager.cs
- HuffmanTree.cs
- WebConfigurationFileMap.cs
- BehaviorEditorPart.cs
- COAUTHINFO.cs
- TdsParameterSetter.cs
- WindowsUpDown.cs
- WinFormsUtils.cs
- QilInvokeLateBound.cs
- ServerValidateEventArgs.cs
- RepeatBehavior.cs
- OleDbStruct.cs
- DataRelationCollection.cs
- EmptyCollection.cs
- XPathSelfQuery.cs
- SqlNodeAnnotation.cs
- ImageClickEventArgs.cs
- SHA384Managed.cs
- QfeChecker.cs
- DataColumnSelectionConverter.cs
- Rect3DValueSerializer.cs
- BitmapEffectInputData.cs
- BitmapFrame.cs
- PrintPreviewGraphics.cs
- SizeFConverter.cs
- ReflectPropertyDescriptor.cs
- FigureParagraph.cs
- WebPartCloseVerb.cs
- NamespaceDisplay.xaml.cs
- VarRemapper.cs
- WindowsTitleBar.cs
- RectangleGeometry.cs
- PhysicalAddress.cs
- ExceptionCollection.cs
- DataBindingList.cs