Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / ClientSideProviderDescription.cs / 1305600 / ClientSideProviderDescription.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Structure containing information about a proxy // // History: // 06/05/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Enum used to indicate results of requesting a property /// [Flags] #if (INTERNAL_COMPILE) internal enum ClientSideProviderMatchIndicator #else public enum ClientSideProviderMatchIndicator #endif { ////// Default settings will be used for this proxy: classname will be matched /// using full string comparison, and a match against the underlying class name /// (for superclassed USER32 and Common Controls) will be allowed. /// None = 0x00, ////// Use substring comparison for comparing classnames /// AllowSubstringMatch = 0x01, ////// The real class name or base class will not be checked /// DisallowBaseClassNameMatch = 0x02, } ////// Implemented by HWND handlers, called by UIAutomation framework to request a proxy for specified window and item. /// Should return a proxy if supported, or null if not supported. /// #if (INTERNAL_COMPILE) internal delegate IRawElementProviderSimple ClientSideProviderFactoryCallback( IntPtr hwnd, int idChild, int idObject ); #else public delegate IRawElementProviderSimple ClientSideProviderFactoryCallback(IntPtr hwnd, int idChild, int idObject); #endif ////// Structure containing information about a proxy /// #if (INTERNAL_COMPILE) internal struct ClientSideProviderDescription #else public struct ClientSideProviderDescription #endif { private string _className; private string _imageName; private ClientSideProviderMatchIndicator _flags; private ClientSideProviderFactoryCallback _proxyFactoryCallback; ////// Constructor /// /// Window classname that the proxy is for. If null is used, the proxy will be called for all /// windows if no other proxy has been found for that window. /// Delegate that PAW will call to request the creation of a proxy public ClientSideProviderDescription(ClientSideProviderFactoryCallback clientSideProviderFactoryCallback, string className) { // Null and Empty string mean different things here. #pragma warning suppress 6507 if (className != null) _className = className.ToLower( System.Globalization.CultureInfo.InvariantCulture ); else _className = null; _flags = 0; _imageName = null; _proxyFactoryCallback = clientSideProviderFactoryCallback; } ////// Constructor /// /// Window classname that the proxy is for. If null is used, the proxy will be called for all /// windows if no other proxy has been found for that window. /// Delegate that PAW will call to request the creation of a proxy /// Enum ProxyMatchFlags /// otherwise the parameter classname can be contained in the window class name /// Name of the executable for the process where this window resides. For example outllib.dll or calc.exe public ClientSideProviderDescription(ClientSideProviderFactoryCallback clientSideProviderFactoryCallback, string className, string imageName, ClientSideProviderMatchIndicator flags) { // Null and Empty string mean different things here #pragma warning suppress 6507 if (className != null) _className = className.ToLower( System.Globalization.CultureInfo.InvariantCulture ); else _className = null; _flags = flags; // Null and Empty string mean different things here #pragma warning suppress 6507 if (imageName != null) _imageName = imageName.ToLower( System.Globalization.CultureInfo.InvariantCulture ); else _imageName = null; _proxyFactoryCallback = clientSideProviderFactoryCallback; } ////// Window classname that the proxy is for. /// public string ClassName { get { return _className; } } ////// Returns the ClientSideProviderMatchIndicator flags that specify options for how /// this description should be used. /// public ClientSideProviderMatchIndicator Flags { get { return _flags; } } ////// Name of the executable for the process where this window resides. For example Winword.exe. /// public string ImageName { get { return _imageName; } } ////// Delegate that UIAutomation will call to request the creation of a proxy /// public ClientSideProviderFactoryCallback ClientSideProviderFactoryCallback { get { return _proxyFactoryCallback; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Structure containing information about a proxy // // History: // 06/05/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Enum used to indicate results of requesting a property /// [Flags] #if (INTERNAL_COMPILE) internal enum ClientSideProviderMatchIndicator #else public enum ClientSideProviderMatchIndicator #endif { ////// Default settings will be used for this proxy: classname will be matched /// using full string comparison, and a match against the underlying class name /// (for superclassed USER32 and Common Controls) will be allowed. /// None = 0x00, ////// Use substring comparison for comparing classnames /// AllowSubstringMatch = 0x01, ////// The real class name or base class will not be checked /// DisallowBaseClassNameMatch = 0x02, } ////// Implemented by HWND handlers, called by UIAutomation framework to request a proxy for specified window and item. /// Should return a proxy if supported, or null if not supported. /// #if (INTERNAL_COMPILE) internal delegate IRawElementProviderSimple ClientSideProviderFactoryCallback( IntPtr hwnd, int idChild, int idObject ); #else public delegate IRawElementProviderSimple ClientSideProviderFactoryCallback(IntPtr hwnd, int idChild, int idObject); #endif ////// Structure containing information about a proxy /// #if (INTERNAL_COMPILE) internal struct ClientSideProviderDescription #else public struct ClientSideProviderDescription #endif { private string _className; private string _imageName; private ClientSideProviderMatchIndicator _flags; private ClientSideProviderFactoryCallback _proxyFactoryCallback; ////// Constructor /// /// Window classname that the proxy is for. If null is used, the proxy will be called for all /// windows if no other proxy has been found for that window. /// Delegate that PAW will call to request the creation of a proxy public ClientSideProviderDescription(ClientSideProviderFactoryCallback clientSideProviderFactoryCallback, string className) { // Null and Empty string mean different things here. #pragma warning suppress 6507 if (className != null) _className = className.ToLower( System.Globalization.CultureInfo.InvariantCulture ); else _className = null; _flags = 0; _imageName = null; _proxyFactoryCallback = clientSideProviderFactoryCallback; } ////// Constructor /// /// Window classname that the proxy is for. If null is used, the proxy will be called for all /// windows if no other proxy has been found for that window. /// Delegate that PAW will call to request the creation of a proxy /// Enum ProxyMatchFlags /// otherwise the parameter classname can be contained in the window class name /// Name of the executable for the process where this window resides. For example outllib.dll or calc.exe public ClientSideProviderDescription(ClientSideProviderFactoryCallback clientSideProviderFactoryCallback, string className, string imageName, ClientSideProviderMatchIndicator flags) { // Null and Empty string mean different things here #pragma warning suppress 6507 if (className != null) _className = className.ToLower( System.Globalization.CultureInfo.InvariantCulture ); else _className = null; _flags = flags; // Null and Empty string mean different things here #pragma warning suppress 6507 if (imageName != null) _imageName = imageName.ToLower( System.Globalization.CultureInfo.InvariantCulture ); else _imageName = null; _proxyFactoryCallback = clientSideProviderFactoryCallback; } ////// Window classname that the proxy is for. /// public string ClassName { get { return _className; } } ////// Returns the ClientSideProviderMatchIndicator flags that specify options for how /// this description should be used. /// public ClientSideProviderMatchIndicator Flags { get { return _flags; } } ////// Name of the executable for the process where this window resides. For example Winword.exe. /// public string ImageName { get { return _imageName; } } ////// Delegate that UIAutomation will call to request the creation of a proxy /// public ClientSideProviderFactoryCallback ClientSideProviderFactoryCallback { get { return _proxyFactoryCallback; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
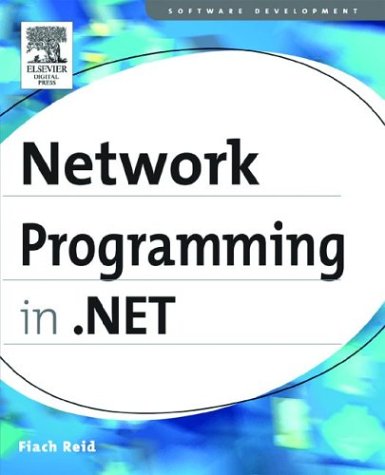
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostingEnvironmentWrapper.cs
- SmiContext.cs
- wmiprovider.cs
- TextAutomationPeer.cs
- isolationinterop.cs
- ResourceAssociationTypeEnd.cs
- TextTrailingCharacterEllipsis.cs
- RepeatBehaviorConverter.cs
- RoutedPropertyChangedEventArgs.cs
- PrintPageEvent.cs
- DrawingBrush.cs
- RuntimeWrappedException.cs
- CellParagraph.cs
- PackWebResponse.cs
- SHA256Managed.cs
- ModifierKeysValueSerializer.cs
- XmlSchemaCompilationSettings.cs
- MessageQuerySet.cs
- CallTemplateAction.cs
- ParameterCollectionEditor.cs
- ALinqExpressionVisitor.cs
- Predicate.cs
- ReachPrintTicketSerializerAsync.cs
- SingleConverter.cs
- ProcessModelInfo.cs
- OutKeywords.cs
- RegionInfo.cs
- Fx.cs
- ThreadStaticAttribute.cs
- KeyToListMap.cs
- DataListItemEventArgs.cs
- EntityDataSourceDesignerHelper.cs
- DataRecordObjectView.cs
- RectangleGeometry.cs
- TrackBar.cs
- FigureHelper.cs
- ExceptionWrapper.cs
- DataSetMappper.cs
- SplitterEvent.cs
- TargetInvocationException.cs
- FieldAccessException.cs
- GregorianCalendar.cs
- DesignerAttribute.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- CompilerScope.cs
- Clipboard.cs
- UserUseLicenseDictionaryLoader.cs
- WebConfigurationManager.cs
- MembershipPasswordException.cs
- Pen.cs
- SerialPinChanges.cs
- StringUtil.cs
- LicenseContext.cs
- XmlNodeReader.cs
- SharedUtils.cs
- IPAddressCollection.cs
- SqlCachedBuffer.cs
- BamlTreeNode.cs
- PackageRelationship.cs
- IImplicitResourceProvider.cs
- StateMachineWorkflowDesigner.cs
- StringValidator.cs
- DynamicFilterExpression.cs
- ToolStripPanelRenderEventArgs.cs
- XamlBrushSerializer.cs
- GridViewColumn.cs
- Rule.cs
- CodeExporter.cs
- DispatcherExceptionFilterEventArgs.cs
- TimeoutException.cs
- DirectionalLight.cs
- AvTrace.cs
- DecimalAnimationBase.cs
- TextPointerBase.cs
- XmlSchemaAny.cs
- AttachInfo.cs
- DateTime.cs
- IdentityNotMappedException.cs
- ObjectDataSourceView.cs
- SecurityKeyEntropyMode.cs
- EntityContainerAssociationSet.cs
- HostedHttpTransportManager.cs
- DllNotFoundException.cs
- Hyperlink.cs
- MenuRendererStandards.cs
- Debug.cs
- ExpressionVisitorHelpers.cs
- BoundsDrawingContextWalker.cs
- MailHeaderInfo.cs
- RayHitTestParameters.cs
- DataComponentGenerator.cs
- ResXResourceWriter.cs
- MenuItemStyleCollection.cs
- HtmlTitle.cs
- BindingContext.cs
- BindToObject.cs
- GraphicsPath.cs
- COSERVERINFO.cs
- PageParser.cs
- Converter.cs