Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Configuration / ProcessHostConfigUtils.cs / 1305376 / ProcessHostConfigUtils.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Configuration; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Xml; using System.Security; using System.Text; using System.Web.Util; using System.Web.UI; using System.IO; using System.Web.Hosting; using System.Runtime.ConstrainedExecution; // // Uses IIS 7 native config // internal static class ProcessHostConfigUtils { internal const uint DEFAULT_SITE_ID_UINT = 1; internal const string DEFAULT_SITE_ID_STRING = "1"; private static string s_defaultSiteName; private static int s_InitedExternalConfig; private static NativeConfigWrapper _configWrapper; // static class ctor static ProcessHostConfigUtils() { HttpRuntime.ForceStaticInit(); } internal static void InitStandaloneConfig() { if (!HostingEnvironment.IsUnderIISProcess) { if (!ServerConfig.UseMetabase) { int inited= Interlocked.Exchange(ref s_InitedExternalConfig, 1); // only do this once if (0 == inited) { _configWrapper = new NativeConfigWrapper(); } } } } internal static string MapPathActual(string siteName, VirtualPath path) { string physicalPath = null; IntPtr pBstr = IntPtr.Zero; int cBstr = 0; try { int result = UnsafeIISMethods.MgdMapPathDirect(siteName, path.VirtualPathString, out pBstr, out cBstr); if (result < 0) { throw new InvalidOperationException(SR.GetString(SR.Cannot_map_path, path.VirtualPathString)); } physicalPath = (pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : null; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } return physicalPath; } internal static string GetSiteNameFromId(uint siteId) { if ( siteId == DEFAULT_SITE_ID_UINT && s_defaultSiteName != null) { return s_defaultSiteName; } IntPtr pBstr = IntPtr.Zero; int cBstr = 0; string siteName = null; try { int result = UnsafeIISMethods.MgdGetSiteNameFromId(siteId, out pBstr, out cBstr); siteName = (result == 0 && pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : String.Empty; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } if ( siteId == DEFAULT_SITE_ID_UINT) { s_defaultSiteName = siteName; } return siteName; } private class NativeConfigWrapper : CriticalFinalizerObject { internal NativeConfigWrapper() { int result = UnsafeIISMethods.MgdInitNativeConfig(); if (result < 0) { s_InitedExternalConfig = 0; throw new InvalidOperationException(SR.GetString(SR.Cant_Init_Native_Config, result.ToString("X8", CultureInfo.InvariantCulture))); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] ~NativeConfigWrapper() { UnsafeIISMethods.MgdTerminateNativeConfig(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Configuration; using System.Collections; using System.Globalization; using System.Runtime.InteropServices; using System.Threading; using System.Xml; using System.Security; using System.Text; using System.Web.Util; using System.Web.UI; using System.IO; using System.Web.Hosting; using System.Runtime.ConstrainedExecution; // // Uses IIS 7 native config // internal static class ProcessHostConfigUtils { internal const uint DEFAULT_SITE_ID_UINT = 1; internal const string DEFAULT_SITE_ID_STRING = "1"; private static string s_defaultSiteName; private static int s_InitedExternalConfig; private static NativeConfigWrapper _configWrapper; // static class ctor static ProcessHostConfigUtils() { HttpRuntime.ForceStaticInit(); } internal static void InitStandaloneConfig() { if (!HostingEnvironment.IsUnderIISProcess) { if (!ServerConfig.UseMetabase) { int inited= Interlocked.Exchange(ref s_InitedExternalConfig, 1); // only do this once if (0 == inited) { _configWrapper = new NativeConfigWrapper(); } } } } internal static string MapPathActual(string siteName, VirtualPath path) { string physicalPath = null; IntPtr pBstr = IntPtr.Zero; int cBstr = 0; try { int result = UnsafeIISMethods.MgdMapPathDirect(siteName, path.VirtualPathString, out pBstr, out cBstr); if (result < 0) { throw new InvalidOperationException(SR.GetString(SR.Cannot_map_path, path.VirtualPathString)); } physicalPath = (pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : null; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } return physicalPath; } internal static string GetSiteNameFromId(uint siteId) { if ( siteId == DEFAULT_SITE_ID_UINT && s_defaultSiteName != null) { return s_defaultSiteName; } IntPtr pBstr = IntPtr.Zero; int cBstr = 0; string siteName = null; try { int result = UnsafeIISMethods.MgdGetSiteNameFromId(siteId, out pBstr, out cBstr); siteName = (result == 0 && pBstr != IntPtr.Zero) ? StringUtil.StringFromWCharPtr(pBstr, cBstr) : String.Empty; } finally { if (pBstr != IntPtr.Zero) { Marshal.FreeBSTR(pBstr); } } if ( siteId == DEFAULT_SITE_ID_UINT) { s_defaultSiteName = siteName; } return siteName; } private class NativeConfigWrapper : CriticalFinalizerObject { internal NativeConfigWrapper() { int result = UnsafeIISMethods.MgdInitNativeConfig(); if (result < 0) { s_InitedExternalConfig = 0; throw new InvalidOperationException(SR.GetString(SR.Cant_Init_Native_Config, result.ToString("X8", CultureInfo.InvariantCulture))); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] ~NativeConfigWrapper() { UnsafeIISMethods.MgdTerminateNativeConfig(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
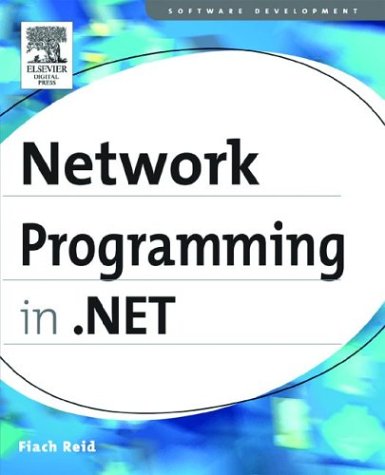
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScrollProperties.cs
- HitTestParameters.cs
- SecurityHelper.cs
- DoubleAverageAggregationOperator.cs
- StylusPointPropertyId.cs
- EnumUnknown.cs
- CacheDependency.cs
- ExceptionTranslationTable.cs
- SQLMoney.cs
- BindUriHelper.cs
- CultureInfoConverter.cs
- Style.cs
- GridViewSelectEventArgs.cs
- DefaultAssemblyResolver.cs
- UndoEngine.cs
- GeneralTransform2DTo3D.cs
- XsltArgumentList.cs
- CornerRadiusConverter.cs
- ZoneLinkButton.cs
- TypedRowHandler.cs
- ImportCatalogPart.cs
- HtmlToClrEventProxy.cs
- recordstatefactory.cs
- Wrapper.cs
- DataControlLinkButton.cs
- PropertyGrid.cs
- GlobalizationSection.cs
- PersistenceException.cs
- DependencySource.cs
- SizeLimitedCache.cs
- ScrollProperties.cs
- _NetRes.cs
- _ListenerRequestStream.cs
- ServiceEndpointElementCollection.cs
- CalculatedColumn.cs
- HostedAspNetEnvironment.cs
- SystemPens.cs
- Int32Converter.cs
- SqlBinder.cs
- PnrpPeerResolverBindingElement.cs
- RuntimeArgumentHandle.cs
- WasAdminWrapper.cs
- DataTableTypeConverter.cs
- EncoderExceptionFallback.cs
- OutputCacheModule.cs
- SqlProcedureAttribute.cs
- SmtpAuthenticationManager.cs
- Variant.cs
- PageAction.cs
- XmlNamespaceDeclarationsAttribute.cs
- keycontainerpermission.cs
- SqlBuilder.cs
- HtmlInputRadioButton.cs
- BitmapCacheBrush.cs
- CustomTypeDescriptor.cs
- GlobalItem.cs
- WebBrowser.cs
- MimeTextImporter.cs
- EventDescriptor.cs
- InvalidOperationException.cs
- ToolCreatedEventArgs.cs
- OletxVolatileEnlistment.cs
- PageCache.cs
- ArgumentDirectionHelper.cs
- WindowsContainer.cs
- InkCanvasInnerCanvas.cs
- SoapSchemaImporter.cs
- FileLevelControlBuilderAttribute.cs
- _AcceptOverlappedAsyncResult.cs
- XPathException.cs
- SqlBuilder.cs
- DataViewManager.cs
- COAUTHINFO.cs
- BaseResourcesBuildProvider.cs
- SpotLight.cs
- NegotiateStream.cs
- ErrorView.xaml.cs
- DelegateSerializationHolder.cs
- EdmRelationshipRoleAttribute.cs
- Group.cs
- PolyLineSegment.cs
- AgileSafeNativeMemoryHandle.cs
- AbandonedMutexException.cs
- PrivilegedConfigurationManager.cs
- HwndMouseInputProvider.cs
- WindowsRegion.cs
- PropertyValueChangedEvent.cs
- XmlSchemaDatatype.cs
- Html32TextWriter.cs
- DbBuffer.cs
- ReachUIElementCollectionSerializer.cs
- TypeReference.cs
- LinqDataSourceStatusEventArgs.cs
- ActivityExecutor.cs
- XmlSchemaInfo.cs
- ApplicationContext.cs
- IsolatedStorageException.cs
- ParserHooks.cs
- AttributeUsageAttribute.cs
- DbProviderFactoriesConfigurationHandler.cs