Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Server / System / Data / Services / Parsing / Token.cs / 1 / Token.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to represent a parsed token. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Parsing { using System; using System.Diagnostics; ///Use this class to represent a lexical token. [DebuggerDisplay("{Id} @ {Position}: [{Text}]")] internal struct Token { ///Kind of token. internal TokenId Id; ///Token text. internal string Text; ///Position of token. internal int Position; ///Checks whether this token is a comparison operator. internal bool IsComparisonOperator { get { if (this.Id != TokenId.Identifier) { return false; } return this.Text == ExpressionConstants.KeywordEqual || this.Text == ExpressionConstants.KeywordNotEqual || this.Text == ExpressionConstants.KeywordLessThan || this.Text == ExpressionConstants.KeywordGreaterThan || this.Text == ExpressionConstants.KeywordLessThanOrEqual || this.Text == ExpressionConstants.KeywordGreaterThanOrEqual; } } ///Checks whether this token is an equality operator. internal bool IsEqualityOperator { get { return this.Id == TokenId.Identifier && (this.Text == ExpressionConstants.KeywordEqual || this.Text == ExpressionConstants.KeywordNotEqual); } } ///Checks whether this token is a valid token for a key value. internal bool IsKeyValueToken { get { return this.Id == TokenId.BinaryLiteral || this.Id == TokenId.BooleanLiteral || this.Id == TokenId.DateTimeLiteral || this.Id == TokenId.GuidLiteral || this.Id == TokenId.StringLiteral || ExpressionLexer.IsNumeric(this.Id); } } ///Provides a string representation of this token. ///String representation of this token. public override string ToString() { return String.Format(System.Globalization.CultureInfo.InvariantCulture, "{0} @ {1}: [{2}]", this.Id, this.Position, this.Text); } ///Gets the current identifier text. ///The current identifier text. internal string GetIdentifier() { if (this.Id != TokenId.Identifier) { throw DataServiceException.CreateSyntaxError(Strings.RequestQueryParser_IdentifierExpected(this.Position)); } return this.Text; } ///Checks that this token has the specified identifier. /// Identifier to check. ///true if this is an identifier with the specified text. internal bool IdentifierIs(string id) { return this.Id == TokenId.Identifier && this.Text == id; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a type to represent a parsed token. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Parsing { using System; using System.Diagnostics; ///Use this class to represent a lexical token. [DebuggerDisplay("{Id} @ {Position}: [{Text}]")] internal struct Token { ///Kind of token. internal TokenId Id; ///Token text. internal string Text; ///Position of token. internal int Position; ///Checks whether this token is a comparison operator. internal bool IsComparisonOperator { get { if (this.Id != TokenId.Identifier) { return false; } return this.Text == ExpressionConstants.KeywordEqual || this.Text == ExpressionConstants.KeywordNotEqual || this.Text == ExpressionConstants.KeywordLessThan || this.Text == ExpressionConstants.KeywordGreaterThan || this.Text == ExpressionConstants.KeywordLessThanOrEqual || this.Text == ExpressionConstants.KeywordGreaterThanOrEqual; } } ///Checks whether this token is an equality operator. internal bool IsEqualityOperator { get { return this.Id == TokenId.Identifier && (this.Text == ExpressionConstants.KeywordEqual || this.Text == ExpressionConstants.KeywordNotEqual); } } ///Checks whether this token is a valid token for a key value. internal bool IsKeyValueToken { get { return this.Id == TokenId.BinaryLiteral || this.Id == TokenId.BooleanLiteral || this.Id == TokenId.DateTimeLiteral || this.Id == TokenId.GuidLiteral || this.Id == TokenId.StringLiteral || ExpressionLexer.IsNumeric(this.Id); } } ///Provides a string representation of this token. ///String representation of this token. public override string ToString() { return String.Format(System.Globalization.CultureInfo.InvariantCulture, "{0} @ {1}: [{2}]", this.Id, this.Position, this.Text); } ///Gets the current identifier text. ///The current identifier text. internal string GetIdentifier() { if (this.Id != TokenId.Identifier) { throw DataServiceException.CreateSyntaxError(Strings.RequestQueryParser_IdentifierExpected(this.Position)); } return this.Text; } ///Checks that this token has the specified identifier. /// Identifier to check. ///true if this is an identifier with the specified text. internal bool IdentifierIs(string id) { return this.Id == TokenId.Identifier && this.Text == id; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
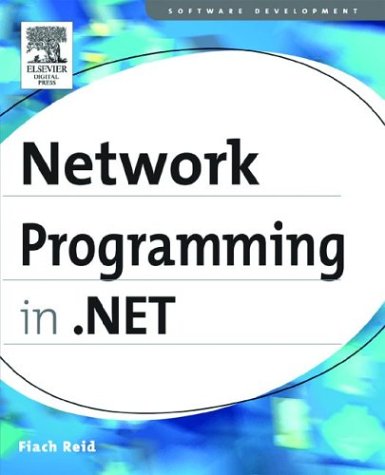
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeUnloadedException.cs
- UIElement3D.cs
- SqlMethodTransformer.cs
- RootBuilder.cs
- StrokeCollectionDefaultValueFactory.cs
- EventMap.cs
- PropertyConverter.cs
- GZipStream.cs
- DataGridViewRowPostPaintEventArgs.cs
- keycontainerpermission.cs
- TemplateXamlParser.cs
- DataMisalignedException.cs
- VirtualPathProvider.cs
- Models.cs
- GradientBrush.cs
- ToolStripItemImageRenderEventArgs.cs
- PolicyValidationException.cs
- GridViewRow.cs
- InvalidProgramException.cs
- _NegoStream.cs
- FrameworkContentElement.cs
- MouseGesture.cs
- HMACSHA1.cs
- AsymmetricCryptoHandle.cs
- FrameworkObject.cs
- RestHandlerFactory.cs
- WebPartMinimizeVerb.cs
- EventBuilder.cs
- Panel.cs
- GroupLabel.cs
- Int32CAMarshaler.cs
- RepeaterItemEventArgs.cs
- RegionData.cs
- ConstraintEnumerator.cs
- EditorPartCollection.cs
- InvariantComparer.cs
- DelegateArgumentReference.cs
- Compiler.cs
- LazyLoadBehavior.cs
- RemotingConfiguration.cs
- SimpleNameService.cs
- Utils.cs
- RecommendedAsConfigurableAttribute.cs
- ConfigurationElement.cs
- WebRequestModuleElementCollection.cs
- HttpModulesSection.cs
- XslUrlEditor.cs
- AudienceUriMode.cs
- MimeReflector.cs
- XmlSchemaSimpleContentExtension.cs
- TextServicesManager.cs
- WebPartEventArgs.cs
- TemplateXamlParser.cs
- Mappings.cs
- CompositeCollection.cs
- ConstructorNeedsTagAttribute.cs
- DataBindingsDialog.cs
- SafeFreeMibTable.cs
- OperandQuery.cs
- DoubleKeyFrameCollection.cs
- AutomationProperty.cs
- CheckableControlBaseAdapter.cs
- ImmutableObjectAttribute.cs
- ProxyGenerator.cs
- X509Chain.cs
- SqlParameter.cs
- HorizontalAlignConverter.cs
- TypeReference.cs
- Facet.cs
- UnmanagedMemoryStreamWrapper.cs
- HMACSHA512.cs
- CheckBoxPopupAdapter.cs
- _NTAuthentication.cs
- RowToFieldTransformer.cs
- RadioButtonStandardAdapter.cs
- ApplicationSecurityManager.cs
- SessionEndingCancelEventArgs.cs
- TreeViewImageKeyConverter.cs
- SmtpClient.cs
- AnnotationResource.cs
- ConfigXmlCDataSection.cs
- DataControlButton.cs
- ProcessHostFactoryHelper.cs
- EditCommandColumn.cs
- StrongNameKeyPair.cs
- FileResponseElement.cs
- WebPartMovingEventArgs.cs
- StorageTypeMapping.cs
- BitmapMetadataBlob.cs
- _NegoStream.cs
- BatchWriter.cs
- AnnotationResourceCollection.cs
- XmlNamedNodeMap.cs
- GridViewCommandEventArgs.cs
- SourceFileInfo.cs
- XsltOutput.cs
- TextAutomationPeer.cs
- XslAstAnalyzer.cs
- ISAPIApplicationHost.cs
- DuplexChannelBinder.cs