Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Panel.cs / 1305376 / Panel.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Collections; using System.Web.UI; using System.ComponentModel; using System.ComponentModel.Design; using System.Security.Permissions; namespace System.Web.UI.MobileControls { ///[ ControlBuilderAttribute(typeof(PanelControlBuilder)), Designer(typeof(System.Web.UI.Design.MobileControls.PanelDesigner)), DesignerAdapter(typeof(System.Web.UI.MobileControls.Adapters.HtmlPanelAdapter)), PersistChildren(true), ToolboxData("<{0}:Panel runat=\"server\">{0}:Panel>"), ToolboxItem("System.Web.UI.Design.WebControlToolboxItem, " + AssemblyRef.SystemDesign) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class Panel : MobileControl, ITemplateable { Panel _deviceSpecificContents = null; /// public Panel() : base() { Form frm = this as Form; if(frm == null) { _breakAfter = false; } } /// public override void AddLinkedForms(IList linkedForms) { foreach (Control child in Controls) { if (child is MobileControl) { ((MobileControl)child).AddLinkedForms(linkedForms); } } } /// [ DefaultValue(false), ] public override bool BreakAfter { get { return base.BreakAfter; } set { base.BreakAfter = value; } } /// protected override bool PaginateChildren { get { return Paginate; } } private bool _paginationStateChanged = false; internal bool PaginationStateChanged { get { return _paginationStateChanged; } set { _paginationStateChanged = value; } } /// [ Bindable(true), DefaultValue(false), MobileCategory(SR.Category_Behavior), MobileSysDescription(SR.Panel_Paginate) ] public virtual bool Paginate { get { Object o = ViewState["Paginate"]; return o != null ? (bool)o : false; } set { bool wasPaginating = Paginate; ViewState["Paginate"] = value; if (IsTrackingViewState) { PaginationStateChanged = true; if (value == false && wasPaginating == true ) { SetControlPageRecursive(this, 1); } } } } /// protected override void OnInit(EventArgs e) { base.OnInit(e); if (IsTemplated) { ClearChildViewState(); CreateTemplatedUI(false); ChildControlsCreated = true; } } /// public override void PaginateRecursive(ControlPager pager) { if (!EnablePagination) { return; } if (Paginate && Content != null) { Content.Paginate = true; Content.PaginateRecursive(pager); this.FirstPage = Content.FirstPage; this.LastPage = pager.PageCount; } else { base.PaginateRecursive(pager); } } /// public override void CreateDefaultTemplatedUI(bool doDataBind) { ITemplate contentTemplate = GetTemplate(Constants.ContentTemplateTag); if (contentTemplate != null) { _deviceSpecificContents = new TemplateContainer(); CheckedInstantiateTemplate (contentTemplate, _deviceSpecificContents, this); Controls.AddAt(0, _deviceSpecificContents); } } /// [ Bindable(false), Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public Panel Content { get { return _deviceSpecificContents; } } } /* * Control builder for panels. * * Copyright (c) 2000 Microsoft Corporation */ /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class PanelControlBuilder : LiteralTextContainerControlBuilder { } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Collections; using System.Web.UI; using System.ComponentModel; using System.ComponentModel.Design; using System.Security.Permissions; namespace System.Web.UI.MobileControls { ///[ ControlBuilderAttribute(typeof(PanelControlBuilder)), Designer(typeof(System.Web.UI.Design.MobileControls.PanelDesigner)), DesignerAdapter(typeof(System.Web.UI.MobileControls.Adapters.HtmlPanelAdapter)), PersistChildren(true), ToolboxData("<{0}:Panel runat=\"server\">{0}:Panel>"), ToolboxItem("System.Web.UI.Design.WebControlToolboxItem, " + AssemblyRef.SystemDesign) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class Panel : MobileControl, ITemplateable { Panel _deviceSpecificContents = null; /// public Panel() : base() { Form frm = this as Form; if(frm == null) { _breakAfter = false; } } /// public override void AddLinkedForms(IList linkedForms) { foreach (Control child in Controls) { if (child is MobileControl) { ((MobileControl)child).AddLinkedForms(linkedForms); } } } /// [ DefaultValue(false), ] public override bool BreakAfter { get { return base.BreakAfter; } set { base.BreakAfter = value; } } /// protected override bool PaginateChildren { get { return Paginate; } } private bool _paginationStateChanged = false; internal bool PaginationStateChanged { get { return _paginationStateChanged; } set { _paginationStateChanged = value; } } /// [ Bindable(true), DefaultValue(false), MobileCategory(SR.Category_Behavior), MobileSysDescription(SR.Panel_Paginate) ] public virtual bool Paginate { get { Object o = ViewState["Paginate"]; return o != null ? (bool)o : false; } set { bool wasPaginating = Paginate; ViewState["Paginate"] = value; if (IsTrackingViewState) { PaginationStateChanged = true; if (value == false && wasPaginating == true ) { SetControlPageRecursive(this, 1); } } } } /// protected override void OnInit(EventArgs e) { base.OnInit(e); if (IsTemplated) { ClearChildViewState(); CreateTemplatedUI(false); ChildControlsCreated = true; } } /// public override void PaginateRecursive(ControlPager pager) { if (!EnablePagination) { return; } if (Paginate && Content != null) { Content.Paginate = true; Content.PaginateRecursive(pager); this.FirstPage = Content.FirstPage; this.LastPage = pager.PageCount; } else { base.PaginateRecursive(pager); } } /// public override void CreateDefaultTemplatedUI(bool doDataBind) { ITemplate contentTemplate = GetTemplate(Constants.ContentTemplateTag); if (contentTemplate != null) { _deviceSpecificContents = new TemplateContainer(); CheckedInstantiateTemplate (contentTemplate, _deviceSpecificContents, this); Controls.AddAt(0, _deviceSpecificContents); } } /// [ Bindable(false), Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public Panel Content { get { return _deviceSpecificContents; } } } /* * Control builder for panels. * * Copyright (c) 2000 Microsoft Corporation */ /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class PanelControlBuilder : LiteralTextContainerControlBuilder { } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
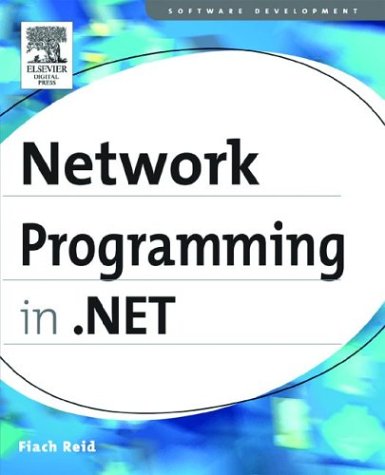
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Parser.cs
- X509CertificateClaimSet.cs
- AssemblyInfo.cs
- MsmqNonTransactedPoisonHandler.cs
- NumberFunctions.cs
- ExtensionSimplifierMarkupObject.cs
- Control.cs
- OperandQuery.cs
- GroupBoxAutomationPeer.cs
- XmlSchemaGroupRef.cs
- SymbolEqualComparer.cs
- AccessDataSourceDesigner.cs
- StylusDownEventArgs.cs
- EntityDesignerDataSourceView.cs
- EndSelectCardRequest.cs
- EraserBehavior.cs
- TypeInitializationException.cs
- FamilyMapCollection.cs
- SafeSecurityHelper.cs
- ButtonColumn.cs
- Rfc2898DeriveBytes.cs
- ToolboxControl.cs
- IPipelineRuntime.cs
- Rect3DConverter.cs
- DocumentSchemaValidator.cs
- LinkedResourceCollection.cs
- DropAnimation.xaml.cs
- ConfigurationException.cs
- UdpDiscoveryEndpoint.cs
- RemoteWebConfigurationHost.cs
- TogglePatternIdentifiers.cs
- RedistVersionInfo.cs
- SByteStorage.cs
- BamlRecordWriter.cs
- NativeMethods.cs
- DescendantQuery.cs
- WizardForm.cs
- DateTimePicker.cs
- HttpRequestMessageProperty.cs
- AccessKeyManager.cs
- entitydatasourceentitysetnameconverter.cs
- ReaderContextStackData.cs
- DSACryptoServiceProvider.cs
- DesignerTextWriter.cs
- EntityObject.cs
- ScrollData.cs
- XmlSerializationReader.cs
- ServiceChannelFactory.cs
- OutOfProcStateClientManager.cs
- ListBox.cs
- DelegatingConfigHost.cs
- PeerNameRegistration.cs
- EventArgs.cs
- Attachment.cs
- KeyboardDevice.cs
- MailAddress.cs
- PasswordTextNavigator.cs
- RegexMatchCollection.cs
- DescriptionAttribute.cs
- MultipleViewPattern.cs
- DynamicValidatorEventArgs.cs
- XmlSubtreeReader.cs
- JsonSerializer.cs
- SelectionPattern.cs
- EventSinkActivity.cs
- ConstNode.cs
- AttributedMetaModel.cs
- loginstatus.cs
- XmlBinaryReaderSession.cs
- MessageParameterAttribute.cs
- DSGeneratorProblem.cs
- InstalledFontCollection.cs
- ZipIORawDataFileBlock.cs
- xdrvalidator.cs
- StreamGeometry.cs
- WindowsRichEdit.cs
- ElementFactory.cs
- Transform.cs
- DbDataSourceEnumerator.cs
- NotifyParentPropertyAttribute.cs
- FieldCollectionEditor.cs
- WS2007HttpBindingCollectionElement.cs
- RefreshPropertiesAttribute.cs
- DataAccessor.cs
- XmlSchemaAttribute.cs
- StringDictionaryEditor.cs
- DependencyPropertyKind.cs
- ValidationRuleCollection.cs
- InkCanvasInnerCanvas.cs
- CodeGotoStatement.cs
- MdiWindowListItemConverter.cs
- BlockCollection.cs
- BindingGroup.cs
- ConnectionsZone.cs
- SwitchAttribute.cs
- ClassicBorderDecorator.cs
- Domain.cs
- EntityClassGenerator.cs
- BinaryUtilClasses.cs
- UrlMappingsSection.cs