Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / InputMethodStateTypeInfo.cs / 1 / InputMethodStateTypeInfo.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: The information for the compartments. // // History: // 07/30/2003 : yutakas - Ported from .net tree. // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Security.Permissions; using System.Windows.Threading; using System.Windows; using MS.Internal; //TextServicesInterop using MS.Utility; using MS.Win32; using System; namespace System.Windows.Input { //----------------------------------------------------- // // InputMethodStateType enum // //----------------------------------------------------- ////// This is an internal. /// This enum identifies the type of input method event. /// internal enum InputMethodStateType { Invalid, ImeState, MicrophoneState, HandwritingState, SpeechMode, ImeConversionModeValues, ImeSentenceModeValues, } internal enum CompartmentScope { Invalid, Thread, Global, } //------------------------------------------------------ // // InputMethodEventTypeInfo class // //----------------------------------------------------- ////// This is an internal. /// This is a holder of compartment type information. /// internal class InputMethodEventTypeInfo { //------------------------------------------------------ // // Constructors // //------------------------------------------------------ internal InputMethodEventTypeInfo( InputMethodStateType type, Guid guid, CompartmentScope scope) { _inputmethodstatetype = type; _guid = guid; _scope = scope; } //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// This converts from GUID for ITfCompartment to InputMethodStateType. /// internal static InputMethodStateType ToType(ref Guid rguid) { for (int i = 0; i < _iminfo.Length; i++) { InputMethodEventTypeInfo im = _iminfo[i]; if (rguid == im._guid) return im._inputmethodstatetype; } Debug.Assert(false, "The guid does not match."); return InputMethodStateType.Invalid; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties internal InputMethodStateType Type {get{return _inputmethodstatetype;}} internal Guid Guid {get{return _guid;}} internal CompartmentScope Scope {get{return _scope;}} internal static InputMethodEventTypeInfo[] InfoList {get{return _iminfo;}} #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private static readonly InputMethodEventTypeInfo _iminfoImeState = new InputMethodEventTypeInfo( InputMethodStateType.ImeState, UnsafeNativeMethods.GUID_COMPARTMENT_KEYBOARD_OPENCLOSE, CompartmentScope.Thread); private static readonly InputMethodEventTypeInfo _iminfoHandwritingState = new InputMethodEventTypeInfo( InputMethodStateType.HandwritingState, UnsafeNativeMethods.GUID_COMPARTMENT_HANDWRITING_OPENCLOSE, CompartmentScope.Thread); private static readonly InputMethodEventTypeInfo _iminfoMicrophoneState = new InputMethodEventTypeInfo( InputMethodStateType.MicrophoneState, UnsafeNativeMethods.GUID_COMPARTMENT_SPEECH_OPENCLOSE, CompartmentScope.Global); private static readonly InputMethodEventTypeInfo _iminfoSpeechMode = new InputMethodEventTypeInfo( InputMethodStateType.SpeechMode, UnsafeNativeMethods.GUID_COMPARTMENT_SPEECH_GLOBALSTATE, CompartmentScope.Global); private static readonly InputMethodEventTypeInfo _iminfoImeConversionMode = new InputMethodEventTypeInfo( InputMethodStateType.ImeConversionModeValues, UnsafeNativeMethods.GUID_COMPARTMENT_KEYBOARD_INPUTMODE_CONVERSION, CompartmentScope.Thread); private static readonly InputMethodEventTypeInfo _iminfoImeSentenceMode = new InputMethodEventTypeInfo( InputMethodStateType.ImeSentenceModeValues, UnsafeNativeMethods.GUID_COMPARTMENT_KEYBOARD_INPUTMODE_SENTENCE, CompartmentScope.Thread); private static readonly InputMethodEventTypeInfo[] _iminfo = new InputMethodEventTypeInfo[] { _iminfoImeState, _iminfoHandwritingState, _iminfoMicrophoneState, _iminfoSpeechMode, _iminfoImeConversionMode, _iminfoImeSentenceMode}; private InputMethodStateType _inputmethodstatetype; private Guid _guid; private CompartmentScope _scope; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: The information for the compartments. // // History: // 07/30/2003 : yutakas - Ported from .net tree. // //--------------------------------------------------------------------------- using System.Runtime.InteropServices; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Security.Permissions; using System.Windows.Threading; using System.Windows; using MS.Internal; //TextServicesInterop using MS.Utility; using MS.Win32; using System; namespace System.Windows.Input { //----------------------------------------------------- // // InputMethodStateType enum // //----------------------------------------------------- ////// This is an internal. /// This enum identifies the type of input method event. /// internal enum InputMethodStateType { Invalid, ImeState, MicrophoneState, HandwritingState, SpeechMode, ImeConversionModeValues, ImeSentenceModeValues, } internal enum CompartmentScope { Invalid, Thread, Global, } //------------------------------------------------------ // // InputMethodEventTypeInfo class // //----------------------------------------------------- ////// This is an internal. /// This is a holder of compartment type information. /// internal class InputMethodEventTypeInfo { //------------------------------------------------------ // // Constructors // //------------------------------------------------------ internal InputMethodEventTypeInfo( InputMethodStateType type, Guid guid, CompartmentScope scope) { _inputmethodstatetype = type; _guid = guid; _scope = scope; } //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// This converts from GUID for ITfCompartment to InputMethodStateType. /// internal static InputMethodStateType ToType(ref Guid rguid) { for (int i = 0; i < _iminfo.Length; i++) { InputMethodEventTypeInfo im = _iminfo[i]; if (rguid == im._guid) return im._inputmethodstatetype; } Debug.Assert(false, "The guid does not match."); return InputMethodStateType.Invalid; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties internal InputMethodStateType Type {get{return _inputmethodstatetype;}} internal Guid Guid {get{return _guid;}} internal CompartmentScope Scope {get{return _scope;}} internal static InputMethodEventTypeInfo[] InfoList {get{return _iminfo;}} #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private static readonly InputMethodEventTypeInfo _iminfoImeState = new InputMethodEventTypeInfo( InputMethodStateType.ImeState, UnsafeNativeMethods.GUID_COMPARTMENT_KEYBOARD_OPENCLOSE, CompartmentScope.Thread); private static readonly InputMethodEventTypeInfo _iminfoHandwritingState = new InputMethodEventTypeInfo( InputMethodStateType.HandwritingState, UnsafeNativeMethods.GUID_COMPARTMENT_HANDWRITING_OPENCLOSE, CompartmentScope.Thread); private static readonly InputMethodEventTypeInfo _iminfoMicrophoneState = new InputMethodEventTypeInfo( InputMethodStateType.MicrophoneState, UnsafeNativeMethods.GUID_COMPARTMENT_SPEECH_OPENCLOSE, CompartmentScope.Global); private static readonly InputMethodEventTypeInfo _iminfoSpeechMode = new InputMethodEventTypeInfo( InputMethodStateType.SpeechMode, UnsafeNativeMethods.GUID_COMPARTMENT_SPEECH_GLOBALSTATE, CompartmentScope.Global); private static readonly InputMethodEventTypeInfo _iminfoImeConversionMode = new InputMethodEventTypeInfo( InputMethodStateType.ImeConversionModeValues, UnsafeNativeMethods.GUID_COMPARTMENT_KEYBOARD_INPUTMODE_CONVERSION, CompartmentScope.Thread); private static readonly InputMethodEventTypeInfo _iminfoImeSentenceMode = new InputMethodEventTypeInfo( InputMethodStateType.ImeSentenceModeValues, UnsafeNativeMethods.GUID_COMPARTMENT_KEYBOARD_INPUTMODE_SENTENCE, CompartmentScope.Thread); private static readonly InputMethodEventTypeInfo[] _iminfo = new InputMethodEventTypeInfo[] { _iminfoImeState, _iminfoHandwritingState, _iminfoMicrophoneState, _iminfoSpeechMode, _iminfoImeConversionMode, _iminfoImeSentenceMode}; private InputMethodStateType _inputmethodstatetype; private Guid _guid; private CompartmentScope _scope; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
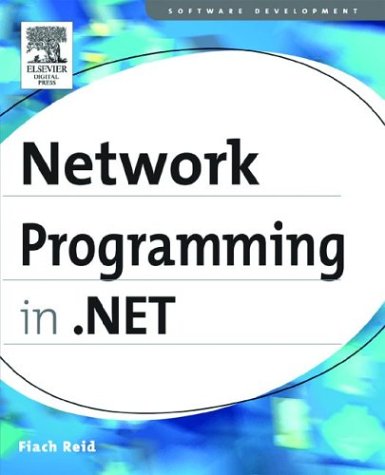
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberListBinding.cs
- StringUtil.cs
- WorkflowInstanceContextProvider.cs
- TextSelectionHelper.cs
- GridPattern.cs
- ELinqQueryState.cs
- ParsedAttributeCollection.cs
- WindowsStatusBar.cs
- PenContext.cs
- LinkedDataMemberFieldEditor.cs
- SiteMapPath.cs
- ObjectAssociationEndMapping.cs
- DbXmlEnabledProviderManifest.cs
- ValidateNames.cs
- Int32Storage.cs
- PenLineCapValidation.cs
- EditorPartCollection.cs
- ProfileSettingsCollection.cs
- MatrixAnimationUsingKeyFrames.cs
- XmlCDATASection.cs
- Regex.cs
- TemplateXamlParser.cs
- ZeroOpNode.cs
- WriterOutput.cs
- ServiceHostingEnvironmentSection.cs
- LinkedResourceCollection.cs
- XmlHelper.cs
- VScrollBar.cs
- PeerToPeerException.cs
- StrongNameIdentityPermission.cs
- DataGridBoolColumn.cs
- ClientProxyGenerator.cs
- SettingsBindableAttribute.cs
- ClientConfigurationSystem.cs
- MemberListBinding.cs
- LocatorPartList.cs
- CreateUserErrorEventArgs.cs
- ToolStripSystemRenderer.cs
- XXXOnTypeBuilderInstantiation.cs
- SystemInfo.cs
- XsltInput.cs
- TextSelectionHighlightLayer.cs
- HtmlForm.cs
- ClientFormsAuthenticationMembershipProvider.cs
- ChainedAsyncResult.cs
- ColorConverter.cs
- SelectionChangedEventArgs.cs
- ServiceDescriptionData.cs
- CompositeDesignerAccessibleObject.cs
- VirtualDirectoryMapping.cs
- RadioButtonRenderer.cs
- TextTreeTextElementNode.cs
- CompositeControl.cs
- CodeDomComponentSerializationService.cs
- UnknownBitmapEncoder.cs
- Timer.cs
- OutOfMemoryException.cs
- GZipDecoder.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- DataColumnMappingCollection.cs
- TypeElement.cs
- ContextTokenTypeConverter.cs
- GroupByQueryOperator.cs
- FilterException.cs
- WindowsAuthenticationEventArgs.cs
- SyntaxCheck.cs
- AQNBuilder.cs
- StructuredTypeInfo.cs
- BinaryWriter.cs
- ColumnHeaderConverter.cs
- MarshalDirectiveException.cs
- BuildProviderCollection.cs
- ContextQuery.cs
- CompressionTransform.cs
- CreateUserWizardStep.cs
- InfoCardArgumentException.cs
- nulltextcontainer.cs
- PreservationFileReader.cs
- ListViewInsertionMark.cs
- ContextMenuService.cs
- ToolStripRenderer.cs
- _ConnectOverlappedAsyncResult.cs
- PlacementWorkspace.cs
- MemberDomainMap.cs
- Label.cs
- XdrBuilder.cs
- PeerPresenceInfo.cs
- RtfToXamlReader.cs
- RedistVersionInfo.cs
- TemplateXamlParser.cs
- RotateTransform3D.cs
- PeerNodeAddress.cs
- JavaScriptObjectDeserializer.cs
- LinearKeyFrames.cs
- CommandConverter.cs
- XmlWriterTraceListener.cs
- BitmapPalettes.cs
- XmlBoundElement.cs
- ADMembershipUser.cs
- ObfuscationAttribute.cs