Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / ConditionalExpression.cs / 1305376 / ConditionalExpression.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Dynamic.Utils; using System.Diagnostics; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Represents an expression that has a conditional operator. /// #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.ConditionalExpressionProxy))] #endif public class ConditionalExpression : Expression { private readonly Expression _test; private readonly Expression _true; internal ConditionalExpression(Expression test, Expression ifTrue) { _test = test; _true = ifTrue; } internal static ConditionalExpression Make(Expression test, Expression ifTrue, Expression ifFalse, Type type) { if (ifTrue.Type != type || ifFalse.Type != type) { return new FullConditionalExpressionWithType(test, ifTrue, ifFalse, type); } if (ifFalse is DefaultExpression && ifFalse.Type == typeof(void)) { return new ConditionalExpression(test, ifTrue); } else { return new FullConditionalExpression(test, ifTrue, ifFalse); } } ////// Returns the node type of this Expression. Extension nodes should return /// ExpressionType.Extension when overriding this method. /// ///The public sealed override ExpressionType NodeType { get { return ExpressionType.Conditional; } } ///of the expression. /// Gets the static type of the expression that this ///represents. /// The public override Type Type { get { return IfTrue.Type; } } ///that represents the static type of the expression. /// Gets the test of the conditional operation. /// public Expression Test { get { return _test; } } ////// Gets the expression to execute if the test evaluates to true. /// public Expression IfTrue { get { return _true; } } ////// Gets the expression to execute if the test evaluates to false. /// public Expression IfFalse { get { return GetFalse(); } } internal virtual Expression GetFalse() { return Expression.Empty(); } ////// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitConditional(this); } ////// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// The property of the result. /// The property of the result. /// This expression if no children changed, or an expression with the updated children. public ConditionalExpression Update(Expression test, Expression ifTrue, Expression ifFalse) { if (test == Test && ifTrue == IfTrue && ifFalse == IfFalse) { return this; } return Expression.Condition(test, ifTrue, ifFalse, Type); } } internal class FullConditionalExpression : ConditionalExpression { private readonly Expression _false; internal FullConditionalExpression(Expression test, Expression ifTrue, Expression ifFalse) : base(test, ifTrue) { _false = ifFalse; } internal override Expression GetFalse() { return _false; } } internal class FullConditionalExpressionWithType : FullConditionalExpression { private readonly Type _type; internal FullConditionalExpressionWithType(Expression test, Expression ifTrue, Expression ifFalse, Type type) : base(test, ifTrue, ifFalse) { _type = type; } public sealed override Type Type { get { return _type; } } } public partial class Expression { ////// Creates a /// An. /// to set the property equal to. /// An to set the property equal to. /// An to set the property equal to. /// A public static ConditionalExpression Condition(Expression test, Expression ifTrue, Expression ifFalse) { RequiresCanRead(test, "test"); RequiresCanRead(ifTrue, "ifTrue"); RequiresCanRead(ifFalse, "ifFalse"); if (test.Type != typeof(bool)) { throw Error.ArgumentMustBeBoolean(); } if (!TypeUtils.AreEquivalent(ifTrue.Type, ifFalse.Type)) { throw Error.ArgumentTypesMustMatch(); } return ConditionalExpression.Make(test, ifTrue, ifFalse, ifTrue.Type); } ///that has the property equal to /// and the , , /// and properties set to the specified values. /// Creates a /// An. /// to set the property equal to. /// An to set the property equal to. /// An to set the property equal to. /// A to set the property equal to. /// A ///that has the property equal to /// and the , , /// and properties set to the specified values. This method allows explicitly unifying the result type of the conditional expression in cases where the types of public static ConditionalExpression Condition(Expression test, Expression ifTrue, Expression ifFalse, Type type) { RequiresCanRead(test, "test"); RequiresCanRead(ifTrue, "ifTrue"); RequiresCanRead(ifFalse, "ifFalse"); ContractUtils.RequiresNotNull(type, "type"); if (test.Type != typeof(bool)) { throw Error.ArgumentMustBeBoolean(); } if (type != typeof(void)) { if (!TypeUtils.AreReferenceAssignable(type, ifTrue.Type) || !TypeUtils.AreReferenceAssignable(type, ifFalse.Type)) { throw Error.ArgumentTypesMustMatch(); } } return ConditionalExpression.Make(test, ifTrue, ifFalse, type); } ////// and expressions are not equal. Types of both and must be implicitly /// reference assignable to the result type. The is allowed to be . /// Creates a /// An. /// to set the property equal to. /// An to set the property equal to. /// A public static ConditionalExpression IfThen(Expression test, Expression ifTrue) { return Condition(test, ifTrue, Expression.Empty(), typeof(void)); } ///that has the property equal to /// and the , , /// properties set to the specified values. The property is set to default expression and /// the type of the resulting returned by this method is . /// Creates a /// An. /// to set the property equal to. /// An to set the property equal to. /// An to set the property equal to. /// A public static ConditionalExpression IfThenElse(Expression test, Expression ifTrue, Expression ifFalse) { return Condition(test, ifTrue, ifFalse, typeof(void)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Dynamic.Utils; using System.Diagnostics; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ///that has the property equal to /// and the , , /// and properties set to the specified values. The type of the resulting /// returned by this method is . /// Represents an expression that has a conditional operator. /// #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.ConditionalExpressionProxy))] #endif public class ConditionalExpression : Expression { private readonly Expression _test; private readonly Expression _true; internal ConditionalExpression(Expression test, Expression ifTrue) { _test = test; _true = ifTrue; } internal static ConditionalExpression Make(Expression test, Expression ifTrue, Expression ifFalse, Type type) { if (ifTrue.Type != type || ifFalse.Type != type) { return new FullConditionalExpressionWithType(test, ifTrue, ifFalse, type); } if (ifFalse is DefaultExpression && ifFalse.Type == typeof(void)) { return new ConditionalExpression(test, ifTrue); } else { return new FullConditionalExpression(test, ifTrue, ifFalse); } } ////// Returns the node type of this Expression. Extension nodes should return /// ExpressionType.Extension when overriding this method. /// ///The public sealed override ExpressionType NodeType { get { return ExpressionType.Conditional; } } ///of the expression. /// Gets the static type of the expression that this ///represents. /// The public override Type Type { get { return IfTrue.Type; } } ///that represents the static type of the expression. /// Gets the test of the conditional operation. /// public Expression Test { get { return _test; } } ////// Gets the expression to execute if the test evaluates to true. /// public Expression IfTrue { get { return _true; } } ////// Gets the expression to execute if the test evaluates to false. /// public Expression IfFalse { get { return GetFalse(); } } internal virtual Expression GetFalse() { return Expression.Empty(); } ////// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitConditional(this); } ////// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// The property of the result. /// The property of the result. /// This expression if no children changed, or an expression with the updated children. public ConditionalExpression Update(Expression test, Expression ifTrue, Expression ifFalse) { if (test == Test && ifTrue == IfTrue && ifFalse == IfFalse) { return this; } return Expression.Condition(test, ifTrue, ifFalse, Type); } } internal class FullConditionalExpression : ConditionalExpression { private readonly Expression _false; internal FullConditionalExpression(Expression test, Expression ifTrue, Expression ifFalse) : base(test, ifTrue) { _false = ifFalse; } internal override Expression GetFalse() { return _false; } } internal class FullConditionalExpressionWithType : FullConditionalExpression { private readonly Type _type; internal FullConditionalExpressionWithType(Expression test, Expression ifTrue, Expression ifFalse, Type type) : base(test, ifTrue, ifFalse) { _type = type; } public sealed override Type Type { get { return _type; } } } public partial class Expression { ////// Creates a /// An. /// to set the property equal to. /// An to set the property equal to. /// An to set the property equal to. /// A public static ConditionalExpression Condition(Expression test, Expression ifTrue, Expression ifFalse) { RequiresCanRead(test, "test"); RequiresCanRead(ifTrue, "ifTrue"); RequiresCanRead(ifFalse, "ifFalse"); if (test.Type != typeof(bool)) { throw Error.ArgumentMustBeBoolean(); } if (!TypeUtils.AreEquivalent(ifTrue.Type, ifFalse.Type)) { throw Error.ArgumentTypesMustMatch(); } return ConditionalExpression.Make(test, ifTrue, ifFalse, ifTrue.Type); } ///that has the property equal to /// and the , , /// and properties set to the specified values. /// Creates a /// An. /// to set the property equal to. /// An to set the property equal to. /// An to set the property equal to. /// A to set the property equal to. /// A ///that has the property equal to /// and the , , /// and properties set to the specified values. This method allows explicitly unifying the result type of the conditional expression in cases where the types of public static ConditionalExpression Condition(Expression test, Expression ifTrue, Expression ifFalse, Type type) { RequiresCanRead(test, "test"); RequiresCanRead(ifTrue, "ifTrue"); RequiresCanRead(ifFalse, "ifFalse"); ContractUtils.RequiresNotNull(type, "type"); if (test.Type != typeof(bool)) { throw Error.ArgumentMustBeBoolean(); } if (type != typeof(void)) { if (!TypeUtils.AreReferenceAssignable(type, ifTrue.Type) || !TypeUtils.AreReferenceAssignable(type, ifFalse.Type)) { throw Error.ArgumentTypesMustMatch(); } } return ConditionalExpression.Make(test, ifTrue, ifFalse, type); } ////// and expressions are not equal. Types of both and must be implicitly /// reference assignable to the result type. The is allowed to be . /// Creates a /// An. /// to set the property equal to. /// An to set the property equal to. /// A public static ConditionalExpression IfThen(Expression test, Expression ifTrue) { return Condition(test, ifTrue, Expression.Empty(), typeof(void)); } ///that has the property equal to /// and the , , /// properties set to the specified values. The property is set to default expression and /// the type of the resulting returned by this method is . /// Creates a /// An. /// to set the property equal to. /// An to set the property equal to. /// An to set the property equal to. /// A public static ConditionalExpression IfThenElse(Expression test, Expression ifTrue, Expression ifFalse) { return Condition(test, ifTrue, ifFalse, typeof(void)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.that has the property equal to /// and the , , /// and properties set to the specified values. The type of the resulting /// returned by this method is .
Link Menu
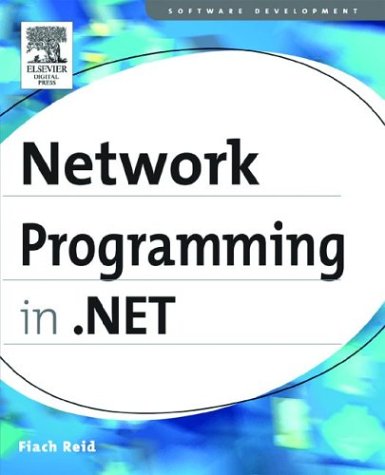
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmtpAuthenticationManager.cs
- AccessibleObject.cs
- ModifierKeysValueSerializer.cs
- ChangeConflicts.cs
- CredentialCache.cs
- ModifierKeysValueSerializer.cs
- HintTextMaxWidthConverter.cs
- StyleReferenceConverter.cs
- WorkflowTransactionOptions.cs
- Empty.cs
- RtfToXamlLexer.cs
- TypeReference.cs
- CookieProtection.cs
- XmlSchemaObjectTable.cs
- XmlLanguage.cs
- _Semaphore.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- TextSimpleMarkerProperties.cs
- ParameterCollection.cs
- ReachDocumentPageSerializer.cs
- PeerNameRecordCollection.cs
- CompositeTypefaceMetrics.cs
- AssemblyAssociatedContentFileAttribute.cs
- SrgsElementFactory.cs
- BindingOperations.cs
- XmlDocument.cs
- ListViewTableCell.cs
- WorkflowElementDialog.cs
- ResourceContainer.cs
- ComMethodElement.cs
- PresentationAppDomainManager.cs
- SystemResourceKey.cs
- HotSpot.cs
- ScrollEventArgs.cs
- HierarchicalDataTemplate.cs
- MD5.cs
- FilteredXmlReader.cs
- WebPartPersonalization.cs
- TransformerTypeCollection.cs
- XmlSerializationGeneratedCode.cs
- WeakEventTable.cs
- PointF.cs
- CodePrimitiveExpression.cs
- ColorConverter.cs
- UIElement.cs
- TimeZone.cs
- DateTimeUtil.cs
- DPCustomTypeDescriptor.cs
- NativeMethods.cs
- TagPrefixAttribute.cs
- LongValidator.cs
- LogEntrySerializer.cs
- WindowsScrollBarBits.cs
- ActionFrame.cs
- TaskHelper.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- Rethrow.cs
- _AuthenticationState.cs
- StorageEntityContainerMapping.cs
- DataGridClipboardHelper.cs
- ListViewDeletedEventArgs.cs
- TreeNode.cs
- TextTreeText.cs
- UdpChannelListener.cs
- X509ServiceCertificateAuthenticationElement.cs
- NetworkInformationPermission.cs
- SqlFactory.cs
- OracleEncoding.cs
- COM2PropertyPageUITypeConverter.cs
- FontStyle.cs
- CodeParameterDeclarationExpressionCollection.cs
- MenuItemCollectionEditorDialog.cs
- ColorMatrix.cs
- WebPartRestoreVerb.cs
- CommandField.cs
- TypeReference.cs
- DataSpaceManager.cs
- SettingsPropertyWrongTypeException.cs
- SQLDateTime.cs
- _HTTPDateParse.cs
- IRCollection.cs
- XmlAggregates.cs
- Pair.cs
- ExecutedRoutedEventArgs.cs
- XmlEntityReference.cs
- OpCellTreeNode.cs
- MimeMapping.cs
- LinqDataSourceUpdateEventArgs.cs
- ArcSegment.cs
- BaseParaClient.cs
- TypedReference.cs
- ConfigurationStrings.cs
- DecimalAnimationBase.cs
- IndicCharClassifier.cs
- HtmlControl.cs
- FontDifferentiator.cs
- AbstractExpressions.cs
- DragDeltaEventArgs.cs
- InheritablePropertyChangeInfo.cs
- UmAlQuraCalendar.cs